Sockets Tutorial with Python 3 part 1 - sending and receiving data
Summary
TLDRThis Python tutorial series introduces sockets programming with a focus on creating a server and client using Python's socket library. The video demonstrates setting up a TCP socket, binding it to a local IP and port, and listening for connections. It also covers sending and receiving data between the server and client, and the importance of buffering data streams. The tutorial simplifies the concepts for beginners, highlighting the need for handling larger messages and maintaining open connections in future videos.
Takeaways
- 📝 The tutorial covers creating a basic server and client using Python's socket library.
- 🔌 The socket object is created using `socket.socket()` with the AF_INET family for IPv4 and SOCK_STREAM for TCP.
- 📍 The server binds to a local IP and port, in this case, 'localhost' and port 1234.
- 🔗 The server listens for incoming connections with a queue of five to handle multiple requests.
- 🤝 Upon receiving a connection, the server accepts it and stores the client socket and address information.
- 💬 The server sends a welcome message to the client using UTF-8 encoded bytes.
- 🔄 The client connects to the server using the same socket object but with the connect method instead of bind.
- 📡 The client receives messages from the server in chunks, determined by the buffer size set in the receive method.
- 🔍 The script explains the concept of a socket as an endpoint for sending and receiving data on a network.
- 🔑 The tutorial touches on the importance of proper buffer management for handling data streams in socket communication.
- 🔄 The script demonstrates a basic example of socket communication but mentions the need for more complex logic for long-term connections.
Q & A
What is the purpose of the 'socket' module in Python?
-The 'socket' module in Python provides a way to create and manipulate network connections using the BSD socket interface. It is part of Python's standard library and does not require installation.
What does 'AF_INET' and 'SOCK_STREAM' represent in the context of creating a socket?
-'AF_INET' represents the address family, which corresponds to IPv4. 'SOCK_STREAM' represents the type of socket, which in this case is a TCP socket for a streaming connection.
Why is the server binding to 'socket.gethostname()' and port 1234?
-The server is binding to 'socket.gethostname()' to listen on the local machine's hostname and port 1234 to specify the port number on which it will accept incoming connections.
What is a socket in networking terms?
-A socket is an endpoint for sending and receiving data across a network. It sits at an IP address and a port, facilitating communication between networked devices.
What does the 'listen' method do for a server socket?
-The 'listen' method on a server socket allows the server to prepare for incoming connections. It sets up a queue for managing multiple incoming connection requests.
How does the server accept a client connection?
-The server accepts a client connection using the 'accept' method, which returns a new socket object representing the connection, as well as the address of the client.
What is the purpose of sending a welcome message to the client socket?
-Sending a welcome message to the client socket is a way to establish communication and inform the client that the connection has been successfully established.
What is the difference between binding a socket and connecting a socket?
-Binding a socket is done on the server side to specify the IP and port on which it will listen for incoming connections. Connecting a socket is done on the client side to specify the IP and port of the server to which it wants to connect.
Why is it necessary to buffer data when using sockets?
-Buffering data is necessary because sockets deal with a stream of data. The buffer determines how much data is received at a time, which is important for handling varying data sizes and maintaining the flow of data.
What is the significance of the 'utf-8' encoding when sending and receiving messages?
-The 'utf-8' encoding is significant because it allows for the transmission of text data in a standardized format that can be correctly interpreted by both the sender and the receiver.
How can you handle receiving messages larger than the buffer size?
-To handle messages larger than the buffer size, you can implement a loop that continues to receive data until the entire message is buffered. This often involves checking the length of the received message and concatenating the chunks until the complete message is assembled.
What is the role of the 'close' method in socket programming?
-The 'close' method is used to close the socket connection, freeing up the resources associated with the socket. It is important to properly close sockets when they are no longer needed to avoid resource leaks.
Outlines
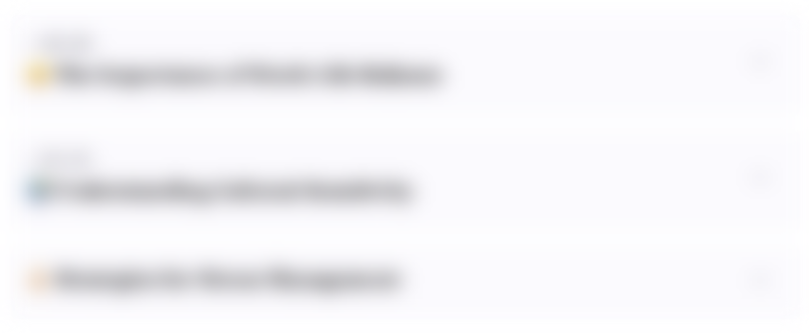
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
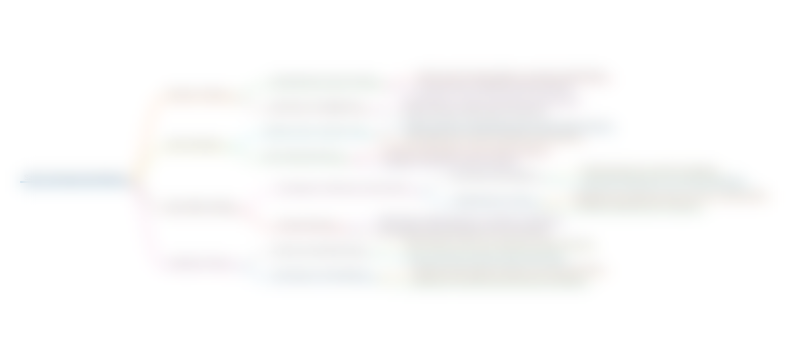
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
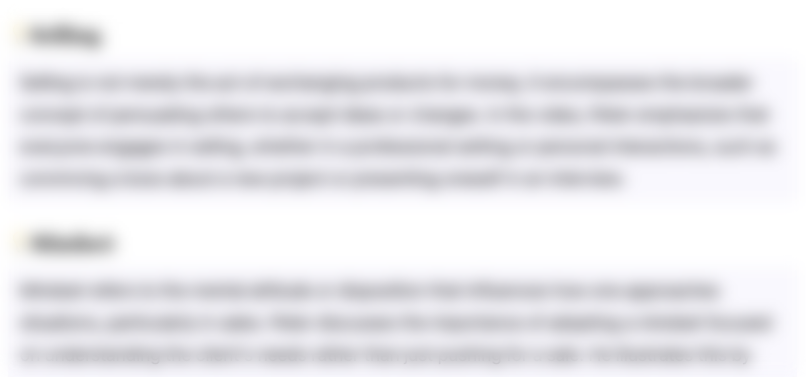
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
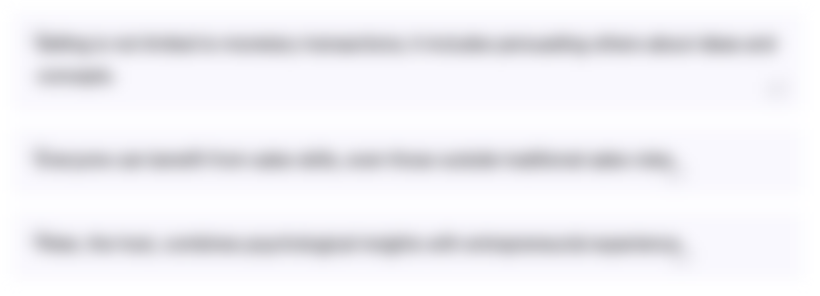
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
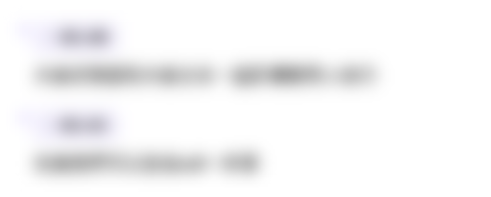
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)