Why you need hooks and project
Summary
TLDRThis video tutorial walks through the creation of a simple counter project in React, covering key concepts such as state management with `useState`, updating UI dynamically, and handling errors. The tutorial demonstrates how to handle click events to modify the counter value, explore functions for adding and removing values, and ensure values stay within specified limits (no negative values or values above 20). The session emphasizes React's automatic UI updates and provides practical advice on how to apply these concepts in real-world projects, with references to GitHub for code sharing.
Takeaways
- 😀 State handling in React is crucial for ensuring the UI is always synchronized with the data. React automatically updates the UI when the state changes.
- 😀 The 'counter' application demonstrates how to increment and decrement a value using React's state and event handling.
- 😀 React's `useState` hook is used to manage the counter value and trigger UI updates whenever the value is modified.
- 😀 Handling errors in state updates (e.g., trying to assign a new value to a constant) is important in React. Using `let` instead of `const` can resolve assignment issues.
- 😀 The 'add value' function increases the counter by one, showcasing how to modify state using a function that triggers updates.
- 😀 The 'remove value' function is another custom function that decreases the counter by one, ensuring the value can be decreased or incremented based on user input.
- 😀 You should ensure state updates propagate properly across all relevant components by passing the correct updated value to the setter function.
- 😀 It's essential to understand React's functional approach, where functions modify state and trigger UI updates without direct DOM manipulation.
- 😀 A good practice in React is using descriptive naming conventions for functions like `setCounter`, which makes the code more readable and maintainable.
- 😀 An important concept is preventing values from going below zero or above a specified limit (like 20) by adding validation logic to control state changes.
Q & A
What is the main concept being demonstrated in this React tutorial?
-The main concept being demonstrated is how to use React state with hooks (like `useState`) to manage a simple counter, including updating the state value and handling both increment and decrement operations.
What issue was encountered with the use of a constant for the counter value?
-The issue encountered was that a constant (`const`) was being used to store the counter value, which React expects to be mutable. As a result, it was replaced with a `let` variable to allow the state to be updated dynamically.
Why is it important to understand the 'why' behind the code in React?
-Understanding the 'why' behind the code helps developers grasp core concepts such as state management and component re-rendering. It enables them to make informed decisions when building applications, rather than just following instructions blindly.
What happens when the state is updated using React's `useState` hook?
-When the state is updated using the `useState` hook, React automatically triggers a re-render of the component, ensuring that the UI stays in sync with the latest state values.
What is the significance of using the `setCounter` function?
-The `setCounter` function is used to update the counter's state in React. It is important because it allows React to manage and track state changes and trigger the necessary component re-renders.
How does React's automatic re-rendering affect the counter's UI?
-React's automatic re-rendering ensures that whenever the counter value changes (via `setCounter`), the UI is updated to reflect the new value. This helps maintain synchronization between the state and the visual representation of the component.
What was the goal of the assignment mentioned in the script?
-The goal of the assignment was to implement logic that prevents the counter value from going below zero when decremented and also limits the counter value to a maximum of 20 when incremented.
Why did the instructor suggest that function names like `setCounter` are just conventions?
-The instructor emphasized that while `setCounter` is a common convention, the actual function names can be customized as long as they follow standard practices. This highlights the flexibility React offers in terms of naming and structuring the code.
How does the `removeValue` function work in the counter logic?
-The `removeValue` function is responsible for decrementing the counter. It updates the state by calling `setCounter` with the current counter value minus one, allowing the counter to decrease each time the function is triggered.
What is the importance of the `GitHub repository` mentioned in the video?
-The GitHub repository serves as a reference where students can access the source code for the tutorial. It allows them to review the code, practice by cloning the repository, and explore further modifications.
Outlines
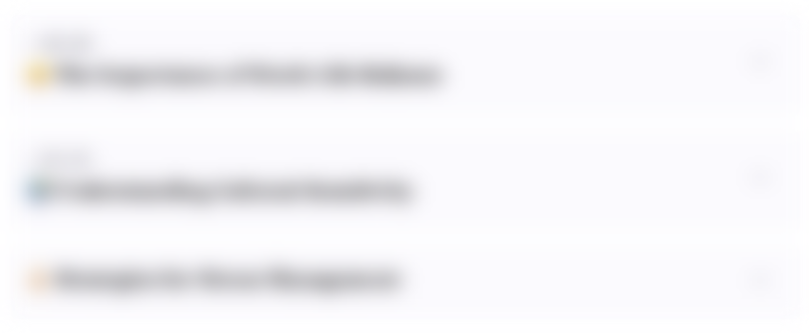
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
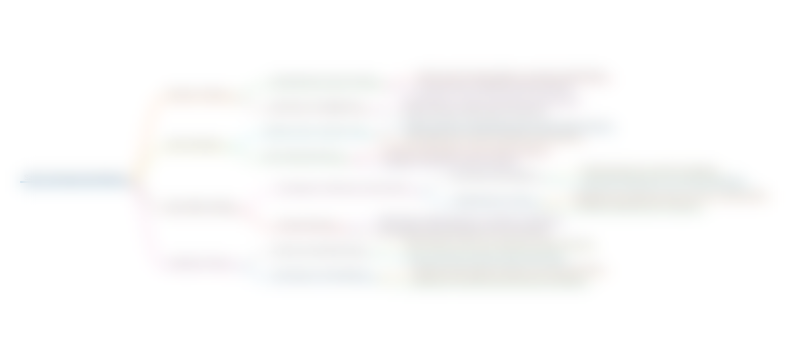
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
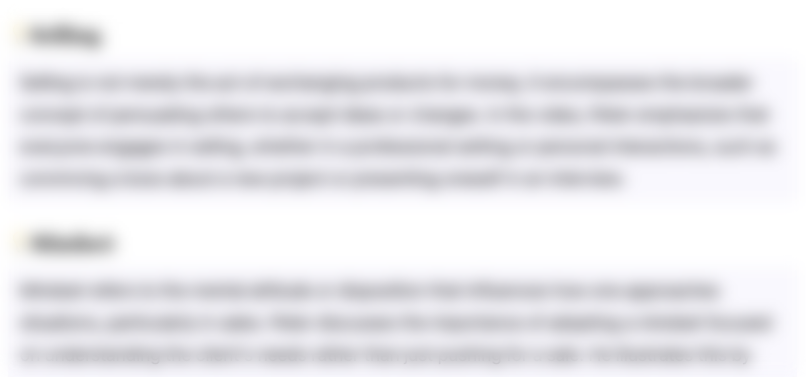
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
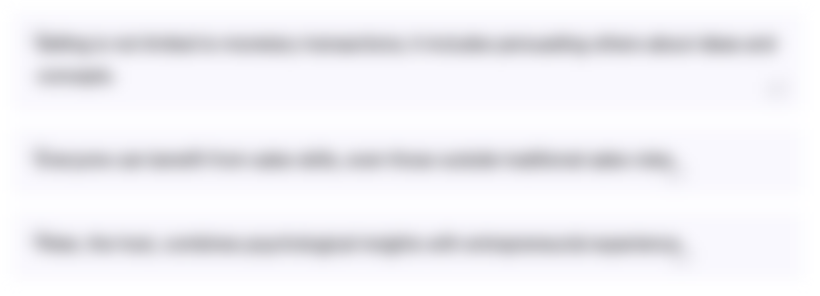
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
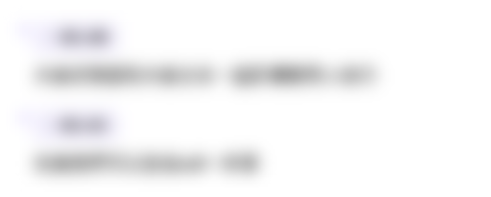
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

useState Hook | Mastering React: An In-Depth Zero to Hero Video Series
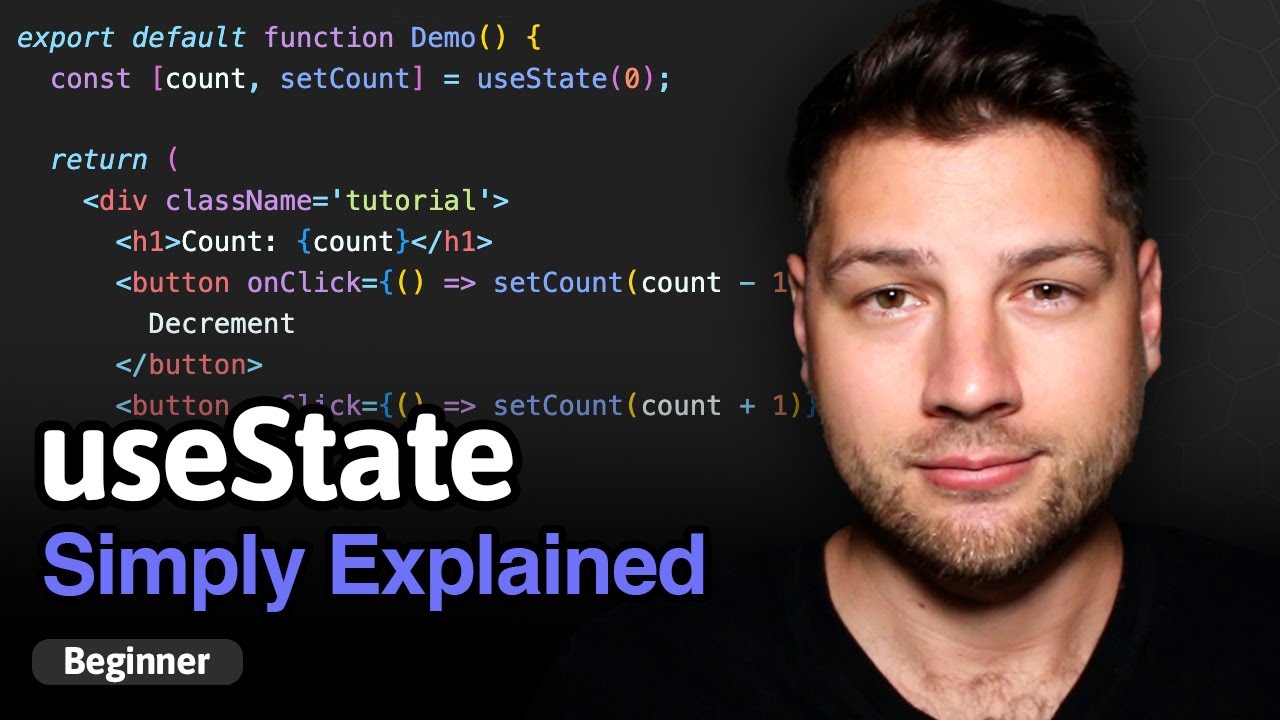
Learn React Hooks: useState - Simply Explained!
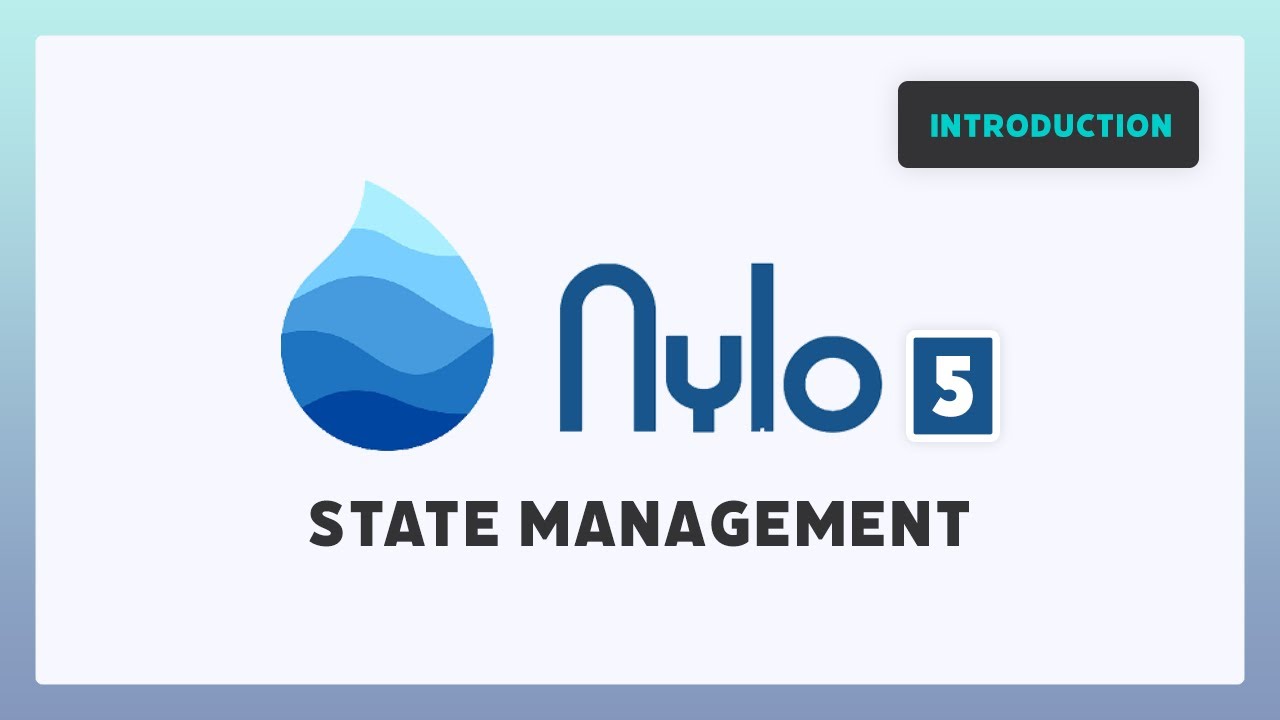
State Management in Nylo 5 | Flutter Framework
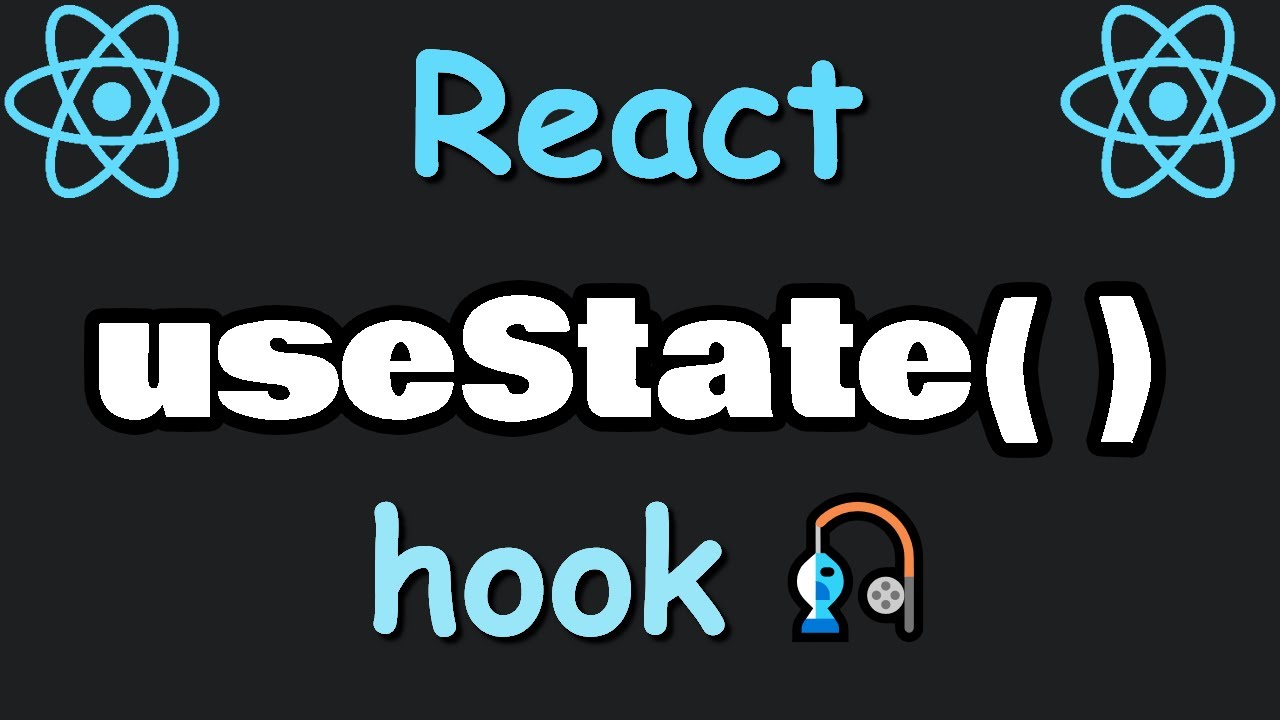
React useState() hook introduction 🎣

States | Mastering React: An In-Depth Zero to Hero Video Series
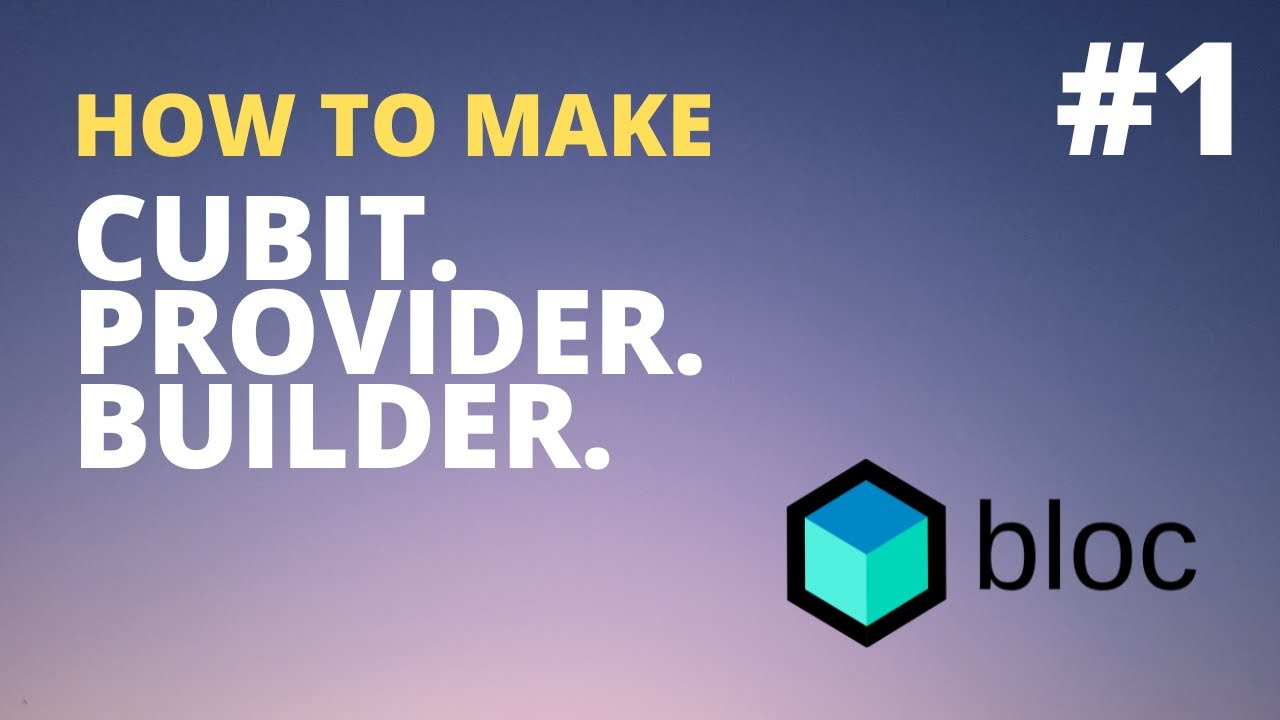
😽 MENURUTKU CUBIT/BLOC ITU POWERFUL LOH❗️❗️, KALAU TAU CARA PAKAINYA 😆
5.0 / 5 (0 votes)