Python Karel Algorithms
Summary
TLDRThis video script delves into the concept of algorithms, emphasizing their importance in computer science and programming. It illustrates algorithms with everyday examples like recipes and directions, and uses the 'hokey-pokey' dance as a playful example. The script explains how algorithms are translated into programs, highlighting the building blocks of sequencing, iteration, and selection. It also touches on the significance of efficiency and clarity in algorithm design, and introduces pseudocode as a bridge between natural language and code. The process of developing an algorithm is outlined, from understanding the problem to defining it in natural language, pseudocode, and finally, code.
Takeaways
- π An algorithm is a self-contained, step-by-step set of instructions designed to solve a problem.
- π Algorithms are a fundamental concept in computer science and are commonly used in programming.
- π½οΈ Real-world examples of algorithms include recipes and directions, illustrating the concept's universality.
- π The 'hokey-pokey' dance is used as a fun example to demonstrate a step-by-step algorithmic process.
- π₯ͺ The process of making a peanut butter and jelly sandwich is also an example of an algorithm.
- π In the context of programming, moving a character to a wall is an example of an algorithm implemented in code.
- π€ Algorithms are the conceptual ideas that solve problems, while programs are their physical, computer-executable implementations.
- π Algorithms are constructed using three main techniques: sequencing, iteration, and selection.
- π Sequencing is the execution of instructions in the order they are given, which is the natural flow of a program.
- π Iteration involves repeating instructions either a set number of times or until a condition is met, using loops in code.
- π οΈ Selection uses conditions to determine which part of the algorithm gets executed, often implemented with if-else statements.
- π When comparing algorithms, efficiency (speed) and clarity (readability) are important criteria for evaluation.
- π Writing algorithms is crucial for complex programming; it's easier to correct a program when the algorithm is well-defined beforehand.
- π The problem-solving process should start with developing an algorithm in natural language, then pseudocode, and finally, actual code.
- π Pseudocode is a bridge between natural language and code, helping to clarify the algorithm before coding.
- π Algorithms can be nested, using other algorithms as part of their process, as seen in the 'run race' example.
Q & A
What is an algorithm according to the video?
-An algorithm is a self-contained, step-by-step set of instructions designed to solve a problem, and it is a fundamental concept in computer science and programming.
Can you give some real-world examples of algorithms mentioned in the video?
-Yes, examples include recipes for preparing a dish, directions for getting somewhere, and the steps of the Hokey-Pokey dance.
What is the relationship between an algorithm and a program?
-An algorithm is the conceptual idea that solves a problem, while a program is the implementation of that algorithm in a form that a computer can execute.
What are the three building blocks of all algorithms?
-The three building blocks of algorithms are sequencing, iteration, and selection.
Can you explain what sequencing is in the context of algorithms?
-Sequencing is the step-by-step execution of instructions in the order they are given, which is the natural flow of a program executing line by line.
What is iteration in algorithms, and how is it implemented in code?
-Iteration is the repetition of instructions either a specified number of times or until a certain condition is met. In code, iteration is implemented using control structures like for loops or while loops.
How is selection different from sequencing in algorithms?
-Selection involves using a condition to determine which part of the algorithm gets executed, unlike sequencing which follows instructions in the given order. Selection is implemented in code using if-else statements.
What are the two main factors to consider when comparing algorithms?
-The two main factors are efficiency, which refers to how fast the algorithm is, and clarity, which pertains to how readable and understandable the algorithm is.
Why is it important to develop an algorithm before writing the actual code?
-Developing an algorithm beforehand helps in creating a clear and structured approach to problem-solving, making it easier to write correct and efficient code.
What is pseudocode and why is it used in the problem-solving process?
-Pseudocode is a way of writing out an algorithm in a language that resembles code but is not tied to any specific programming language. It helps in outlining the logic of the program before translating it into actual code.
Can you describe the problem-solving process with algorithms as outlined in the video?
-The problem-solving process involves understanding the problem, developing the algorithm in natural language, writing pseudocode to outline the program structure, and finally translating the pseudocode into actual code that can be executed by a computer.
Outlines
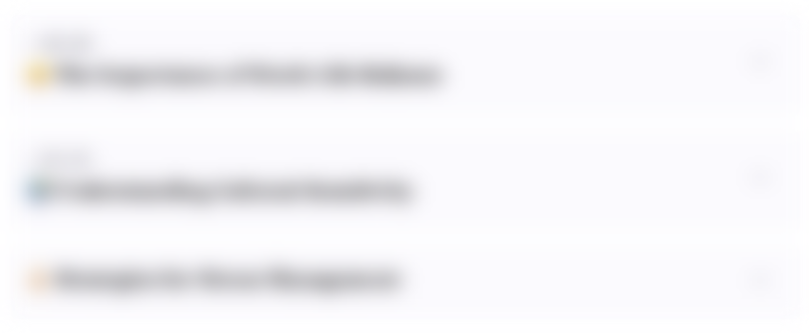
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
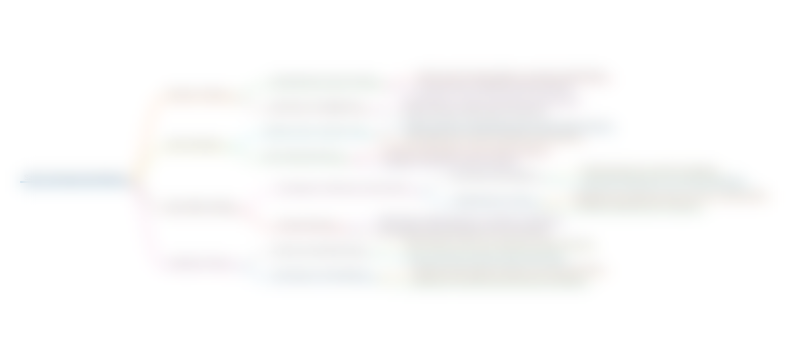
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
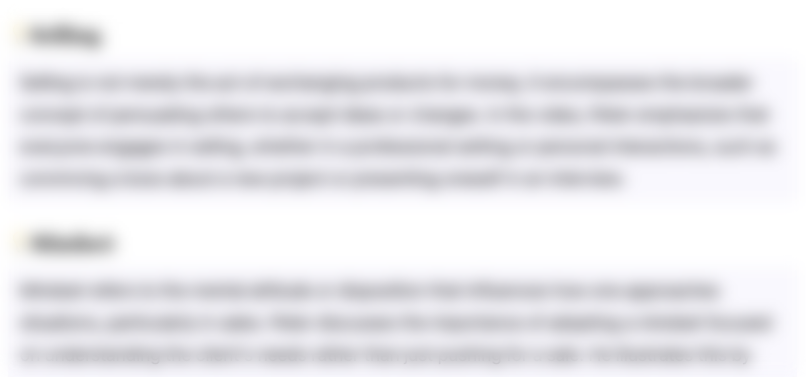
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
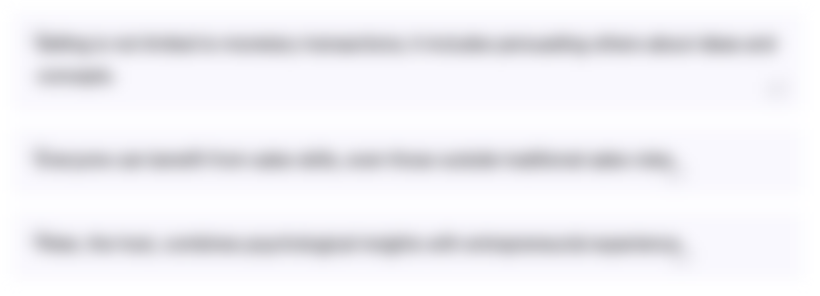
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
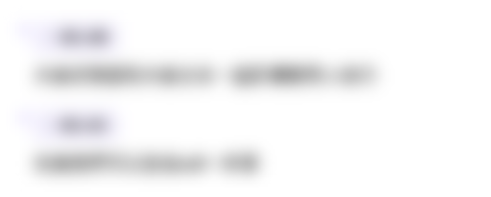
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)