3scopeintro
Summary
TLDRThis video script delves into the lifecycle of variables in computer memory, focusing on their creation and duration. It explores the scenario where two variables share the same name across different functions, using 'convert to minutes' and 'convert to seconds' as examples. The script explains how Python manages separate memory areas for each function's variables to avoid confusion. It illustrates the process of function calls, stack frames, and variable assignments, culminating in the demonstration of how the 'convert to seconds' function calculates the total number of seconds from hours.
Takeaways
- π The video discusses the lifecycle of variables in computer memory and how they are managed when two variables share the same name across different functions.
- π It uses a function visualization tool to illustrate the concepts, starting with the 'convert to minutes' and 'convert to seconds' functions.
- π The 'convert to seconds' function works by calling 'convert to minutes' and then multiplying the result by 60.
- π‘ Function objects are created when the function definitions are reached, and they are stored in the call stack as frames.
- π When a function is called, Python evaluates the arguments and creates a new stack frame, storing the memory address of the passed arguments.
- π The call stack is an area of memory that holds information about the active and inactive functions, shown as an upside-down stack of frames.
- π When a function exits, its stack frame is erased, and execution continues with the previous function.
- π Python keeps separate stack frames for each function call, ensuring that variables within different functions do not conflict.
- π’ The script explains how variables are evaluated and assigned within the stack frames, using the example of 'num_hours' and 'minutes'.
- π― It clarifies that when a variable does not exist in the current stack frame, it will be created there.
- π The video describes the process of returning values from functions, which involves erasing the current stack frame and passing the return value to the calling function.
- π The final takeaway is the demonstration of how the 'convert to seconds' function creates a new 'seconds' variable and returns its value, concluding with the result of 7,200 seconds.
Q & A
What is the main topic of the video?
-The main topic of the video is to explore how variables are created, their lifespan in computer memory, and the implications of having two variables with the same name in different functions.
What functions are discussed in the video?
-The functions discussed in the video are 'convert to minutes' and 'convert to seconds'.
How does the 'convert to seconds' function operate?
-The 'convert to seconds' function operates by calling the 'convert to minutes' function and then multiplying the result by 60.
What is a stack frame in the context of this video?
-A stack frame in this context is a structure that stores the memory address of variables and parameters during a function call. It is part of the call stack, which is an upside-down stack of frames.
How does Python handle variables with the same name in different functions?
-Python handles this by keeping the variables in separate areas of memory, one for each function's stack frame, to avoid confusion about which variable to use.
What happens when a function call is initiated in Python?
-When a function call is initiated, Python creates a new stack frame on the call stack and stores the memory address of the arguments and local variables.
What occurs when a function exits?
-When a function exits, the current stack frame is erased, and execution continues with the previous function in the call stack.
How is the value of a variable returned in a function?
-The value of a variable is returned by assigning it to a variable in the calling function's stack frame, which then contains the memory address of the returned value.
What is the purpose of the assignment statement in the 'convert to minutes' function?
-The purpose of the assignment statement in the 'convert to minutes' function is to calculate the number of minutes by multiplying 'num hours' by 60 and assigning the result to the variable 'minutes'.
How does the 'convert to seconds' function use the 'convert to minutes' function?
-The 'convert to seconds' function uses the 'convert to minutes' function by calling it with 'num hours' as an argument, then takes the returned number of minutes and multiplies it by 60 to get the total number of seconds.
What is the final value produced by the 'convert to seconds' function as per the script?
-The final value produced by the 'convert to seconds' function, as per the script, is 7,200 seconds.
Outlines
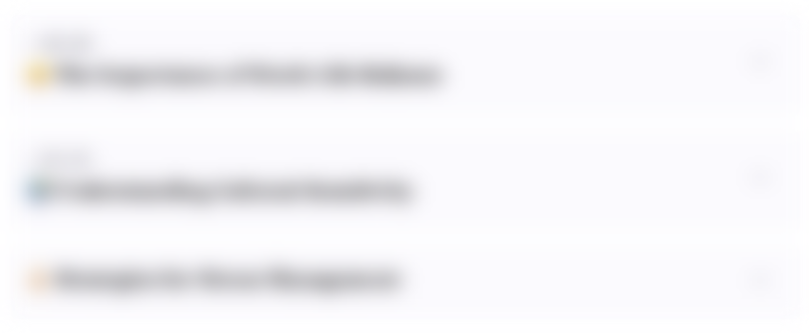
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
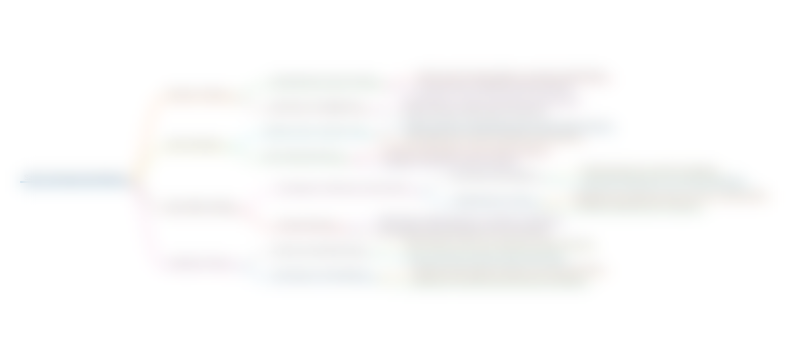
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
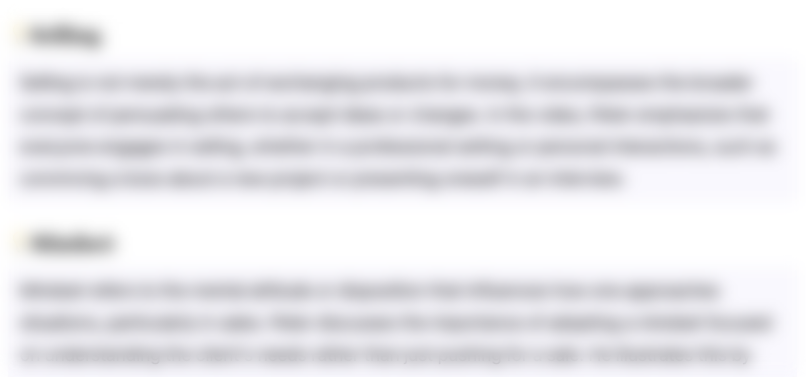
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
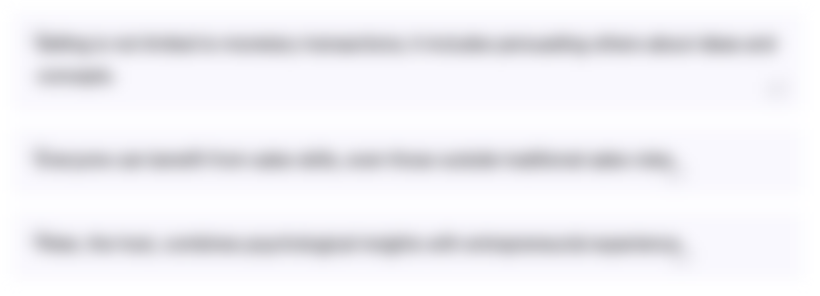
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
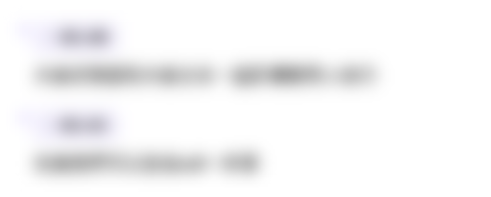
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

How JavaScript Code is executed? β€οΈ& Call Stack | Namaste JavaScript Ep. 2
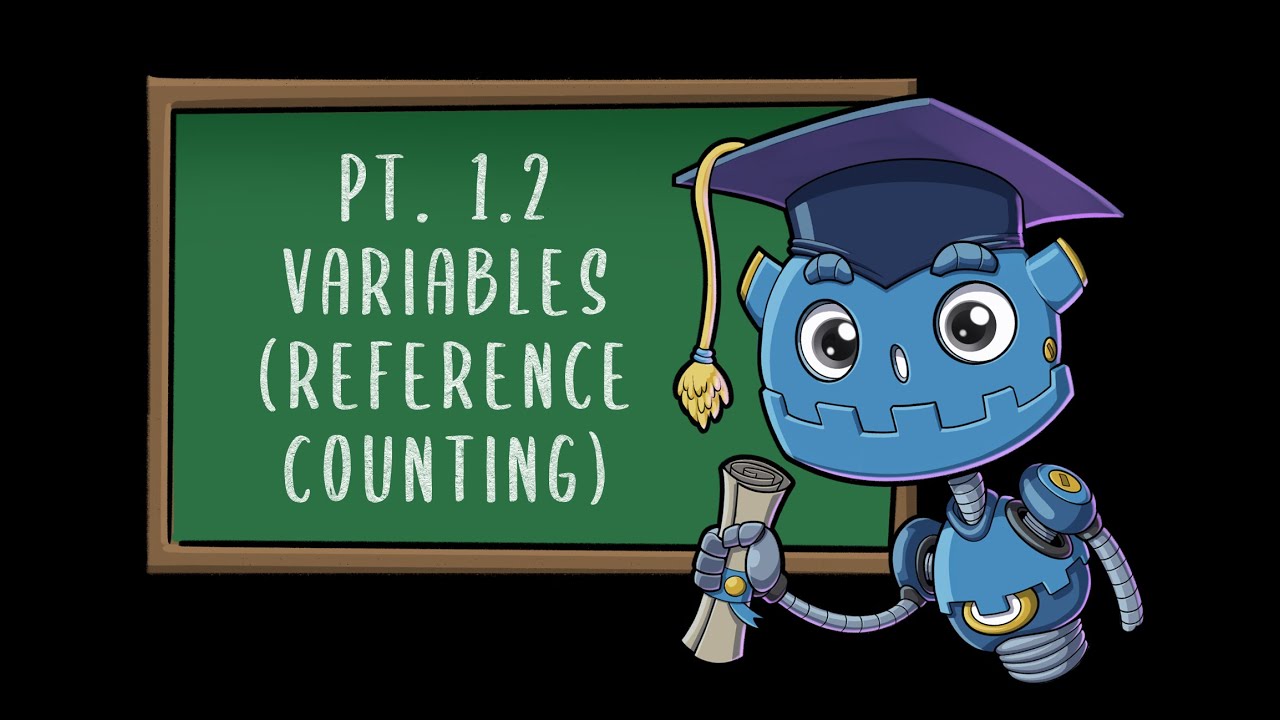
Life Cycle & Reference Counting | Godot GDScript Tutorial | Ep 1.2

Registers and RAM: Crash Course Computer Science #6

COS 333: Chapter 5, Part 1
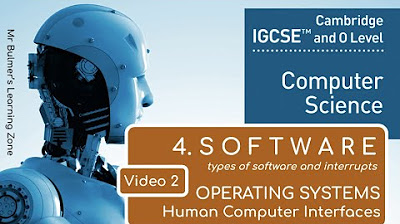
IGCSE Computer Science 2023-25 ββ- SOFTWARE: Video 2 - THE OPERATING SYSTEM
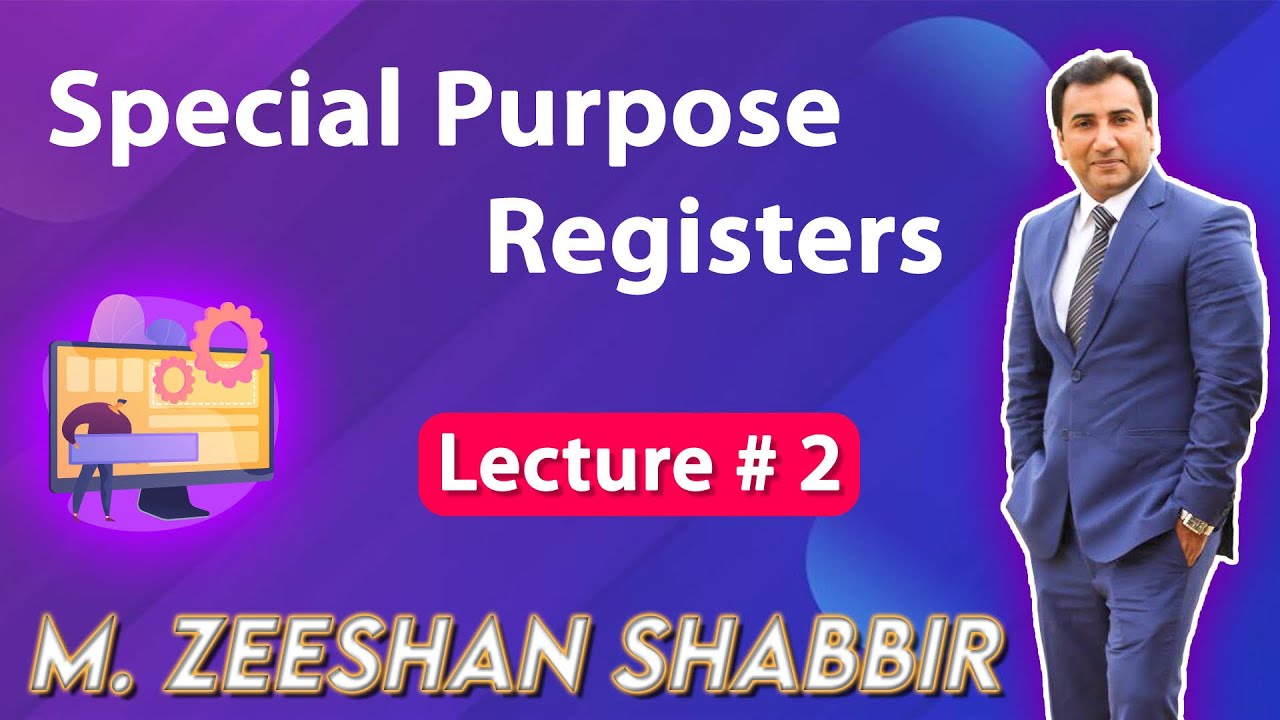
Registers & Special Purpose Registers in Assembly Language Urdu/Hindi | Lecture # 2 | very Important
5.0 / 5 (0 votes)