File Transfer using TCP Socket in Python | Socket Programming
Summary
TLDRIn this video, the creator demonstrates how to build a simple client-server program using Python sockets. The client reads a file, sends both its name and contents to the server, which then saves the file on its side. The server listens for incoming connections, receives the data, and creates a new file with the same name to store the received content. The video covers key concepts like TCP communication, file handling, and basic error management. The tutorial is beginner-friendly and provides a clear example of transferring files between a client and a server.
Takeaways
- π The client-server program demonstrates how to send a file from the client to the server using Python's socket library.
- π The server listens for incoming connections on a specified IP and port, and can only handle one client at a time (non-concurrent).
- π The server uses TCP (Transmission Control Protocol) to establish a reliable connection with the client for file transfer.
- π The client reads a file (e.g., 'yt.txt') from its local directory and sends both the file name and its content to the server.
- π The server receives the file name first, creates a file with that name, and then saves the received data into it.
- π The server confirms the receipt of the file name and data by sending an acknowledgment back to the client.
- π The client waits for these acknowledgments before proceeding with the next step in the file transfer process.
- π The file is saved in a specific directory (e.g., 'server_data') on the server, and the server can handle file creation dynamically.
- π The client disconnects after the file transfer is complete, and the server is ready to accept the next client after the current connection is closed.
- π The script mentions the possibility of using threading to allow the server to handle multiple clients concurrently in future enhancements.
Q & A
What is the purpose of the client-server program discussed in the video?
-The purpose of the program is to enable the client to send a file's name and data to the server, where the server saves the file with the same name and contents.
What Python library is used to implement the server and client sockets in the video?
-The Python `socket` library is used to implement the server and client sockets in the program.
How does the server determine its IP address dynamically?
-The server uses `socket.gethostbyname(socket.gethostname())` to dynamically determine its IP address, avoiding the need to manually configure it.
What is the difference between TCP and UDP as described in the video?
-TCP (Transmission Control Protocol) is connection-oriented, meaning it ensures data is transmitted reliably between the client and server, whereas UDP (User Datagram Protocol) is connectionless and does not guarantee the delivery or order of data.
How does the server handle multiple client connections in the current program?
-The server in this program is designed to handle only one client connection at a time. It accepts a connection, processes it, and then closes the connection before accepting the next client.
What is the purpose of the `socket.bind()` method in the server code?
-The `socket.bind()` method is used to bind the server socket to a specific IP address and port so that the server can listen for incoming client connections on that address.
What does the client send first to the server?
-The client first sends the file name (e.g., `yt.txt`) to the server, allowing the server to create a file with the same name on the server side.
How does the server acknowledge the receipt of the file name from the client?
-The server sends a confirmation message back to the client, such as 'File name received,' to acknowledge the receipt of the file name.
What happens after the server receives the file name?
-After receiving the file name, the server creates a new file with that name and sends a confirmation message to the client that the file data can now be sent.
What could be the advantage of using threading in the server program?
-Using threading would allow the server to handle multiple client connections simultaneously, improving its efficiency and responsiveness in a real-world scenario where multiple clients may try to connect at the same time.
Outlines
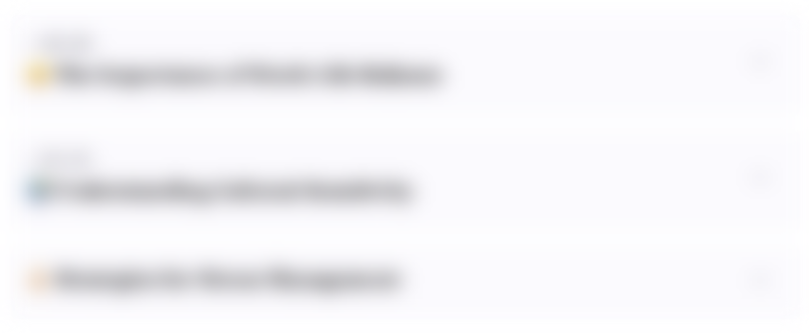
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
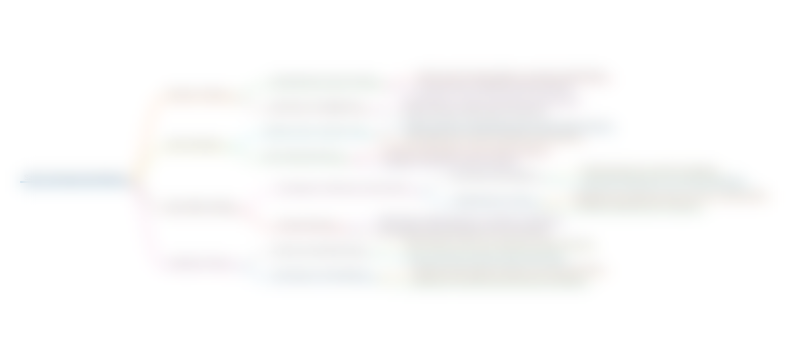
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
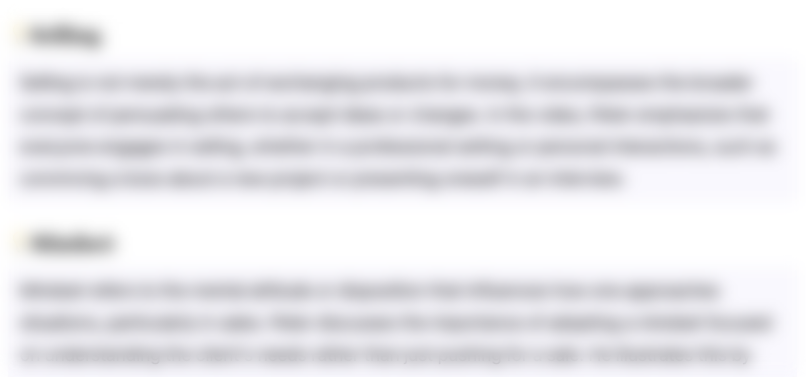
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
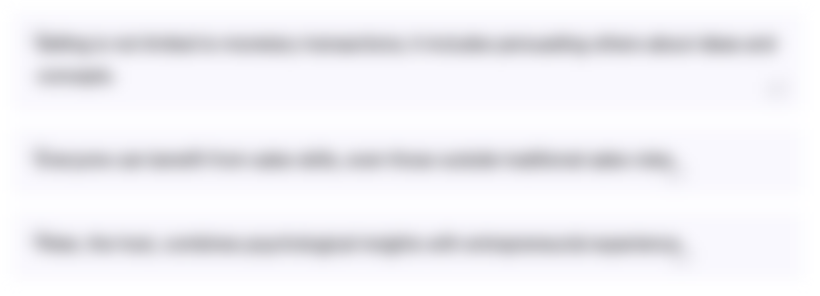
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
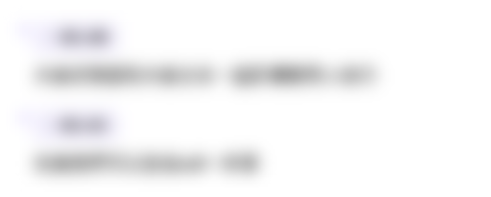
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
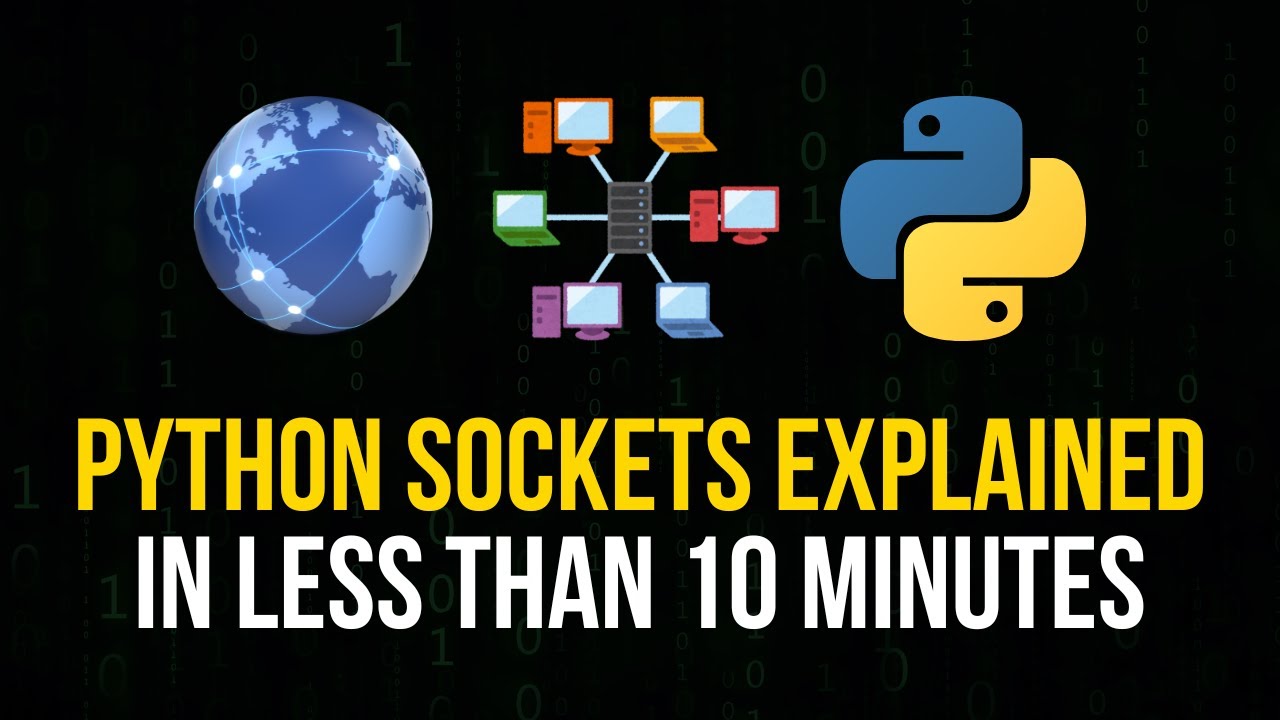
Python Sockets Explained in 10 Minutes

Sockets Tutorial with Python 3 part 1 - sending and receiving data
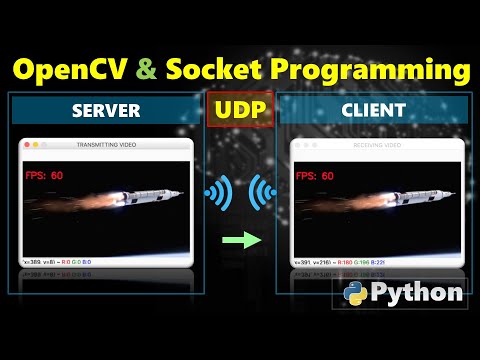
How to send video using UDP socket in Python: Socket Programming tutorial
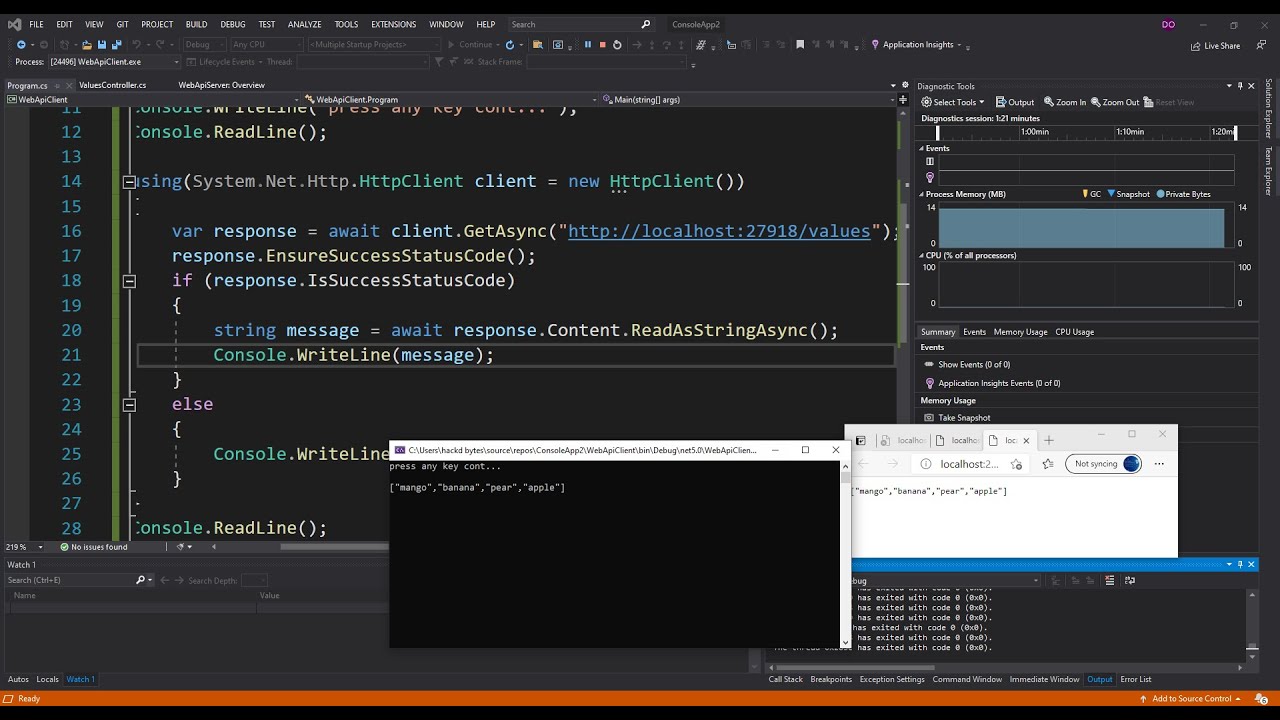
Asp.Net Core Web API Client/Server Application | Visual Studio 2019

NW LAB 1. Basics of Socket Programming in C : TCP and UDP - Program Demo
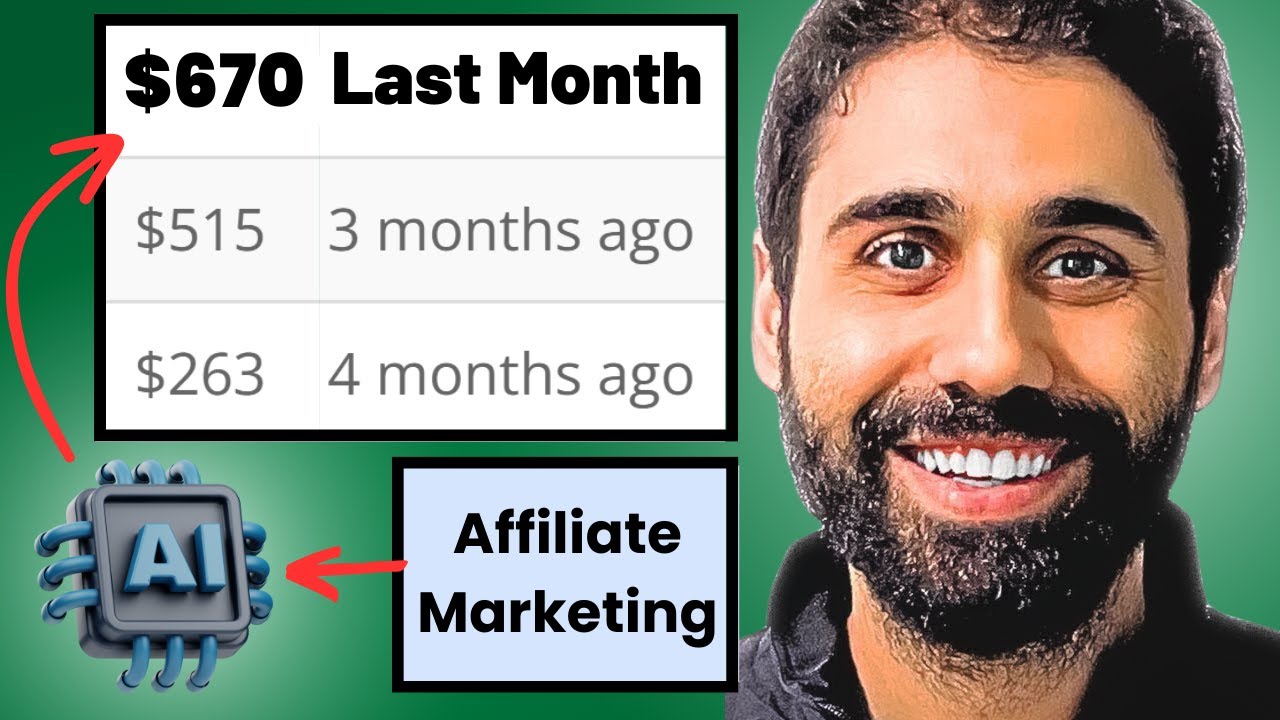
Affiliate Marketing with AI - New Method (2024)
5.0 / 5 (0 votes)