Life Cycle & Reference Counting | Godot GDScript Tutorial | Ep 1.2
Summary
TLDRThis script delves into the concept of variables and memory lifecycle in programming. It explains how variables are storage addresses with symbolic names, and how memory is allocated, used, and released. The script contrasts memory management in C, where variables point to different addresses but can hold the same value, with Godot's reference counting system, which manages memory by tracking object references. It also offers a tip on optimizing memory usage in games and managing nodes in Godot, emphasizing the importance of efficient memory management for performance.
Takeaways
- 📚 A variable, also known as a scalar, is a storage address paired with a symbolic name that holds a quantity of information.
- 🔄 The memory lifecycle involves allocation, usage, and release of memory, which is the same across all programming languages.
- 💾 Memory allocation is managed by the operating system and involves using RAM, which is an array of bytes.
- 🔍 After allocation, the application can perform read and write operations on the memory, such as assigning and changing variable values.
- 🗑️ Memory is released when variables are no longer in use, either manually or automatically depending on the programming language.
- 🛠️ The programming language, not the operating system, determines how memory is handled within an application.
- 🚀 Using less memory is generally better for performance, especially in games and for users with lower-spec hardware.
- 🔃 If an application requires more memory than initially allocated, the OS may allocate additional memory from the hard drive or SSD, which is slower than RAM.
- 🔑 In the C language example, variables X and Y can have the same value but different memory addresses, allowing independent value changes.
- 🔄 Godot engine uses reference counting for memory management instead of garbage collection, which means objects remain in memory as long as their reference count is greater than zero.
- 🌐 When a new variable is created and assigned the value of an existing variable in Godot, it points to the same object, increasing the reference count.
- 🔧 Changing the value of a variable in Godot involves creating a new object and pointing the variable to this new object, which can lead to the previous object being freed if its reference count drops to zero.
- 📌 Godot nodes are not reference counted, so removing a node from the tree does not free its memory; nodes should be managed carefully to avoid memory leaks.
Q & A
What is the definition of a variable in the context of programming?
-A variable, also known as a scalar, is a storage address paired with an associated symbolic name that contains a known or unknown quantity of information.
What is a memory lifecycle in programming?
-A memory lifecycle refers to the period between an object's creation and its destruction, which includes allocating memory, using memory, and releasing memory.
How does a computer's operating system allocate memory to an application?
-The operating system allocates memory by assigning a portion of the computer's RAM to the application, allowing it to use the memory for read and write operations.
What happens when an application is done using allocated memory?
-When an application is finished using the allocated memory, the operating system releases it, making it available for other applications or for new memory allocation by the same application.
How does the programming language influence memory management?
-The programming language determines how memory is handled, including how it is allocated, used, and released, and whether it is done manually or automatically.
Why is it beneficial to use less memory in applications, especially in games?
-Using less memory is beneficial because it improves performance for users with lower RAM and CPU capacities, ensuring smoother operation and better user experience.
What happens if an application uses more memory than initially allocated by the operating system?
-If an application exceeds the initially allocated memory, the operating system may allocate additional memory from the hard drive or solid-state drive, which is slower than using RAM.
Can you explain the basic process of variable assignment in the C language?
-In the C language, the process involves allocating memory for the variable, assigning a value to that memory address, pointing the variable to that value, and freeing the memory when the variable is no longer in use.
How does Godot's reference counting differ from garbage collection in memory management?
-Godot uses reference counting to determine if an object should be removed from memory based on the number of references to it. This is different from garbage collection, which automatically identifies and removes unused objects.
What is the effect of creating a new variable Y that equals an existing variable X in Godot?
-When creating a new variable Y that equals X in Godot, the new variable Y points to the same object as X, increasing the reference count of the object, but does not create a new object or duplicate the value.
How does changing the value of a variable in Godot affect memory management?
-Changing the value of a variable in Godot may create a new object with a new memory address for the new value, and the variable is then pointed to this new object, potentially leading to the previous object being released from memory if its reference count drops to zero.
Why are nodes in Godot not reference counted like other objects?
-Nodes in Godot are not reference counted because removing a node from the tree does not delete it from memory. To manage nodes effectively, one should create a pool of objects and reuse node objects or explicitly destroy them using 'queue_free' and 'exit_tree'.
Outlines
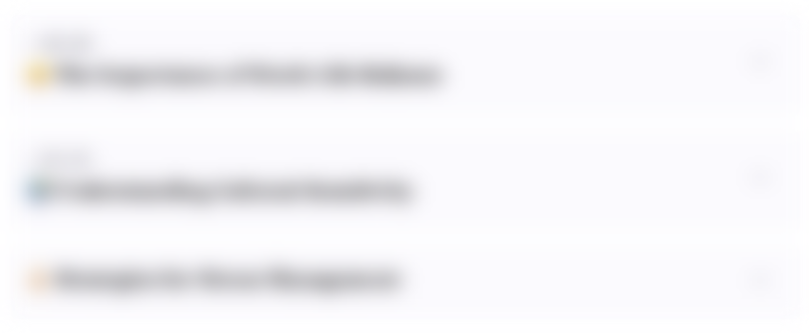
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
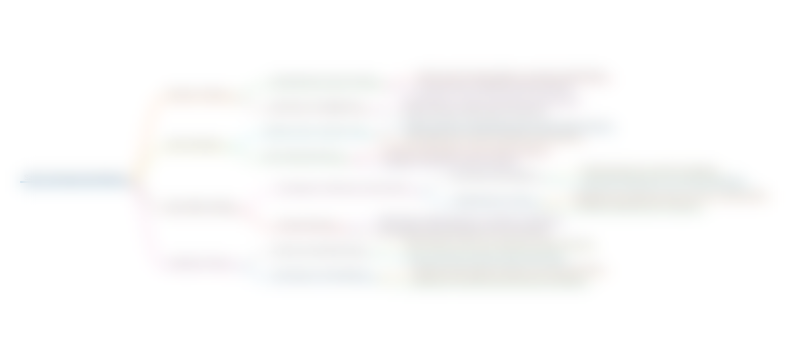
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
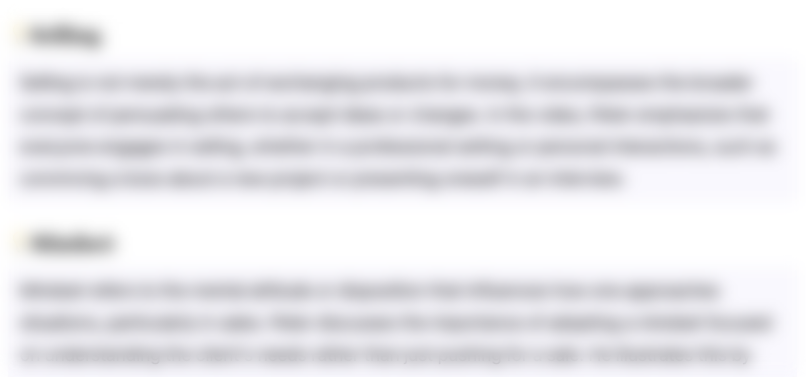
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
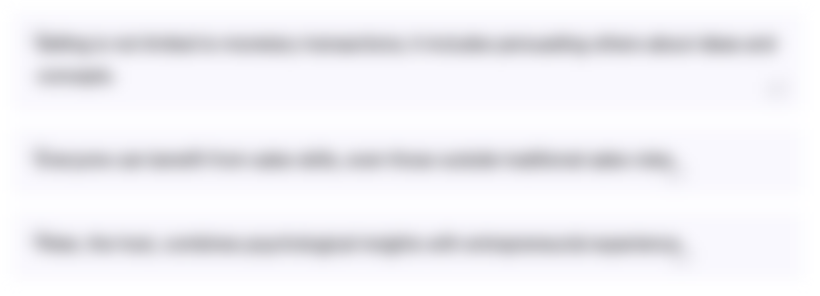
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
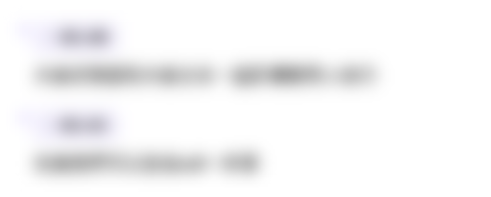
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)