Producer Consumer Problem Program
Summary
TLDRThis tutorial explains how to solve the producer-consumer problem using semaphore variables. It covers the concepts of bounded and unbounded buffers, where producers create items and consumers consume them. The script details how synchronization between the producer and consumer is achieved through semaphore operations like 'wait' and 'signal', managing the access to shared resources. It walks through the use of mutex, full, and empty variables, demonstrating how they control item production and consumption. Key concepts such as buffer fullness, the importance of proper semaphore signaling, and avoiding synchronization issues are also discussed.
Takeaways
- 😀 Semaphore variables are used to implement synchronization between the producer and consumer in the producer-consumer problem.
- 😀 The producer generates items, while the consumer consumes those items from a shared buffer, either bounded or unbounded.
- 😀 In a bounded buffer, the producer is limited by a fixed number of slots and waits if the buffer is full until the consumer consumes an item.
- 😀 In an unbounded buffer, the producer can produce items indefinitely, and the consumer consumes them as they arrive without any limit on buffer size.
- 😀 Mutex, full, and empty are the semaphore variables used to control access to the shared buffer and prevent race conditions.
- 😀 The weight operation decrements the semaphore's value, while the signal operation increments it, ensuring proper synchronization.
- 😀 The mutex variable ensures mutual exclusion, allowing only one process (producer or consumer) to access the shared buffer at a time.
- 😀 The full semaphore keeps track of how many items have been produced, and the empty semaphore monitors available slots for the producer.
- 😀 The program avoids synchronization errors, such as deadlocks or incorrect buffer statuses, by correctly using the wait and signal operations on semaphores.
- 😀 In the case of an unbounded buffer, the consumer consumes items in sequence as they are produced by the producer, while the producer waits if the buffer is full in a bounded scenario.
Q & A
What is the producer-consumer problem?
-The producer-consumer problem involves a producer generating items and a consumer consuming them. The challenge lies in synchronizing these two processes to avoid issues like race conditions and deadlocks.
What is the role of semaphores in solving the producer-consumer problem?
-Semaphores are used for synchronization between the producer and the consumer. They manage access to shared resources by controlling the number of items in the buffer, ensuring that the producer does not produce when the buffer is full, and the consumer does not consume when it is empty.
What is the difference between a bounded and an unbounded buffer?
-A bounded buffer has a fixed limit on the number of items it can hold, meaning the producer must wait when the buffer is full. An unbounded buffer, on the other hand, allows the producer to produce an unlimited number of items without waiting.
What are the semaphore variables used in this program?
-The program uses three semaphore variables: 'mutex' (for binary synchronization), 'full' (which tracks the number of produced items), and 'empty' (which tracks the available slots for production).
How do the 'wait' and 'signal' operations function on semaphore variables?
-'Wait' decrements the value of a semaphore (typically signaling a process to wait until a resource is available), while 'signal' increments the value (indicating that the resource is now available for the next process).
What happens when the 'mutex' value becomes zero?
-When the 'mutex' value becomes zero, it indicates that a process (either the producer or consumer) has entered the critical section, and other processes must wait until it signals (increment) the mutex to allow another process to proceed.
What happens when the 'empty' semaphore reaches zero?
-When the 'empty' semaphore reaches zero, it means there are no available slots for the producer to add new items to the buffer, and the producer must wait for the consumer to consume items and free up space.
How does the producer signal the consumer that it has produced an item?
-After the producer produces an item, it signals the 'full' semaphore, indicating that there is one more item available for the consumer to consume.
How does the consumer know when an item is available for consumption?
-The consumer performs a 'wait' operation on the 'full' semaphore to ensure that there is an item available for consumption. Once the item is consumed, the consumer signals the 'empty' semaphore to indicate that a slot is now free.
What could happen if the signal and wait operations are not properly implemented?
-If the signal and wait operations are not correctly implemented, it could lead to issues like deadlock, where neither the producer nor the consumer can proceed, or incorrect behavior, such as trying to consume from an empty buffer or produce to a full buffer.
Outlines
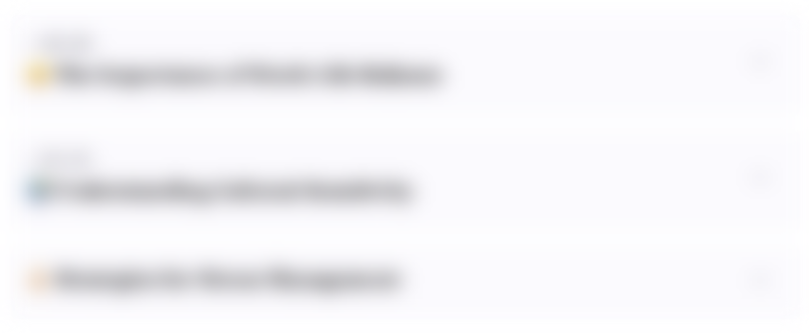
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
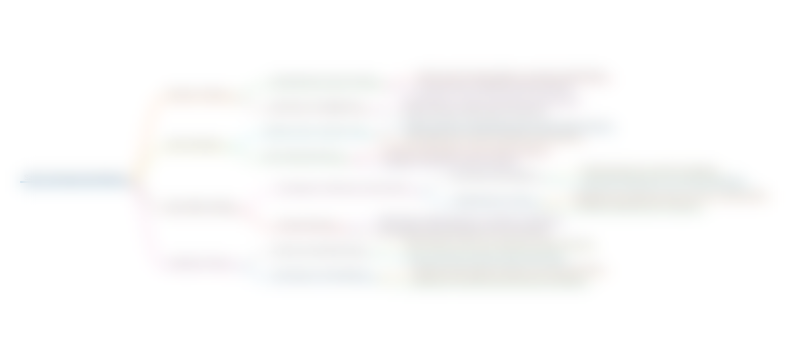
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
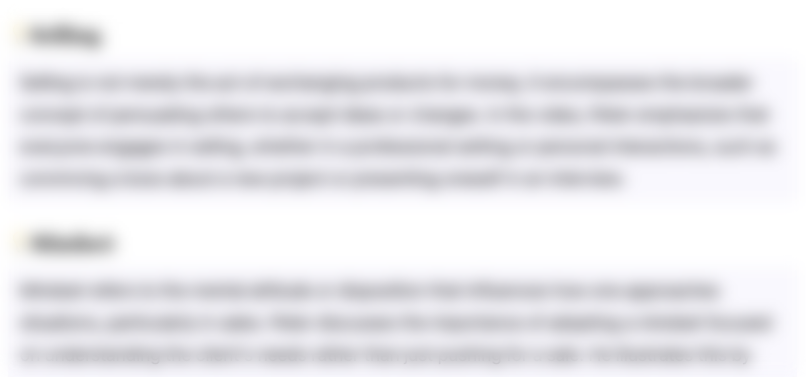
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
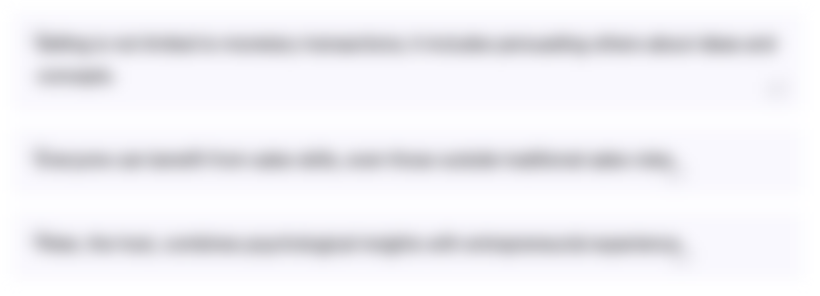
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
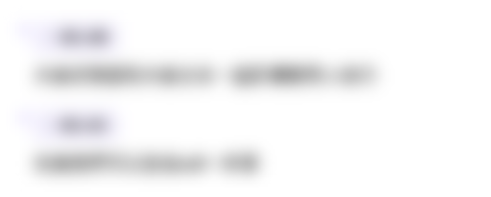
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
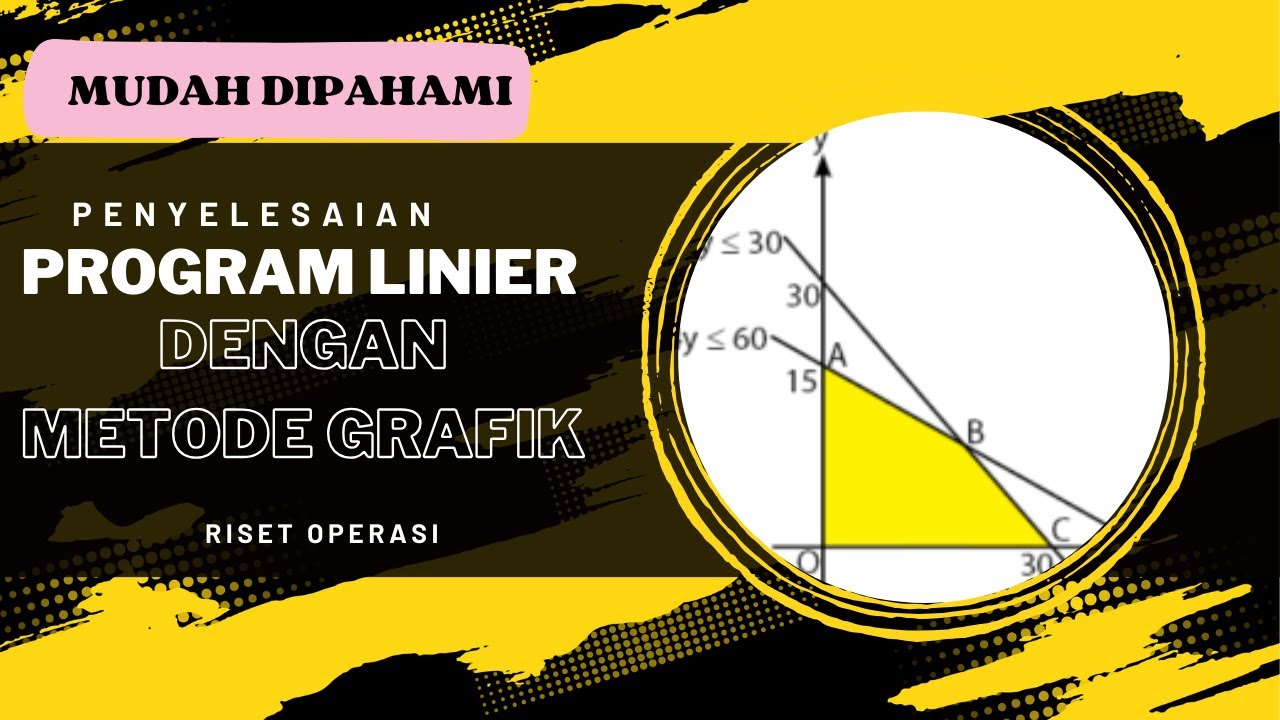
PROGRAM LINIER - METODE GRAFIK - RISET OPERASI
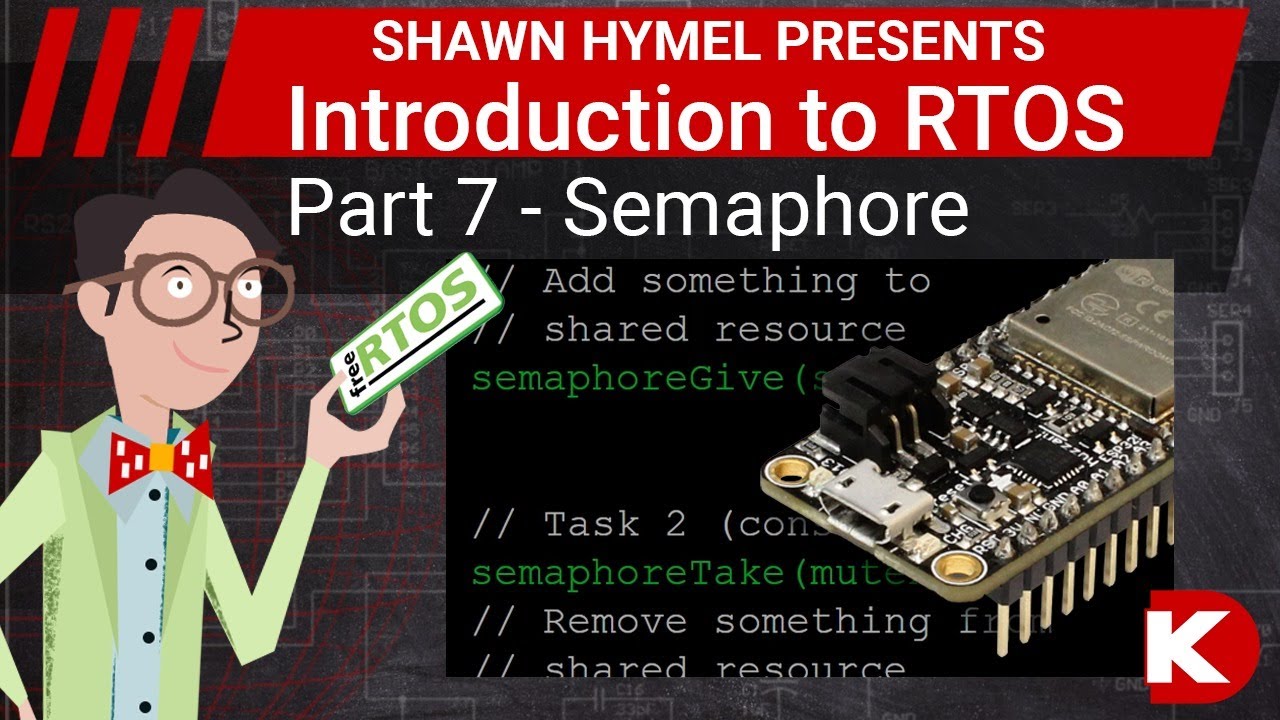
Introduction to RTOS Part 7 - Semaphore | Digi-Key Electronics

Kurikulum Merdeka Matematika Kelas 9 Bab 1 Sistem Persamaan Linear Dua Variabel
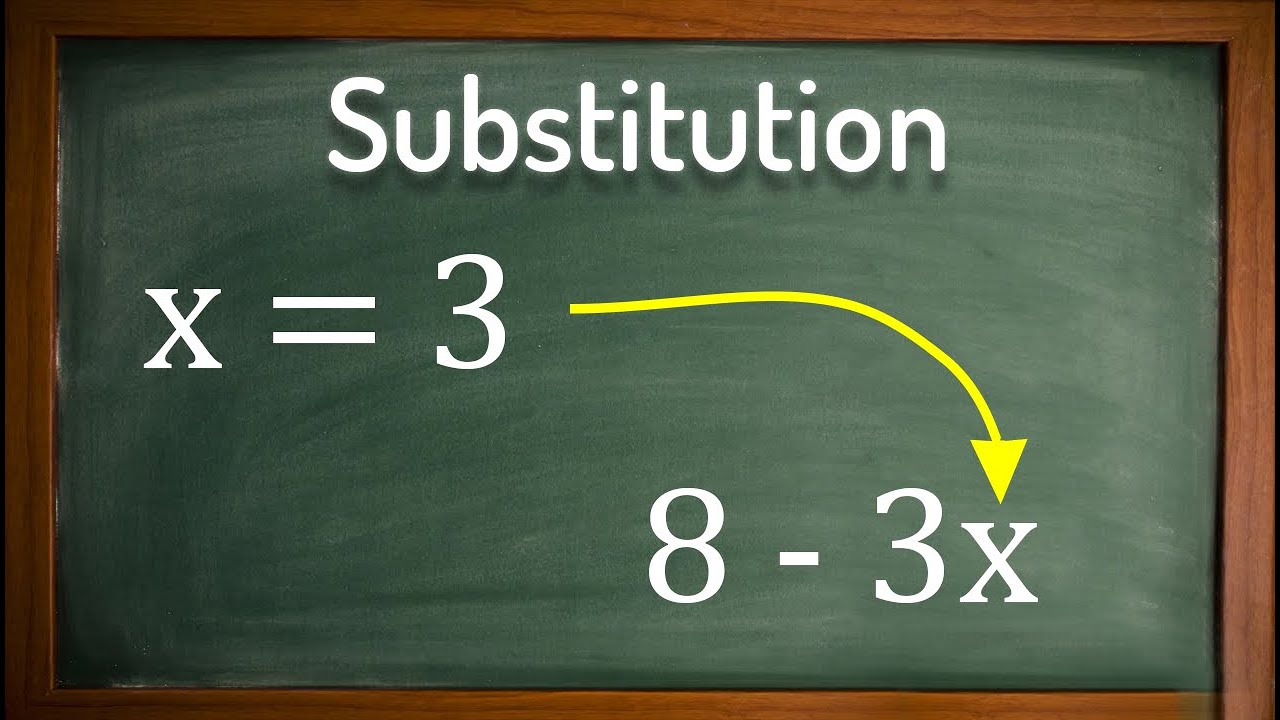
Algebra Substitution - GCSE Maths

Video Primagama | SMA | Ekonomi | Permintaan, Penawaran dan Harga Keseimbangan
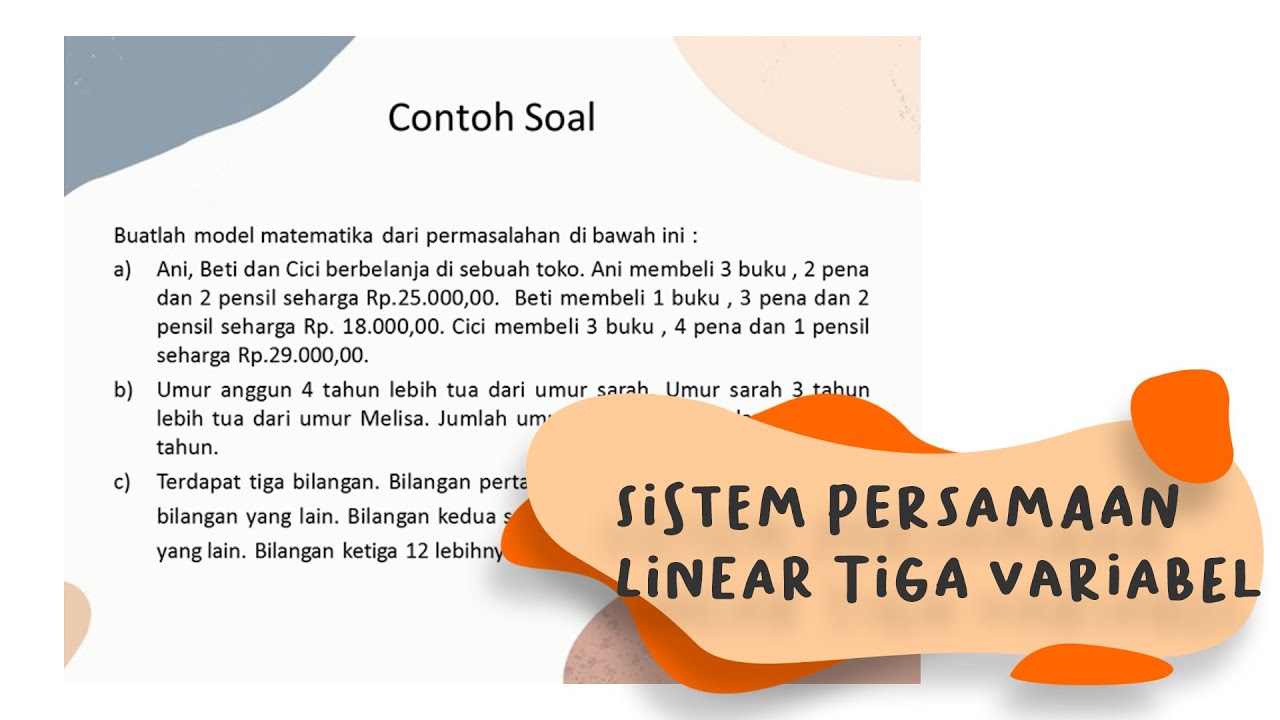
Sistem Persamaan Linear Tiga Variabel (SPLTV) membuat model matematika | by Iga Apriliana Mahardika
5.0 / 5 (0 votes)