#25 C Pointers and Functions | C Programming For Beginners
Summary
TLDRIn this video, the instructor explores the use of pointers with functions in C programming. The tutorial covers passing pointer variables as function arguments, modifying values at memory addresses, and returning pointer values from functions. Examples include finding the square of a number and summing two integers using pointers. Additionally, viewers are assigned a task to create a multiplication function using pointers. The video emphasizes hands-on learning, offering resources like quizzes, challenges, and a GitHub repository for further practice. Engagement is encouraged through likes, comments, and subscriptions.
Takeaways
- 📌 Pointers in C can be used to pass arguments to functions, allowing the function to modify the value of a variable in the calling scope.
- 🔢 A function can accept a pointer as an argument and change the value it points to, demonstrating how pointers can affect data outside their scope.
- 💡 The script provides an example of a function that calculates the square of a number using a pointer to modify the original variable's value.
- 👨🏫 The tutorial explains how to use pointers to find the square of a number, emphasizing the use of pointer syntax within functions.
- 🎯 It showcases a function that returns a pointer to an integer, illustrating how functions can return addresses in addition to values.
- 🛠️ The script includes a practical example where a function adds two numbers using pointers and returns the sum through a pointer.
- 📚 The video encourages viewers to practice programming with quizzes and challenges through the introduction of a learning platform called 'Programmies Pro'.
- 🔄 The tutorial demonstrates the concept of returning a pointer from a function and how to use the returned pointer to access the result.
- 📈 The script motivates engagement by asking viewers to like, comment, and subscribe to support free content creation.
- 📝 A programming task is provided at the end of the script to reinforce learning, involving creating a function that multiplies two numbers using pointers.
- 🎉 The video concludes with a question to engage viewers, asking them to comment with the return type of a certain function.
Q & A
What is the purpose of using pointers with functions in C programming?
-Pointers allow functions to directly modify the values of variables by passing their memory addresses, enabling more efficient memory use and manipulation of data.
In the example where a pointer is passed to a function, how is the value of a variable changed?
-The address of the variable is passed to the function, and inside the function, the value at the memory address is changed using the pointer. This results in the original variable's value being modified outside the function.
What does the `find_square` function do in the video example?
-The `find_square` function calculates the square of a number by accepting a pointer to an integer, multiplying the value pointed by the pointer with itself, and then updating the original value with the square.
How can a function return a pointer in C?
-A function can return a pointer by changing its return type to a pointer type (e.g., `int*` for an integer pointer) and using the `return` statement to return the pointer. The returned pointer can then be used to access or modify the data stored at that memory address.
Why is it necessary to use an asterisk (*) before a pointer variable inside a function?
-The asterisk (*) dereferences the pointer, which means it accesses the value stored at the memory address the pointer points to. Without dereferencing, the function would only access the memory address, not the actual data.
What is the difference between passing a regular variable and a pointer variable to a function in C?
-When passing a regular variable, the function receives a copy of the variable's value, so changes made inside the function don't affect the original variable. When passing a pointer, the function receives the memory address, allowing it to modify the original variable directly.
In the last example, how does the function add two numbers using pointers?
-The function `add_number` accepts three pointers: one for each number and one for storing the sum. It computes the sum by dereferencing the first two pointers, adds their values, and stores the result in the location pointed by the third pointer.
How is the result of a function that returns a pointer printed in the video example?
-The result is printed by dereferencing the pointer returned by the function, using an asterisk (*) before the pointer variable in the `printf` statement.
What are the advantages of using pointers when returning values from functions?
-Using pointers to return values allows functions to return more than one value and modify variables directly, enabling efficient memory use and bypassing the need to return copies of large data structures.
What task is given to viewers at the end of the video?
-Viewers are asked to create a program that multiplies two numbers using a function and pointers. The function should accept three pointers, multiply the values pointed to by the first two, and store the result in the location pointed to by the third pointer.
Outlines
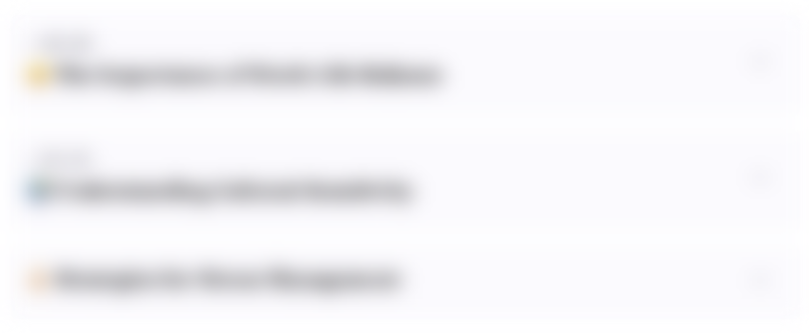
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنMindmap
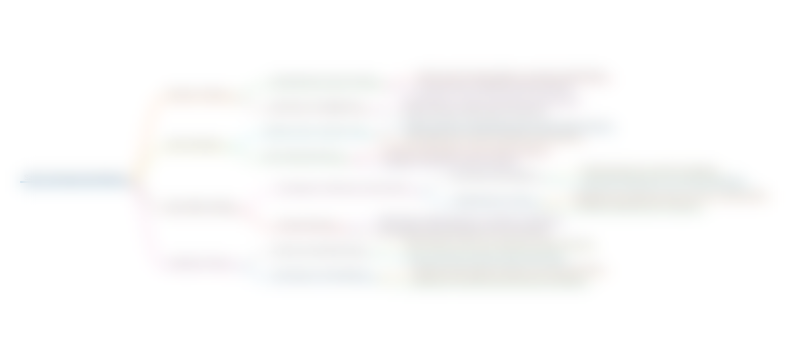
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنKeywords
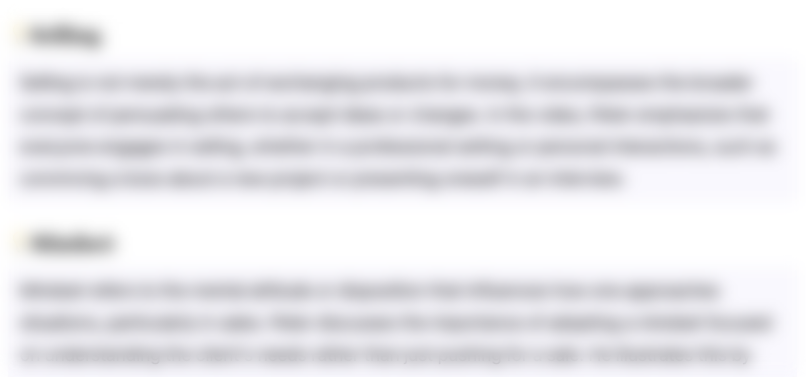
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنHighlights
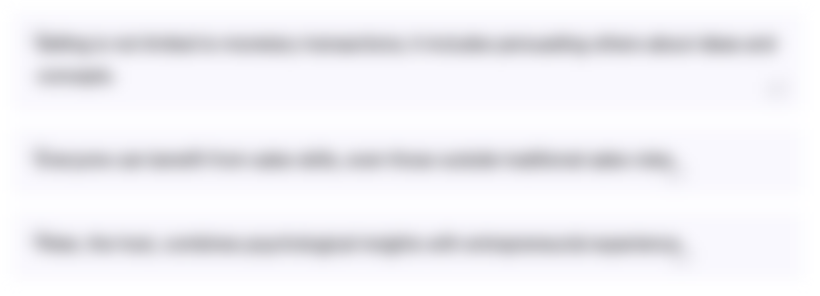
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنTranscripts
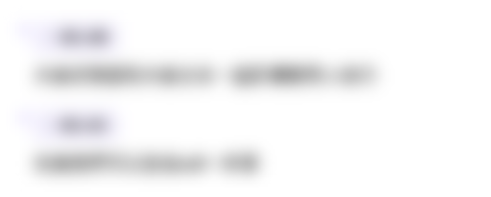
هذا القسم متوفر فقط للمشتركين. يرجى الترقية للوصول إلى هذه الميزة.
قم بالترقية الآنتصفح المزيد من مقاطع الفيديو ذات الصلة
5.0 / 5 (0 votes)