React useState() hook introduction π£
Summary
TLDRIn this video, the presenter introduces React Hooks, a feature that enables functional components to use React features without writing class components, introduced in React 16.8. The focus is on the useState hook, which allows for stateful variables and their update functions. The tutorial walks through creating a reactive counter program, demonstrating how to use useState to manage state changes that trigger re-renders in the virtual DOM. The video also covers creating stateful variables for name, age, and Boolean values, and concludes with styling the counter with CSS.
Takeaways
- π React hooks were introduced in version 16.8, allowing functional components to use state and other React features without writing class components.
- π The `useState` hook is fundamental for creating stateful variables and their setter functions within functional components.
- π‘ Using `useState` provides a way to update state and trigger re-renders in the React virtual DOM, unlike regular variables which do not cause re-renders.
- π οΈ The `useState` hook returns an array with two elements: the current state value and a function to update it.
- π When using `useState`, you can optionally pass an initial state value; if not provided, it defaults to `null`.
- π The script demonstrates creating a counter program using `useState` to manage incrementing, decrementing, and resetting a count.
- π¨ Conditional rendering is shown by toggling a Boolean state to display 'yes' or 'no' based on its value.
- π©βπ» The video includes a step-by-step guide on setting up a new React component and using hooks within it.
- π The script covers the necessity of importing React and destructuring `useState` from it to use within functional components.
- ποΈ CSS styling is applied to enhance the visual presentation of the counter component, demonstrating the integration of React functionality with styling.
Q & A
What is a React Hook?
-A React Hook is a special function that allows functional components to use React features without writing class components. It was introduced in React version 16.8.
Why were React Hooks introduced?
-React Hooks were introduced to allow developers to use state and other React features in functional components without the need to write class components.
What is the most widely used React Hook?
-The most widely used React Hook is `useState`, which allows the creation of a stateful variable and a setter function to update its value.
How does the `useState` hook work?
-The `useState` hook returns an array with two elements: a stateful variable and a setter function. The setter function is used to update the state, which triggers a re-render of the component.
Can you provide an example of how to use the `useState` hook?
-To use `useState`, you import it from React and call it within a functional component. For example, `const [name, setName] = useState('');` creates a stateful variable `name` and a setter function `setName`.
What is the purpose of the setter function returned by `useState`?
-The setter function returned by `useState` is used to update the stateful variable. When called with a new value, it updates the state and causes the component to re-render with the new state.
How can you set an initial state with `useState`?
-You can set an initial state by passing it as an argument to the `useState` hook. For example, `const [age, setAge] = useState(0);` sets the initial state of `age` to 0.
What is the difference between a normal variable and a stateful variable in React?
-A normal variable does not trigger a re-render when updated, whereas a stateful variable created with `useState` does trigger a re-render, allowing the UI to reflect the updated state.
Can you explain the concept of conditional rendering in the context of the `useState` hook?
-Conditional rendering with `useState` can be achieved using logical operators to determine what to display based on the state. For example, `isEmployed ? 'Yes' : 'No'` will display 'Yes' if `isEmployed` is true, and 'No' otherwise.
How do you create a counter program using React Hooks?
-To create a counter program, you use the `useState` hook to manage the count state and provide functions to increment, decrement, and reset the count. These functions are then used as event handlers for buttons in the component's JSX.
What are some other React Hooks mentioned in the script?
-Other React Hooks mentioned in the script include `useEffect`, `useContext`, `useReducer`, and `useCallback`, each serving different purposes such as managing side effects, providing context, and optimizing performance.
Outlines
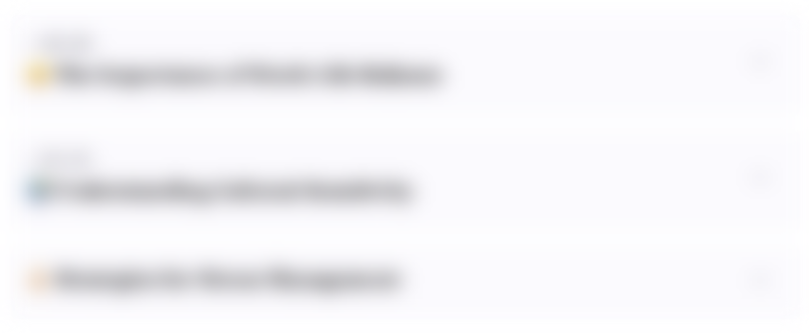
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
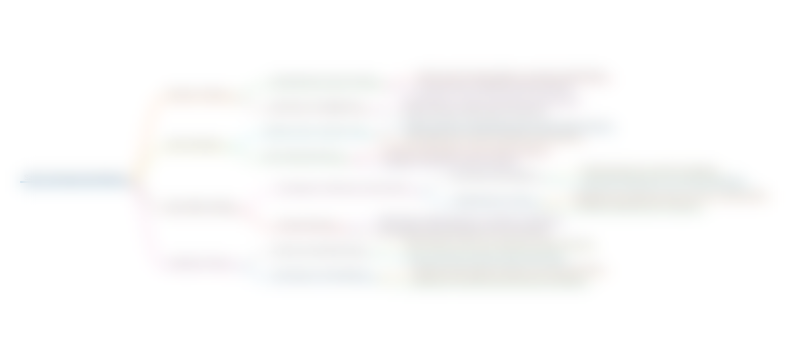
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
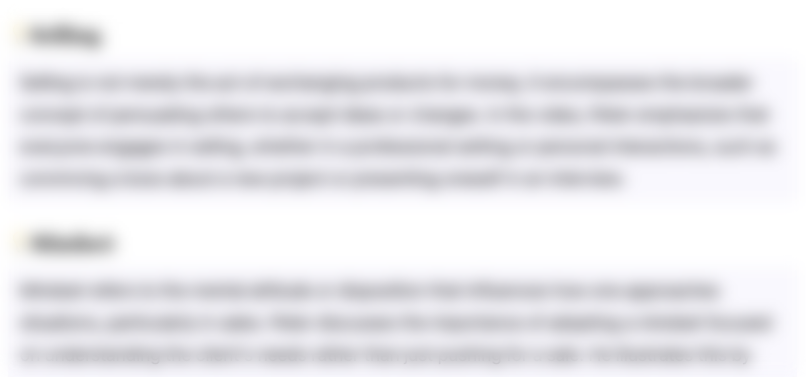
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
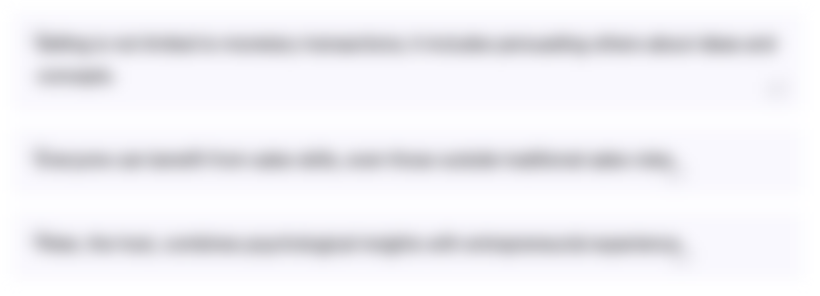
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
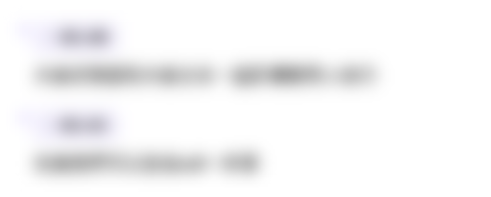
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
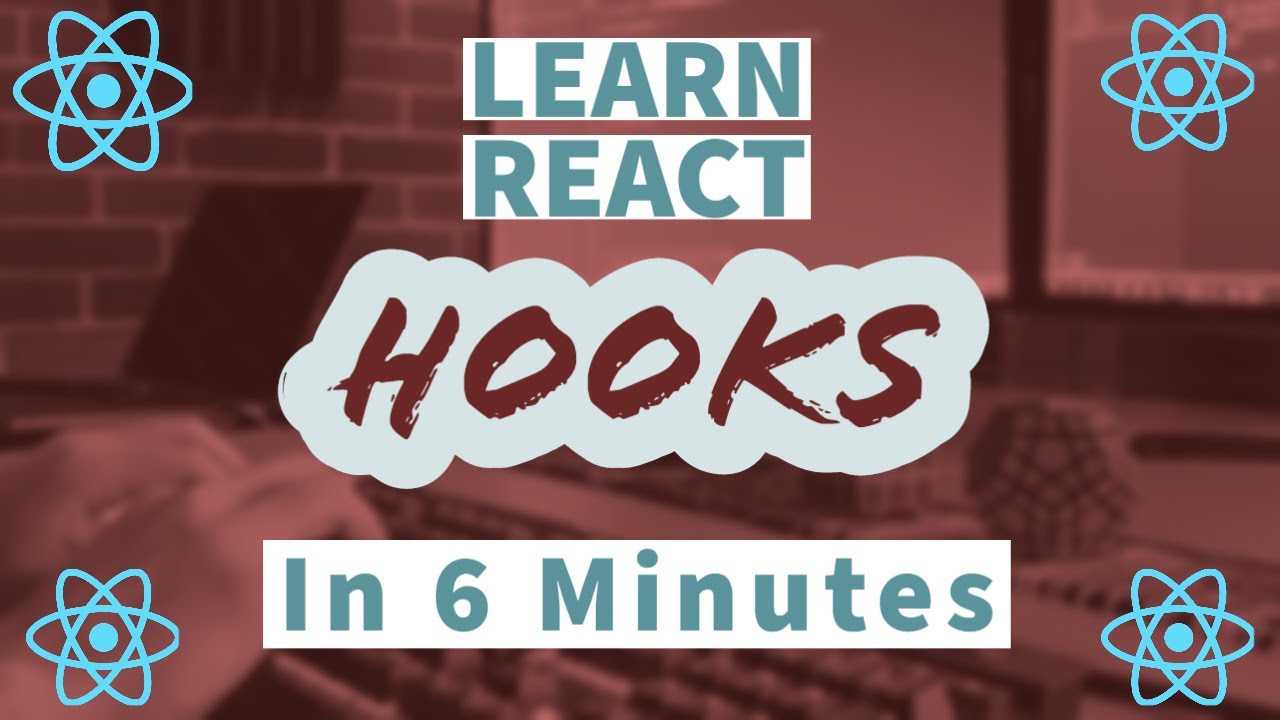
Learn React Hooks In 6 Minutes
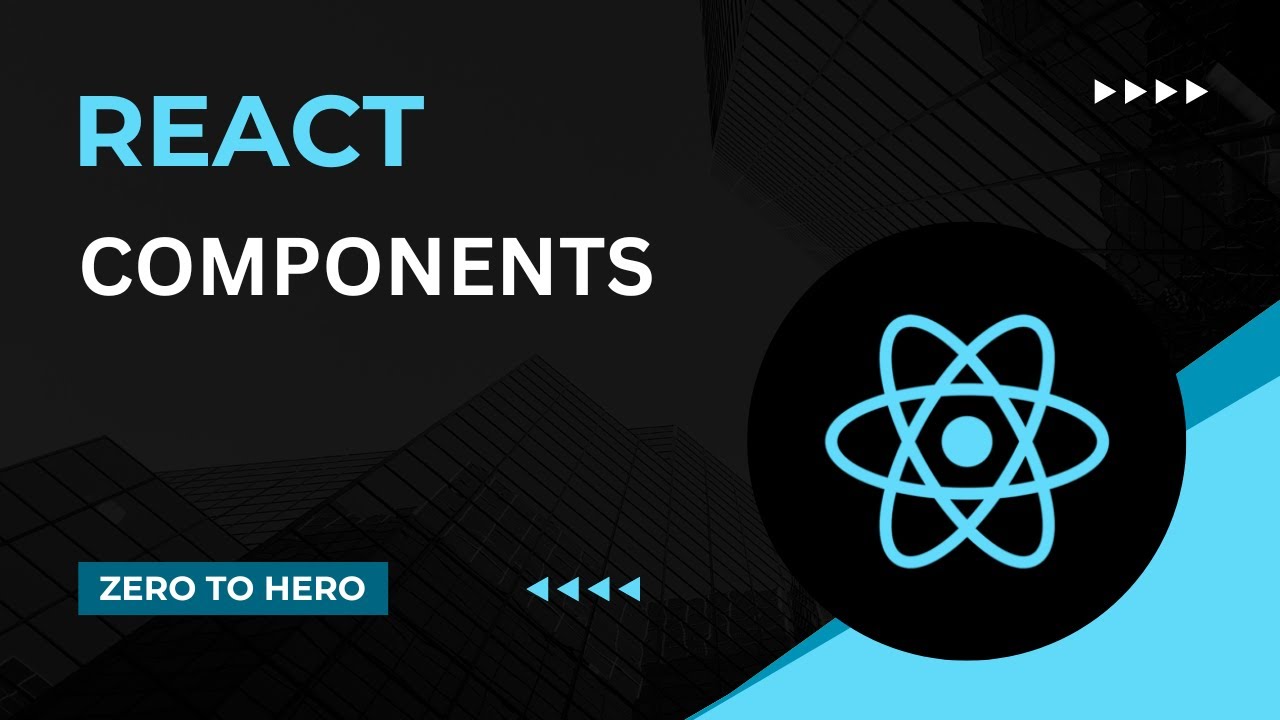
Components | Mastering React: An In-Depth Zero to Hero Video Series

React Hooks and Their Rules | Lecture 157 | React.JS π₯
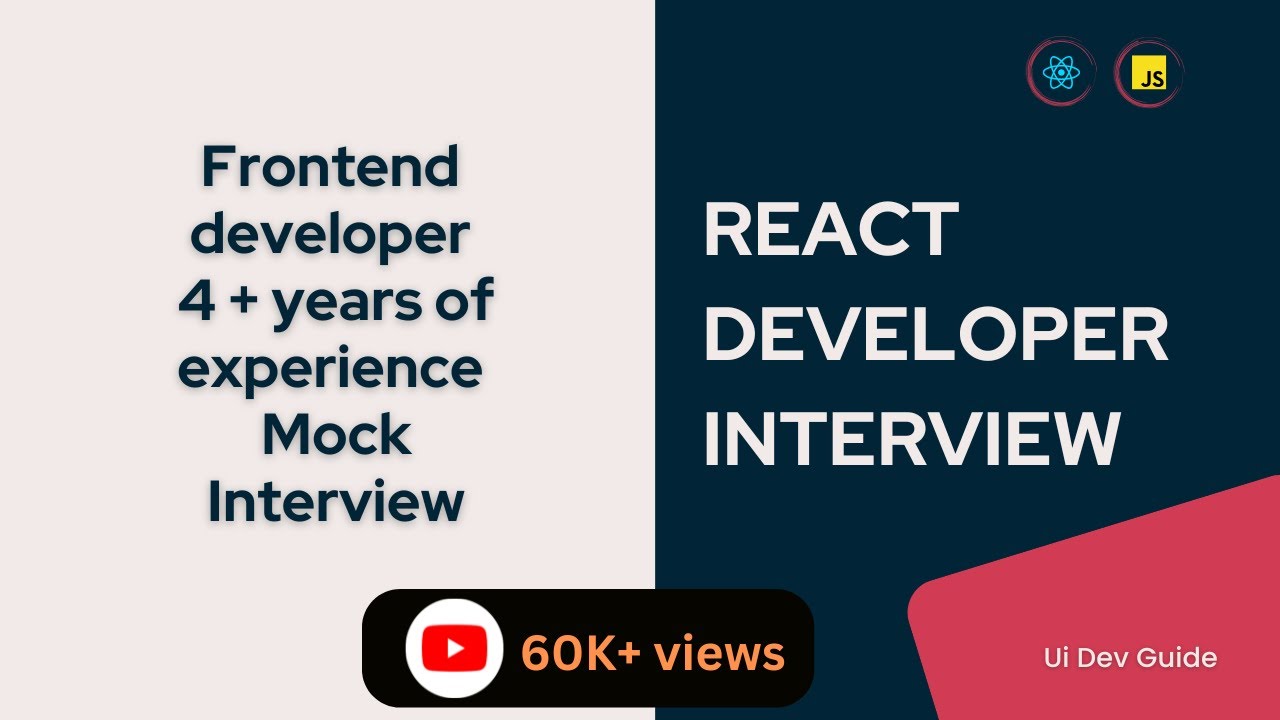
React Interview | Frontend Developer | React interview questions 5 to 8 years 2023 #reactjs
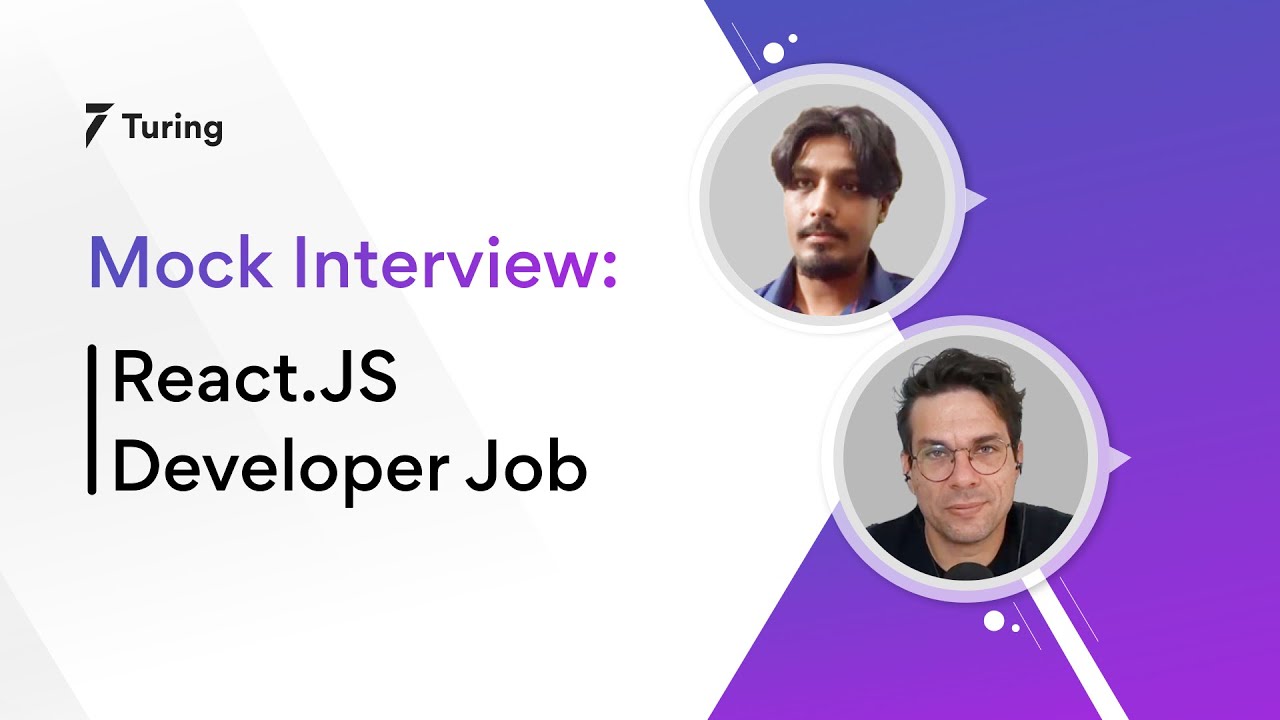
React.JS Mock Interview | Interview Questions for Senior React.JS Developers

What are Custom Hooks When to Create One | Lecture 166 | React.JS π₯
5.0 / 5 (0 votes)