The JavaScript DOM explained in 5 minutes! 🌳
Summary
TLDRThis script offers an insightful introduction to the Document Object Model (DOM) in JavaScript, explaining how it represents the structure of a web page as a tree of objects. It demonstrates how to interact with the DOM using JavaScript, allowing dynamic changes to the page's content, structure, and style after it loads. Examples include changing the web page's title and background color, and creating and updating an H1 element based on user input, showcasing the power of JavaScript in manipulating web pages.
Takeaways
- 🌐 The DOM (Document Object Model) is a JavaScript object that represents the structure of a web page as seen in the browser.
- 📚 The DOM provides an API for interacting with the web page, allowing dynamic changes to its content, structure, and style.
- 🌳 The browser constructs the DOM by structuring HTML elements in a tree-like representation when loading a document.
- 🔍 Elements within the DOM can be accessed and manipulated using methods like `getElementById`.
- 📝 The `document` object is a key part of the DOM, containing properties and methods for interacting with the HTML document.
- 💬 The `document` object can be logged to the console to display the HTML document, and `dir` can be used to list all properties of the object.
- 🎨 You can dynamically change the page's title and style properties, such as the background color, using the DOM API.
- 🔑 The `document` object can be accessed like any other JavaScript object, with properties like `title` that can be modified.
- 📝 Creating new elements, such as an H1, and manipulating their properties, like `textContent`, is possible through the DOM.
- 🔄 Conditional content updates, such as changing a welcome message based on user input, can be implemented using the DOM.
- 🔄 The DOM allows for the dynamic creation and modification of web page elements after the page has loaded.
Q & A
What is the DOM in JavaScript?
-The DOM, or Document Object Model, is a JavaScript object that represents the structure of a web page. It provides an API to interact with the page, allowing developers to dynamically access and modify the content, structure, and style of a document.
How does the web browser construct the DOM?
-When a web browser loads an HTML document, it constructs the DOM by structuring all of the elements in a tree-like representation, with the HTML element as the root node.
What is the root element of the DOM tree?
-The root element of the DOM tree is the HTML element, which contains the head and body elements, among others.
How can you select an element by its ID using the DOM?
-To select an element by its ID, you can use the method `document.getElementById` followed by the ID of the element you want to select.
What does the document object contain?
-The document object contains properties and methods, as well as nested objects. It represents the entire HTML document and can be used to access and manipulate various aspects of the page.
How can you change the title of a web page using JavaScript?
-You can change the title of a web page dynamically by accessing the document object's `title` property and assigning it a new value.
Can you change the background color of a document using the DOM?
-Yes, you can change the background color of a document by accessing the document object, then the body, and finally setting the `style.backgroundColor` property to the desired color value.
How can you create a new HTML element using JavaScript?
-You can create a new HTML element by using the `document.createElement` method, passing in the tag name of the element you want to create.
What is the purpose of the 'dir' method in the context of the DOM?
-The 'dir' method, which stands for directory, is used to list all of the properties of an object, providing an overview of the object's structure and available properties.
How can you update the text content of an HTML element?
-To update the text content of an HTML element, you can access the element using its ID or another selector, and then modify the `textContent` property with the new text you want to display.
What is the significance of camel case naming convention mentioned in the script?
-The camel case naming convention is a practice in programming where the first word is in lowercase and the first letter of each subsequent word is capitalized. It is used for readability and to distinguish variable names, as demonstrated with 'welcomeMessage'.
Outlines
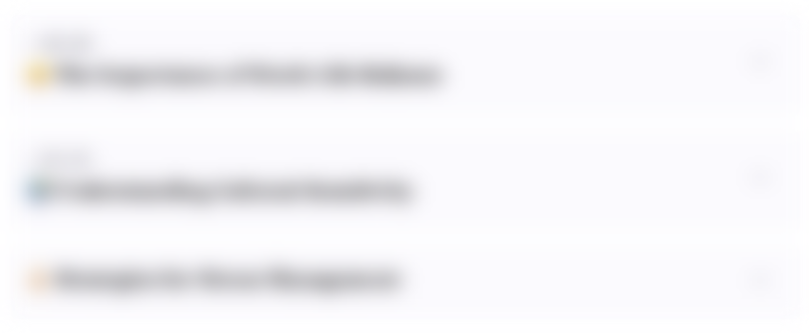
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
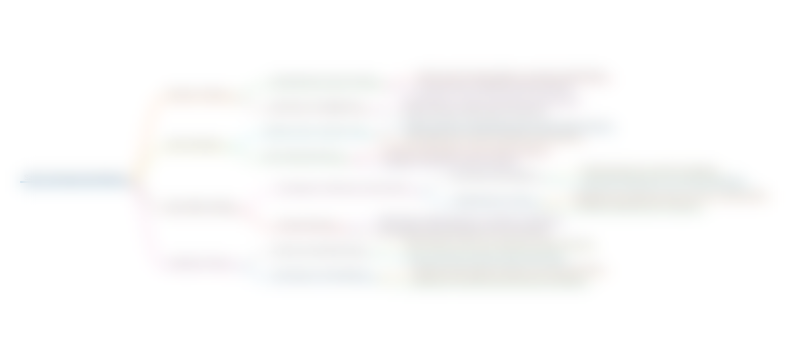
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
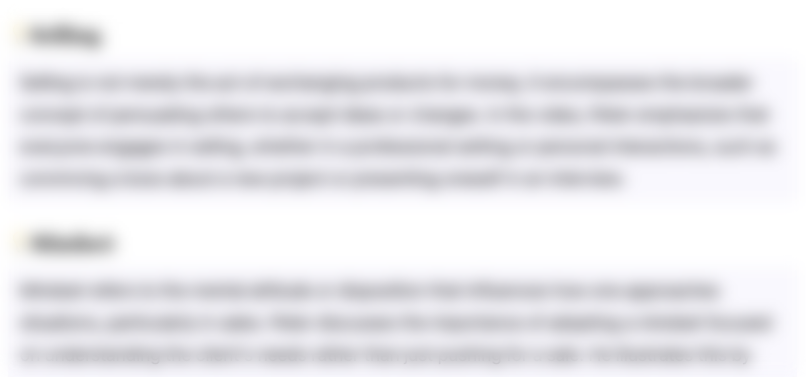
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
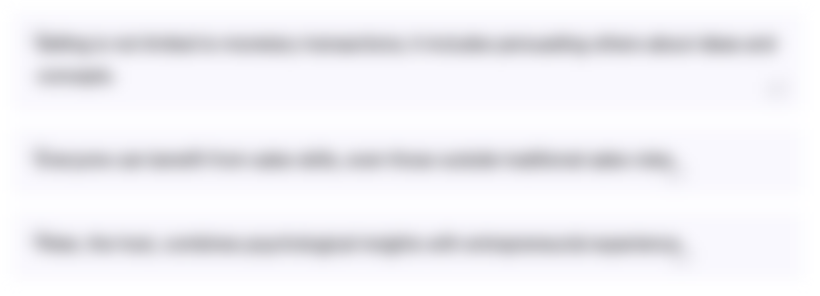
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
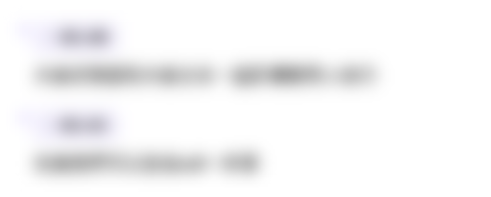
此内容仅限付费用户访问。 请升级后访问。
立即升级5.0 / 5 (0 votes)