Class Objects & Constructors | Godot GDScript Tutorial | Ep 16
Summary
TLDRThis tutorial delves into the concept of objects and constructors in GDScript, explaining how objects are instances of classes and the process of instantiation. It clarifies the role of instance variables, unique to each object, and demonstrates how to create and use class objects with examples. The video also covers the use of constructors to initialize objects with unique properties, showcasing the flexibility and power of object-oriented programming in GDScript.
Takeaways
- 📚 An object in GDScript is an instance of a class, also known as a class instance, instance object, or class object.
- 🔨 Instantiation, or construction, is the process of creating an instance of a predefined object with a unique name for use in a program.
- 🔑 Instance variables are member variables that each object has a separate copy of, ensuring changes in one do not affect others.
- 🧱 The compiler in GDScript will attempt to typecast values to match the declared data type of an instance variable if a different type is passed.
- 🔄 When creating multiple objects from a class, each object has its own isolated copy of the member variables.
- 🛠️ Instance objects are useful when you need to work with multiple items or copies of a class, like having multiple enemies on the screen in a game.
- 📝 To create an object in GDScript, you can use the registered class name with the 'new' keyword or load the class via file paths and then use 'new'.
- 🎯 The 'initialize' method, or '__init__' for short, is used in GDScript to set up instance objects with specific properties during instantiation.
- 🛑 If parameters are declared in the '__init__' method, they must be specified when creating an instance object to avoid errors; default values can be set to prevent this.
- 📌 Using the constructor method allows for variation in object properties, making each instance unique, by passing values during the instantiation process.
- 📑 The script provided in the tutorial demonstrates creating class objects with unique names and properties, showcasing the power of instance objects in GDScript.
Q & A
What is an object in the context of this script?
-An object is an instance of a class, also referred to as a class instance, instance object, or class object. It is the realization of a predefined object through a process known as instantiation or construction.
What is the term used for the process of creating an instance of a class?
-The term used for creating an instance of a class is 'instantiation', also known as 'construction'. It involves declaring an instance of an object with a unique name for use in a program.
What is an instance variable and how does it differ from other variables?
-An instance variable is a member variable that has a separate copy for each instantiated object of the class. Changes to the data in one instance variable will not affect the same variable in another instance object, ensuring data isolation.
How does the compiler handle a mismatch between the declared data type of an instance variable and the actual value passed at runtime?
-If an instance variable is declared with a specific data type and a different data type is passed at runtime, the compiler will attempt to typecast the passed value to the specified data type, for example, wrapping an integer in double quotes to convert it into a string.
What is the purpose of using non-static variables in the context of instance variables?
-Non-static variables are important when discussing instance variables because they ensure that each instance of a class has its own copy of the variable, supporting code isolation and preventing unintended data sharing between instances.
When might you choose to use instance objects in your program?
-Instance objects are useful when you need to work with multiple items or copies of a class, such as having multiple enemies on the screen in a game, allowing for unique properties and behaviors for each object.
How do you create an instance object using a registered class name?
-To create an instance object using a registered class name, you use the registered name followed by the 'new' keyword or 'new' method, which instantiates the class.
What is the difference between using a registered class name and file paths to create an instance object?
-Using a registered class name to create an instance object requires only one line of code with the 'new' method. In contrast, using file paths involves loading the class into the current class with a 'load' method and then calling the 'new' method, which takes two lines of code.
What is the purpose of the 'initialize' method or '__init__' in a class?
-The 'initialize' method, or '__init__', is a constructor method in a class that is called when the class is instantiated. It allows for setting initial values for member variables and is particularly useful for creating unique instances with different properties.
How can you avoid errors when using the 'initialize' method with parameters but not passing any values during instantiation?
-To avoid errors when using the 'initialize' method with parameters, you can assign default values to the parameters or declare the parameters with inferred data types using the colon and equal sign syntax, ensuring that instantiation can occur without passing values.
What happens if you assign a value of a different datatype to a member variable that is declared to only accept string values?
-If you assign a value of a different datatype to a member variable declared to only accept strings, the system will attempt to cast the value as a string, effectively converting the integer to a string representation when used in string-based operations.
Outlines
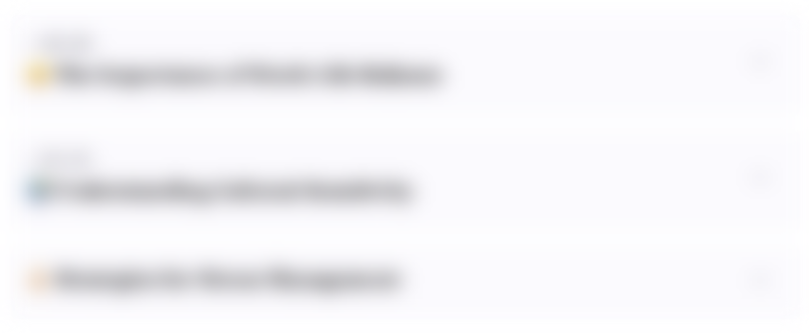
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
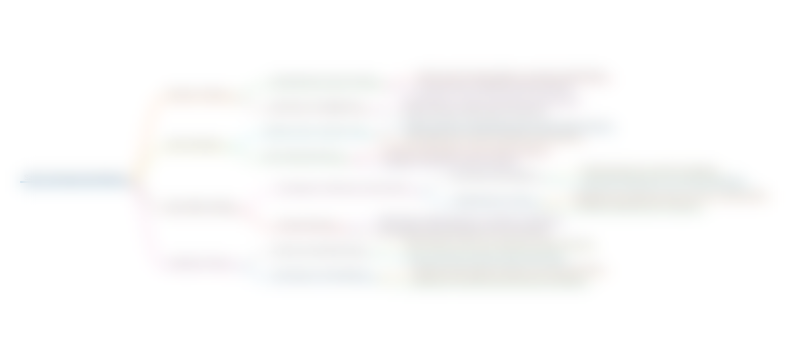
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
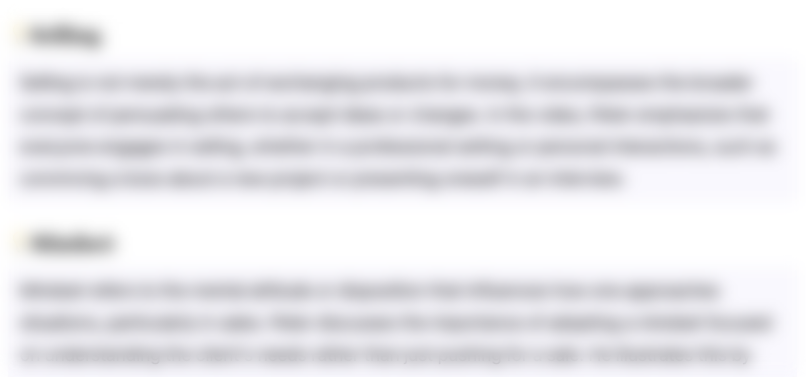
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
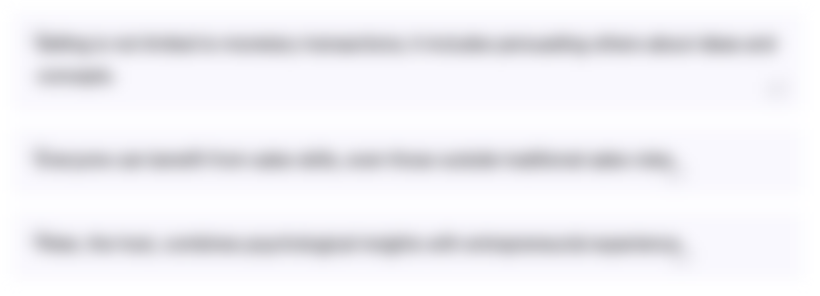
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
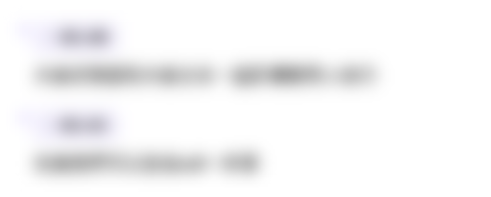
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
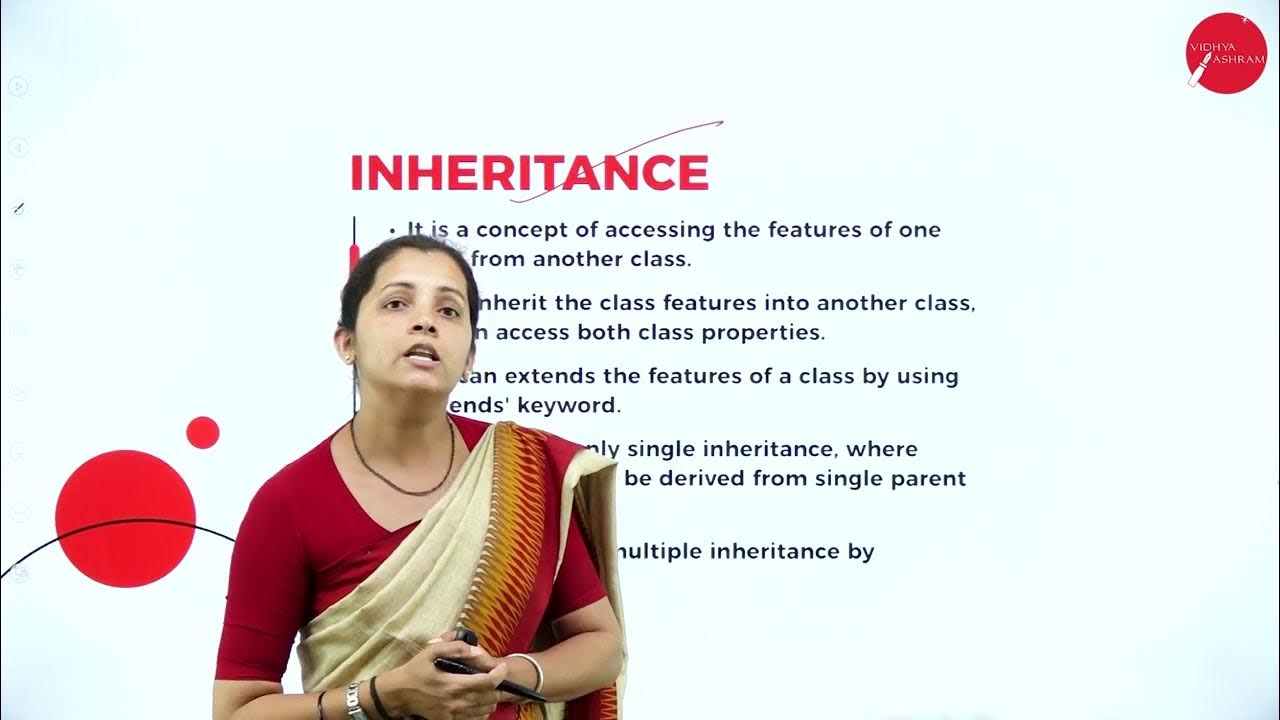
DAY 07 | PHP AND MYSQL | VI SEM | B.CA | CLASS AND OBJECTS IN PHP | L1
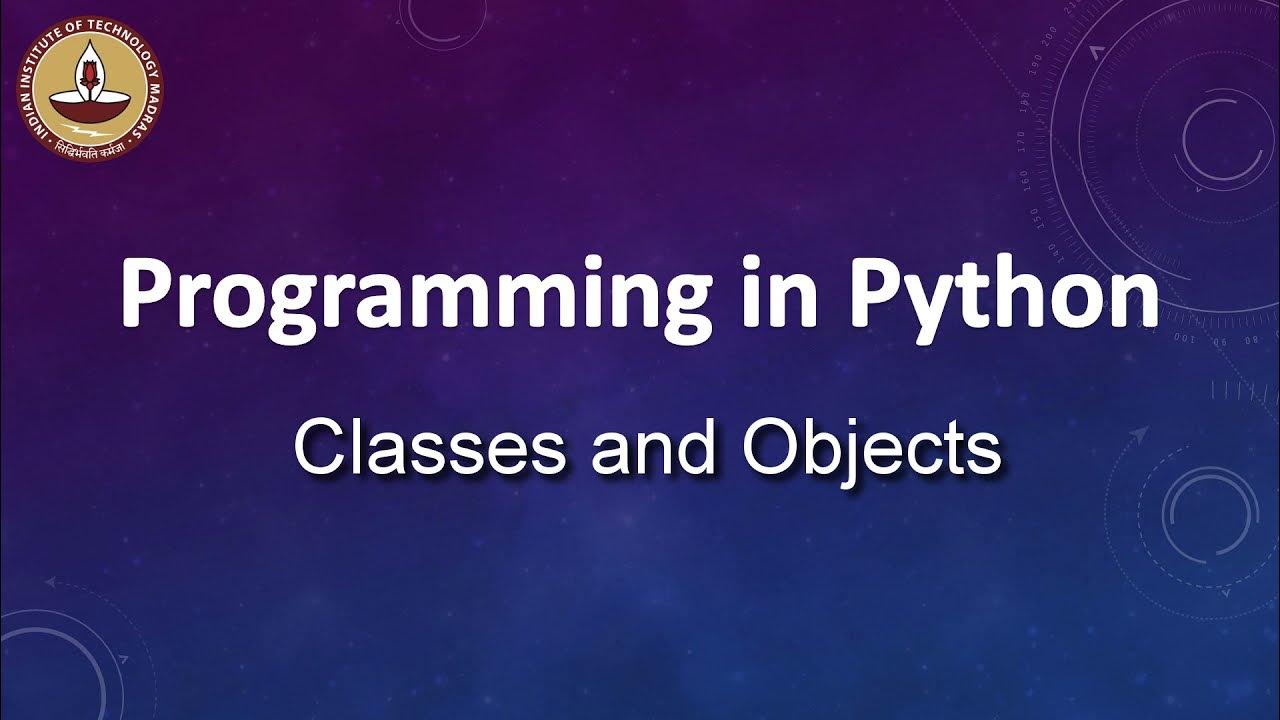
Classes and Objects

JAVA TECHNICAL QUESTION AND ANSWERS FOR INTERVIEW PART III (Classes & Objects)
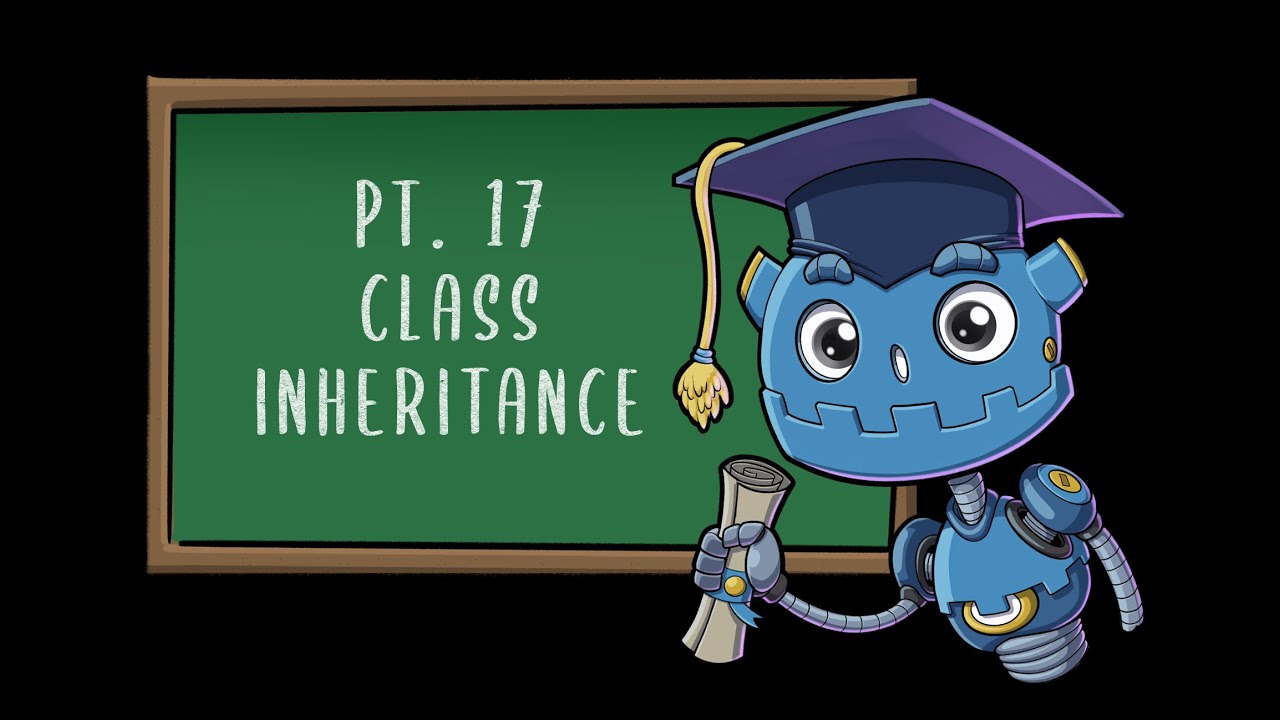
Class Inheritance | Godot GDScript Tutorial | Ep 17
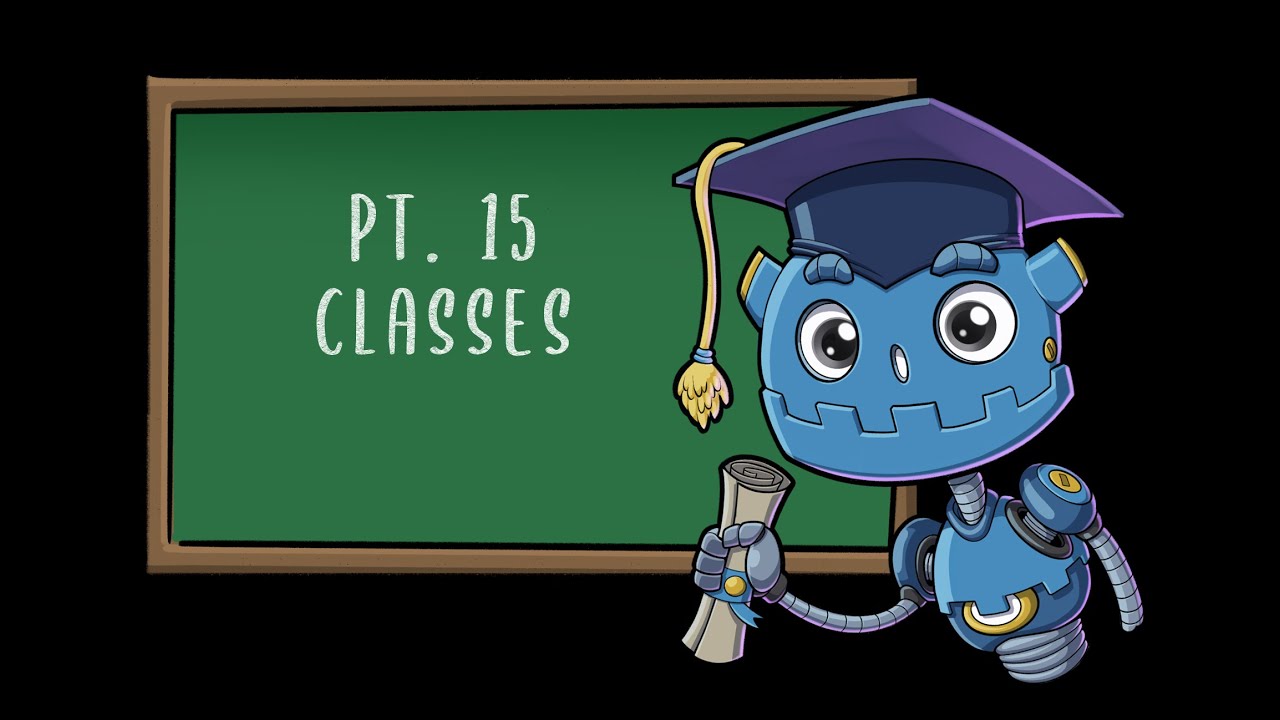
Classes | Godot GDScript Tutorial | Ep 15
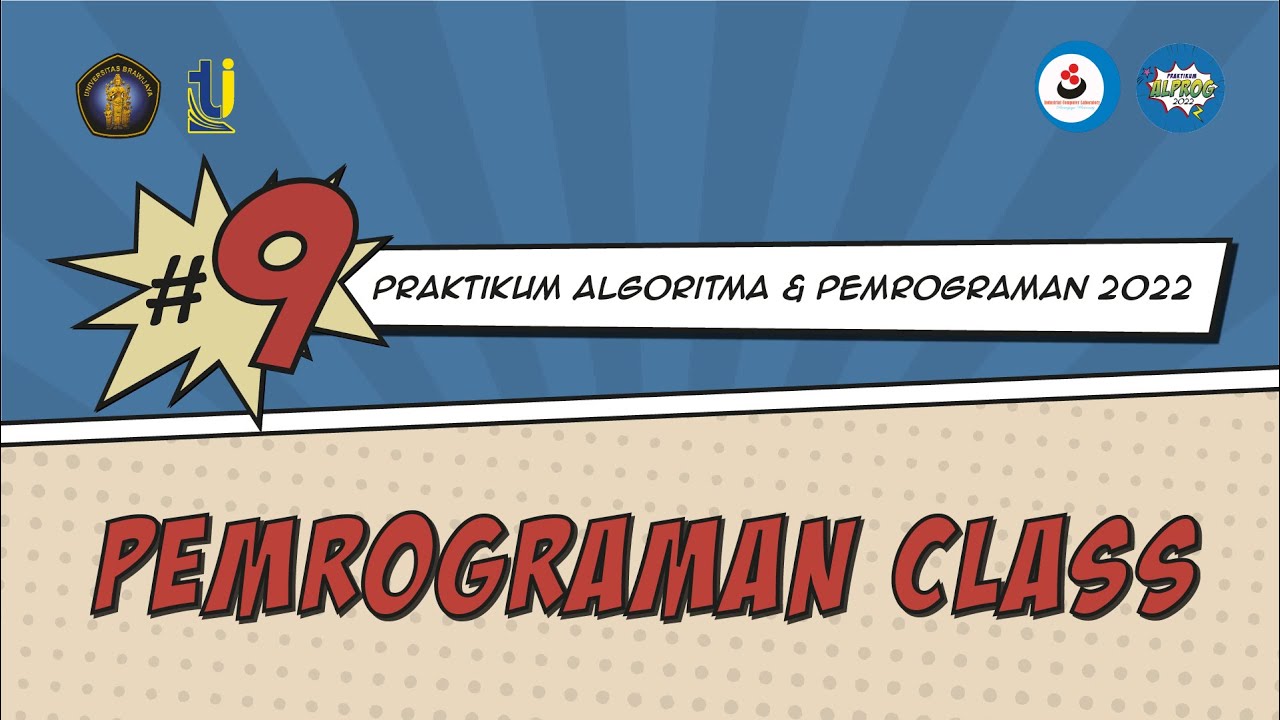
9. Pemrograman Class - Praktikum Algoritma dan Pemrograman 2022
5.0 / 5 (0 votes)