What's an algorithm? - David J. Malan
Summary
TLDRThis script explores the concept of algorithms, both in computer science and everyday human activities. It uses the example of counting people in a room to illustrate the step-by-step process of an algorithm. The video demonstrates two versions of counting algorithms, one basic and one optimized for pairs, highlighting the importance of correctness and addressing potential errors. It concludes by emphasizing the universality of algorithms in problem-solving, inviting viewers to consider their own applications.
Takeaways
- 📘 An algorithm is a step-by-step set of instructions to solve a problem, typically executed by computers but also used by humans.
- 👤 The example of counting people in a room illustrates a simple human algorithm where one counts individuals sequentially.
- 🔢 Pseudocode is an English-like syntax that represents a programming language, used to express algorithms more formally.
- 💡 The script demonstrates initializing a variable 'n' to zero, which represents the starting point of counting before any individuals are counted.
- 🔁 The concept of a loop is introduced, where a set of steps is repeated a certain number of times, corresponding to the number of people in the room.
- 🔄 The pseudocode explains incrementing the count 'n' by 1 for each person, which is the core of the counting algorithm.
- 🤔 The script questions the correctness of the algorithm, testing it with different scenarios, including 2 people and 0 people in the room.
- 🔄 An optimization is proposed to count in pairs, doubling the counting speed, but it introduces a potential error with odd numbers of people.
- 🛠 The script corrects the counting in pairs algorithm by adding a condition to handle the case where one person remains unpaired.
- 🔢 The importance of considering edge cases, such as zero or odd numbers of people, is highlighted to ensure algorithm accuracy.
- 🚀 The script concludes by suggesting that algorithms can be adapted to count in larger groups, but it becomes more challenging with larger numbers.
Q & A
What is an algorithm in the context of computer science?
-An algorithm in computer science is a set of instructions designed to solve a specific problem in a step-by-step manner, which is typically executed by computers.
Do humans also use algorithms in their daily activities?
-Yes, humans use algorithms too, although they might not be as formalized or structured as those used in computer programming. For example, counting the number of people in a room is an everyday human algorithm.
How is the process of counting people in a room described as an algorithm?
-The process is described as an algorithm by starting at zero and incrementing a counter for each person pointed at, which is a systematic and repeatable method to determine the number of people present.
What is pseudocode and why is it used?
-Pseudocode is an informal high-level description of an algorithm in English-like syntax that resembles a programming language. It is used to express the logic of an algorithm in a way that is easily understandable by humans before it is translated into actual code.
How does the script illustrate the concept of a loop in an algorithm?
-The script uses the example of counting people in a room to illustrate a loop, where the action of incrementing the count 'n' is repeated for each person in the room.
What is the purpose of initializing a variable to zero in the algorithm?
-Initializing a variable to zero sets a starting point for counting, ensuring that the algorithm begins with a known state before it starts processing the input data.
How does the script handle the corner case of an empty room?
-The script handles the corner case by initializing the count 'n' to zero and not executing the increment step since there are no people in the room, resulting in 'n' remaining zero, which correctly reflects the count of people.
What is the optimization proposed in the script to improve counting efficiency?
-The optimization proposed is to count in pairs (or twos) instead of counting individuals one by one, effectively doubling the counting speed for each iteration of the loop.
What issue arises when counting in pairs and how is it addressed in the script?
-The issue arises when there is an odd number of people, and the algorithm fails to account for the last unpaired individual. The script addresses this by adding a conditional branch to increment 'n' by one if there is a remaining person after pairing.
What is the significance of the final algorithm presented in the script?
-The final algorithm is significant as it provides a more robust solution that can handle any number of people in the room, whether even or odd, by using both pairing and a conditional check for a remaining individual.
How does the script suggest that algorithms can be applied to various problems?
-The script suggests that algorithms can be applied to various problems by demonstrating how different counting strategies (like counting in ones, twos, or other numbers) can be formulated as algorithms to solve the problem of counting people in a room.
Outlines
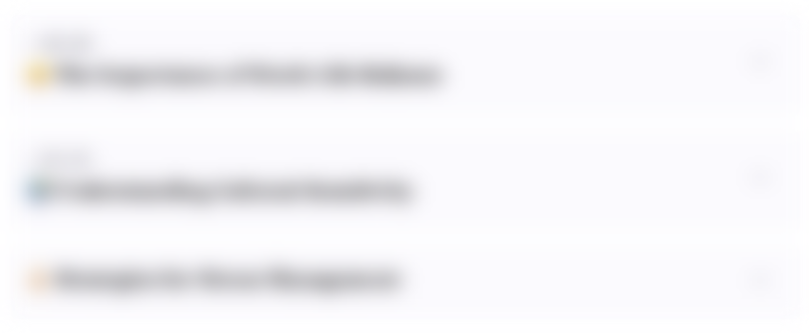
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
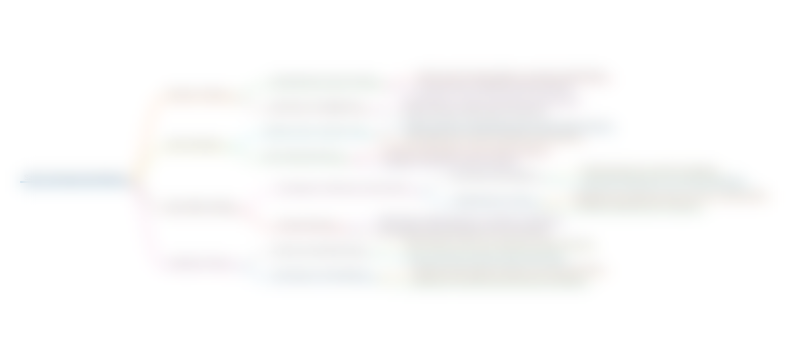
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
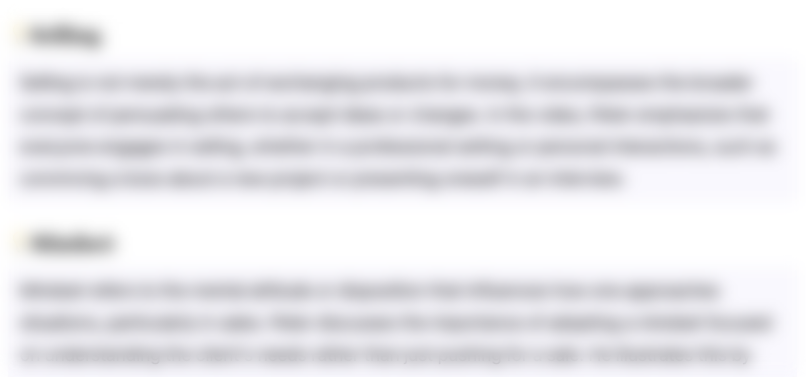
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
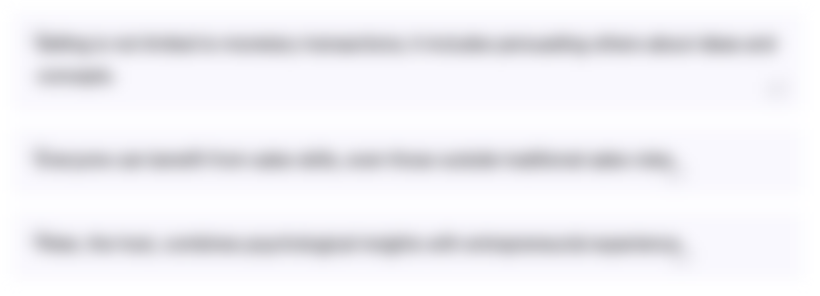
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
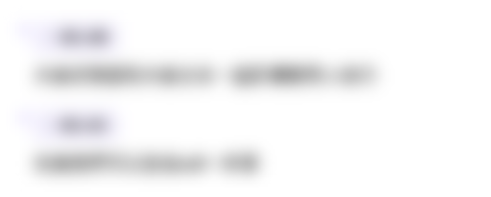
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)