Constants | Godot GDScript Tutorial | Ep 03
Summary
TLDRThe video script explains the concept of constants in computer science, specifically within the context of GDScript used in game development. It details how to declare constants with the 'Const' keyword, emphasizing their immutability post-declaration. The script advocates for the use of constants over literals for better code readability, easier debugging, and efficient code maintenance. It illustrates the benefits with examples, such as changing game item speeds by modifying a single constant instead of numerous hardcoded values, and discusses the importance of naming conventions to visually distinguish constants from variables.
Takeaways
- ๐ Constants in computer science are values that do not change.
- ๐ In GDScript, constants are declared with the keyword 'Const' followed by a unique name, an equal sign, and a value.
- ๐ฒ๏ธ Assigning an empty constant or a constant without a value is not allowed.
- ๐ The difference between a variable and a constant is the keyword used; everything else is the same.
- ๐ Data types can be explicitly added to constants with a colon and data type name, but it's optional as the type is inferred.
- ๐ซ Constants are read-only and cannot be modified after declaration and assignment.
- ๐ Using constants is beneficial for debugging, editing, and reading code.
- ๐ก Beginners often use literals instead of constants, but constants improve code readability and maintainability.
- ๐ Constants should be visually distinct from variables, often using uppercase letters for easy identification.
- ๐ Changing a constant's value automatically propagates the change throughout the code where the constant is used.
- ๐ฎ Constants are ideal for values that will not change during the lifecycle of a game, such as player dimensions.
Q & A
What is a constant in computer science?
-In computer science, a constant is a value that does not change throughout the execution of a program.
How do you declare a constant in GDScript?
-In GDScript, constants are declared using the keyword 'const' followed by a unique name, an equal sign, and a value.
Is it possible to assign an empty value to a constant in GDScript?
-No, you cannot assign an empty constant; it must have an assigned value upon declaration.
What is the difference between a variable and a constant in GDScript?
-The only difference between a variable and a constant in GDScript is the keyword used for declaration; 'var' for variables and 'const' for constants.
Can you modify the value of a constant after it has been declared in GDScript?
-No, constants in GDScript are read-only after declaration, and modifications are not permitted.
Is it necessary to specify a data type when declaring a constant in GDScript?
-Specifying a data type when declaring a constant is not necessary as the data type is inferred from the assigned value, but you can explicitly add a data type for clarity.
Why should beginners avoid using literal values and instead use constants?
-Using constants instead of literals makes code more readable, easier to debug, and simplifies the process of editing code, especially when the same value is used in multiple places.
What is the advantage of using constants over literals in a large codebase?
-Using constants in a large codebase allows for easier maintenance and updating of values. Changing the value of a constant will propagate the change throughout the code where the constant is used, instead of having to find and replace every instance of the literal value.
What is the recommended naming convention for constants in GDScript to differentiate them from variables?
-A recommended naming convention for constants is to start their names with an uppercase letter, while variables start with a lowercase letter, making it visually clear which is which when reading the code.
Can you provide an example of how using constants can simplify changing a value in a large codebase?
-If a game's speed value is initially set as a literal 5 in multiple variables, changing it to 10 later would require finding and editing each instance of the literal 5. However, if the speed is declared as a constant, changing it to 10 would automatically update all instances where the constant is used, reducing the potential for errors and saving time.
Outlines
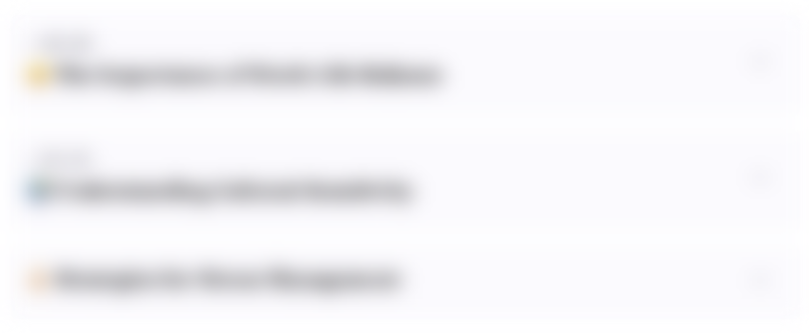
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
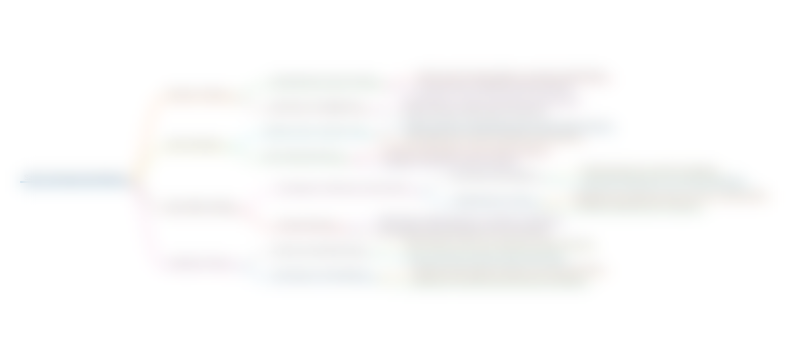
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
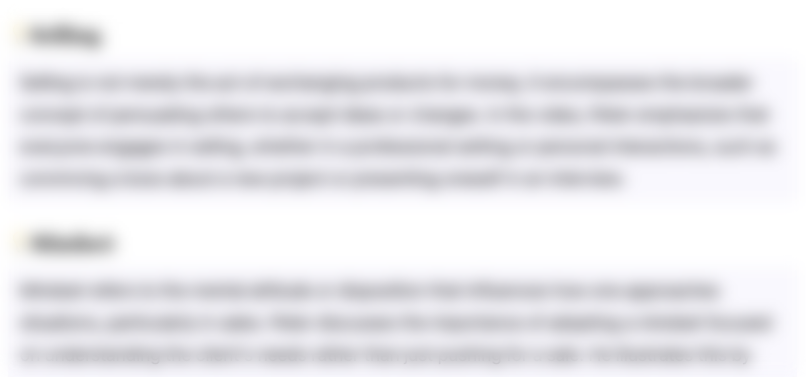
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
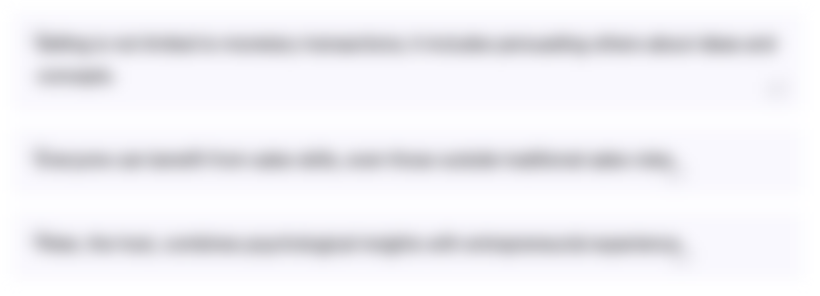
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
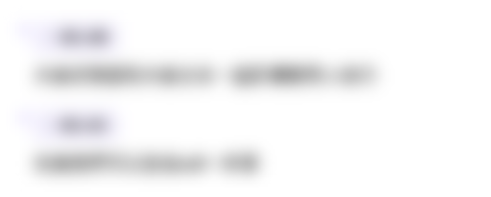
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
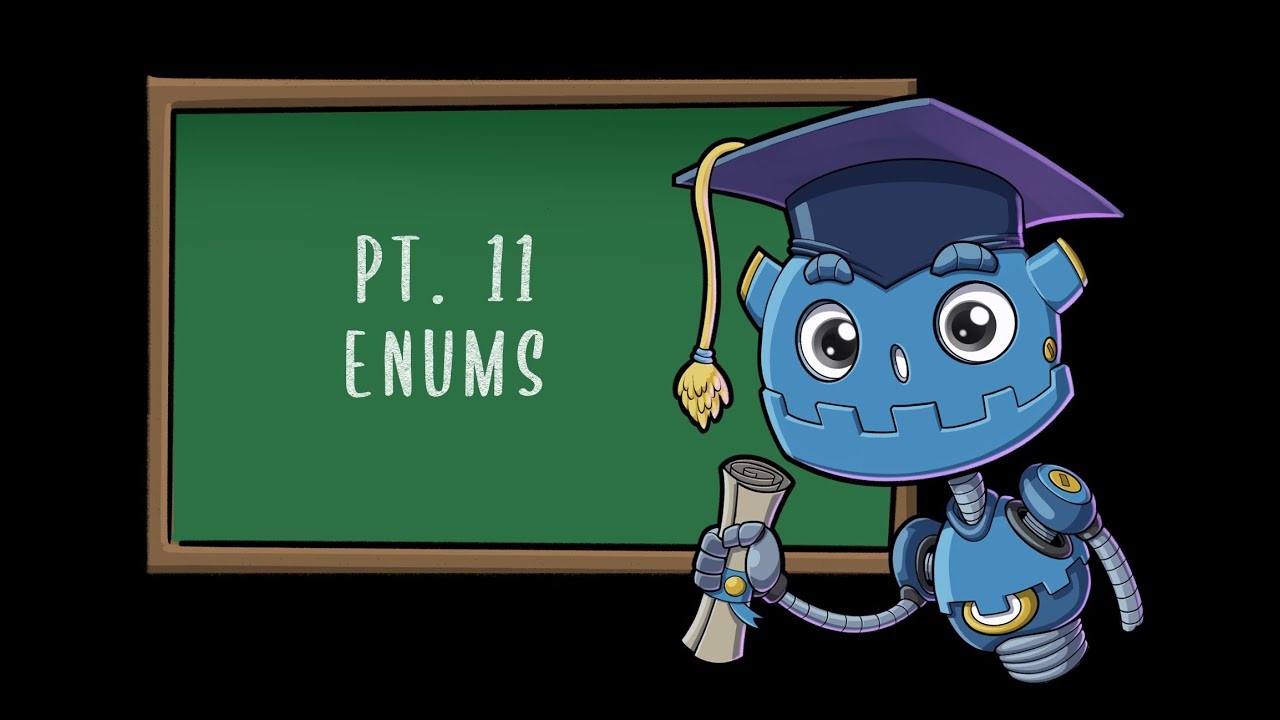
Enums | Godot GDScript Tutorial | Ep 11

Variable Basics (Updated) | Godot GDScript Tutorial | Ep 1.1
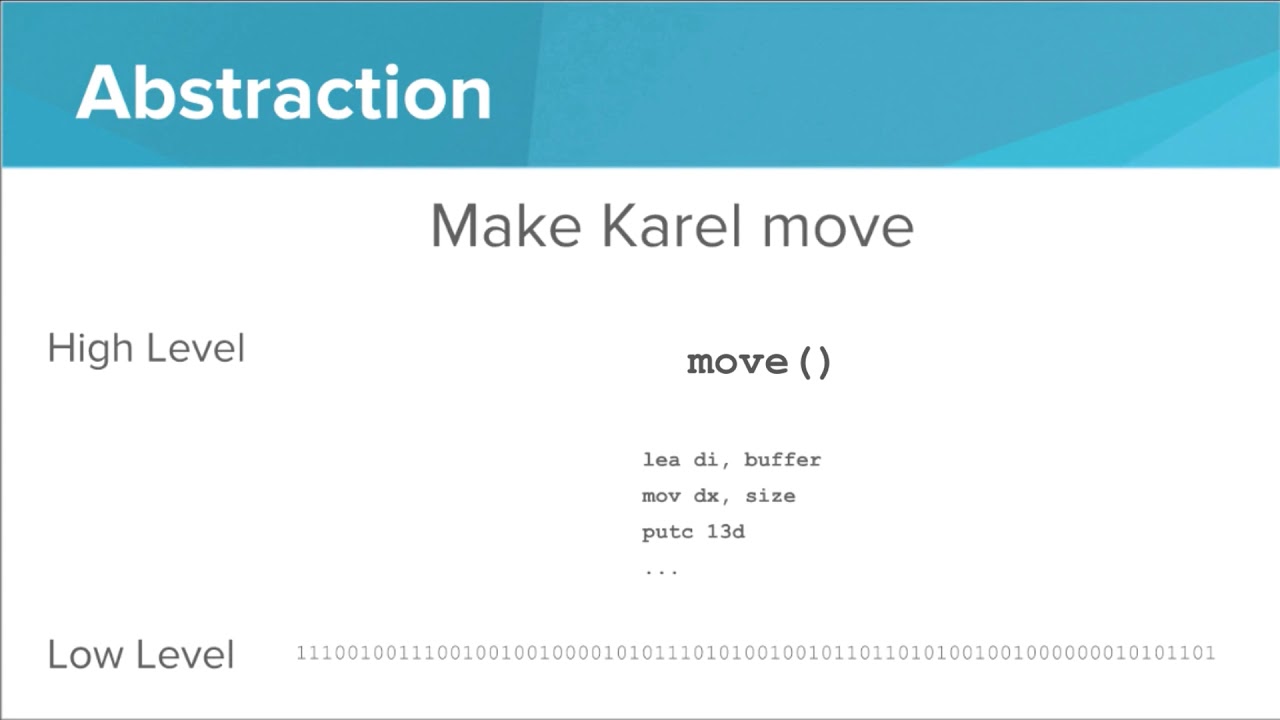
Karel Python - Abstraction

L-2.3: Immediate Addressing Mode | Computer Organisation and Architecture

BAB 4 SISTEM KOMPUTER | MULTITASKING | INFORMATIKA SMA KELAS 10 | KEMENDIKBUD

PBA(NLP) #2 : Regular Expression (Regex) dalam Pemrosesan Bahasa Alami
5.0 / 5 (0 votes)