Important questions for Data Structure Mca 2nd sem
Summary
TLDRThis video covers a range of essential computer science topics, focusing on algorithms and data structures. It explores key concepts such as arrays, stacks (push/pop operations), sorting algorithms like Quick Sort, tree construction using preorder and inorder traversals, and circular queues. The video also delves into graph representations, DFS vs. BFS, hashing techniques (double hashing and quadratic probing), and postfix evaluation. It includes practical algorithms for various data structures, as well as important notes on priority queues and double-ended queues (deque). Aimed at students and professionals, the content offers both theoretical insights and practical applications.
Takeaways
- 😀 Difference between arrays and English structures, focusing on the unique aspects of arrays in programming.
- 😀 Reversing a linked list is a crucial operation, particularly when discussing its implementation and significance in data manipulation.
- 😀 Understanding push and pop operations is essential for stack management, with corresponding algorithms to perform these tasks.
- 😀 Quick sort algorithm is a fundamental sorting technique, along with its time complexity and a practical example to illustrate its use.
- 😀 Tree construction can be done from in-order and pre-order traversals, a key concept for building binary trees.
- 😀 B-trees are often used for efficient database indexing, and mathematical problems related to B3 trees are important to understand.
- 😀 Circular queue operations like insertion and deletion need step-by-step algorithms to manage the queue effectively.
- 😀 DFS (Depth-First Search) and BFS (Breadth-First Search) are essential graph traversal algorithms, each with its own advantages and use cases.
- 😀 Double hashing and quadratic probing are techniques used to resolve hash collisions, with examples and time complexities to better understand them.
- 😀 Graph representation methods like adjacency list and adjacency matrix offer different approaches for storing and manipulating graph data.
- 😀 Postfix evaluation is a key algorithm in evaluating mathematical expressions, and it's essential to understand how to implement this.
- 😀 Deleting a node from a doubly linked list requires an algorithm to handle the removal properly without breaking the list structure.
- 😀 Abstract data types (ADT) are a fundamental concept in computer science, and priority queues, selection sort, and double-ended queues (deque) are practical applications of this concept.
Q & A
What is the difference between an array and a linked list?
-An array is a collection of elements stored in contiguous memory locations, allowing direct access to elements via indices. A linked list, on the other hand, is a linear data structure where each element (node) contains a data part and a reference (link) to the next node. Arrays offer faster access time, while linked lists allow for dynamic memory allocation and easier insertion/deletion.
Can you explain the push and pop operations in data structures?
-Push and pop are operations commonly associated with stacks. The 'push' operation adds an element to the top of the stack, while 'pop' removes the topmost element. These operations follow the Last-In-First-Out (LIFO) principle, meaning the most recently added element is the first to be removed.
What is the Quick Sort algorithm and its time complexity?
-Quick Sort is a divide-and-conquer sorting algorithm. It works by selecting a pivot element, partitioning the array around the pivot, and recursively sorting the subarrays. Its average time complexity is O(n log n), but in the worst case, it can be O(n^2) if the pivot selections are poor.
How do you construct a tree from in-order and pre-order traversals?
-To construct a tree from in-order and pre-order traversals, first, use the root element from the pre-order list (since it appears first). Find this root in the in-order list. The nodes to the left of this root in the in-order list belong to the left subtree, and those to the right belong to the right subtree. Recursively repeat this process for each subtree.
What are some mathematical problems related to Binary Search Trees (BST)?
-Mathematical problems related to Binary Search Trees include calculating the height of a tree, finding the lowest common ancestor (LCA), checking if a tree is balanced, and performing operations like insertion, deletion, and searching, all of which depend on the BST properties where the left child is smaller and the right child is larger than the parent node.
How do insertion and deletion work in a circular queue?
-In a circular queue, both insertion and deletion operations are done at the rear and front of the queue, respectively. Insertion (enqueue) involves adding an element at the rear and incrementing the rear pointer, while deletion (dequeue) removes an element from the front and increments the front pointer. The circular nature ensures that when the rear or front reaches the end of the queue, it wraps around to the beginning.
What is the difference between Depth-First Search (DFS) and Breadth-First Search (BFS)?
-DFS is a traversal algorithm that explores as far as possible along each branch before backtracking. It uses a stack data structure for managing the nodes. BFS, on the other hand, explores all neighbors of a node before moving on to the next level of nodes, using a queue data structure. DFS is more memory efficient in sparse graphs, while BFS guarantees the shortest path in unweighted graphs.
What is double hashing and quadratic probing in hashing?
-Double hashing and quadratic probing are techniques used in open addressing to resolve hash collisions. In double hashing, a second hash function is applied to calculate a step size, while in quadratic probing, the step size increases quadratically (i.e., the next probe is at a distance of 1, 4, 9, etc.). Both methods aim to avoid clustering issues that occur in linear probing.
What is the time complexity of binary search?
-The time complexity of binary search is O(log n), where n is the number of elements in the sorted array. This is because each comparison divides the search interval in half, leading to a logarithmic number of steps to find the target element.
How do you evaluate a postfix expression?
-To evaluate a postfix expression, use a stack to store operands. When encountering an operator, pop the required number of operands from the stack, perform the operation, and push the result back onto the stack. The final result will be the only element remaining in the stack.
Outlines
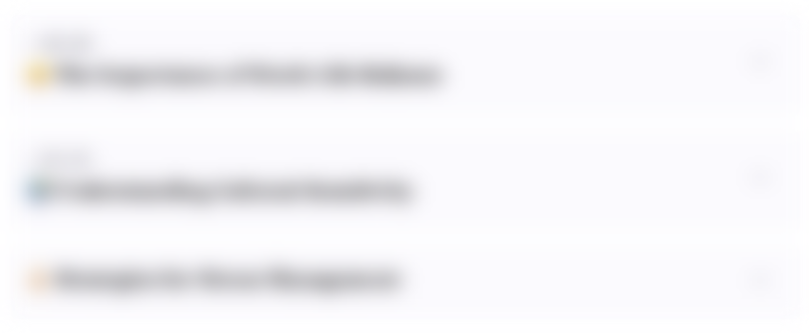
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
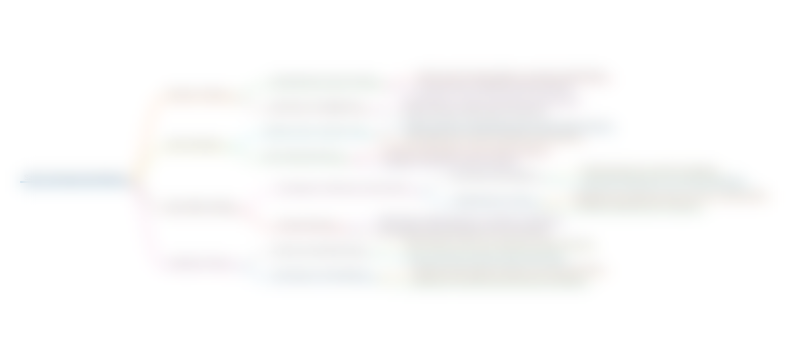
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
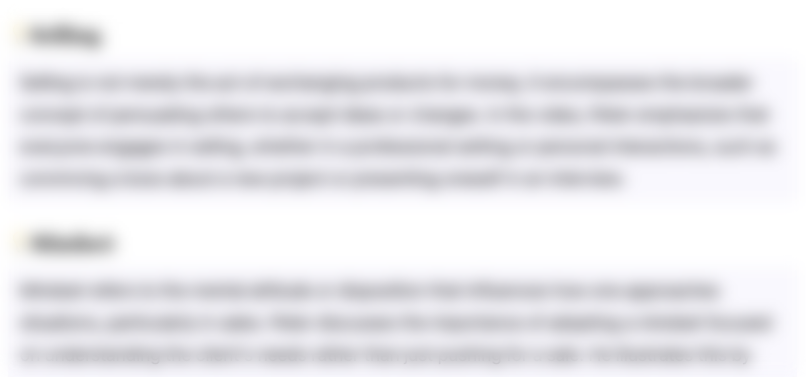
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
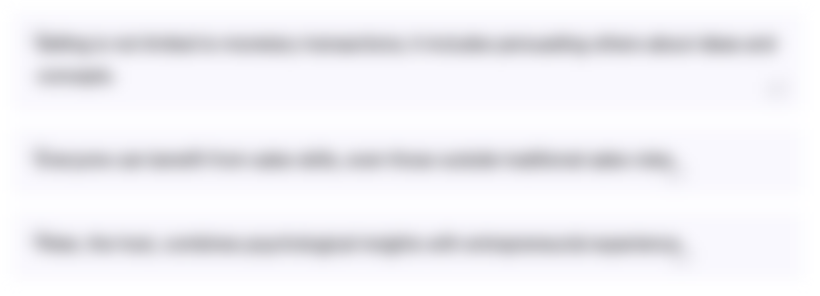
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
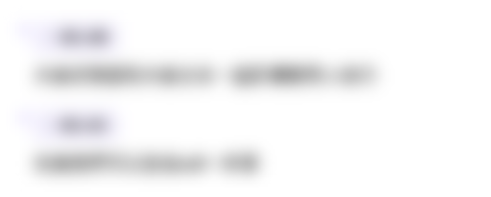
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
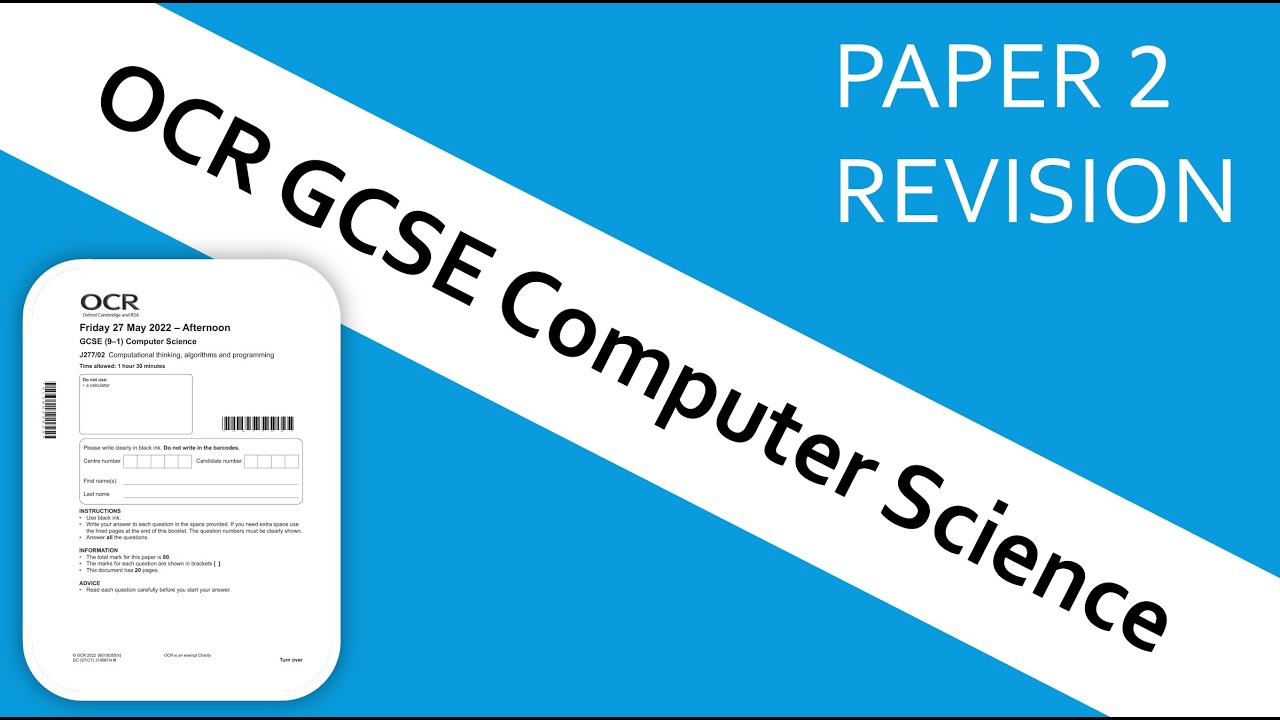
OCR GCSE Computer Science Paper 2 in 30 mins

Data Structures and Algorithms in 15 Minutes
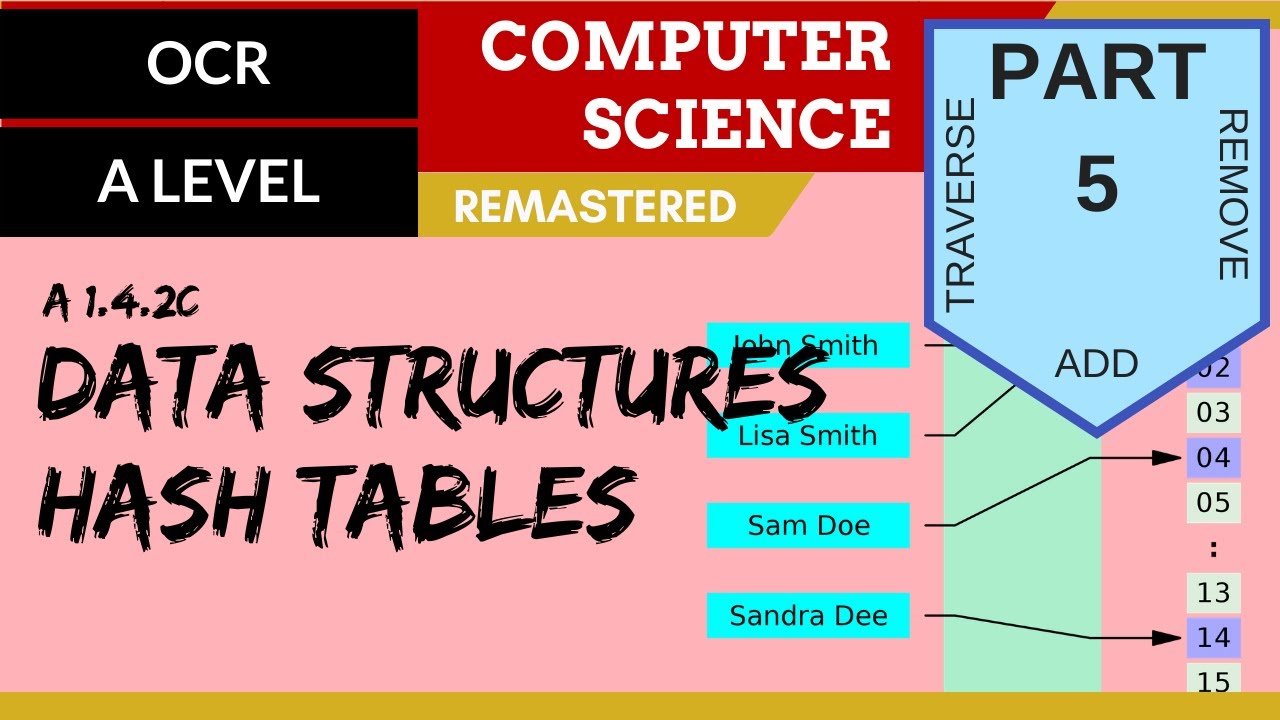
96. OCR A Level (H446) SLR14 - 1.4 Data structures part 5 - Hash tables (operations)

DAA Unit - 1 🎯Foundations of Algorithm 🔍 40 Top most V.V.i questions 🔄 || CSE 408
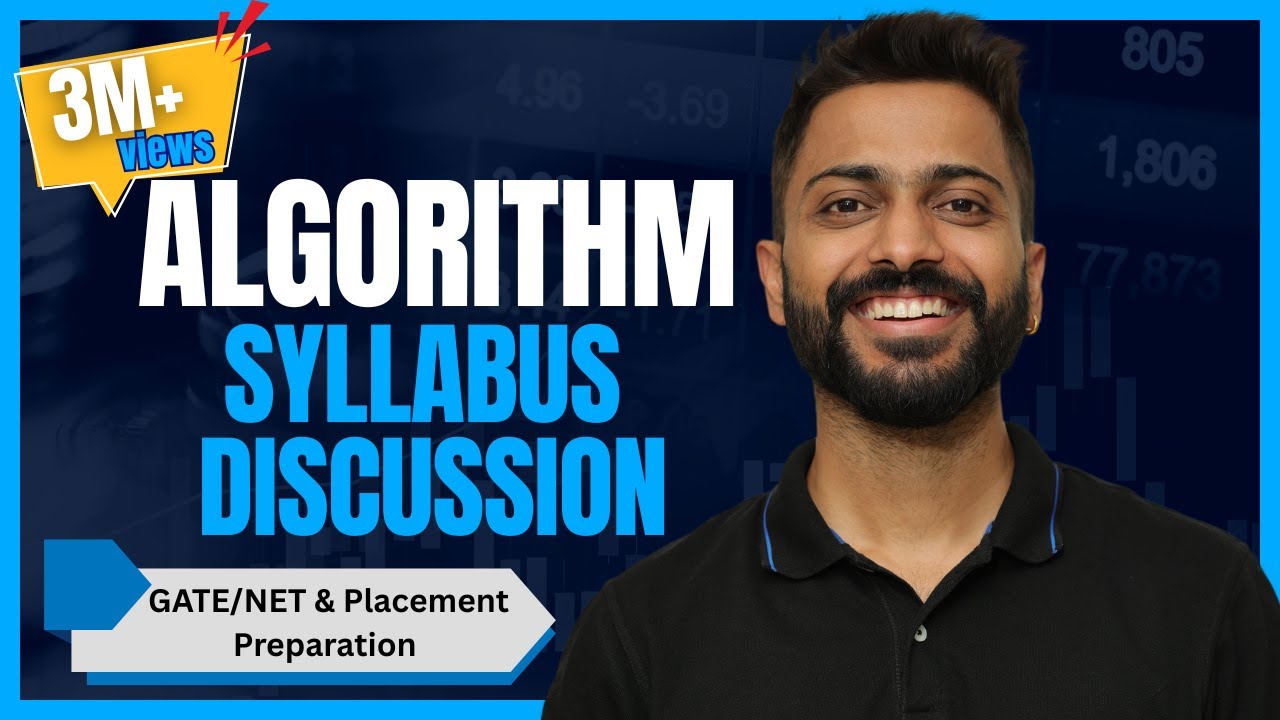
L-1.1: Introduction to Algorithm & Syllabus Discussion for GATE/NET & Placements Preparation | DAA
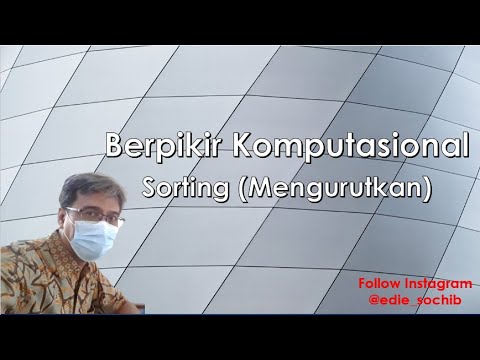
Berpikir Komputasional - Mengurutkan (Sorting), Tumpukan (Stack), dan Antrian (Queue)
5.0 / 5 (0 votes)