you will never ask about pointers again after watching this video
Summary
TLDRThis video script demystifies pointers for new programmers, explaining their importance and syntax in C programming. It starts by illustrating memory with addresses and values, then introduces pointers as variables that store addresses. The script clarifies pointer creation and usage with examples, showing how they allow accessing and modifying memory locations indirectly. It addresses common confusion around pointer syntax and emphasizes pointers' necessity for efficient, scalable code and dynamic memory management. The video aims to help viewers overcome the challenge of understanding pointers, promising to turn them into 'low-level wizards.'
Takeaways
- ๐ต Pointers are one of the most challenging concepts for new programmers to grasp, especially when they involve arrays or multiple layers of pointers.
- ๐จโ๐ซ Understanding pointers begins with comprehending how memory works, including the concepts of memory address and value.
- ๐ A pointer is essentially a variable that stores the memory address of another variable, allowing indirect access to its value.
- ๐ก Pointers are created in C using the asterisk (*) for declaration and the ampersand (&) to get the address of a variable.
- ๐ The syntax involving pointers can be confusing due to the use of asterisks and ampersands, which have different meanings in different contexts.
- ๐ Pointers enable passing variables by reference, which can be more efficient and useful than passing by value, especially for large data structures.
- ๐ The use of pointers is often necessary for writing clean, understandable, and scalable code, particularly when dealing with functions and memory allocation.
- ๐งฉ Dynamic memory allocation, which involves pointers, is crucial for managing memory that is not fixed in size at compile time, such as data structures that grow during runtime.
- ๐ฅ Misuse of pointers can lead to program crashes, emphasizing the importance of careful handling and understanding of pointer operations.
- ๐ Mastery of pointers is a hallmark of a proficient programmer in C, allowing for more efficient and lower-level control over memory and data structures.
Q & A
What is the primary difficulty new programmers face when learning about pointers?
-The primary difficulty new programmers face with pointers is understanding the concept itself, including pointers that point to arrays or other pointers, as well as the syntax involved in creating and using pointers.
How does the video aim to help new programmers with pointers?
-The video aims to help new programmers by explaining what a pointer is, the syntax of pointers, and their practical use cases, making the concept more accessible and less intimidating.
What is the relationship between memory and pointers in the context of the video?
-In the context of the video, memory is described as having an address and a value. A pointer is a variable that stores the address of another variable, effectively 'pointing' to it.
What is the significance of the address and value in memory as explained in the video?
-The address represents the location in memory where data is stored, while the value is the actual data stored at that location. Understanding this relationship is crucial for grasping how pointers work.
Can you explain the notation used in C for pointers as described in the video?
-In C, a pointer is denoted by using an asterisk (*) before the variable name, which indicates that the variable is a pointer to a specific data type. For example, 'int *px' declares 'px' as a pointer to an integer.
What does the ampersand symbol represent in the context of pointers in C?
-The ampersand symbol (&) in C is used to get the address of a variable. It is used when creating a pointer to a variable, allowing the pointer to store the memory address of the variable.
How does the video explain the process of creating a pointer to an integer variable?
-The video explains that to create a pointer to an integer variable, you declare a pointer variable with an asterisk before the variable name and then assign the address of the integer variable to it using the ampersand symbol.
What is the purpose of using pointers in programming as discussed in the video?
-Pointers are used to access and manipulate memory directly, which allows for more efficient and flexible memory management, passing variables by reference, and handling dynamic memory allocation.
Why are pointers considered essential in C programming as per the video?
-Pointers are essential in C programming because they enable functions to modify variables outside their scope, manage dynamic memory allocation, and write clean, efficient, and scalable code.
What is the difference between static and dynamic memory allocation as mentioned in the video?
-Static allocation refers to variables that are allocated on the stack and have a fixed size at compile time. Dynamic allocation, on the other hand, involves memory from the heap that can change in size during runtime, often requiring the use of pointers.
How does the video encourage viewers to feel about mastering pointers?
-The video encourages viewers by suggesting that once they master pointers, they will feel like a 'low-level wizard,' implying a sense of accomplishment and mastery over a challenging aspect of programming.
Outlines
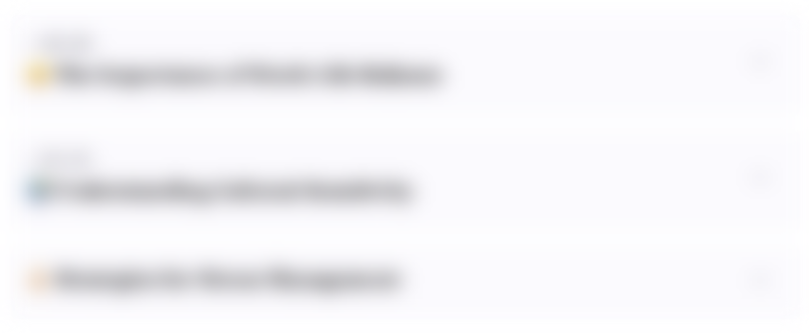
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
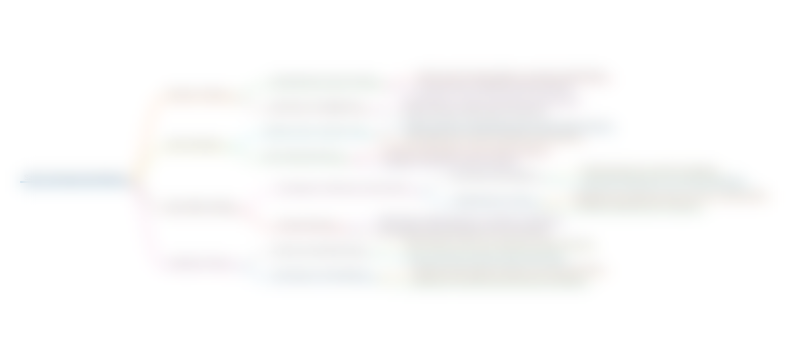
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
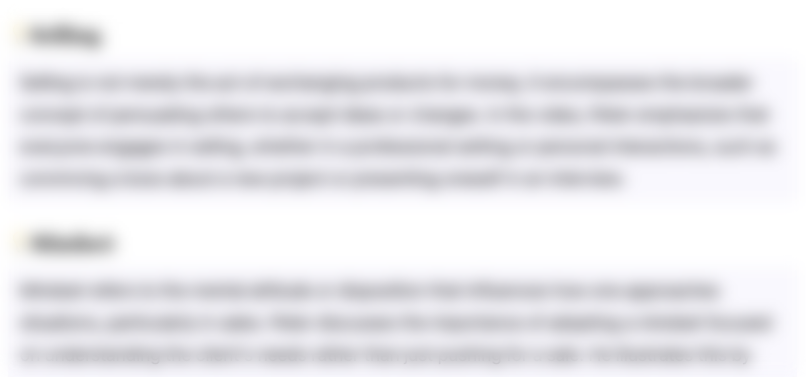
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
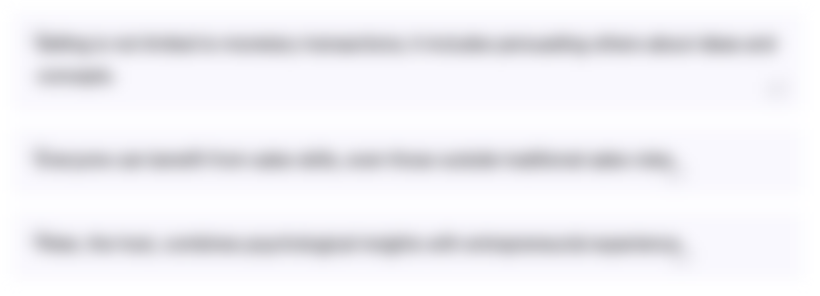
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
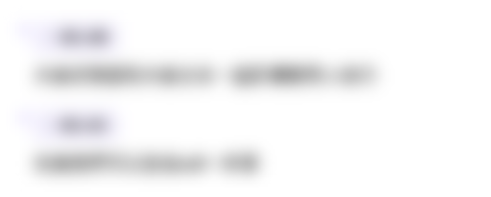
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
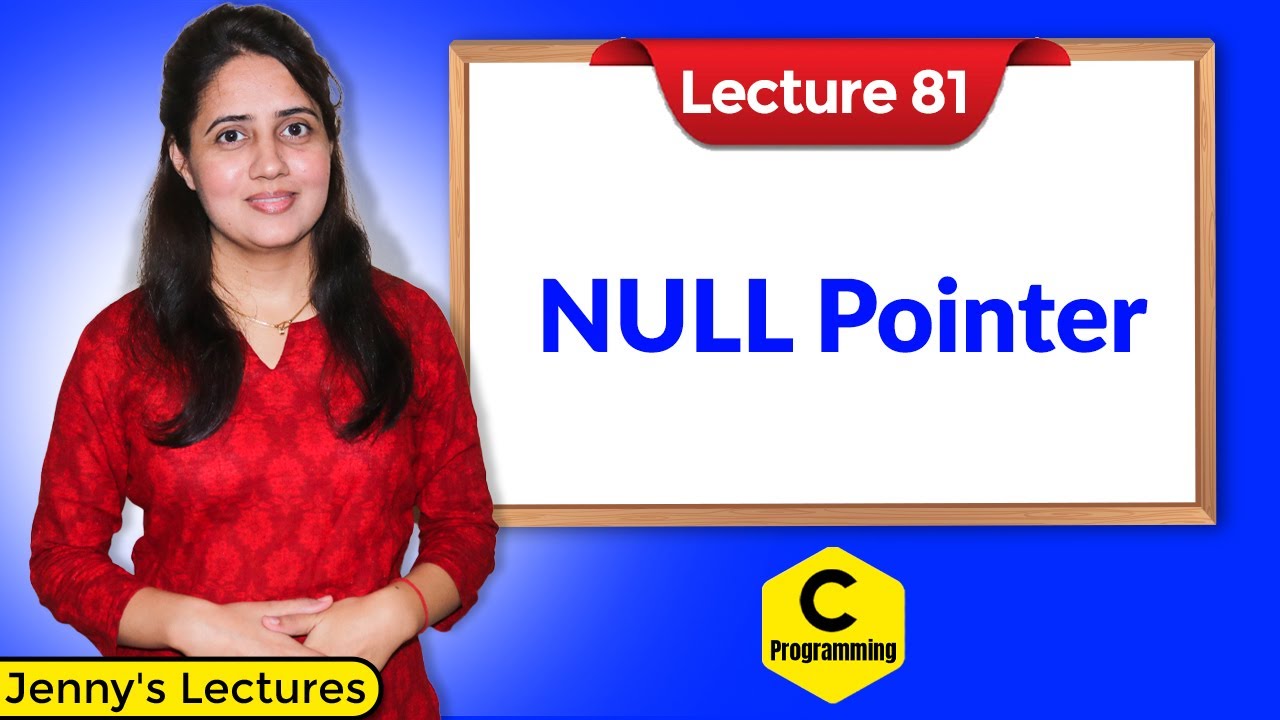
C_81 Null Pointer in C | C Programming Tutorials
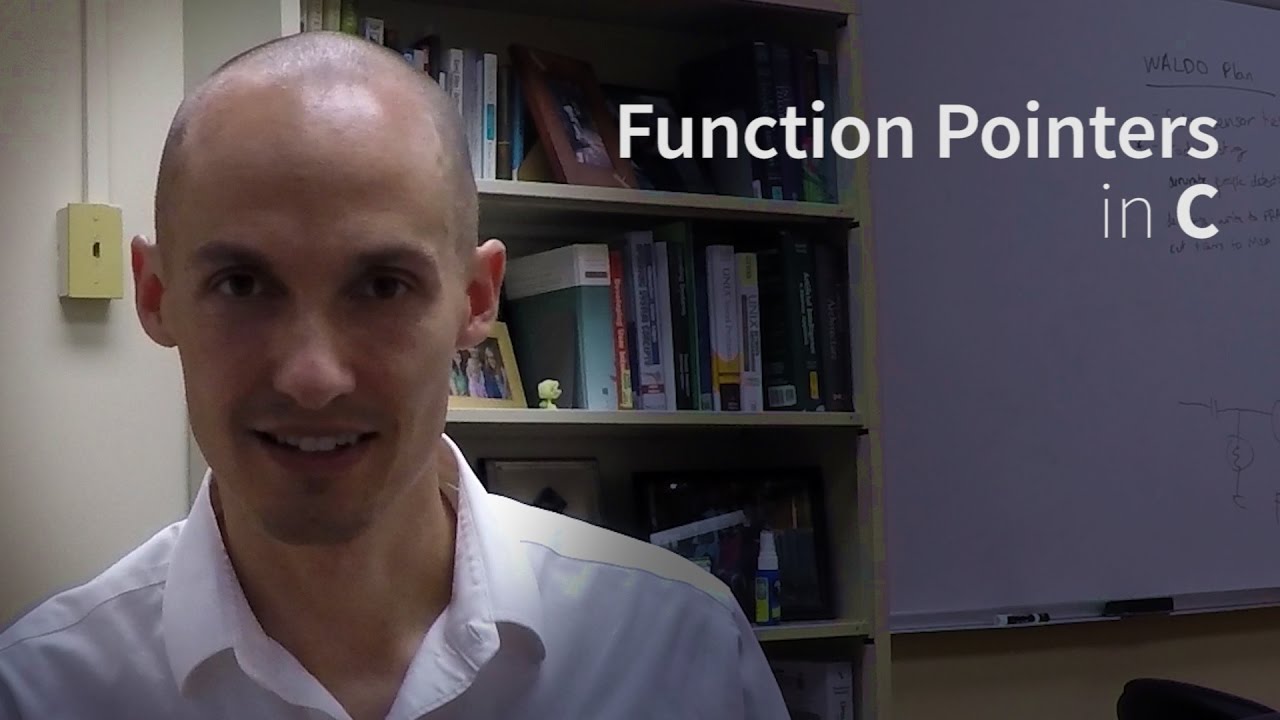
Understanding and Using Function Pointers in C
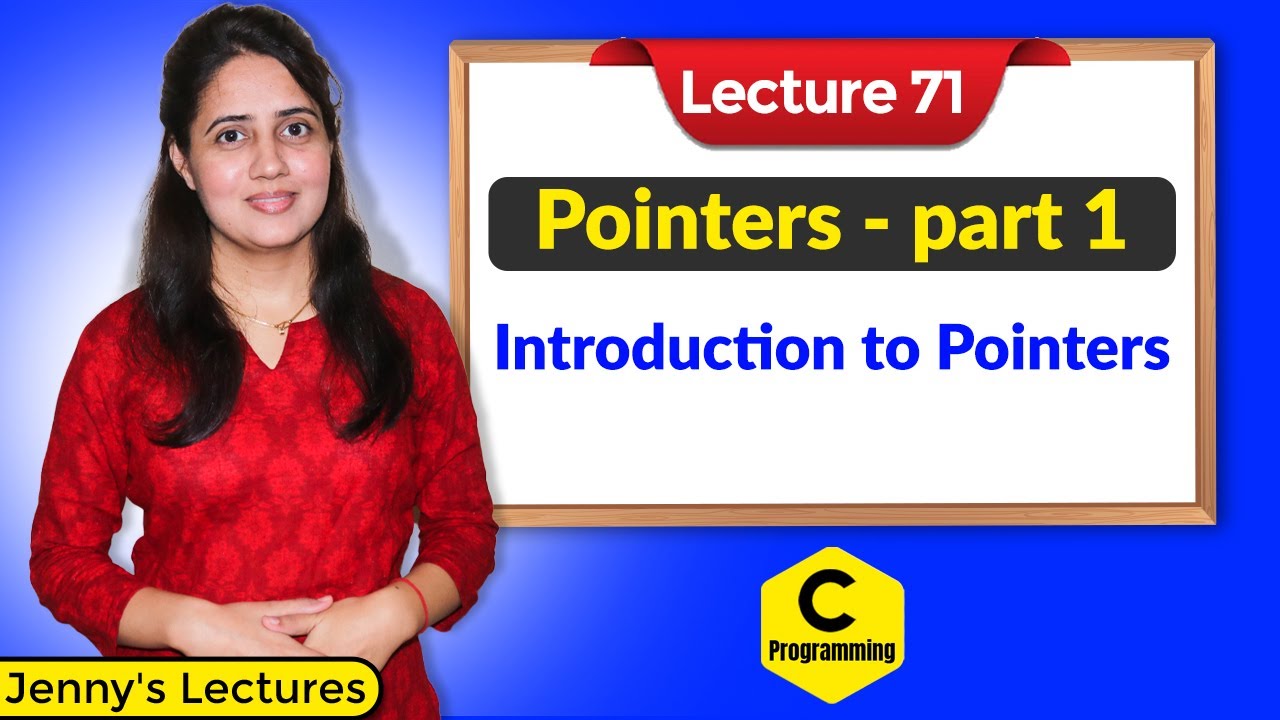
C_71 Pointers in C - part 1| Introduction to pointers in C | C Programming Tutorials

DAY 30 | COMPUTER SCIENCE | II PUC | POINTERS | L1
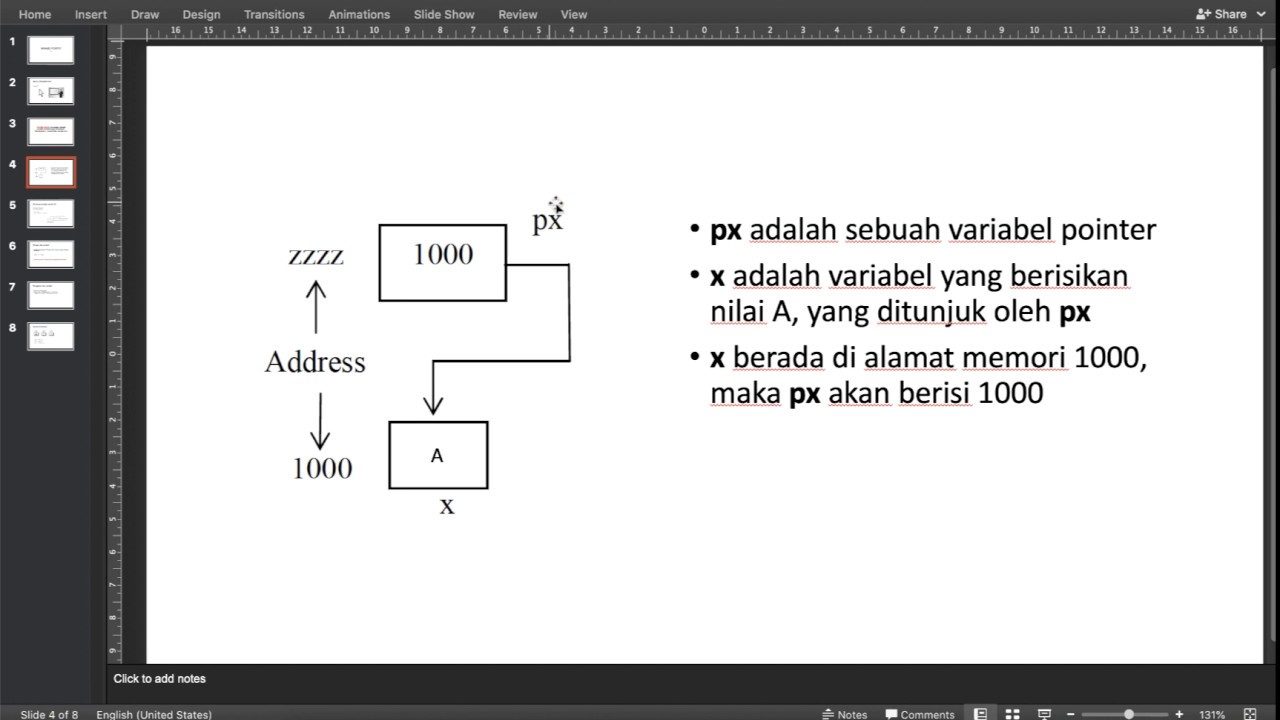
Variabel Pointer
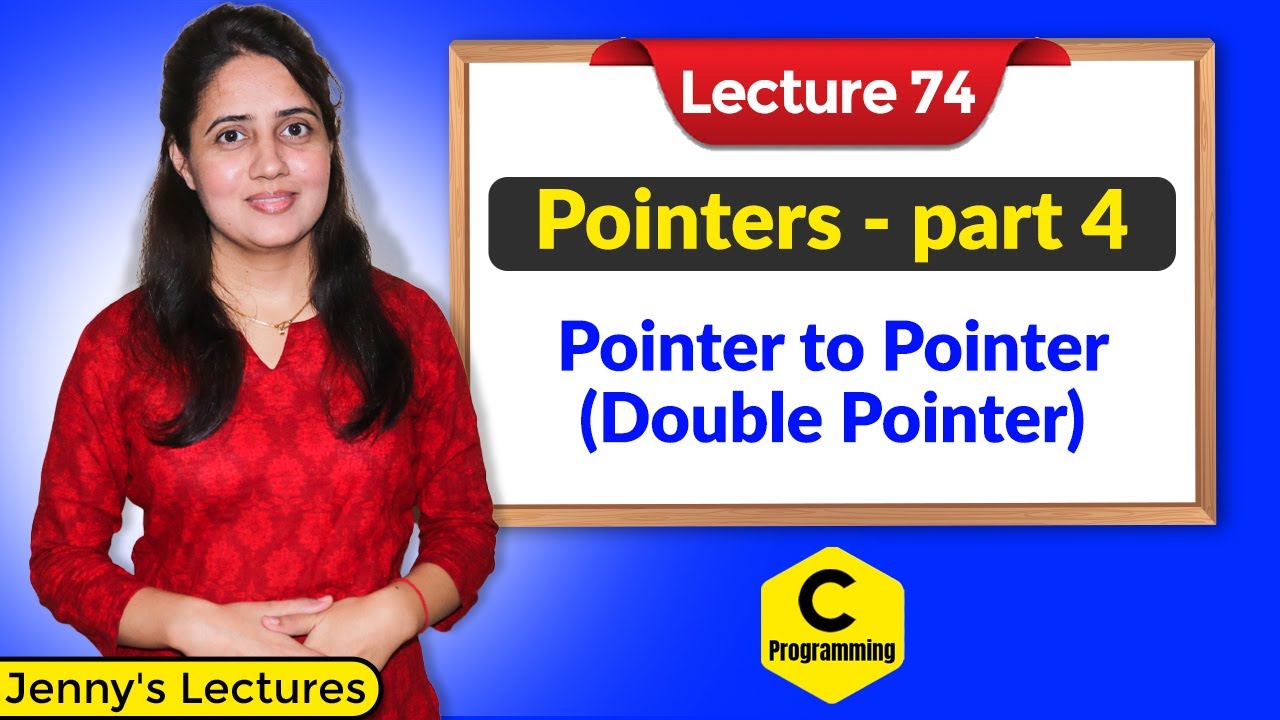
C_74 Pointers in C- part 4 | Pointer to Pointer (Double Pointer)
5.0 / 5 (0 votes)