Algorithm Design | Algorithm Correctness #algorithm #algorithmdesign
Summary
TLDRThis educational video session delves into the importance of algorithm correctness, explaining that an algorithm is considered correct if its output matches the expected result. The instructor uses a simple example involving even numbers to illustrate the concept. The video outlines three primary methods for ensuring correctness: counterexamples, mathematical induction, and loop invariants. It emphasizes the necessity of total correctness over partial correctness and provides an example of an infinite loop to highlight the importance of a stopping condition in algorithms. The session aims to help viewers understand the principles behind creating and verifying accurate algorithms.
Takeaways
- 📘 Algorithm correctness is crucial because it ensures that the output of an algorithm matches the expected outcome.
- 🔍 Total correctness is the goal, as it means the algorithm produces the desired output for all possible inputs, while partial correctness indicates the algorithm may only work correctly for some inputs.
- 🔄 An infinite loop can occur if there is no increment or decrement operator within the loop, causing it to run indefinitely and not reach the stopping condition.
- 🔢 The importance of a stopping property in loops is highlighted, as it ensures the algorithm will terminate and provide a correct output.
- 🔑 Total correctness can be achieved by ensuring both partial correctness and the stopping property are met.
- 🔍 Three methods for proving algorithm correctness are discussed: counterexample, mathematical induction, and loop invariant.
- 🚫 Counterexamples serve as indirect proofs by providing an instance where a statement is false, thus disproving it.
- 📚 Mathematical induction is a direct proof method used to prove that a statement holds for all cases, starting with a base case and then proving the inductive step.
- 🔄 Loop invariants are conditions that are true before, during, and after the execution of a loop, ensuring that the loop behaves correctly and terminates as expected.
- 📉 The script provides an example of a summation formula represented as a mathematical model and discusses its time complexity implications.
- 📝 The presenter encourages students to understand the concepts of total and partial correctness, and to reach out if they have any questions or issues.
Q & A
What is the primary importance of algorithm correctness?
-Algorithm correctness is crucial because it ensures that the output of an algorithm matches the expected output, which is the desired outcome for a given problem.
What are the two main types of algorithm correctness discussed in the script?
-The two main types of algorithm correctness discussed are total correctness and partial correctness.
Why is total correctness preferred over partial correctness?
-Total correctness is preferred because it guarantees that the algorithm consistently produces the correct output for all possible inputs, whereas partial correctness may only be true for some inputs.
What is an example of an incorrect algorithm output mentioned in the script?
-An example given is a code to determine if a number is even. If the input is 10 and the output is 'odd', it is incorrect because the expected output should be 'even'.
What is the significance of the stopping property in the context of algorithm correctness?
-The stopping property ensures that an algorithm will terminate after a finite number of steps and produce the correct output, preventing infinite loops.
Can you explain the concept of a loop invariant as discussed in the script?
-A loop invariant is a condition that remains true before and after each iteration of a loop and upon termination of the loop. It helps in proving the correctness of an algorithm by ensuring that the loop maintains the invariant properties throughout its execution.
What are the three steps involved in checking a loop invariant?
-The three steps are the initial step (ensuring the loop starts correctly), the maintenance step (ensuring the loop maintains the invariant during each iteration), and the end step or stop step (ensuring the loop stops under the correct conditions).
What is the purpose of using counterexamples in proving algorithm correctness?
-Counterexamples are used to disprove a statement by providing an instance where the statement does not hold true, which is an indirect proof method.
How does mathematical induction differ from counterexamples in proving algorithm correctness?
-Mathematical induction is a direct proof method where one proves the base case and then assumes the statement is true for a certain case 'n' to prove it must also be true for 'n+1'. In contrast, counterexamples use specific instances to disprove a statement.
What is the relationship between total correctness and the stopping property?
-Total correctness is achieved when an algorithm is partially correct and adheres to the stopping property, which ensures the algorithm terminates with the correct output.
Can you provide an example of how mathematical induction is used in the script?
-The script uses the example of proving the summation of the first 'n' integers formula. It starts with the base case (n=1), assumes the formula holds for 'n', and then proves it for 'n+1', demonstrating the direct proof method of mathematical induction.
Outlines
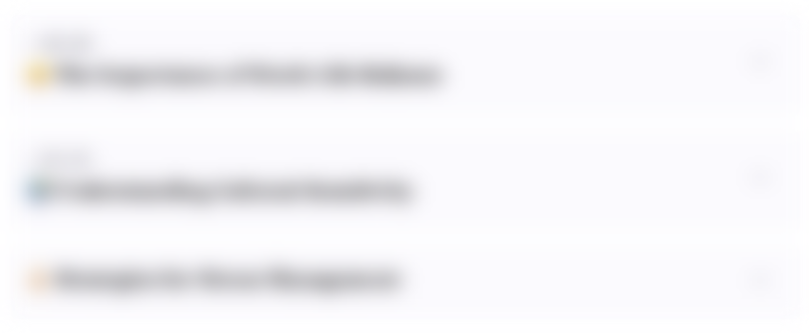
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
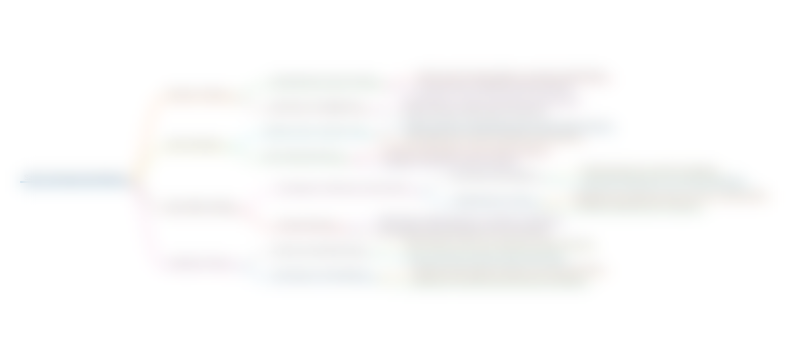
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
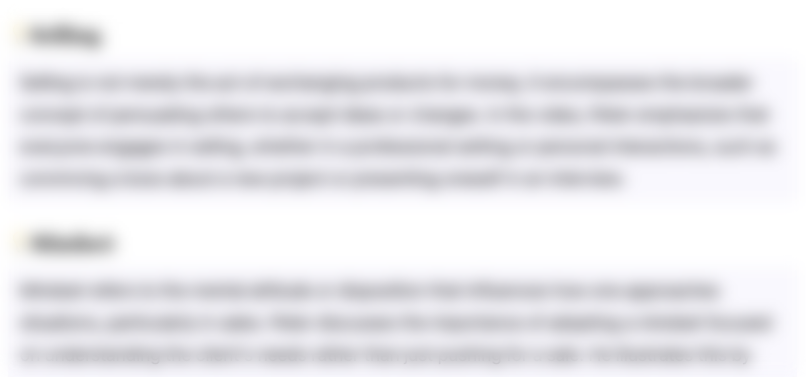
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
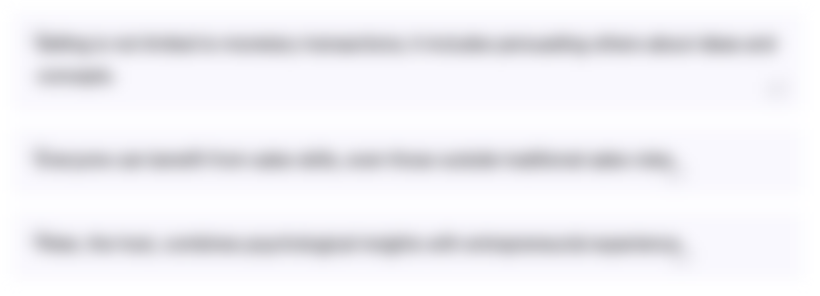
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
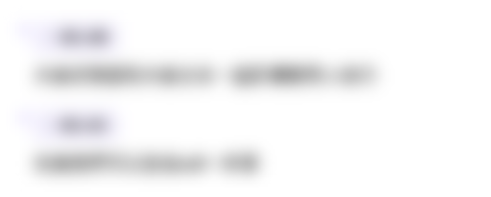
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
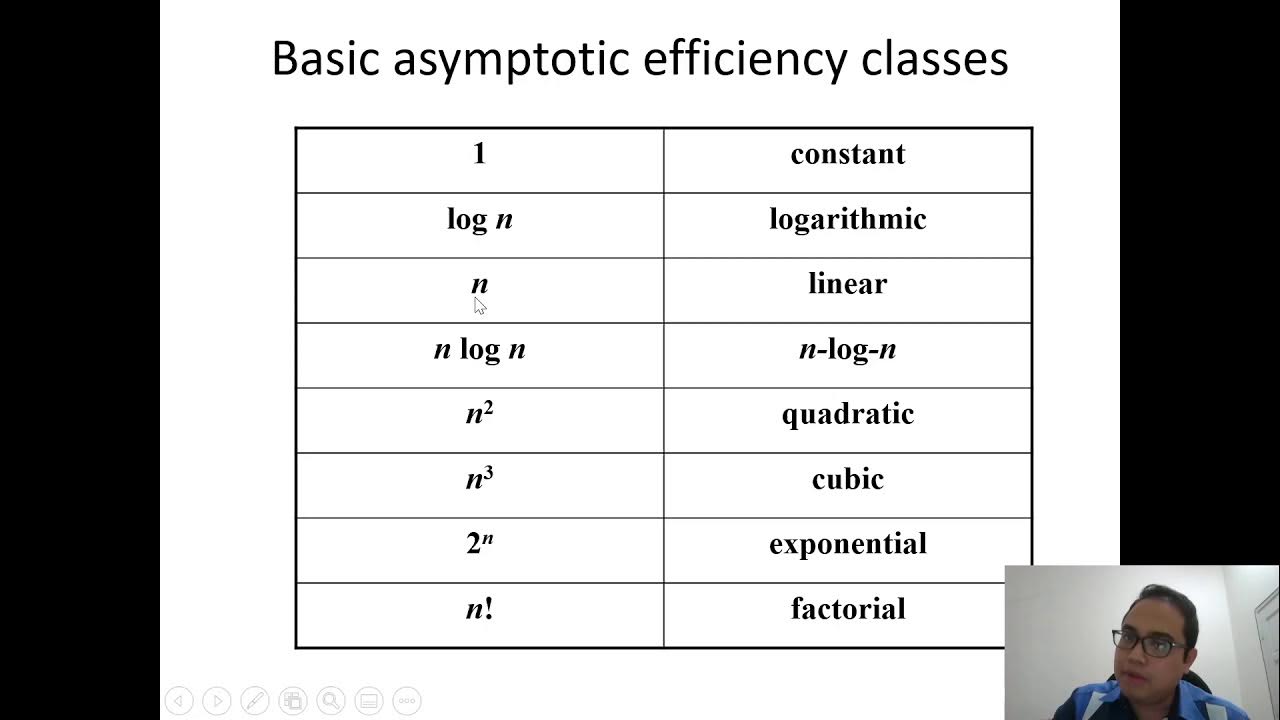
CSC645 - Chapter 1 (Continued) - Fundamentals of Algorithm Analysis
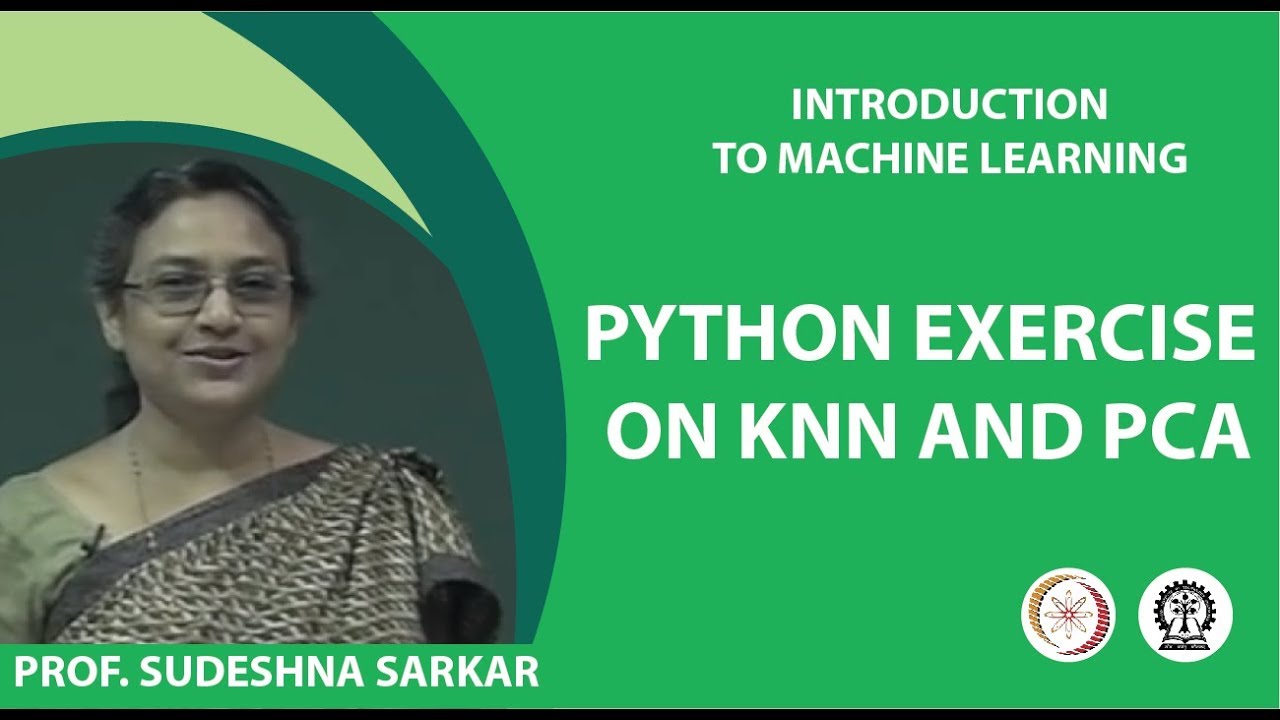
Python Exercise on kNN and PCA
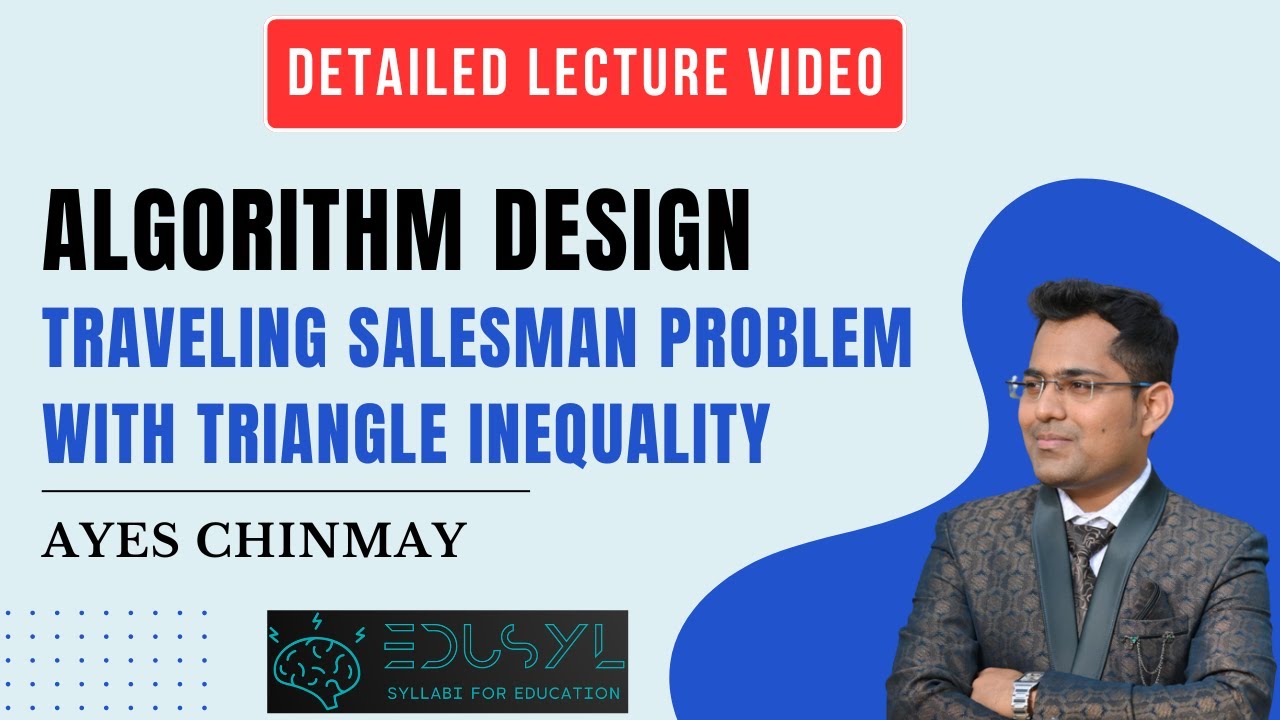
Algorithm Design | Approximation Algorithm | Traveling Salesman Problem with Triangle Inequality

ALGORÍTIMO DO YOUTUBE MUDOU! POR ISSO CANAIS COM MILHÕES DE INSCRITOS ESTÃO TENDO POUCAS VIEWS

Otimizando parâmetros com Irace
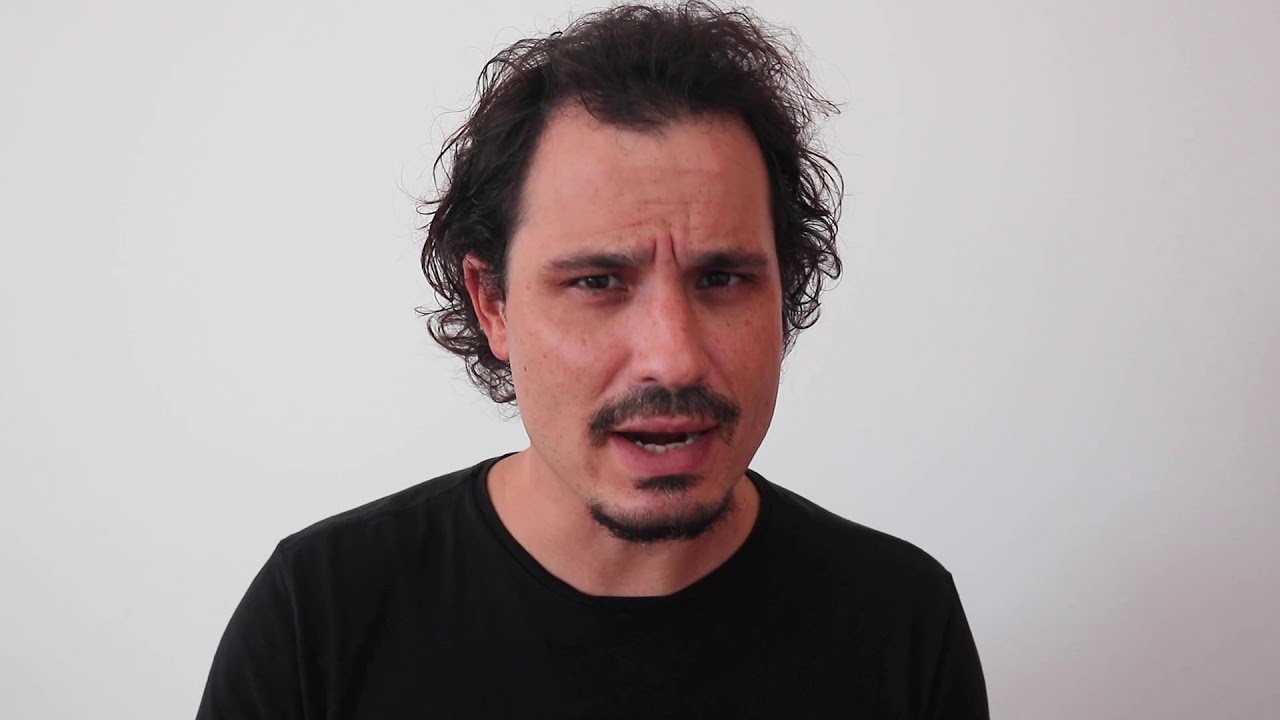
EDB1 IMD UFRN (2020.6): Ordenação por Bolha
5.0 / 5 (0 votes)