Abstract Classes and Methods in Java Explained in 7 Minutes
Summary
TLDRIn this Java tutorial, John, a lead software engineer, demystifies abstract classes by explaining their purpose and usage with an example. He clarifies that abstract classes cannot be instantiated but can be subclassed, illustrating with an 'Animal' class. John also introduces abstract methods, which enforce subclasses to implement specific behaviors. He contrasts abstract classes with interfaces, highlighting that interfaces can't have instance fields and a class can implement multiple interfaces but only extend one class. The tutorial aims to provide a clear understanding of when to use abstract classes and interfaces in Java.
Takeaways
- 📚 Abstract classes in Java cannot be instantiated, meaning you cannot create objects directly from them.
- 🐱 An example given is the 'Cat' class, which if made abstract, cannot be instantiated but can be subclassed.
- 🔑 The 'abstract' keyword is used in the class definition to make a class abstract.
- 🤔 The purpose of an abstract class is to provide a base for subclasses to share fields and methods, without being instantiated themselves.
- 📝 Abstract classes can contain both abstract methods, which have no implementation and must be overridden by subclasses, and concrete methods, which provide default implementations.
- 📣 Abstract methods are declared without a method body and end with a semicolon, requiring subclasses to provide an implementation.
- 🐾 In the example, the 'make noise' method is abstract, so each animal subclass like 'Cat' must implement its own version.
- 🔄 Abstract classes enforce a contract for subclasses, ensuring they provide specific functionality, such as the 'make noise' method.
- 🔄 Interfaces in Java are also used for ensuring classes implement certain methods, but they differ in that they can't contain non-static or non-final fields.
- 🔗 A class can implement multiple interfaces but can only extend one class, highlighting a key difference between abstract classes and interfaces.
- 🌐 The choice between using an abstract class or an interface depends on whether you need to share fields and closely related functionality (abstract class) or ensure unrelated classes can perform certain actions (interface).
Q & A
What is an abstract class in Java?
-An abstract class in Java is a class that cannot be instantiated. It serves as a base class for other classes, providing a common definition for its subclasses.
How do you declare a class as abstract in Java?
-You declare a class as abstract by using the 'abstract' keyword in the class definition, like 'public abstract class ClassName'.
Why would you create an abstract class if you can't instantiate it?
-You create an abstract class to provide a common set of fields and methods that must be shared by its subclasses, but you may not want to instantiate the abstract class itself.
Can you provide an example of when to use an abstract class?
-An example is the 'Animal' class, which might have fields like 'age' and 'name' and methods that all animals should have, but you wouldn't want to create an 'Animal' object without specifying what kind of animal it is.
What is an abstract method and how is it declared?
-An abstract method is a method declared in an abstract class with no implementation. It is declared by providing the method signature and ending with a semicolon instead of a method body.
What is the requirement for subclasses of an abstract class that contains abstract methods?
-Subclasses of an abstract class must provide an implementation for all inherited abstract methods.
Can an abstract class have both abstract and non-abstract (concrete) methods?
-Yes, an abstract class can have a mix of abstract methods, which must be implemented by subclasses, and concrete methods, which provide default implementations that subclasses can use or override.
What is the difference between an abstract class and an interface in Java?
-An abstract class can provide both abstract and concrete methods and fields, while an interface can only have abstract methods. Additionally, a class can implement multiple interfaces but can only extend one class.
How do fields declared in an interface differ from those in an abstract class?
-Fields declared in an interface are automatically static and final, meaning they have the same value for all instances of classes implementing the interface. In contrast, fields in an abstract class can have different values for each instance of its subclasses.
Can a class extend another class and implement multiple interfaces at the same time?
-Yes, a class in Java can extend one class and implement multiple interfaces, combining the functionality and fields from both the superclass and the interfaces.
What is the practical use of having an abstract method in an abstract class?
-The practical use of an abstract method in an abstract class is to enforce that all subclasses implement a specific method, ensuring a consistent contract for the behavior that must be defined by each subclass.
Outlines
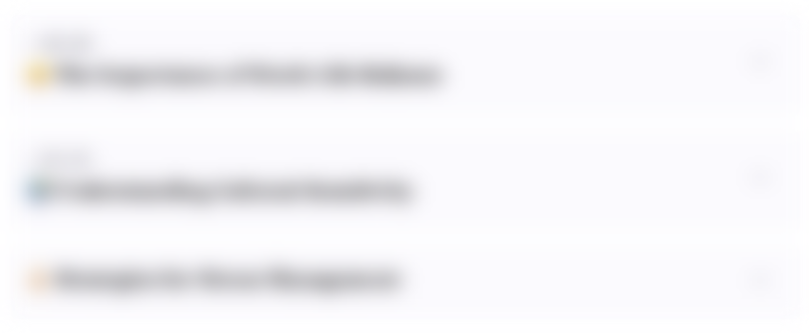
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
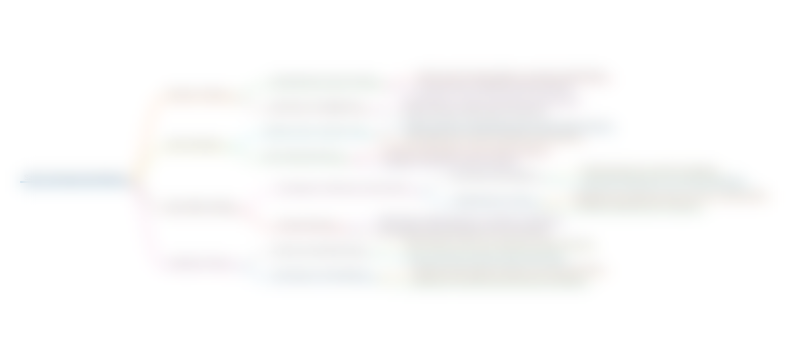
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
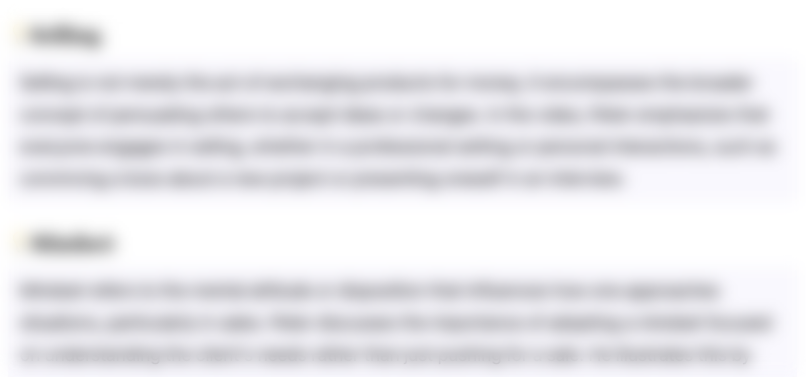
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
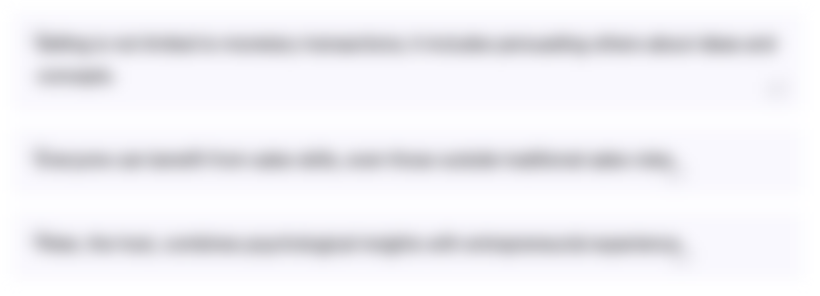
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
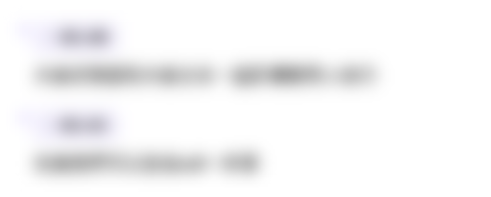
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
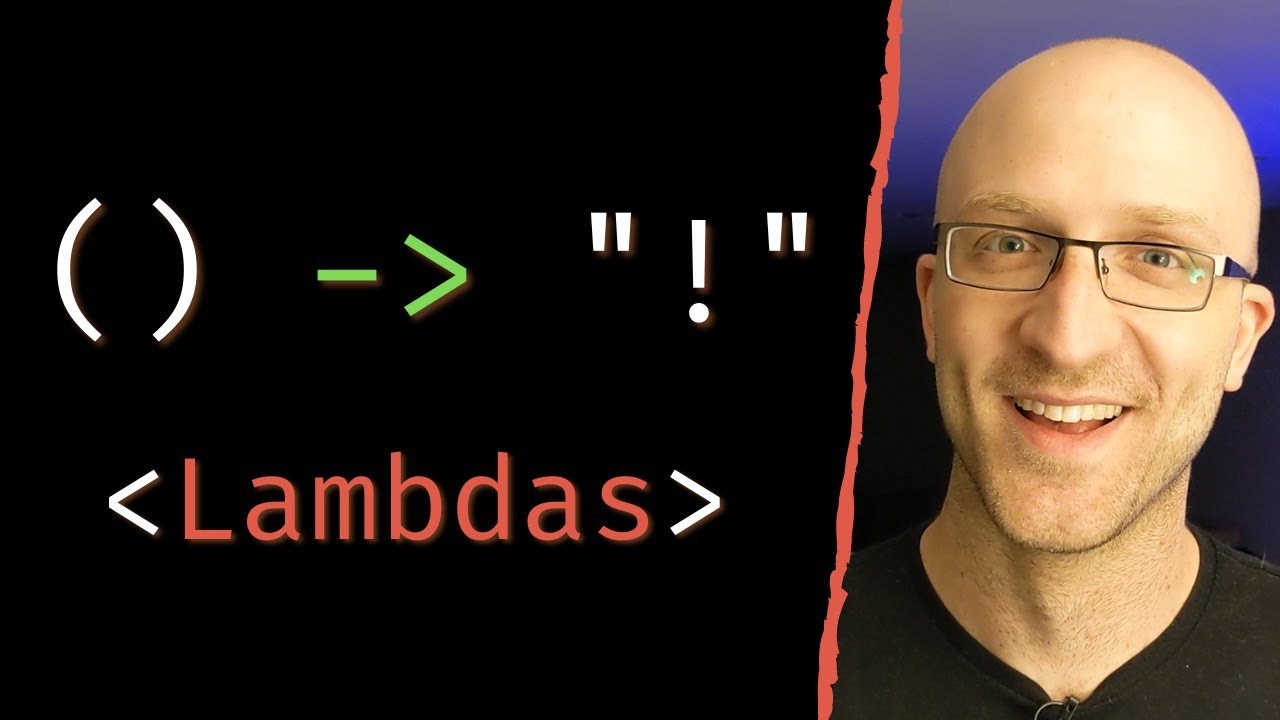
Lambda Expressions in Java - Full Simple Tutorial

Null Pointer Exceptions In Java - What EXACTLY They Are and How to Fix Them
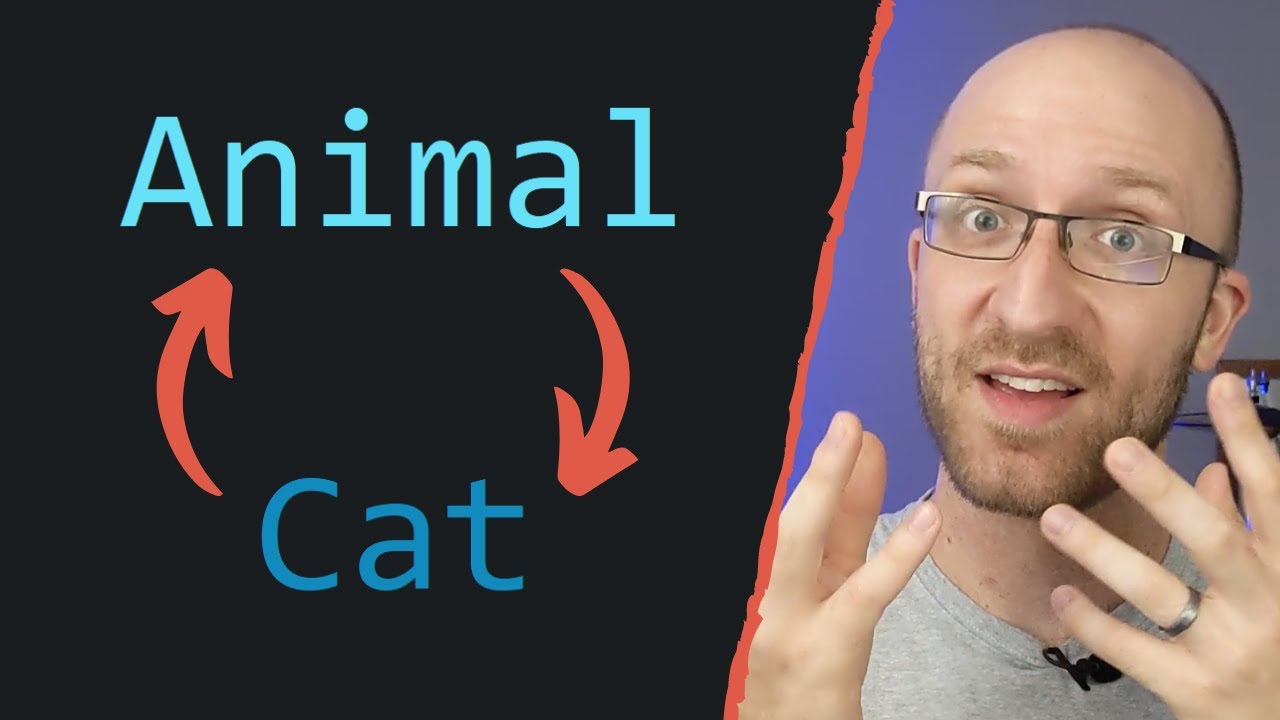
Upcasting and Downcasting in Java - Full Tutorial
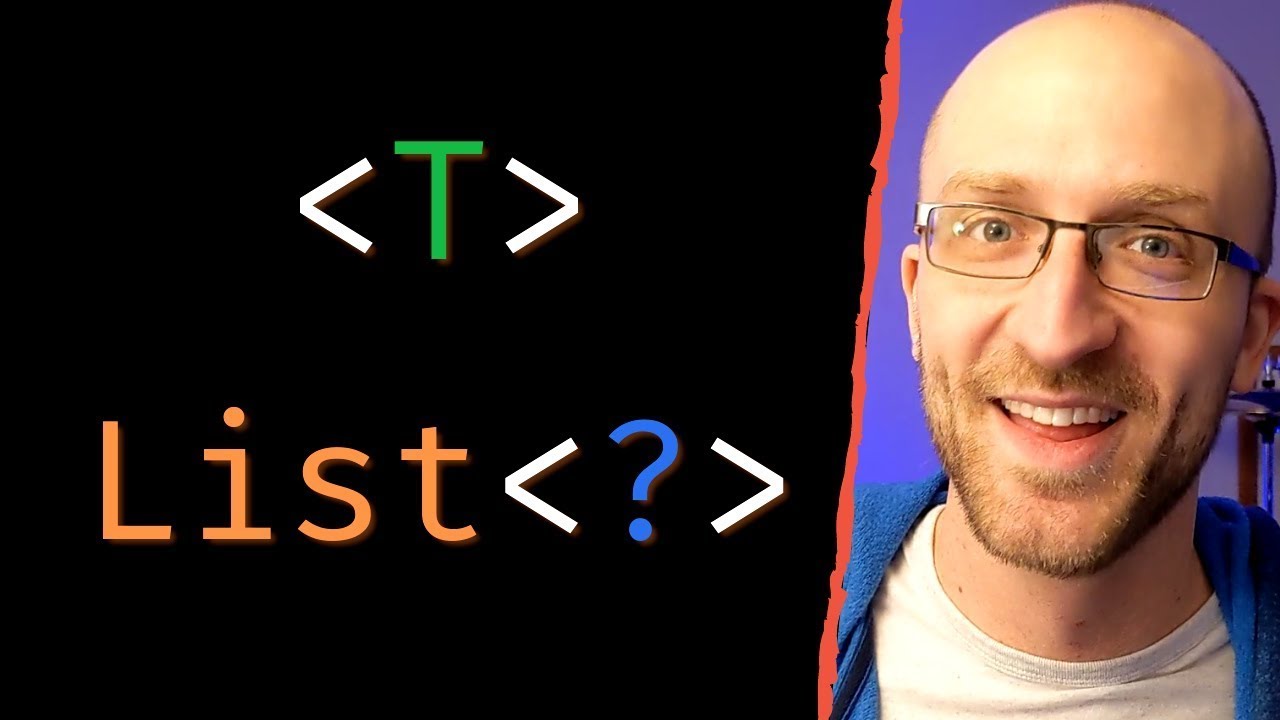
Generics In Java - Full Simple Tutorial
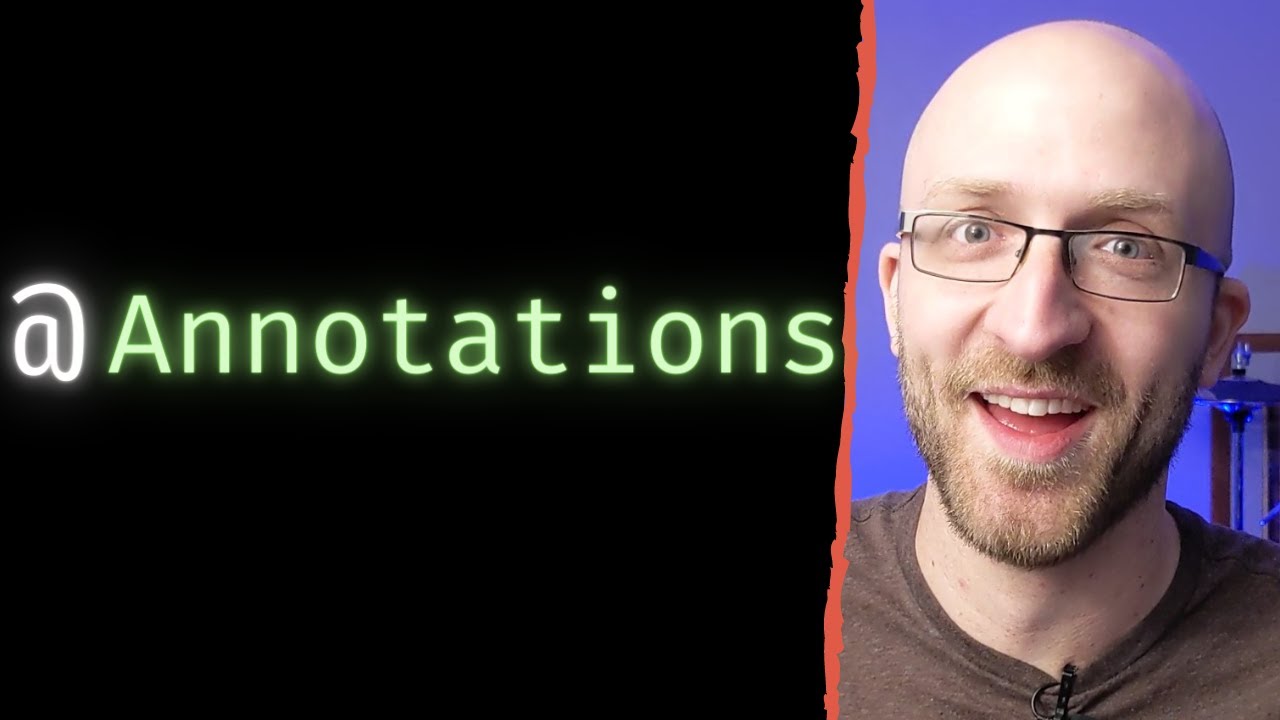
Annotations In Java Tutorial - How To Create And Use Your Own Custom Annotations
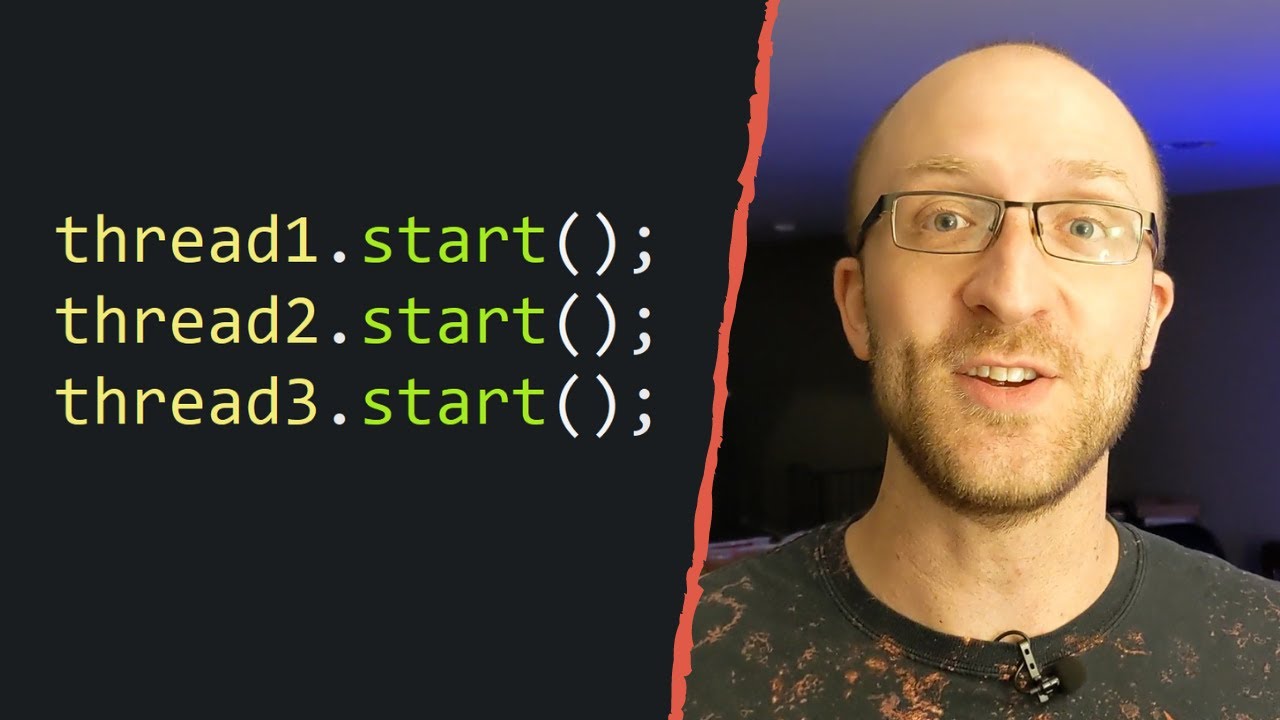
Multithreading in Java Explained in 10 Minutes
5.0 / 5 (0 votes)