Upcasting and Downcasting in Java - Full Tutorial
Summary
TLDRIn this Java tutorial, John, a lead Java software engineer, explains upcasting and downcasting concepts with clarity. Upcasting, which involves casting a subclass object to its superclass, is automatic and error-free in Java. Downcasting, conversely, requires explicit casting from a superclass to a subclass and necessitates careful handling to avoid ClassCastExceptions. The video uses a practical example with an 'Animal' superclass and a 'Dog' subclass to demonstrate these concepts, highlighting the importance of the 'instanceof' operator for safe downcasting. John encourages viewers to subscribe for more Java tutorials and offers a full Java course for further learning.
Takeaways
- π Upcasting in Java is the process of casting a subclass object to its superclass type.
- πΆ Downcasting in Java involves casting a superclass object to one of its subtypes, which requires explicit action and can throw exceptions if not done correctly.
- π Java automatically performs upcasting without the need for explicit casting syntax, as it's a safe operation that never fails.
- π« Downcasting must be done explicitly and can result in a `ClassCastException` if the object is not an instance of the target class.
- πΊ An example used in the video is the `Animal` class and its subclass `Dog`, where `Dog` overrides the `makeNoise` method and adds a `growl` method.
- π When upcasting, you can only access methods and properties that are available in the superclass, not those exclusive to the subclass.
- π οΈ The `instanceof` operator is used to safely check if an object is an instance of a specific class before downcasting.
- π Upcasting allows for writing more flexible and reusable code, as methods can operate on any object of the superclass type or its subclasses.
- π The video emphasizes the importance of understanding the difference between upcasting and downcasting to avoid runtime errors and write more robust Java code.
- π¨βπ« The presenter, John, a lead Java software engineer, aims to teach these concepts in a clear and understandable way, offering a full Java course for further learning.
Q & A
What are upcasting and downcasting in Java?
-Upcasting is the process of casting an object to its superclass type, while downcasting is casting an object to one of its subtypes or child class.
Why is upcasting in Java considered safe and automatic?
-Upcasting is safe and automatic because every subclass is a type of its superclass, so Java can implicitly convert a subclass object to a superclass type without any issues.
What is the difference between the capabilities of an 'Animal' reference and a 'Dog' reference in the context of the video?
-An 'Animal' reference can only access methods and attributes defined in the 'Animal' class, whereas a 'Dog' reference can access all 'Animal' methods and attributes plus the additional 'growl' method specific to the 'Dog' class.
How does method overriding relate to upcasting in Java?
-Method overriding allows a subclass to provide a specific implementation of a method that is already defined in its superclass. When upcasting is used, the correct overridden method is called depending on the actual object type, even though the reference type might be the superclass.
What is the purpose of upcasting in Java as explained in the video?
-Upcasting in Java allows for the creation of methods that can operate on any subclass of a superclass, enabling polymorphic behavior and reducing the need for creating separate methods for each subclass.
Why is downcasting not automatic in Java, and what is required to perform it?
-Downcasting is not automatic because Java cannot guarantee that a superclass reference actually points to an instance of a subclass. To perform downcasting, an explicit cast is required, and it should be done with caution to avoid ClassCastException.
What is the 'instanceof' operator used for in the context of downcasting?
-The 'instanceof' operator is used to check if an object is an instance of a specific class before performing a downcast. It helps to avoid ClassCastException by ensuring that the cast is safe.
How can you safely downcast an 'Animal' object to a 'Dog' in Java as per the video?
-You can safely downcast an 'Animal' object to a 'Dog' by first checking if the object is an instance of 'Dog' using the 'instanceof' operator, and then performing the cast within an 'if' block that only executes if the check is true.
What is the consequence of attempting to downcast an object to a type it is not an instance of?
-Attempting to downcast an object to a type it is not an instance of results in a ClassCastException, which is a runtime error that occurs when the cast is not valid.
What is the benefit of using the 'instanceof' operator before downcasting in Java?
-Using the 'instanceof' operator before downcasting allows you to verify that the object is of the correct type, thus preventing a ClassCastException and enabling safe downcasting when the object is indeed of the expected subclass.
Outlines
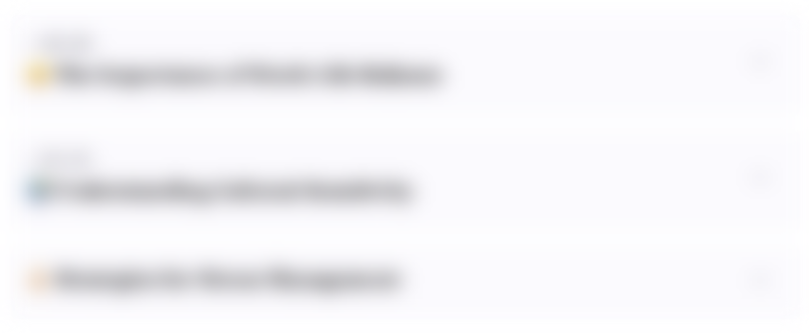
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
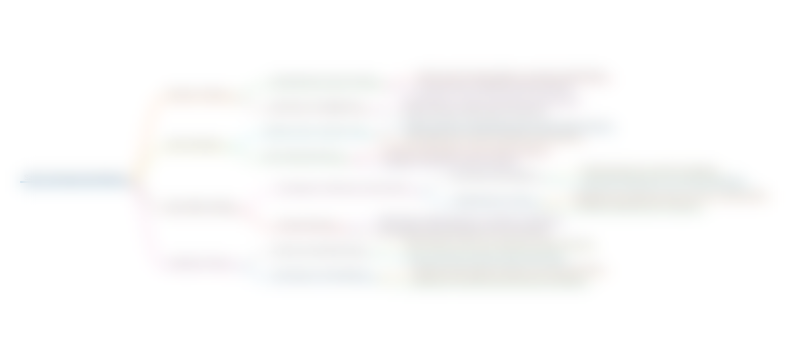
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
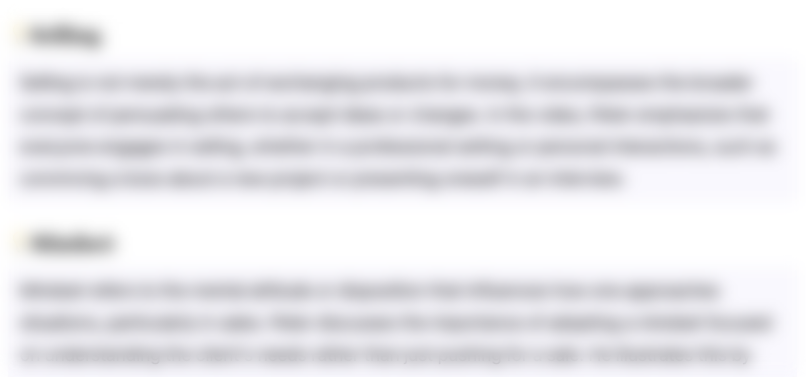
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
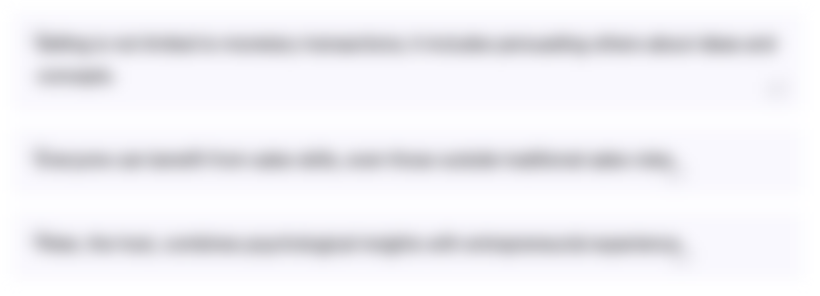
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
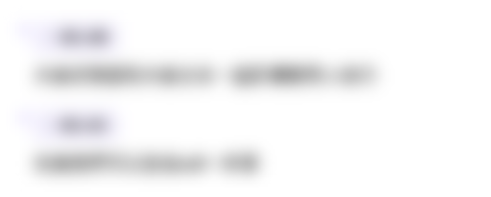
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

Null Pointer Exceptions In Java - What EXACTLY They Are and How to Fix Them
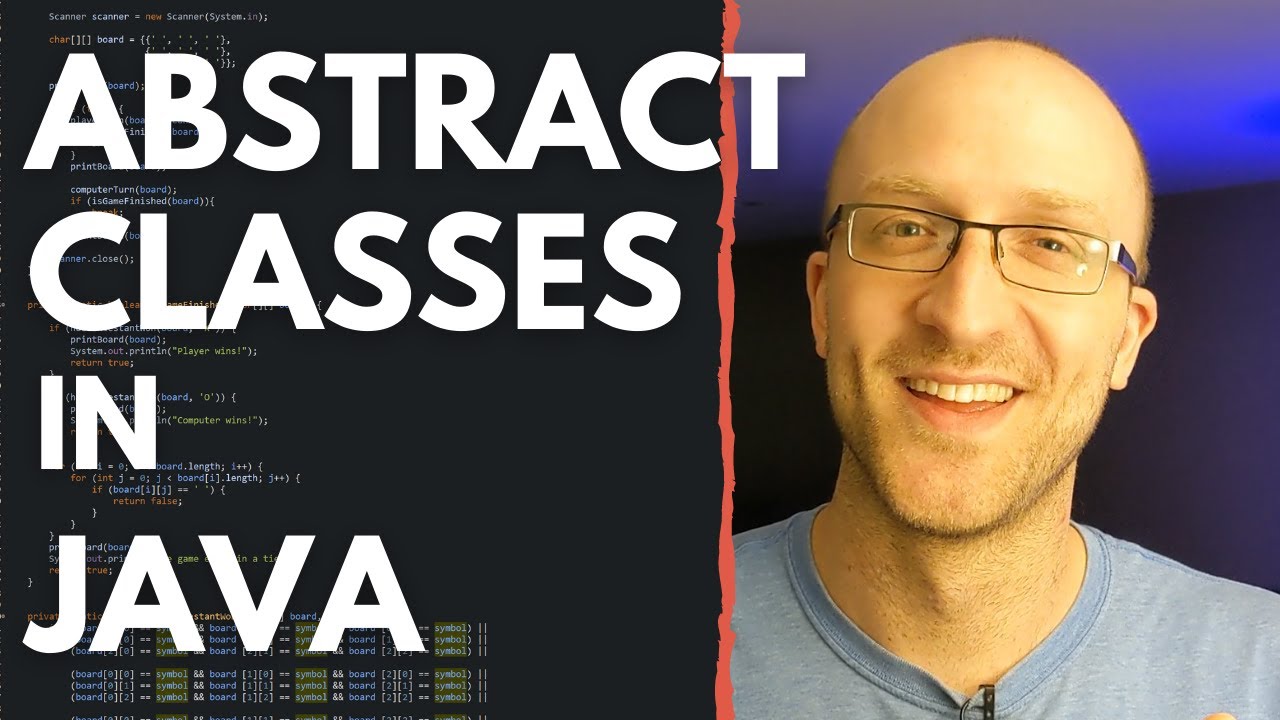
Abstract Classes and Methods in Java Explained in 7 Minutes
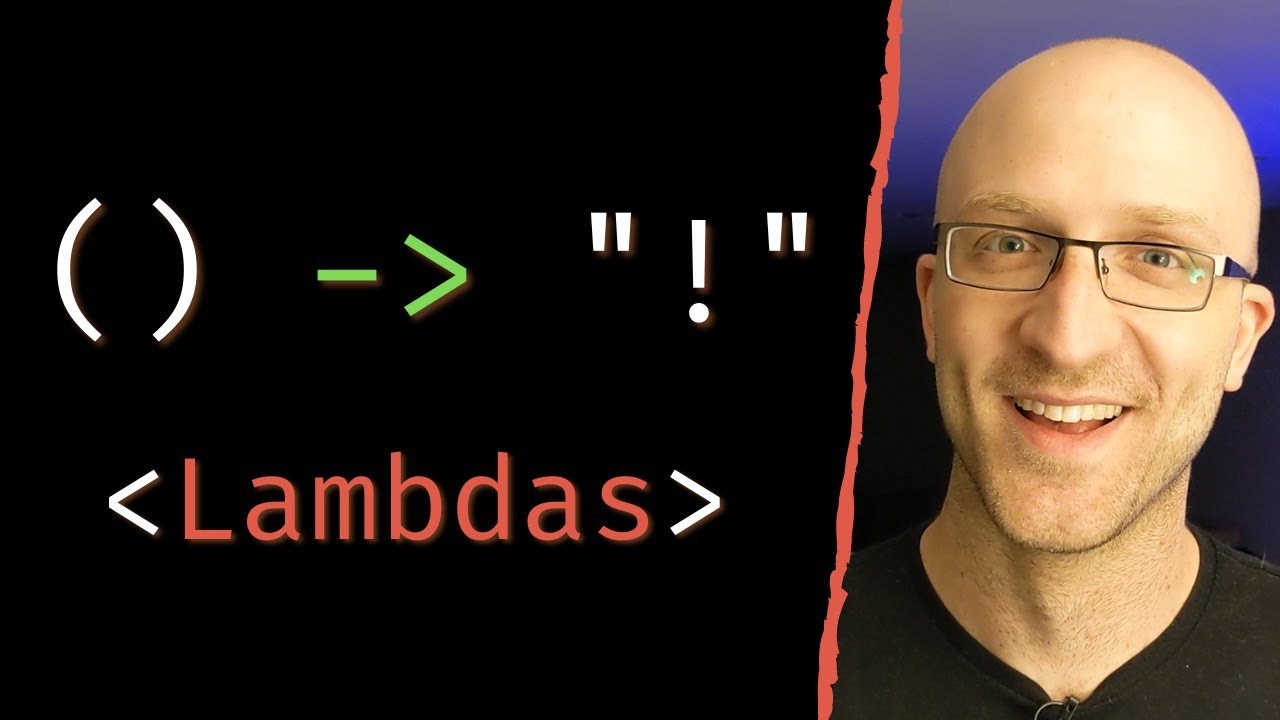
Lambda Expressions in Java - Full Simple Tutorial
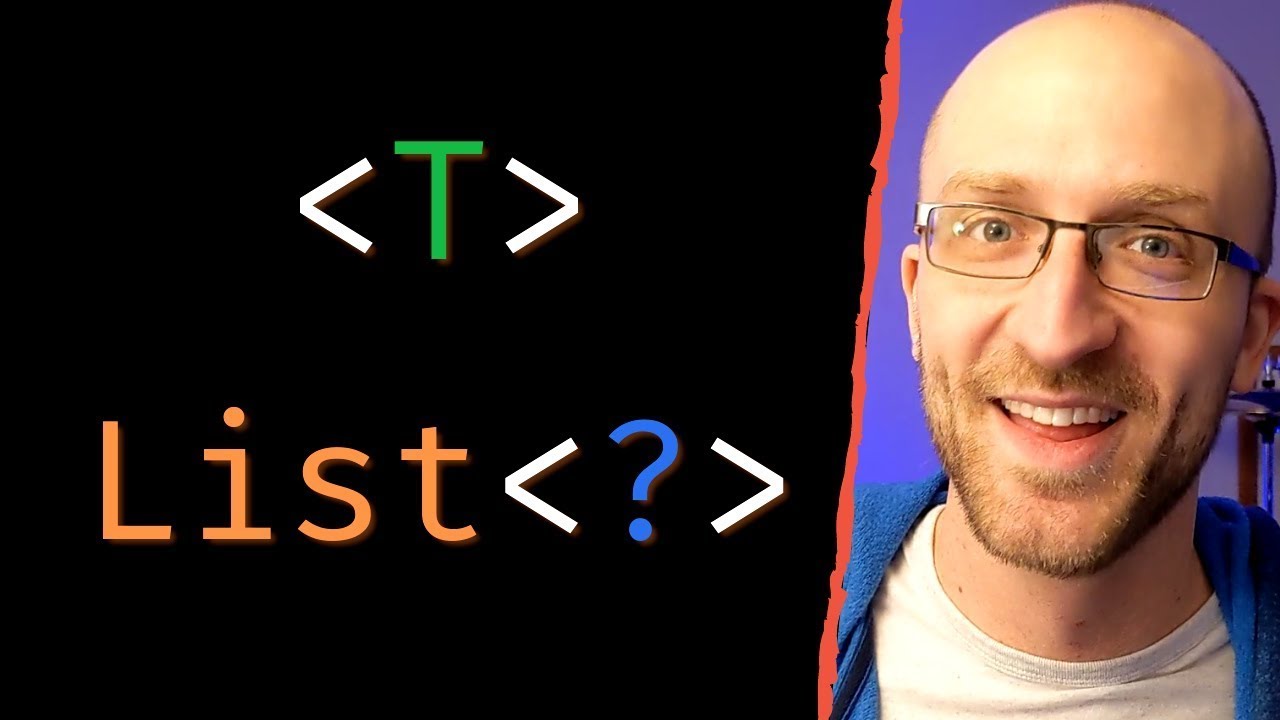
Generics In Java - Full Simple Tutorial
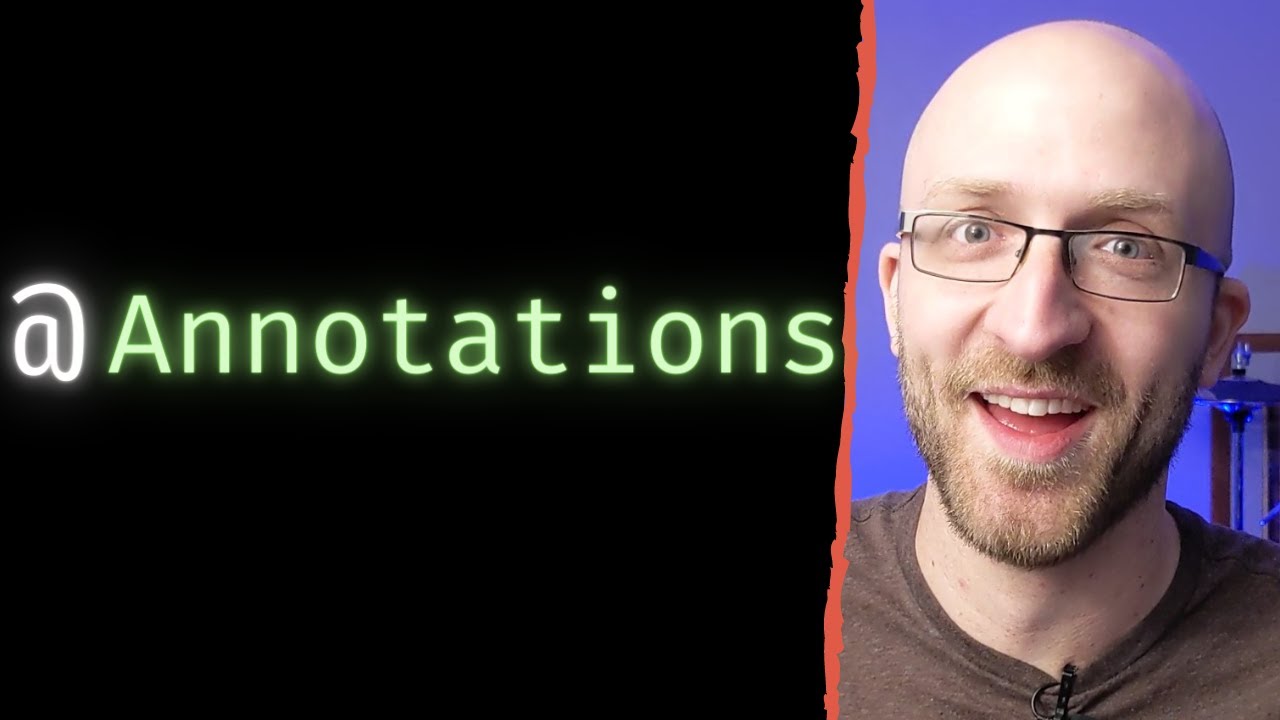
Annotations In Java Tutorial - How To Create And Use Your Own Custom Annotations

P2 - Introduction to Java | Java Programming | Core Java |
5.0 / 5 (0 votes)