Lambda Expressions in Java - Full Simple Tutorial
Summary
TLDRThis video script by John, a lead Java software engineer, dives into the intricacies of Java lambdas, a powerful yet often confusing feature for beginners. It explains the purpose of lambdas, their usage alongside functional interfaces, and how to implement them succinctly. The script provides a clear example using a 'Printable' interface and demonstrates how lambda expressions can simplify the process of passing method implementations as parameters, turning code into a treatable object. The tutorial also covers lambdas with parameters and return types, and emphasizes the importance of functional interfaces with a single abstract method for lambda expressions to work effectively.
Takeaways
- π» Lambdas are a powerful but often confusing feature in Java, especially for beginners.
- π To understand lambdas, it's essential first to understand interfaces in Java.
- π¨οΈ An interface in Java acts like a contract, requiring any implementing class to provide specific method implementations.
- π± Using a 'printable' interface example, a 'Cat' class must implement the 'print' method to print 'meow'.
- π Lambdas simplify the process by allowing direct implementation of methods without needing a class to implement the interface.
- π§ Lambda syntax eliminates the need for method access levels, names, and return types, focusing only on parameters and method implementation.
- π Lambdas in Java allow method implementations to be treated as objects, which can be saved as variables and passed into methods.
- π€ If a functional interface method takes parameters, lambdas can accept and use these parameters in the method implementation.
- π Functional interfaces, which lambdas rely on, have exactly one abstract method, often annotated with @FunctionalInterface.
- βοΈ Technically, lambdas are a shortcut to defining implementations of functional interfaces, enabling code to be treated as parameters.
Q & A
What are lambdas in Java and why are they important?
-Lambdas in Java are a way to implement methods defined in functional interfaces without needing to create a separate class. They are important because they simplify the code, making it more readable and concise by allowing method implementations to be treated as expressions.
What is a functional interface in Java?
-A functional interface in Java is an interface that contains exactly one abstract method. This single abstract method can be implemented using a lambda expression. Functional interfaces can also contain default and static methods.
How do you declare a functional interface in Java?
-You declare a functional interface by defining an interface with a single abstract method and optionally annotating it with @FunctionalInterface. This annotation is not required but is a best practice to ensure the interface only contains one abstract method.
Can you explain the syntax of a lambda expression?
-The syntax of a lambda expression consists of parameters, an arrow operator (->), and a method body. For example, (parameters) -> { method body }. If the method body is a single expression, the curly braces can be omitted.
What is the difference between using lambdas and creating a class to implement a functional interface?
-Using lambdas allows you to define the implementation of a functional interface method directly and concisely, without creating a separate class. It simplifies the code by eliminating boilerplate code associated with class definitions.
How do lambdas improve code readability and maintainability?
-Lambdas improve code readability and maintainability by reducing the amount of boilerplate code and allowing the focus to be on the behavior being implemented. This makes the code more concise and easier to understand.
What happens if a functional interface has more than one abstract method?
-If a functional interface has more than one abstract method, it cannot be used with lambda expressions. The compiler will generate an error because lambdas are intended to provide the implementation for a single abstract method.
How do you pass a lambda expression as a parameter to a method?
-You pass a lambda expression as a parameter to a method by directly providing the lambda expression in place of an argument that expects a functional interface. For example, if a method expects a Printable, you can pass (parameter) -> { implementation }.
Can lambda expressions have parameters and return types?
-Yes, lambda expressions can have parameters and return types. The parameters are defined in the parentheses before the arrow operator, and the return type is inferred from the method body. If the method body is a single expression, it is treated as the return value.
What are some best practices when working with lambda expressions in Java?
-Some best practices when working with lambda expressions include: using meaningful parameter names, keeping lambda expressions short and simple, and avoiding complex logic within lambda expressions. Additionally, use the @FunctionalInterface annotation to ensure your interface remains a valid functional interface.
Outlines
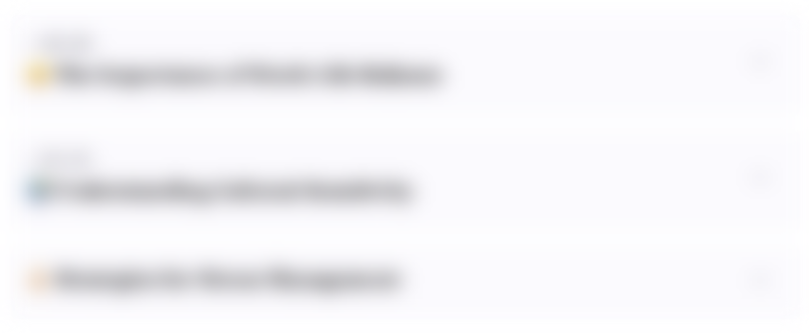
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
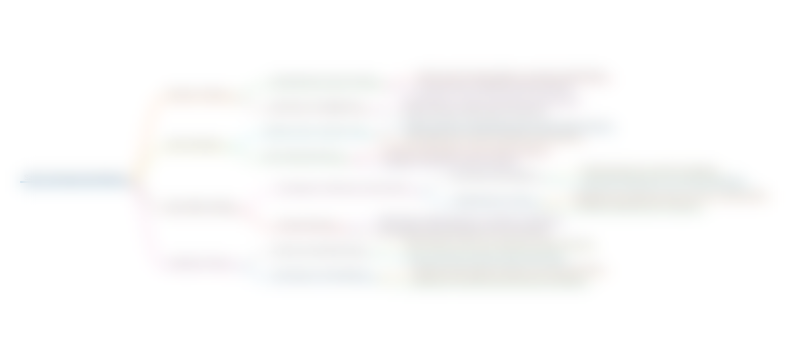
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
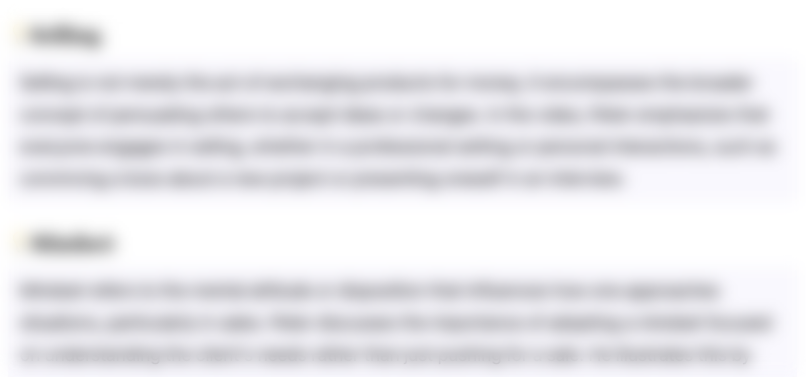
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
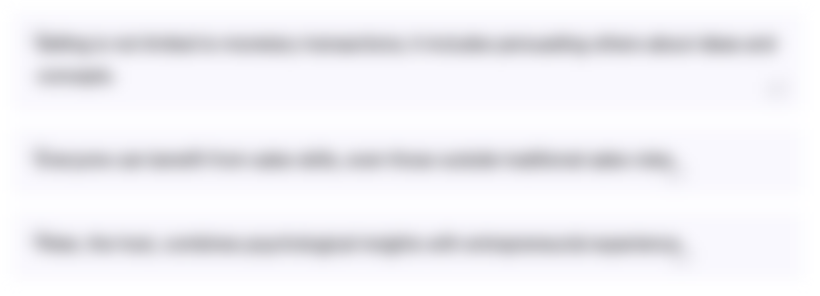
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
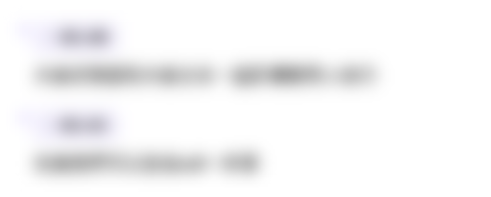
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)