COS 333: Chapter 9, Part 1
Summary
TLDRThis lecture delves into the concept of sub-programs, exploring their role in programming languages and their abstraction capabilities. It covers fundamental aspects such as sub-program definitions, headers, calls, and parameters, including formal and actual parameters, as well as parameter profiles and protocols. The lecture also touches on various programming languages' handling of sub-programs, including executable definitions, anonymous functions, and default parameters. It discusses the distinction between procedures and functions, and raises design issues like local variable allocation and the possibility of nested sub-program definitions.
Takeaways
- π The lecture introduces Chapter 9 focusing on sub-programs, explaining their role in programming languages and fundamental concepts.
- π Abstraction is central to modern programming, with process abstraction being discussed in Chapter 9 and data abstraction in Chapter 11.
- π΅οΈββοΈ Sub-programs, also known as functions or methods, have a single entry point and suspend the execution of the calling program until they complete.
- π The lecture emphasizes the importance of understanding and using correct terminology when discussing sub-programs for test and exam purposes.
- π Sub-program definitions include the interface, detailing how the sub-program is called, and the actions, specifying what the sub-program does.
- π Executable sub-program definitions in languages like Python can vary depending on execution conditions, affecting program language evaluation criteria.
- π·οΈ The lecture distinguishes between sub-program headers, which include the name and parameters, and sub-program calls, which are requests to execute a sub-program.
- π The correspondence between actual and formal parameters can be ensured by positional or keyword parameters, each with its advantages and disadvantages.
- π― Default parameters and variable numbers of parameters offer flexibility in how sub-programs can be called, with specific language requirements and behaviors.
- π οΈ The lecture discusses design issues related to sub-programs, including local variable allocation (static or dynamic) and the possibility of nested sub-program definitions.
- βοΈ Different programming languages handle sub-programs and local variables differently, with implications for recursion, storage sharing, access speed, and history sensitivity.
Q & A
What is the main focus of chapter 9 in the textbook?
-Chapter 9 focuses on sub-programs, discussing their role in programming languages, fundamental concepts, design issues, and local referencing environments.
What are the two fundamental facilities that support abstraction in modern programming?
-The two fundamental facilities that support abstraction are process abstraction and data abstraction, with the latter being supported by abstract data types (ADTs) and object-oriented programming (OOP).
What is the difference between a sub-program and a calling program during execution?
-A sub-program is a part of the code that can be called by another part of the program, the calling program. When a sub-program is called, the execution of the calling program is suspended until the sub-program completes its execution and control returns to the caller.
What are the two main components defined by a sub-program definition?
-A sub-program definition defines the interface of the sub-program, which includes its name, parameter types, and return type, and the actions of the sub-program, which is its body or the operations it performs.
How do executable sub-program definitions affect the evaluation criteria of a programming language?
-Executable sub-program definitions allow for different definitions of the same sub-program to exist based on the execution path, which can affect the predictability and evaluation of the program's behavior in a language.
What is the difference between a sub-program header and a sub-program call?
-A sub-program header is the first part of the sub-program definition that includes the name, type, and formal parameters of the sub-program. A sub-program call is an explicit request to execute the sub-program, which includes the actual parameters passed to the sub-program.
What are formal parameters and actual parameters in the context of sub-programs?
-Formal parameters are the variables listed in the header of the sub-program and used within its body. Actual parameters are the values or addresses passed to the sub-program during a call.
What is the purpose of a sub-program declaration?
-A sub-program declaration provides the protocol of the sub-program, which includes the number, order, and types of parameters, as well as the return type, without providing the body of the sub-program.
How do positional parameters ensure the correspondence between actual and formal parameters?
-Positional parameters ensure correspondence by binding the actual parameters to the formal parameters based on their position in the sub-program call.
What are the advantages and disadvantages of using keyword parameters?
-The advantage of keyword parameters is that they allow parameters to be specified in any order, reducing the chance of errors due to incorrect order. A potential disadvantage is that they may require more code to specify the parameter names, which could make the code more verbose.
Why must default parameters appear last in the list of parameters in C++?
-In C++, default parameters must appear last to ensure that if the function is called with fewer arguments than there are parameters, the correct default values are used without ambiguity.
What is the difference between default parameters and a variable number of parameters?
-Default parameters allow for some parameters to be omitted from a call, using predefined values, while a variable number of parameters allows a sub-program to accept any number of arguments, typically managed as an array or list.
Why must all parameters be of the same type when using a variable number of parameters in C#?
-In C#, the use of the 'params' keyword requires that all parameters be of the same type to ensure consistency when they are treated as an array within the method.
What is the distinction between procedures and functions in terms of sub-programs?
-Procedures are sub-programs that perform actions and may produce results by modifying global variables or parameters passed by reference. Functions are sub-programs that return a value and are generally expected to produce no side effects, although in practice they often do.
What are some design issues related to sub-programs in high-level programming languages?
-Design issues include whether local variables are statically or dynamically allocated, whether sub-program definitions can be nested, the parameter parsing methods provided, parameter type checking, the referencing environment of passed sub-programs, allowing functional side effects, the return types allowed from functions, sub-program overloading, support for generic sub-programs, and closure support for nested sub-programs.
Outlines
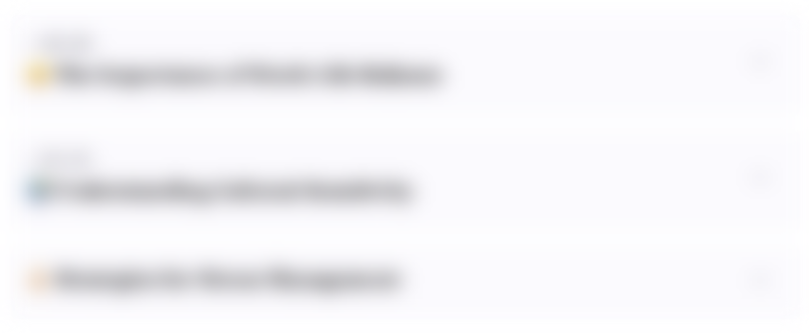
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
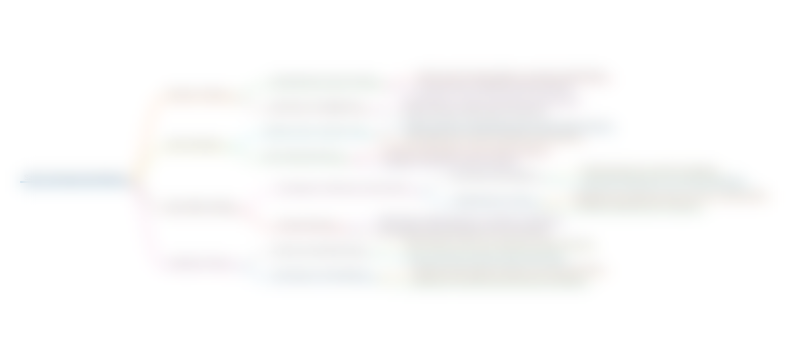
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
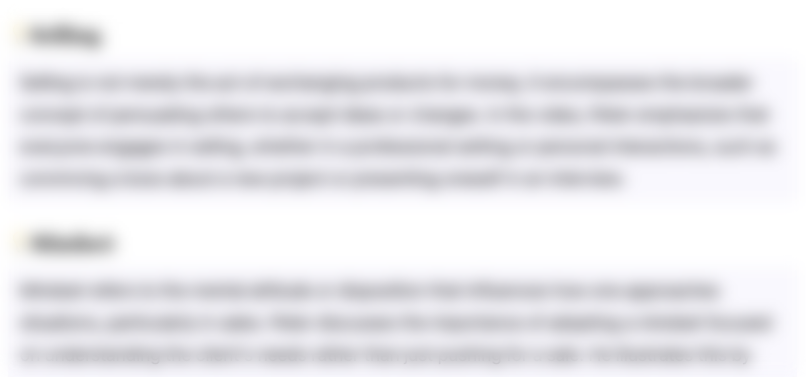
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
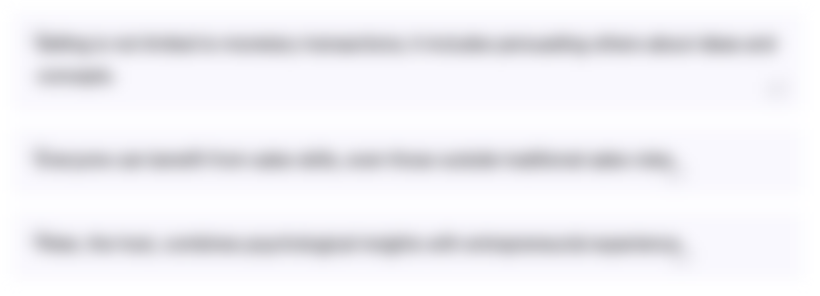
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
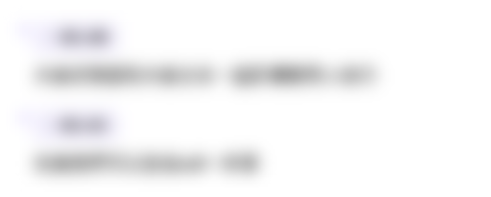
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)