COS 333: Chapter 9, Part 3
Summary
TLDRThis lecture concludes the discussion on sub-programs, focusing on overloaded sub-programs, generic sub-programs including C++ templates and Java generics, and design issues specific to functions. It delves into user-defined overloaded operators, closures, and coroutines, illustrating their practical applications and importance in programming. The lecture emphasizes the flexibility and power of these concepts in various programming languages, highlighting their role in creating efficient and versatile code.
Takeaways
- 📚 The lecture discusses the final part of Chapter Nine, focusing on sub-programs, including overloaded sub-programs, generic sub-programs, design issues related to functions, user-defined overloaded operators, closures, and coroutines.
- 🔄 Overloaded sub-programs have the same name but different protocols, which include parameter types, numbers, and return types. They are supported by languages like C++, Java, C#, and Ada, with some differences in how they handle return types.
- 📌 The concept of generic sub-programs, or polymorphic sub-programs, allows a sub-program to take different parameter types. C++ supports this with template functions, while Java uses generic methods, each with different underlying implementations.
- 🤖 C++ template functions are instantiated at compile time for each type used, whereas Java's generic methods work with a single runtime construct, using instances of the Object class for generic types.
- 🚫 Design issues for functions include side effects, the number of values that can be returned, and the types of return values allowed, which vary across languages like C, C++, Ada, Java, and C#.
- 🔧 User-defined overloaded operators allow programmers to specify their own implementations for existing operators for new types, supported in languages such as Ada, C++, Python, and Ruby.
- 🌌 Closures, as illustrated in JavaScript, capture the environment in which they were created, allowing the function to access those variables even outside their original scope.
- ⭐ Coroutines are sub-programs with multiple entry points, allowing execution to be passed back and forth between them, useful for simulations and creating quasi-concurrent execution.
- 🔄 The execution of coroutines can be resumed and suspended, allowing for an interleaved execution flow that can be ideal for certain types of applications, like traffic light simulation.
- 📘 The lecture also mentions that Chapter Ten, which covers the implementation of sub-programs, will be skipped, proceeding directly to Chapter Eleven on abstract data types and encapsulation.
Q & A
What is an overloaded sub-program?
-An overloaded sub-program is a sub-program that shares the same name with another sub-program in the same referencing environment, but has a different sub-program protocol, which includes a difference in the number, types of parameters, or the return type.
How do programming languages like C++, Java, C#, and Ada disambiguate overloaded sub-programs?
-These languages disambiguate overloaded sub-programs based on their parameter profile, meaning they look at the number and types of parameters involved in the call.
What is the impact of type coercion on disambiguating overloaded sub-programs?
-Type coercion can complicate the disambiguation process because it may change the types of parameters in a way that affects which overloaded version of a sub-program is selected.
Why can't the approach of using return types to disambiguate function calls work in languages like C++?
-In C++, overloaded sub-programs must differ in their parameter profiles; relying on differing return types alone is not sufficient to disambiguate them, as this could lead to redefinition conflicts.
What is a generic sub-program?
-A generic sub-program, also known as a polymorphic sub-program, is capable of taking different parameter types in different calls, supporting ad hoc polymorphism through overloading and parametric polymorphism through generic parameters.
How do template functions in C++ work behind the scenes?
-In C++, template functions create different versions of the function at compile time for each type that is used with the function, allowing for type-specific behavior.
What is the difference between template functions in C++ and generic methods in Java?
-Template functions in C++ create a new function version for each type at compile time, while Java's generic methods are instantiated once at runtime, using instances of the Object class for generic types.
Why must generic parameters in Java be classes and not primitive data types?
-Java's generic methods work with instances of the Object class behind the scenes, so they must be classes. Primitive types cannot be used because they are not objects and do not inherit from the Object class.
What are some design issues specific to functions?
-Design issues specific to functions include the allowance of side effects, the number of values that can be returned, and the types of return values permitted by the programming language.
How do closures work in JavaScript?
-Closures in JavaScript are created by factory functions that return anonymous functions. These closures capture the scope in which they were created, allowing them to access variables from outer functions even after those functions have finished executing.
What is a co-routine and how does it differ from a regular sub-program?
-A co-routine is a sub-program with multiple entry points that controls its own execution flow. It differs from regular sub-programs by allowing a more equal relationship between the caller and the called, and it can be paused and resumed at specific points, facilitating a form of interleaved execution.
Outlines
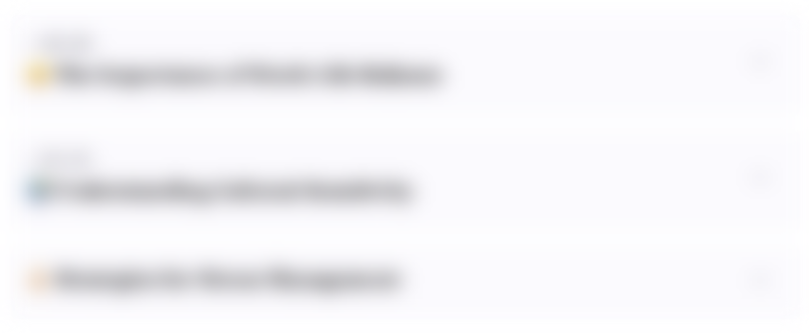
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
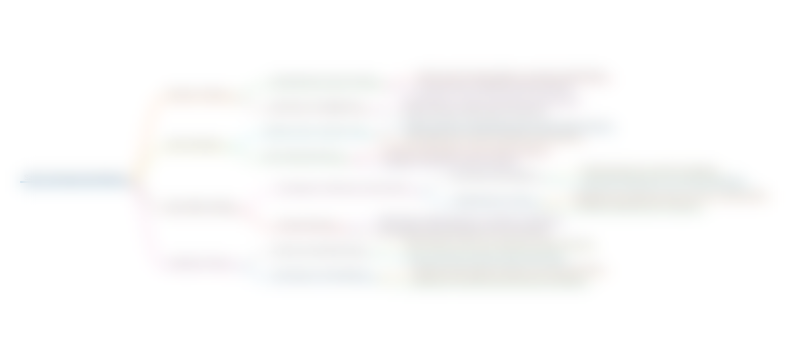
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
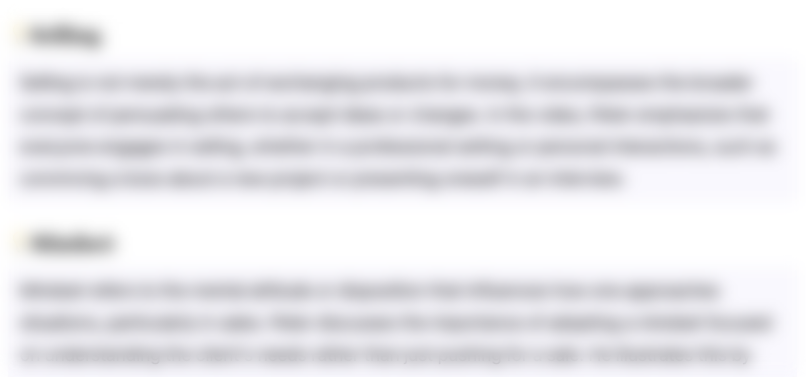
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
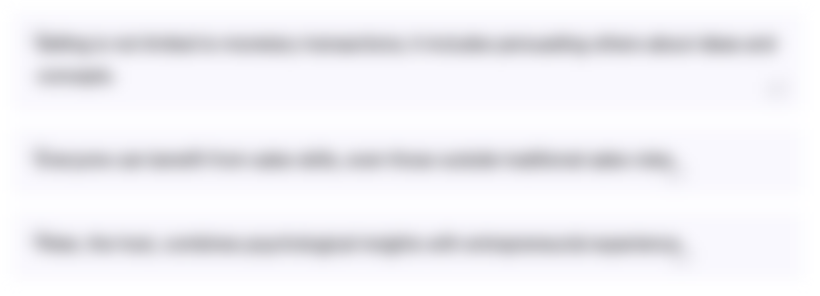
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
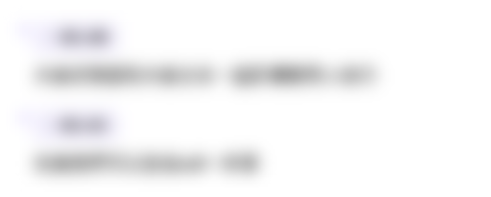
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)