Variable Basics (Updated) | Godot GDScript Tutorial | Ep 1.1
Summary
TLDRThis video script offers an insightful look into the concept of variables in computer programming, particularly within the context of Gdscript. It explains that a variable is essentially a container for values and demonstrates how to create and assign values to them, emphasizing the importance of unique naming to avoid errors. The script delves into type variables, showcasing the use of data types to restrict variable content and the casting operator for value conversion. It also touches on common data types and the use of variables when data changes over time, providing practical examples to illustrate the concepts.
Takeaways
- π A variable in programming is a storage address with a symbolic name that holds a value, essentially a container for data.
- π In GDScript, variables are declared with the keyword 'var' followed by a unique name to ensure no name conflicts in the script.
- π‘ Parentheses in variable declarations are optional in GDScript, allowing for the creation of empty variables or variables with assigned values.
- π The assignment operator (=) is used to assign a literal value or data object to a variable in GDScript.
- π« Variables must have unique names; otherwise, an error is thrown in GDScript.
- π Typed variables are declared with a colon (:) followed by the desired data type, which restricts the variable to that type only.
- π Assigning a value of a different type than declared will result in an error in GDScript, enforcing type consistency.
- π The casting operator can be used to convert values to a specific type, such as converting an integer to a string or vice versa.
- π’ Common data types in GDScript include integers, booleans, strings, and floats, which can be explicitly declared or inferred from assigned values.
- π GDScript also supports casting to object types that are subclasses of another, such as assigning a Sprite to a variable declared as Node2D.
- π‘ Variables are essential when data needs to change over time, like the health of a player in a game, demonstrating the dynamic nature of variables.
Q & A
What is the dictionary definition of a variable in computer programming?
-A variable, also known as a scalar, is a storage address paired with an associated symbolic name that contains a known or unknown quantity of information, often referred to as a value. In simpler terms, it's a container that holds a value.
How is a variable created in Gdscript?
-In Gdscript, a variable is created by typing the keyword 'var' followed by a unique name. This name must be unique within the script to avoid conflicts.
What is the significance of using unique names for variables in Gdscript?
-Unique names are important to ensure that each variable is distinct and to prevent any conflicts or errors that may occur if two variables share the same name.
What is the purpose of the assignment operator in variable declaration?
-The assignment operator, represented by the equals sign, is used to assign a literal value or data object to a variable during its declaration.
What happens if a variable in Gdscript is not assigned a value?
-If a variable is not assigned a value, it will contain the data type 'null' by default, indicating that it has no value.
What is a typed variable and how is it declared in Gdscript?
-A typed variable is a variable that has an explicitly declared data type. It is declared by adding a colon followed by the desired data type after the variable name.
Why is declaring data types on variables considered good practice in Gdscript?
-Declaring data types on variables is good practice as it reduces confusion about the purpose of the variable and ensures that only compatible types are assigned to it, thus preventing type-related errors.
What is the effect of assigning a value of a different type to a typed variable in Gdscript?
-Assigning a value of a different type to a typed variable will result in an error because Gdscript expects the variable to hold values of the declared type only.
How can you convert a value to a specific data type in Gdscript?
-You can convert a value to a specific data type using the casting operator, which allows you to assign a value of one type to a variable of another type, effectively changing the value's type.
What are some common data types you might use with typed variables in Gdscript?
-Some common data types in Gdscript include integers, booleans, strings, and floats.
How can you infer the data type of a variable based on the assigned value?
-You can infer the data type of a variable by assigning a literal value to it without explicitly declaring the type. Gdscript will automatically recognize the type of the value and apply it to the variable.
Can Gdscript handle objects and class casting?
-Yes, Gdscript can handle objects and class casting, allowing you to assign values of subclasses to variables of superclass types, provided the assignment is compatible.
When should you use variables in a Gdscript program?
-Variables should be used when you have data that needs to change over the lifetime of the game or program, such as a player's health that may vary during gameplay.
Outlines
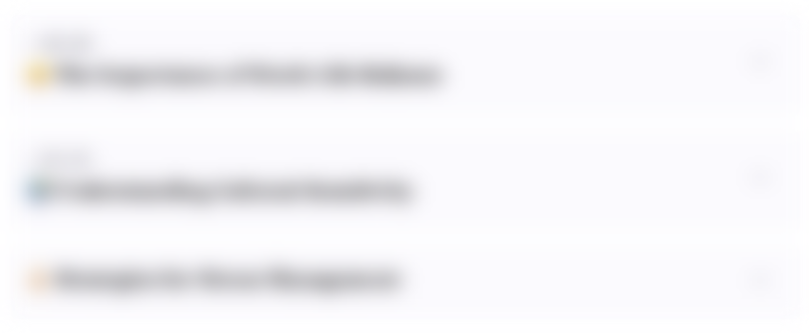
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
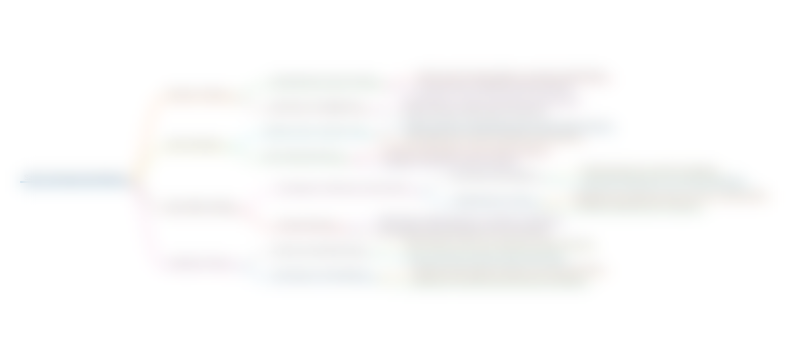
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
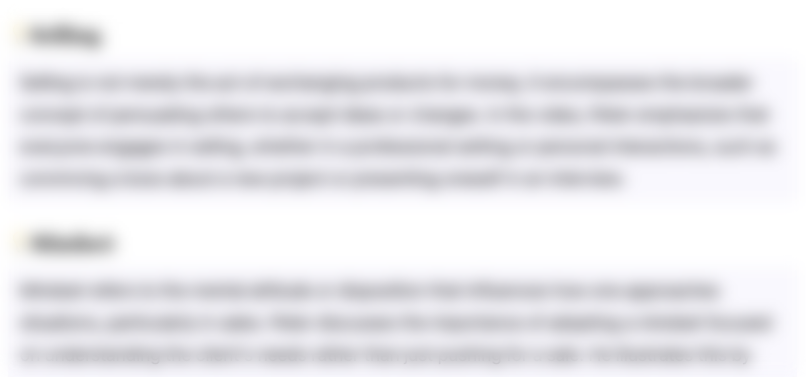
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
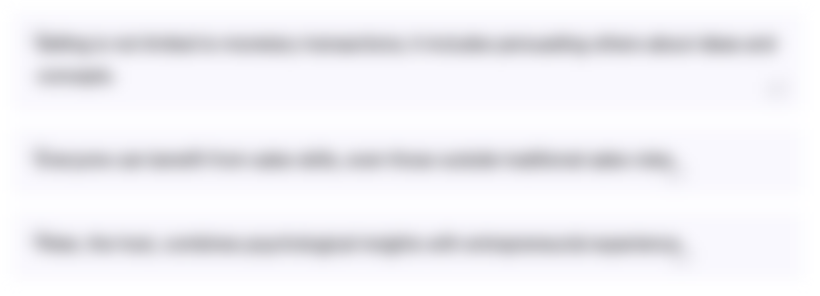
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
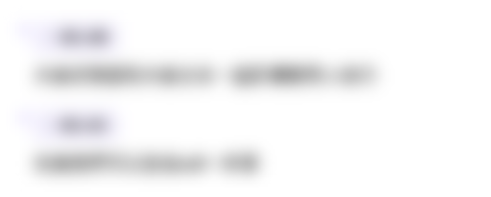
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)