Data Types & Literal Values | Godot GDScript Tutorial | Ep 00
Summary
TLDRThis script offers an insightful overview of data types in programming, focusing on GDScript. It explains the importance of data types for compilers to understand programmer intent, operations on data, and storage methods. The script delves into literal values, showcasing examples of strings, integers, floats, booleans, and 'no' values. It clarifies the distinction between string and integer values, demonstrates integer overflow and wraparound, and highlights the precision offered by floats. The boolean data type is presented as a logical truth value, convertible to numeric form. The 'no' type signifies the absence of data, rounding off the discussion on GDScript's fundamental data types.
Takeaways
- π A data type is an attribute of data that informs the compiler about the programmer's intended use, operations possible on the data, its meaning, and storage method.
- π’ A literal value is a value directly written in the source code, not derived from expressions like variables or constants, and represents something provided in the script.
- π In GDScript, common data types include strings, integers, floats, booleans, and the null value, each with specific representations in code.
- π¬ String data types represent text and can contain characters, spaces, and numbers, but string numbers are not the same as integer values for mathematical operations.
- π‘ The difference between string and integer values is that strings are enclosed in double quotes and cannot be used for mathematical operations without conversion.
- β οΈ Adding string numbers results in concatenation rather than arithmetic addition, as demonstrated by '1' + '1' resulting in '11' instead of 2.
- π’ Integers represent whole numbers within a specific range, and exceeding this range results in overflow and wraparound behavior.
- π Overflow in integers means that adding one to the maximum value results in the minimum value, and subtracting one from the minimum value gives the maximum.
- π Floats are used for precision and represent numbers with decimal points, converting them to integers removes the decimal and precision.
- π Booleans have two possible values, true and false, which can be represented as 0 and 1 in numeric form and 0.0 and 1.0 in float form.
- β The null data type represents the absence of data and contains no information, denoted simply as 'null' in GDScript.
Q & A
What is the dictionary definition of a data type?
-A data type is an attribute of data that informs the compiler how the programmer intends to use the data, determining the operations that can be performed on the data, the meaning of the data, and how values of that type can be stored.
What is a literal value in programming?
-A literal value, also known as a literal, is a value that is directly written in the source code, rather than being the result of another expression, such as referencing a variable or a constant.
What are the common data types used in GDScript?
-In GDScript, common data types include strings, numbers (integers and floats), booleans, and the null value.
How are string values represented in GDScript?
-String values in GDScript are represented within double quotations, indicating to the compiler that the enclosed text is a string.
Can string literals contain numbers?
-Yes, string literals can contain numbers, but they are treated as text and cannot be used for mathematical operations without being converted to a numerical data type.
What is the difference between integer and float values in GDScript?
-Integer values in GDScript are whole numbers without a decimal point, while float values are numbers with a decimal point, allowing for more precision.
What happens when you exceed the range of integer values in GDScript?
-If you exceed the maximum or minimum value in the integer range of GDScript, an overflow occurs, causing the value to wrap around to the opposite end of the range.
What are the two possible values for a boolean data type?
-A boolean data type has two possible values: true and false, representing the truth values of logic and boolean algebra.
How are boolean values represented numerically and in GDScript?
-In GDScript, when converted to numeric values, false becomes 0 and true becomes 1. As float values, false is 0.0 and true is 1.0.
What is the null data type used for in GDScript?
-The null data type in GDScript is used to represent the absence of data, containing no information and not being assignable to any other value.
Why are double quotations important for string values?
-Double quotations are important for string values because they indicate to the compiler that the enclosed text should be treated as a string, rather than as separate characters or a variable name.
Outlines
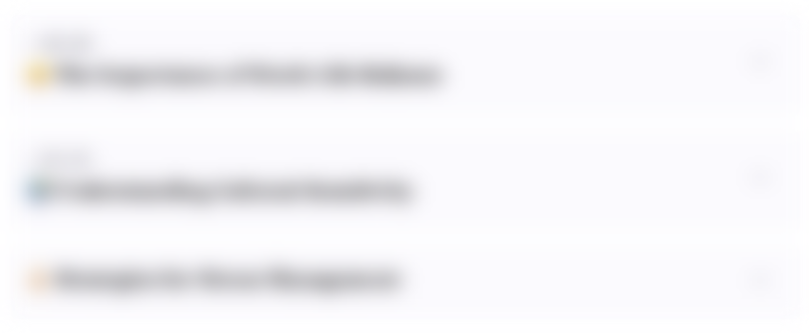
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
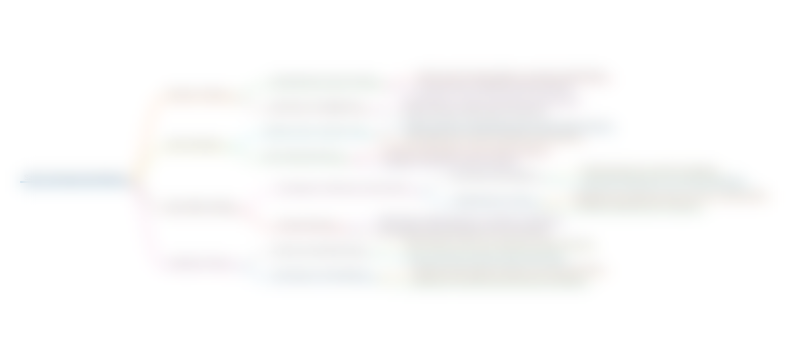
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
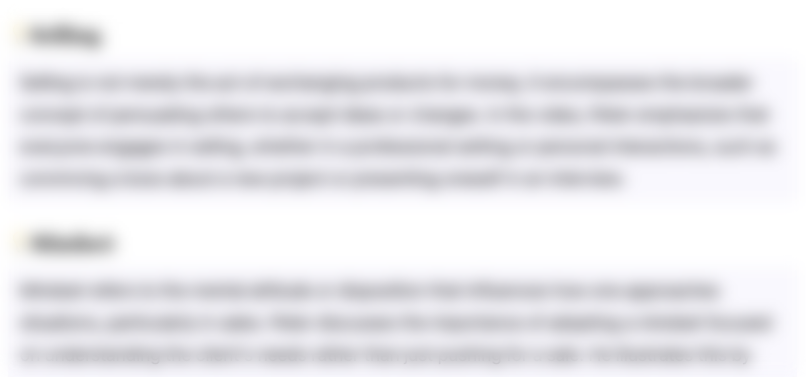
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
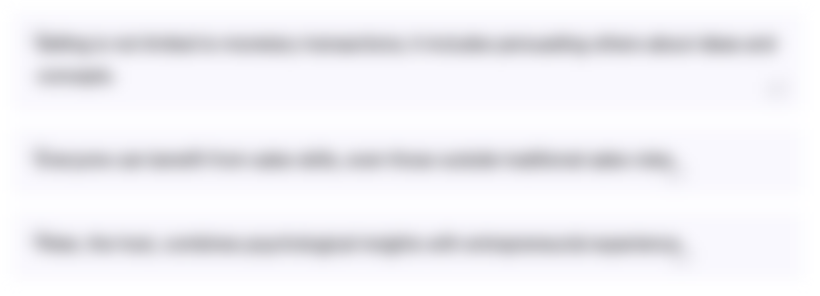
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
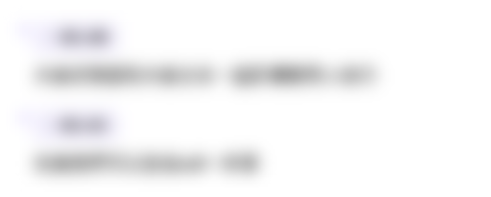
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)