Algorithms Explained for Beginners - How I Wish I Was Taught
Summary
TLDRThis video script emphasizes the significance of algorithms in the field of computer science and coding interviews. It explains that algorithms are a set of instructions to achieve a goal, and their efficiency is crucial when dealing with massive datasets as encountered by tech giants like Google and Facebook. The script introduces two search algorithms: linear search, which is straightforward but slow (O(n)), and binary search, which is faster (O(log n)) by narrowing down the search space significantly with each step. The importance of algorithm design is highlighted, as it can lead to substantial performance gains, even surpassing a decade's worth of hardware improvements. The video also touches on sorting algorithms, mentioning the inefficiency of basic algorithms compared to optimized ones like merge sort. It concludes with recommendations for learning more about algorithms through courses like CS50, online platforms like Zero to Mastery, and books like 'Cracking the Coding Interview', as well as the practical advice of solving problems on platforms like LeetCode.
Takeaways
- 📘 Algorithms are a series of steps to perform an action or reach a goal, often involving data processing.
- 🚀 The importance of algorithms is highlighted by their central role in coding interviews and the tech industry's focus on them.
- 🧠 Designing efficient algorithms can allow us to solve much larger problems and achieve significant performance gains in technology.
- 📈 The speed and memory usage of an algorithm are crucial factors, especially when dealing with large datasets as seen in companies like Google and Facebook.
- 🔍 Linear search is a simple algorithm that checks each element in a list sequentially, but it's not efficient for large data sets.
- 🔑 Big O notation is used to express the worst-case scenario of an algorithm's running time, which is essential for analyzing scalability.
- ⏬ The binary search algorithm is more efficient than linear search as it reduces the problem size by half with each step, with a running time of O(log n).
- 📚 Reading books or summaries like 'Algorithms to Live By' can provide insights into applying algorithmic thinking to everyday life.
- 🎓 Taking courses like CS50, Zero to Mastery, Stanford's Algorithm Specialization, and Princeton's Algorithms Specializations can build a strong foundation in algorithms.
- 📝 'Cracking the Coding Interview' is a valuable resource for reviewing key topics and practicing problems before interviews.
- 💻 Regular practice on platforms like LeetCode is essential for mastering data structures and algorithms.
Q & A
What is the primary focus of the video?
-The video focuses on explaining the importance of algorithms, why they are crucial in coding interviews, and how understanding them can help solve complex problems more efficiently.
Why do companies place a significant emphasis on algorithms during their recruitment process?
-Companies emphasize algorithms because they are fundamental to solving large-scale problems that these companies face. The ability to design and understand efficient algorithms can lead to significant performance gains and is indicative of a candidate's problem-solving skills.
What is the basic definition of an algorithm?
-An algorithm is a series of steps designed to perform a specific action or to reach a particular goal. It involves taking some input data and determining the logical steps a computer must take to produce the desired output.
Why is it important to study the speed of algorithms even when computers are very fast?
-The speed of algorithms becomes critical when dealing with large datasets, as seen in companies like Google and Facebook. Even with fast computers, a poorly designed algorithm can lead to significantly longer processing times, which can affect the performance and efficiency of technology solutions.
What does the term 'Big O' refer to in the context of algorithms?
-In computer science, 'Big O' refers to the worst-case scenario running time of an algorithm. It is a measure of how the runtime of an algorithm grows with the size of the input data, and it is used to predict the performance of an algorithm as the input size increases.
How does the linear search algorithm work?
-The linear search algorithm works by sequentially checking each element in a list or book until the desired item is found. It is a straightforward but inefficient method, especially for large datasets, as it may require checking every single item in the worst-case scenario.
What is the main advantage of a binary search algorithm over a linear search?
-The binary search algorithm has a significant advantage over linear search in terms of efficiency. It works by repeatedly dividing the search space in half, which reduces the problem size with each step. This approach results in a logarithmic running time (O(log n)), which is much faster than the linear time (O(n)) of a linear search for large datasets.
What are some resources recommended in the video for mastering algorithms and data structures?
-The video recommends several resources, including CS50's third lecture, 'Zero to Mastery' coding courses, Stanford's Algorithm Specialization, Princeton's Algorithms I and II specializations, and the book 'Cracking the Coding Interview' for practice problems and interview preparation.
How does the video suggest one should approach learning algorithms and data structures?
-The video suggests starting with a solid theoretical foundation through courses like CS50 or specialized algorithm courses. After gaining a theoretical understanding, one should then practice with resources like 'Cracking the Coding Interview' and engage in a lot of coding problems on platforms like LeetCode.
What is the role of Short Form in the video?
-Short Form is mentioned as a sponsor of the video. They provide high-quality book summaries and study guides, which can be useful for quickly understanding the main ideas and concepts of a book before deciding to read it in full.
Why is it beneficial to understand the theory behind algorithms?
-Understanding the theory behind algorithms is beneficial because it allows one to write efficient and effective code for solving complex problems. It also helps in performing well in coding interviews, which often focus on algorithmic thinking and problem-solving skills.
Outlines
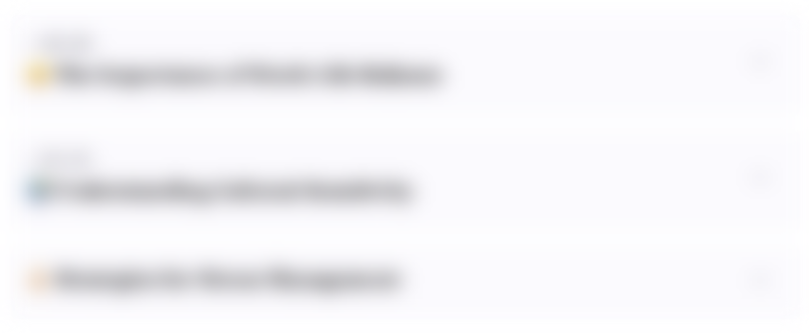
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
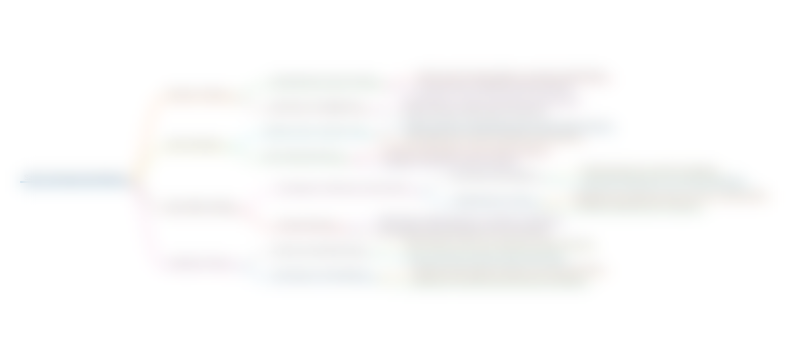
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
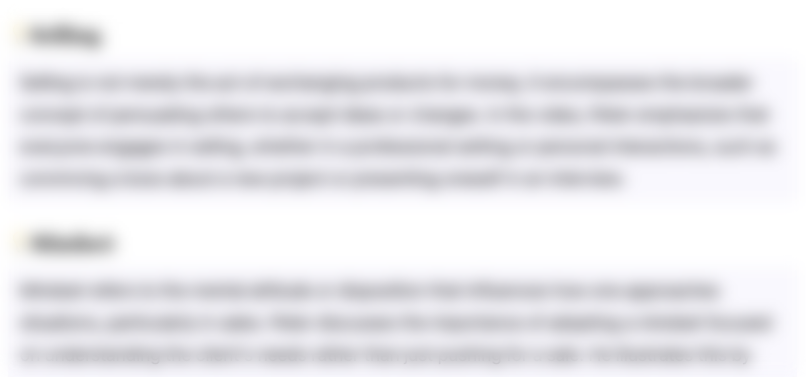
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
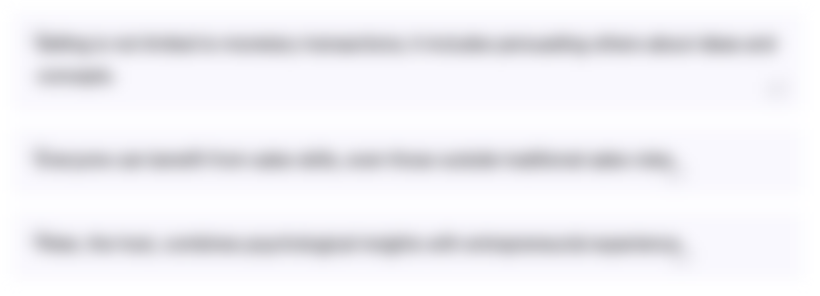
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
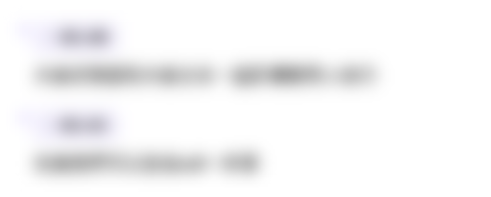
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
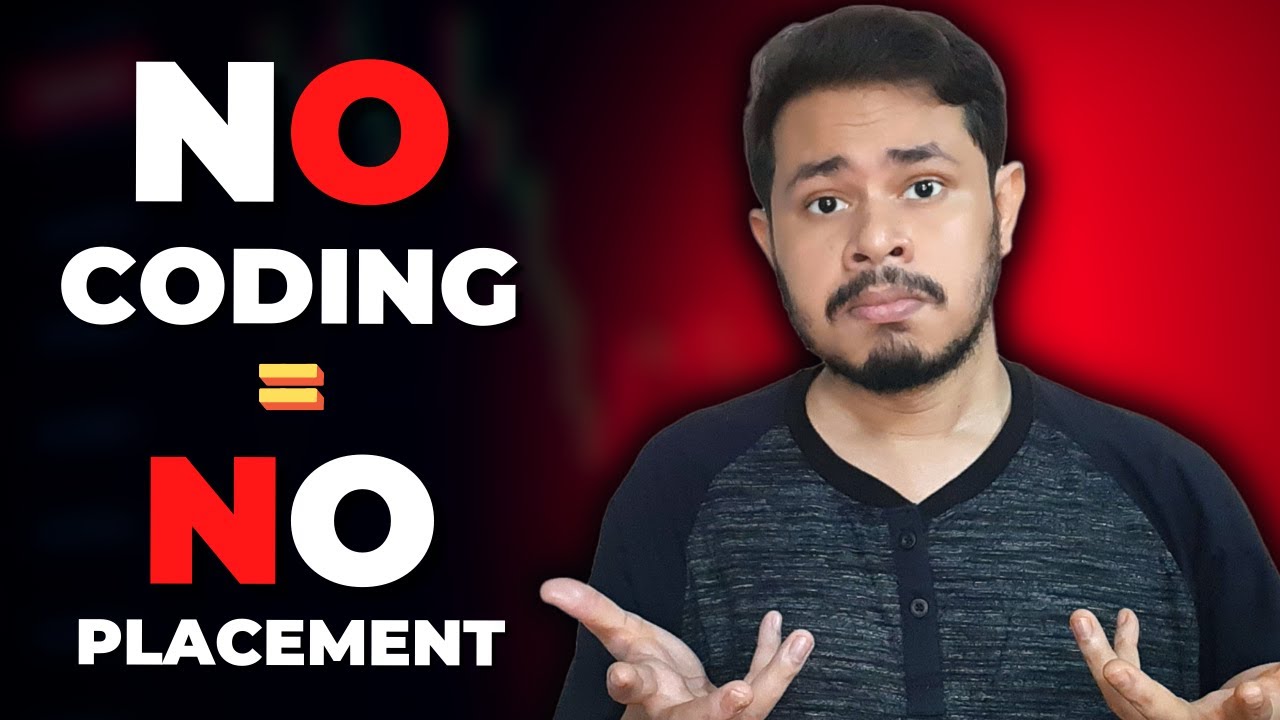
How much Coding *actually* required in MTech at IIT?

90 DAYS PLACEMENT ROADMAP🔥| Step by Step Guide to CRACK ANY COMPANY | DSA, PROJECTS, CS SUBJECTS🧑💻🚀
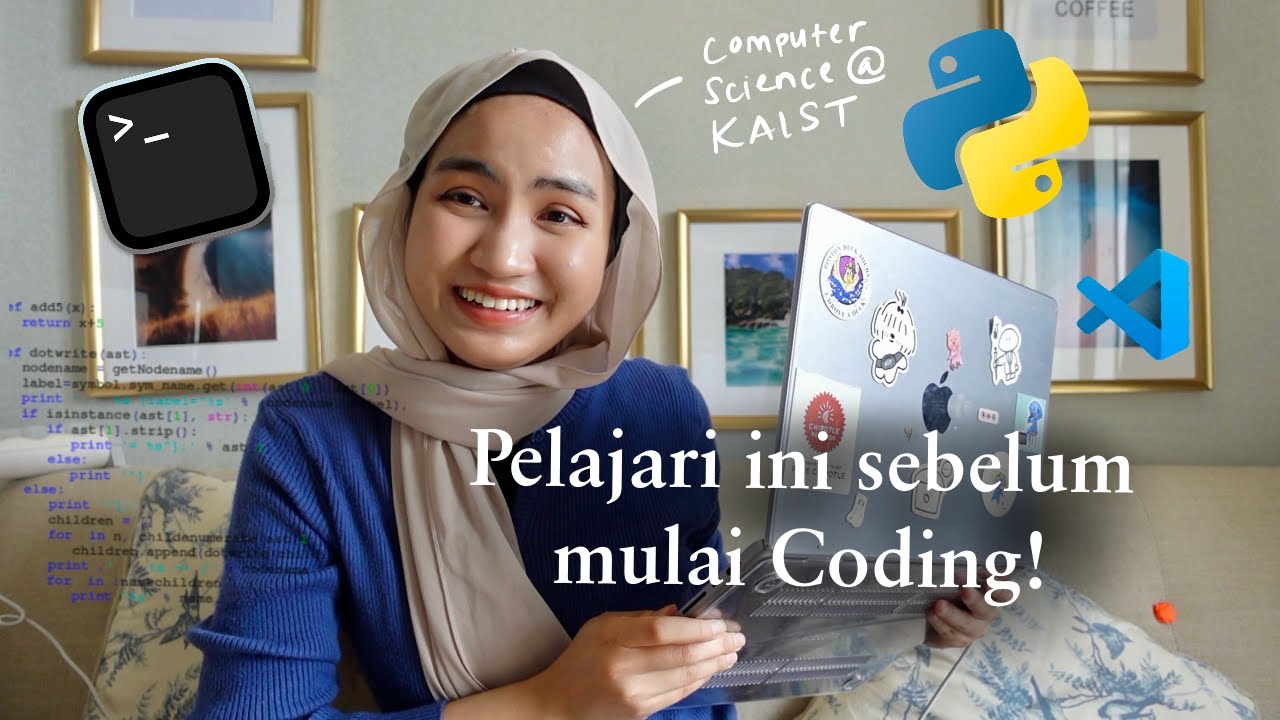
Full Guide Belajar CODING untuk Pemula 💻📚

Every Computer Science Student Should Know these 5 Subjects | Siddharth Singh
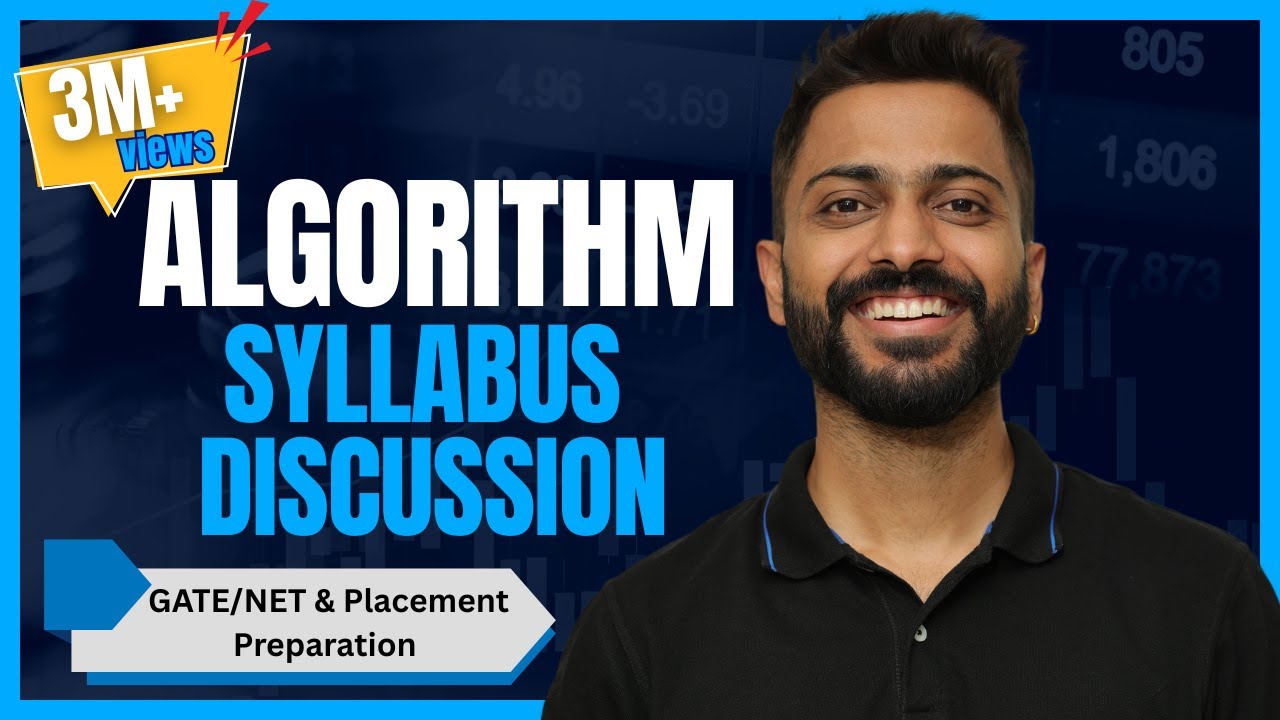
L-1.1: Introduction to Algorithm & Syllabus Discussion for GATE/NET & Placements Preparation | DAA
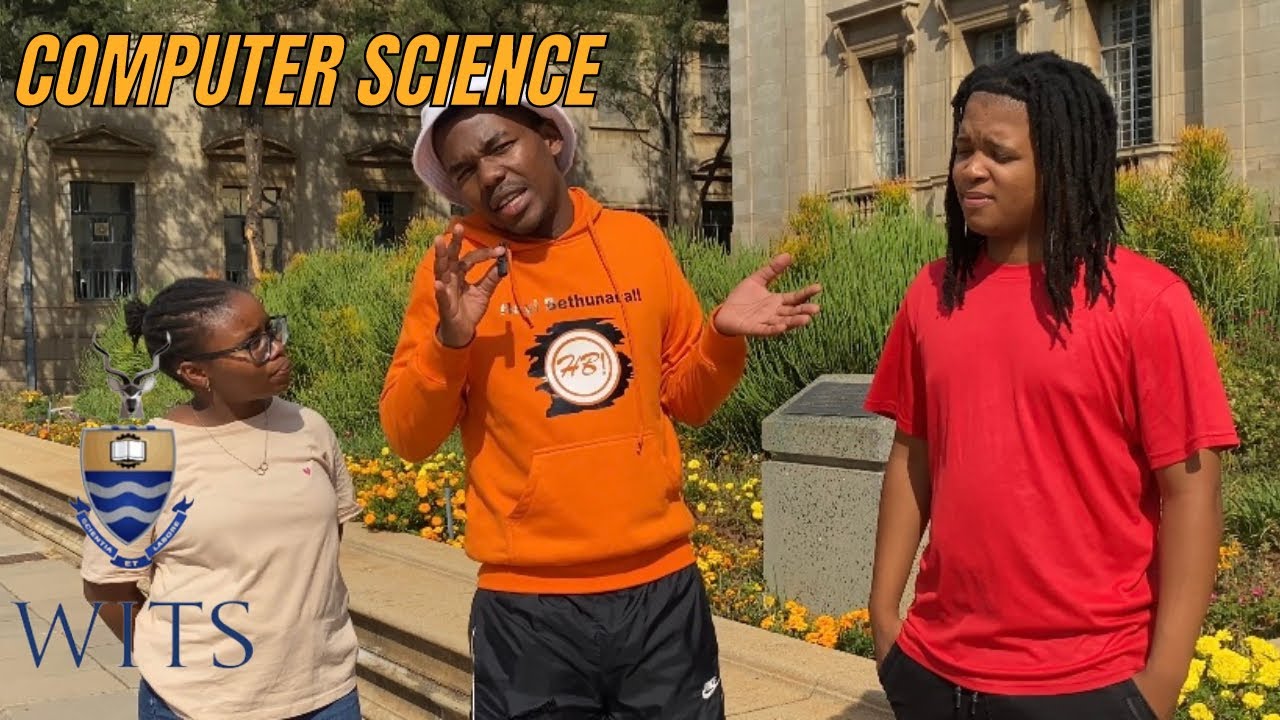
WITS Computer Science |FINAL YEAR STUDENTS | Study Tips
5.0 / 5 (0 votes)