Topic 4 Lesson 1
Summary
TLDRThis video script explores Python's list type, focusing on its mutability and ordered sequence of values. It discusses list manipulation, element distinctness, and list comprehension. The script also highlights the need for storing and manipulating data in memory, contrasting scalar types with the list's ability to handle complex data structures.
Takeaways
- 📚 The lesson introduces lists, tuples, and strings from chapter five of Guttag's book, highlighting their importance in Python programming.
- 🔍 While basic problems can be solved without storing sequences in memory, more complex tasks like reversing a sequence or checking for distinct elements require memory storage and manipulation.
- 🔄 The list type in Python is a mutable, ordered sequence of values that can be modified, unlike scalar types previously discussed.
- 📝 Lists are initialized using brackets and commas to separate values, and each value is identified by an index starting from zero.
- 🔑 The indexing operator, indicated by brackets, allows for both reading and writing access to the list entries, enabling direct modification of list contents.
- 🔢 The built-in `len()` function provides the length of a list, which is crucial for iterating through list elements.
- 🗃️ Lists are stored in contiguous memory cells to allow for fast direct access, facilitated by Python's implementation using pointers to objects rather than the objects themselves.
- 🔠 The script explains the difference between homogeneous lists, where all elements are of the same type, and non-homogeneous lists with mixed types.
- 📉 The need for lists arises in solving problems that involve reversing a sequence, checking for distinct elements, and sorting, which are not possible with scalar types alone.
- 📈 The script uses the Code Visualizer to demonstrate the memory representation of lists and how indices map to specific elements within the list.
- 📝 Lists in Python are implemented with pointers to allow for different data types within the same list, maintaining the efficiency of direct access.
Q & A
What are the main topics covered in the script?
-The script covers Lists, Tuples, and strings, including the list type, manipulating lists, the element distinctness problem, list copy, tuples, lists and tuples with mutable objects, sequences, and list comprehension.
What is the significance of the list type in Python for solving certain problems?
-The list type in Python is significant for solving problems that require storing and manipulating user input, such as reversing a sequence, checking for distinct elements, and sorting.
How does the script illustrate the concept of mutable and immutable data types?
-The script illustrates this by explaining that lists are mutable, meaning they can be modified, in contrast to tuples which are immutable.
What is the purpose of the element distinctness problem mentioned in the script?
-The element distinctness problem is an application of lists to check if all elements in a sequence are unique, which is not possible with scalar types alone.
How does the script explain the concept of direct access in lists?
-The script explains that direct access in lists is very fast because Python implements lists using contiguous memory cells by storing pointers to objects instead of the objects themselves.
What is the difference between homogeneous and non-homogeneous lists as explained in the script?
-Homogeneous lists contain elements of the same type, while non-homogeneous lists contain elements of mixed types.
How does the script demonstrate the initialization of a list in Python?
-The script demonstrates list initialization by using the assignment operator and brackets to define a list with values separated by commas.
What is the role of the indexing operator in accessing elements of a list?
-The indexing operator, indicated by brackets, is used to read from or write to a specific element in the list by referring to its index.
What built-in function is used to determine the length of a list in Python?
-The built-in function 'len()' is used to determine the length of a list in Python.
How does the script explain the implementation of lists in Python at a low level?
-The script explains that Python implements lists using contiguous memory cells and stores pointers to objects rather than the objects themselves to allow for direct access while accommodating different object sizes.
What is the significance of the list comprehension feature in Python?
-List comprehension is a feature in Python that allows for the creation of lists in a concise and readable way, typically used for transforming or filtering elements from an iterable.
Outlines
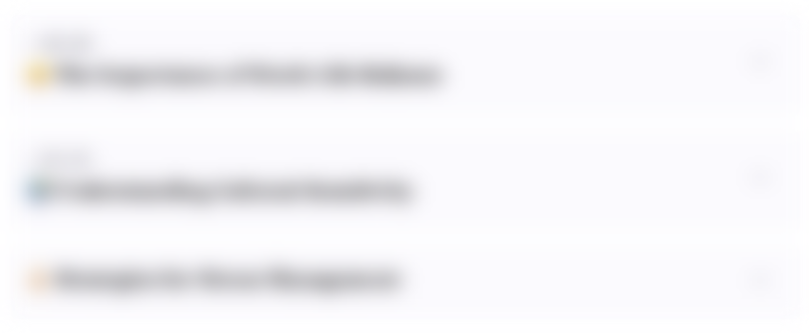
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
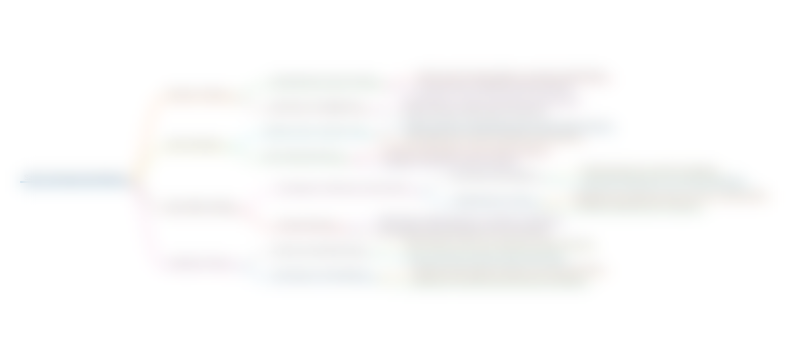
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
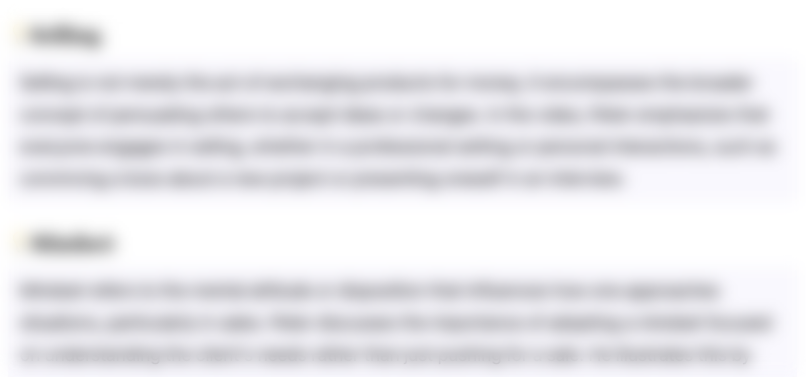
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
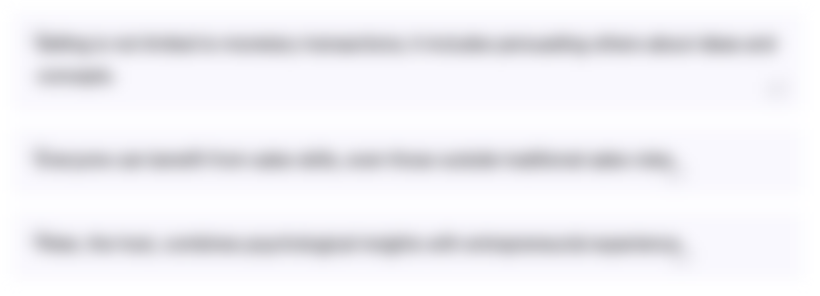
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
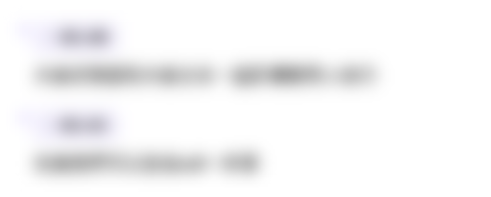
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
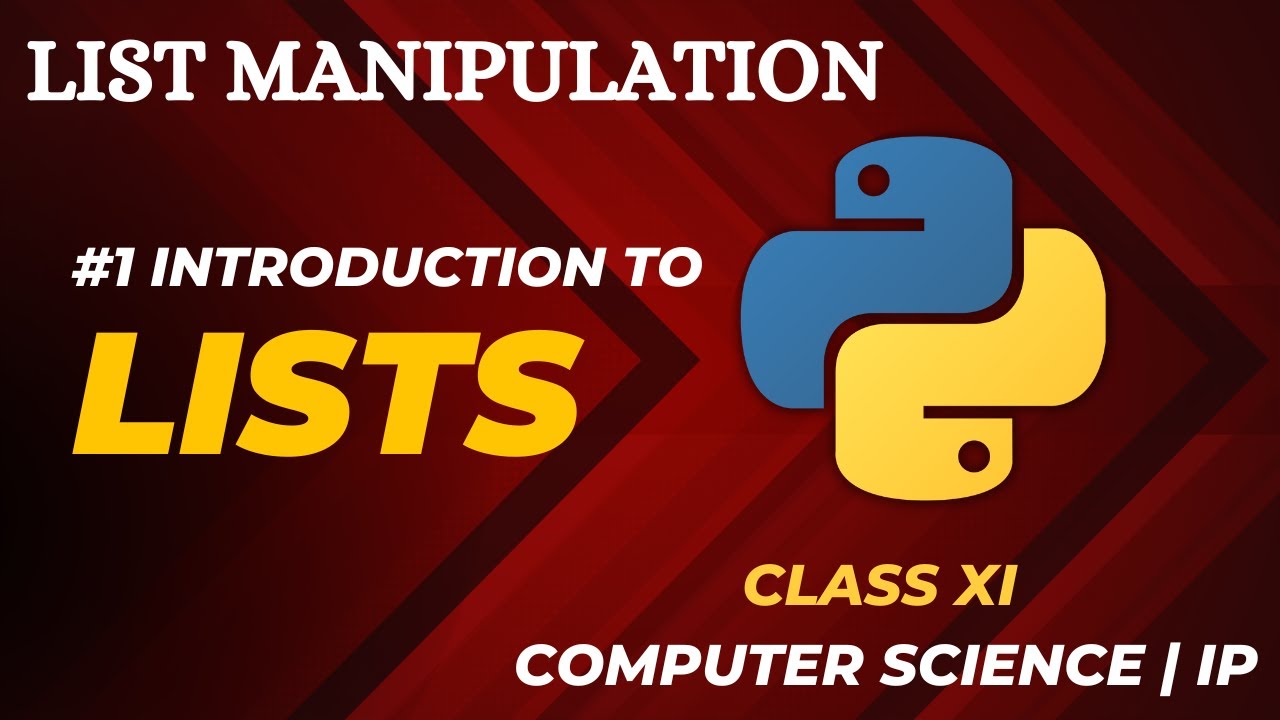
#1 Introduction to Lists | List Manipulation | Class 11 CBSE Computer Science and IP
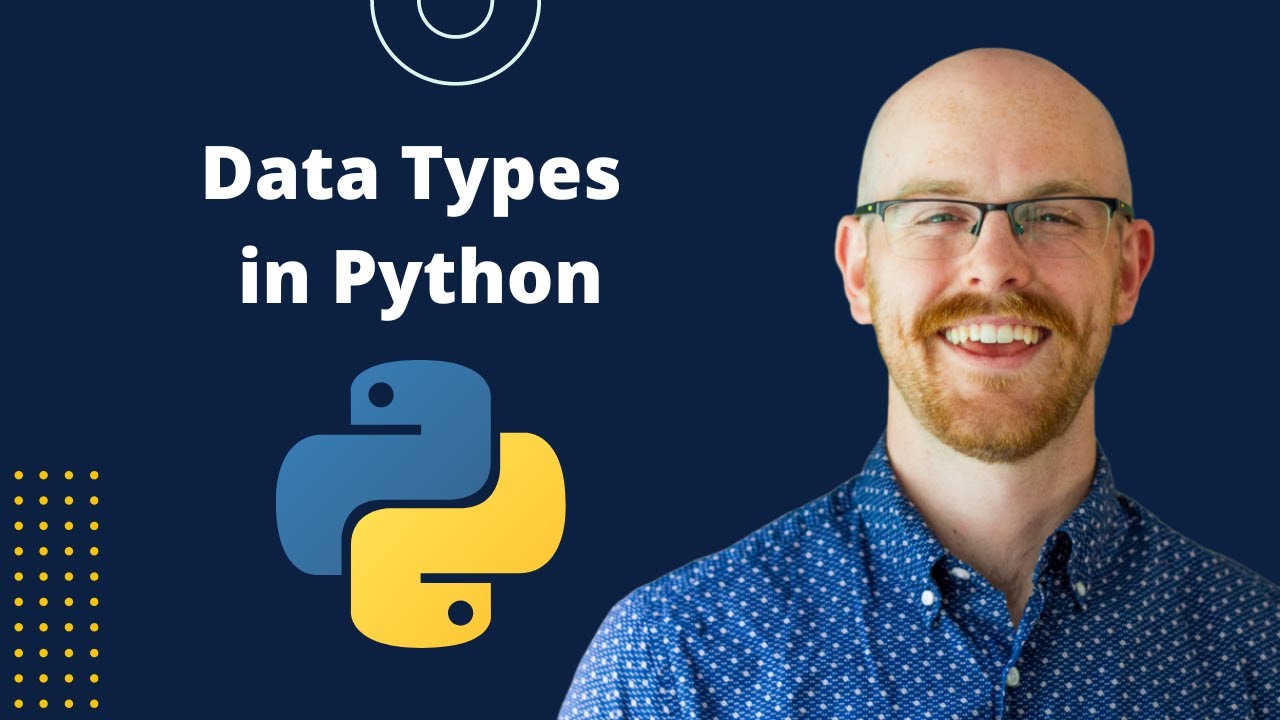
Data Types in Python | Python for Beginners

How variables works in Python | Explained with Animations
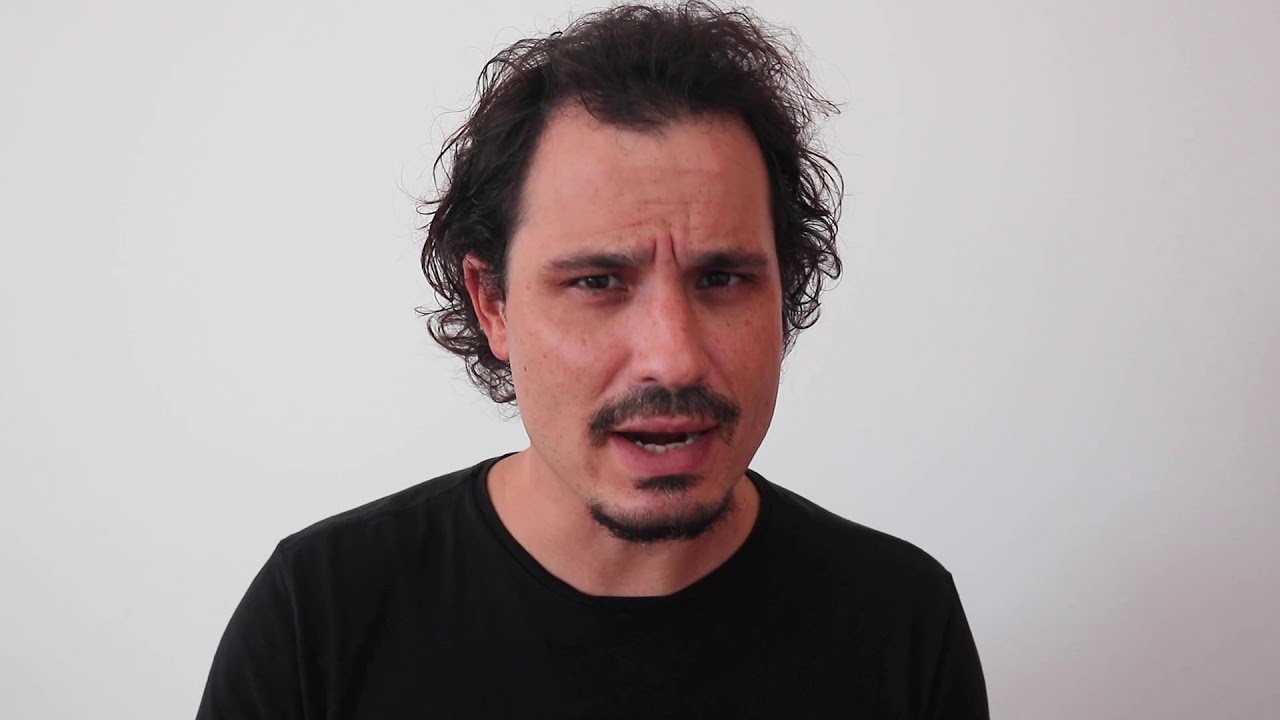
EDB1 IMD UFRN (2020.6): Ordenação por Bolha
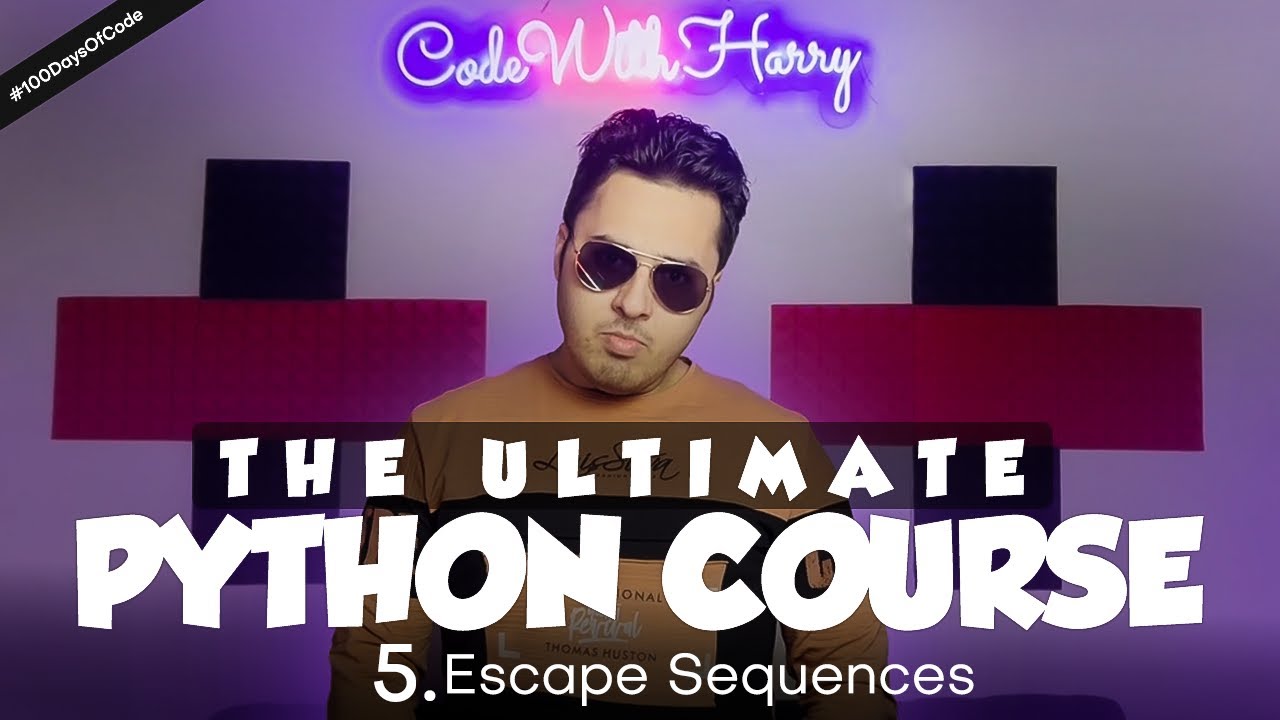
Comments, Escape Sequences & Print Statement | Python Tutorial - Day #5
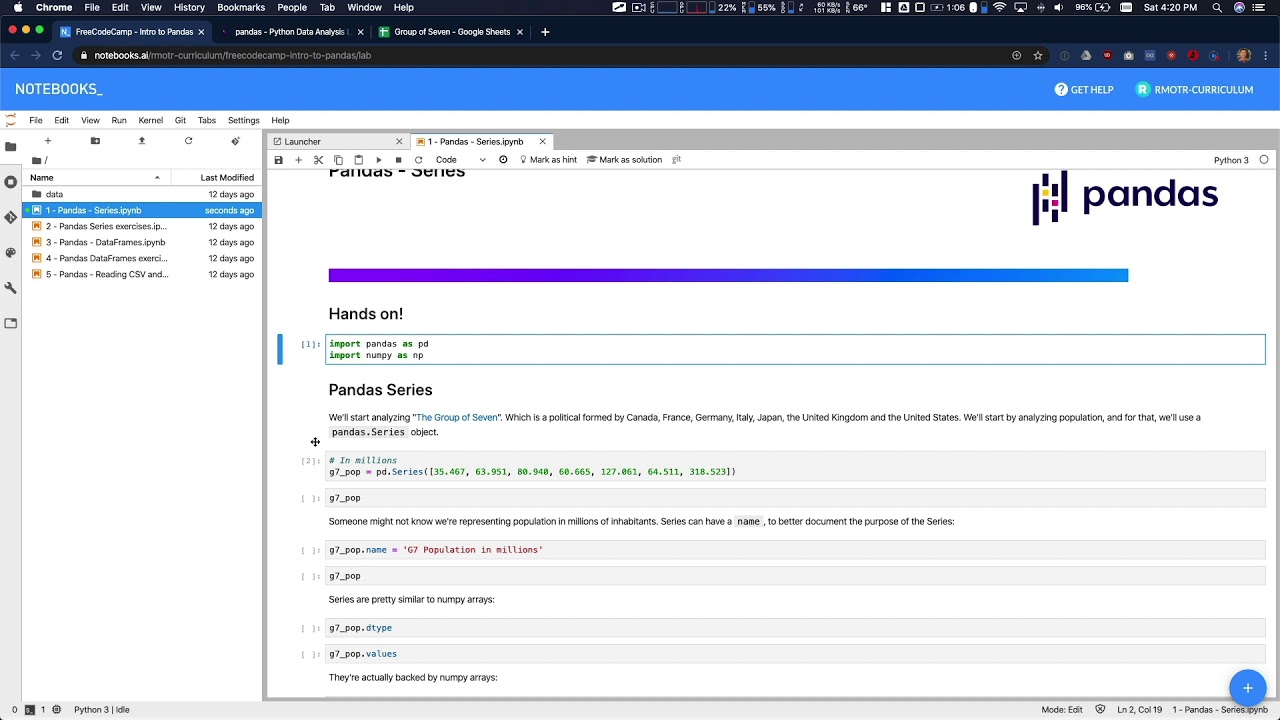
Pandas Introduction - Data Analysis with Python Course
5.0 / 5 (0 votes)