Python Sockets Explained in 10 Minutes
Summary
TLDRIn this quick Python tutorial, the video demonstrates how to use sockets for server-client communication. It explains the process of creating a basic server and client using TCP, where the server listens for connections and sends/receives messages. The video also covers UDP, a connectionless protocol, showing how data can be sent and received without establishing a connection. The tutorial is designed for beginners, offering a clear and concise explanation with practical code examples for both TCP and UDP socket programming.
Takeaways
- 😀 Sockets in Python are communication endpoints used for networking between a client and server.
- 😀 The most common socket type for internet communication is TCP, which ensures a connection is maintained during data transfer.
- 😀 To create a server socket in Python, use the `socket.socket()` function with the socket type `SOCK_STREAM` for TCP connections.
- 😀 The server binds to an IP address and port using the `bind()` method, allowing it to listen for incoming connections.
- 😀 In a TCP connection, the server accepts connections using `server.accept()`, which returns a client socket and the client's address.
- 😀 The server can send and receive messages using the client socket, where the messages are encoded and decoded in byte format.
- 😀 A client connects to the server using the `connect()` method, passing the server's IP address and port.
- 😀 UDP sockets, unlike TCP, do not maintain a connection and use `SOCK_DGRAM` for datagram-based communication.
- 😀 With UDP, there is no need for the server to accept connections. Instead, it receives and sends messages to specific addresses.
- 😀 The client and server can communicate using UDP by calling `sendto()` and `recvfrom()`, sending and receiving raw data packets.
- 😀 The tutorial introduces the basic socket programming concepts in Python, but advanced topics like handling multiple connections and Bluetooth communication are suggested for further study.
Q & A
What is the main topic of the video?
-The video explains the concept of sockets in Python, specifically focusing on TCP and UDP sockets, and demonstrates how to implement a basic client-server architecture.
What is a socket in Python?
-A socket in Python is an endpoint for communication, allowing two devices to exchange data over a network. It can be used with different protocols like TCP (connection-oriented) or UDP (connectionless).
What is the difference between TCP and UDP sockets?
-TCP sockets maintain a connection and ensure reliable data transfer, while UDP sockets send data without establishing a connection, making it faster but less reliable.
How do you create a TCP socket in Python?
-To create a TCP socket, you use `socket.socket(socket.AF_INET, socket.SOCK_STREAM)`, where `AF_INET` is for Internet communication, and `SOCK_STREAM` specifies the TCP protocol.
What is the purpose of the 'bind' function in a server socket?
-The 'bind' function in a server socket is used to associate the socket with a specific IP address and port number, allowing it to listen for incoming connections on that address.
What does the 'listen' method do in a server socket?
-The 'listen' method in a server socket sets up the server to accept incoming connections, specifying the maximum number of connections allowed at once.
What is the role of the 'accept' method in a server socket?
-The 'accept' method in a server socket waits for incoming client connections and returns a new socket object for communication with the client, along with the client's address.
How does a client connect to a server in Python?
-A client connects to a server by creating a socket object using `socket.socket(socket.AF_INET, socket.SOCK_STREAM)` and calling the `connect` method with the server's IP address and port.
What is the key difference between TCP and UDP when it comes to sending and receiving data?
-In TCP, data is sent over a stable connection, ensuring reliability, whereas in UDP, data is sent without establishing a connection, leading to faster but less reliable transmission.
How does the server handle communication in a UDP socket setup?
-In a UDP socket, the server does not accept connections. Instead, it simply receives data from any client, prints it, and sends a response back to the same address using the 'sendto' method.
Outlines
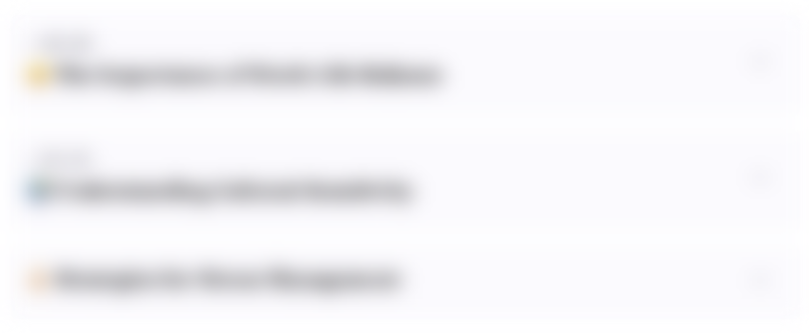
此内容仅限付费用户访问。 请升级后访问。
立即升级Mindmap
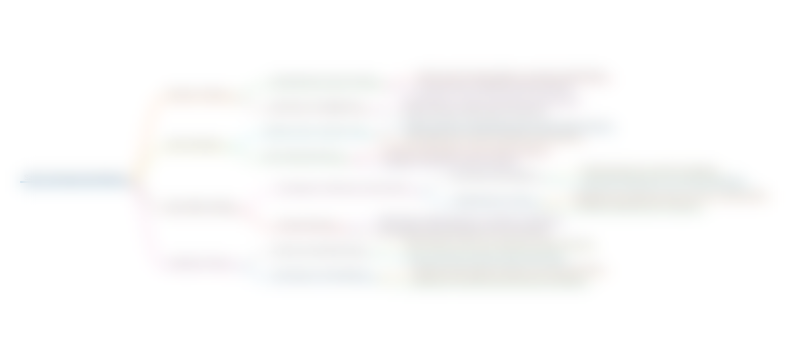
此内容仅限付费用户访问。 请升级后访问。
立即升级Keywords
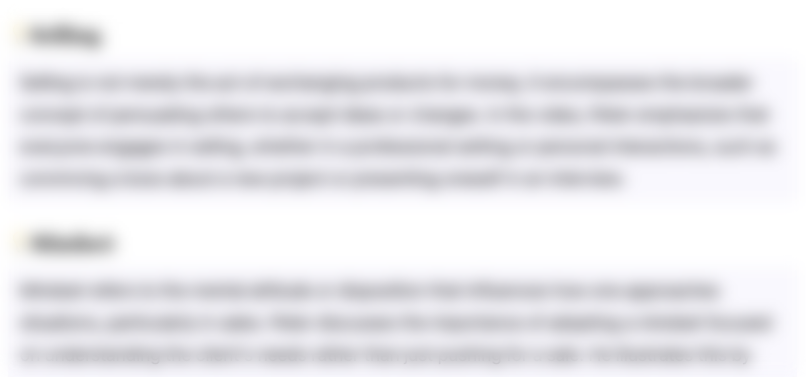
此内容仅限付费用户访问。 请升级后访问。
立即升级Highlights
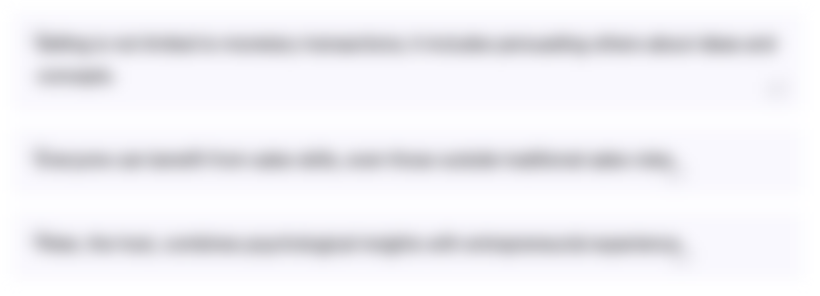
此内容仅限付费用户访问。 请升级后访问。
立即升级Transcripts
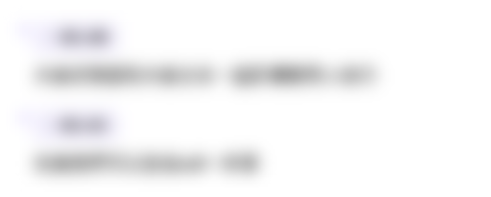
此内容仅限付费用户访问。 请升级后访问。
立即升级浏览更多相关视频
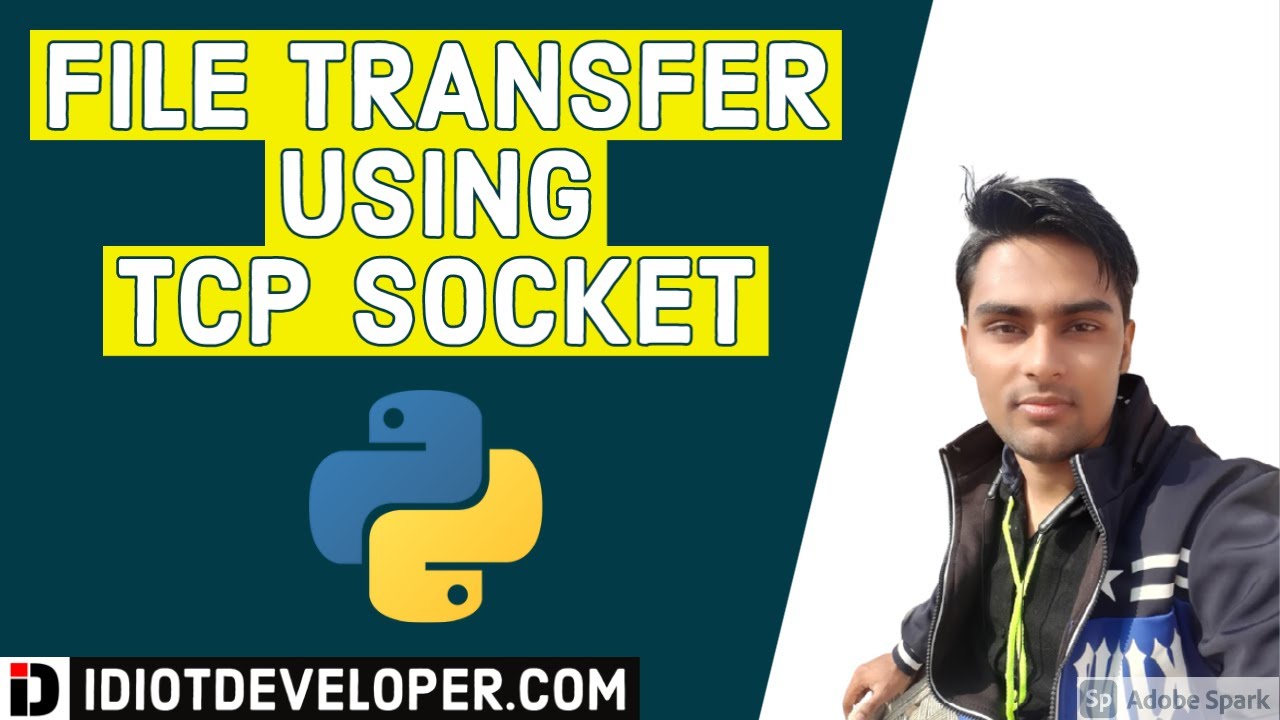
File Transfer using TCP Socket in Python | Socket Programming

NW LAB 1. Basics of Socket Programming in C : TCP and UDP - Program Demo

Sockets Tutorial with Python 3 part 1 - sending and receiving data
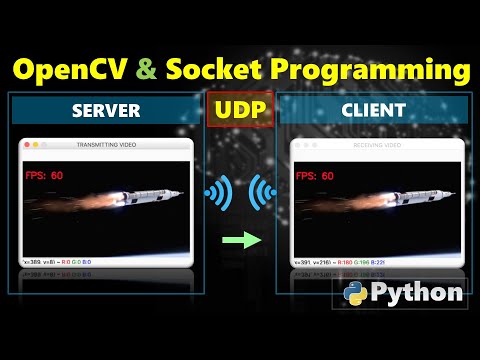
How to send video using UDP socket in Python: Socket Programming tutorial

TCP Connection Establishment || Three Way Handshake || Transport layer || Computer Networks

TCP vs UDP Comparison
5.0 / 5 (0 votes)