Data Structures: Crash Course Computer Science #14
Summary
TLDR这段视频脚本介绍了计算机科学中使用的基本数据结构,包括数组、字符串、矩阵、结构体、链表、队列、栈、树和图。数组是存储在内存中的一系列值,可以通过索引访问。字符串是字符的数组,以空字符结尾。矩阵是数组的数组,用于存储二维数据。结构体允许将多个变量捆绑在一起存储。链表是一种灵活的数据结构,可以通过改变节点的指针来动态地添加或删除节点。队列遵循先进先出(FIFO)的原则,而栈遵循后进先出(LIFO)的原则。树是一种层级结构,其中每个节点可能有多个子节点。图是一种更为通用的数据结构,允许任意节点之间的连接。不同的数据结构适用于不同的计算任务,选择正确的数据结构可以大大简化编程工作。大多数编程语言都提供了内置的数据结构库,如C++的标准模板库和Java的类库,这使得程序员可以不必从头开始实现这些结构,而是利用它们进行更高层次的抽象和更有趣的编程工作。
Takeaways
- 📚 数据结构对于算法的执行至关重要,它们决定了数据在计算机内存中的存储方式。
- 🔍 数组是基本的数据结构,可以存储一系列值,并通过索引来访问特定的值。
- 🔑 字符串实际上是字符的数组,以null字符(二进制0)结束,以标识字符串在内存中的结束。
- 🖇️ 矩阵可以视为数组的数组,用于存储二维数据,如电子表格中的网格或屏幕上的像素。
- 🏢 结构体(Struct)允许将相关的变量组合在一起存储,如银行账户号和余额。
- 🔗 指针是一种特殊变量,指向内存中的一个位置,用于创建链表等灵活的数据结构。
- 🔄 链表是一种可以动态扩展或缩短的数据结构,通过改变节点的指针来添加或删除元素。
- 📈 队列(Queue)和栈(Stack)是基于链表的更复杂的数据结构,分别遵循FIFO和LIFO原则。
- 🌳 树是一种数据结构,由节点组成,每个节点有零个或多个子节点,用于表示层次关系。
- 🔍 二叉树是特殊的树,每个节点最多有两个子节点,常用于各种算法中。
- 🔗 图(Graph)是一种更为通用的数据结构,允许节点之间有任意的连接,包括循环。
- 🛠️ 编程语言通常提供内置的或库中的数据结构,如C++的STL和Java的JCL,以简化实现复杂数据结构的工作。
Q & A
数据结构在计算机科学中的作用是什么?
-数据结构在计算机科学中用于组织和存储数据,以便数据可以被高效地检索和读取。它们允许算法以一种结构化的方式运行,从而提高程序的性能和效率。
数组(Array)在内存中是如何存储的?
-数组在内存中是连续存储的。它们是一系列值,按照内存地址的连续性存放。例如,一个包含7个数字的数组可能被存储在内存位置1000开始的地方,这些数字一个接一个地存储在连续的内存地址中。
如何通过索引访问数组中的特定值?
-通过指定索引来访问数组中的特定值。几乎所有的编程语言都从索引0开始计数,使用方括号语法来表示数组访问。例如,要访问数组中索引为5的元素,程序会跳转到内存位置1000加上5的偏移量。
字符串(String)是如何存储在内存中的?
-字符串实际上是字符的数组。它们在内存中存储时,每个字符占用一个位置,字符串以一个特殊的null字符(二进制值0)结束,这表示字符串的结束。
矩阵(Matrix)可以如何表示?
-矩阵可以被看作是数组的数组。例如,一个3x3的矩阵实际上是一个包含3个元素的数组,每个元素本身也是一个包含3个元素的数组。
如何通过索引访问矩阵中的特定值?
-访问矩阵中的特定值需要指定两个索引。例如,要访问矩阵中索引为(2,1)的元素,意味着要查找子数组2中索引为1的元素。
结构体(Struct)在数据存储中有什么作用?
-结构体允许将相关的变量组合在一起存储。它们可以创建复合数据结构,能够一次性存储多个数据片段,如银行账户的账号和余额。
链表(Linked List)与数组相比有什么优势?
-链表是一种灵活的数据结构,可以通过改变节点的指针来动态地扩展或缩短。与数组相比,链表可以轻松地插入、删除、排序、分割和反转,非常适合需要频繁变动的数据结构。
队列(Queue)和栈(Stack)是如何基于链表实现的?
-队列遵循先进先出(FIFO)的原则,像邮局排队一样,通过改变链表的头指针来实现入队(enqueue)和出队(dequeue)。栈遵循后进先出(LIFO)的原则,像堆叠的煎饼,通过压栈(push)和弹栈(pop)操作来管理数据。
树(Tree)和图(Graph)数据结构的主要区别是什么?
-树是一种有层级结构的数据结构,其中每个节点除了有一个父节点外,还可以有多个子节点,但树中的节点没有循环。而图是一种更为通用的数据结构,允许任意两个节点之间的连接,包括循环。
为什么选择合适的数据结构对于编程很重要?
-选择合适的数据结构可以显著提高程序的性能和开发效率。不同的数据结构有不同的特性,适用于不同的计算需求。大多数编程语言都提供了丰富的数据结构库,如C++的Standard Template Library和Java的Java Class Library,使得程序员可以不必从零开始实现数据结构。
Outlines
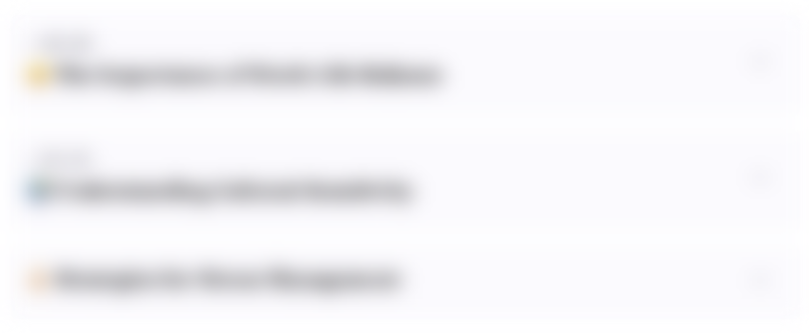
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
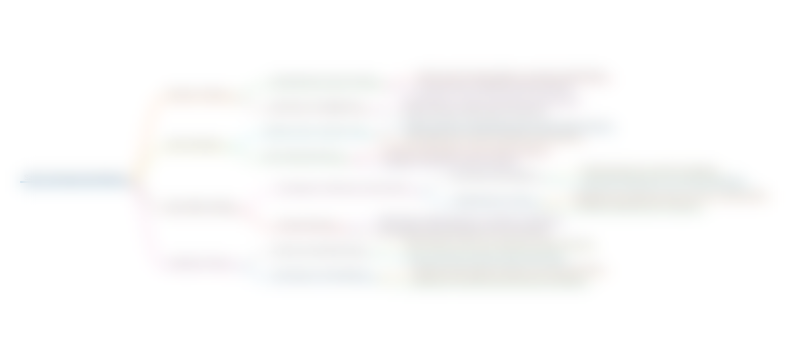
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
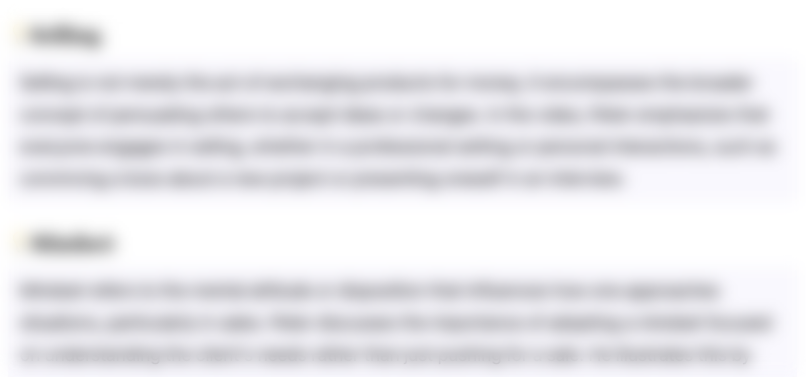
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
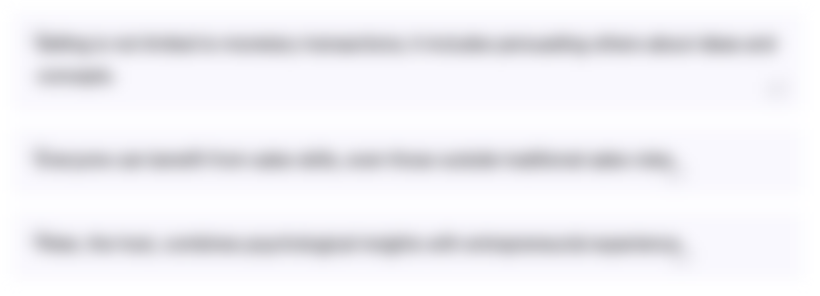
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
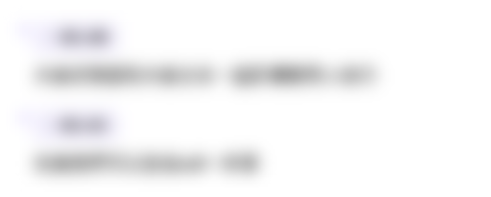
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
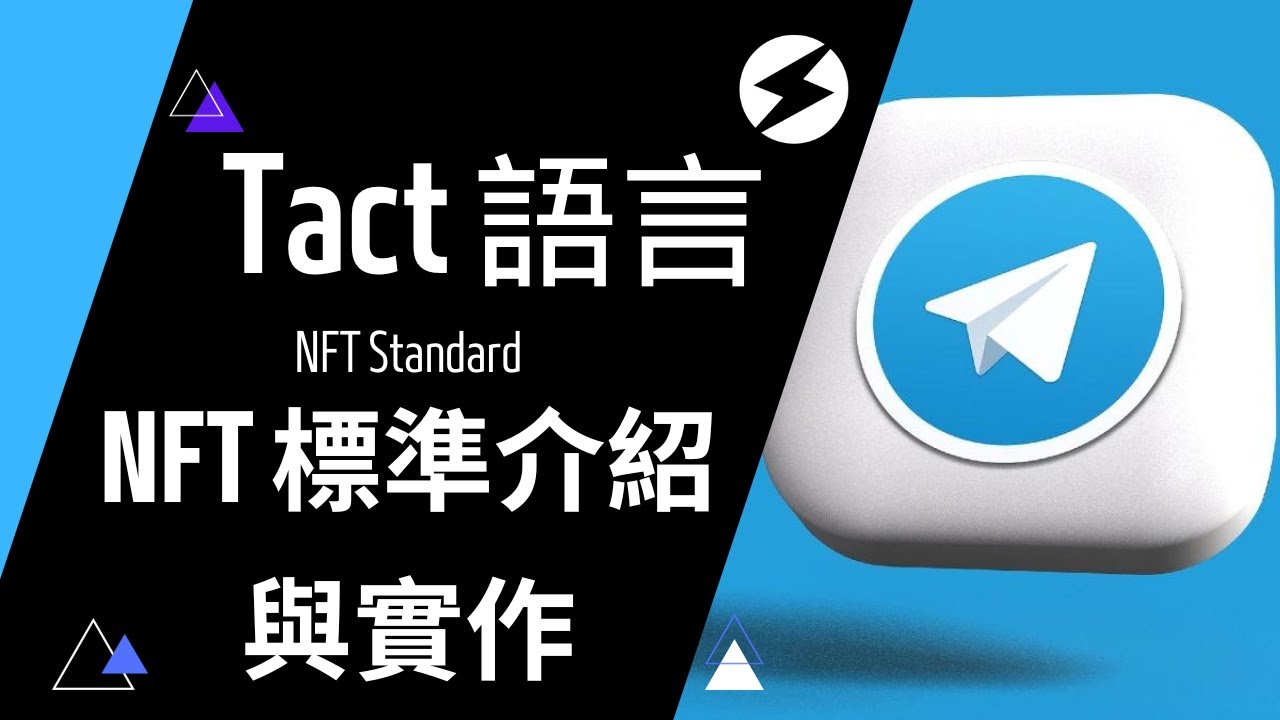
快速上手了解智能合約(NFT標準) | TON Blockchain, TEP62
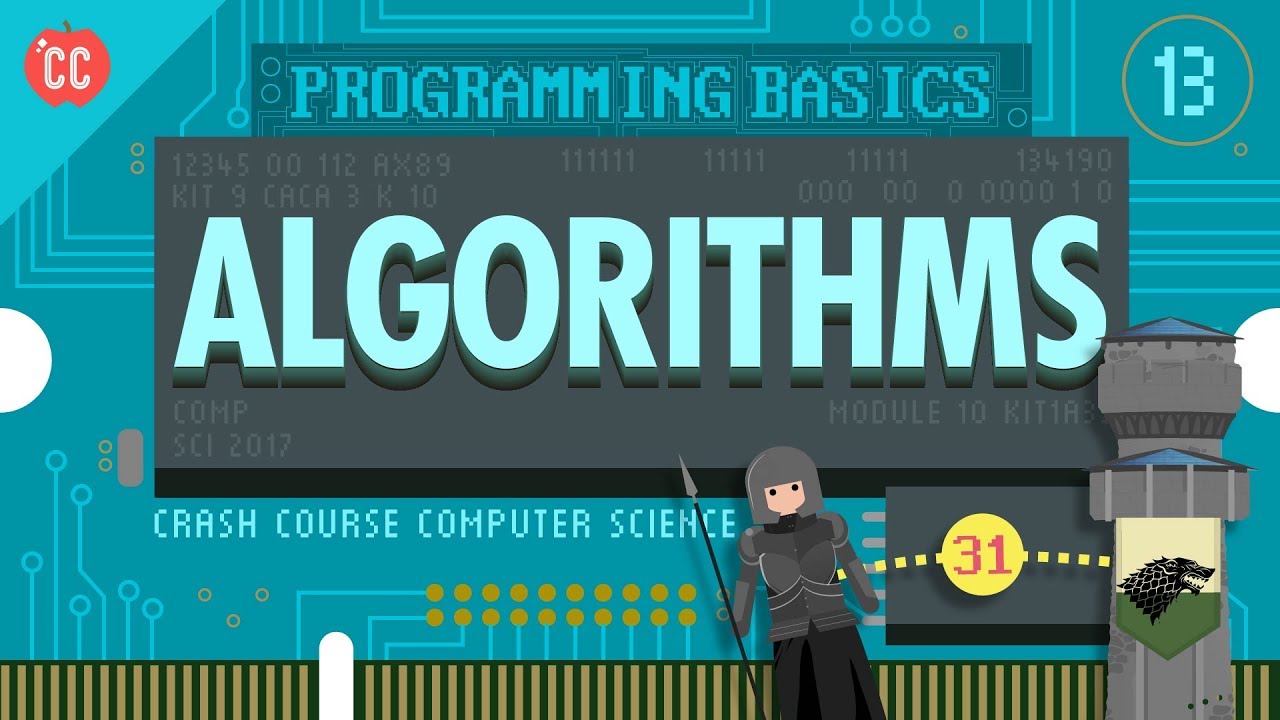
Intro to Algorithms: Crash Course Computer Science #13
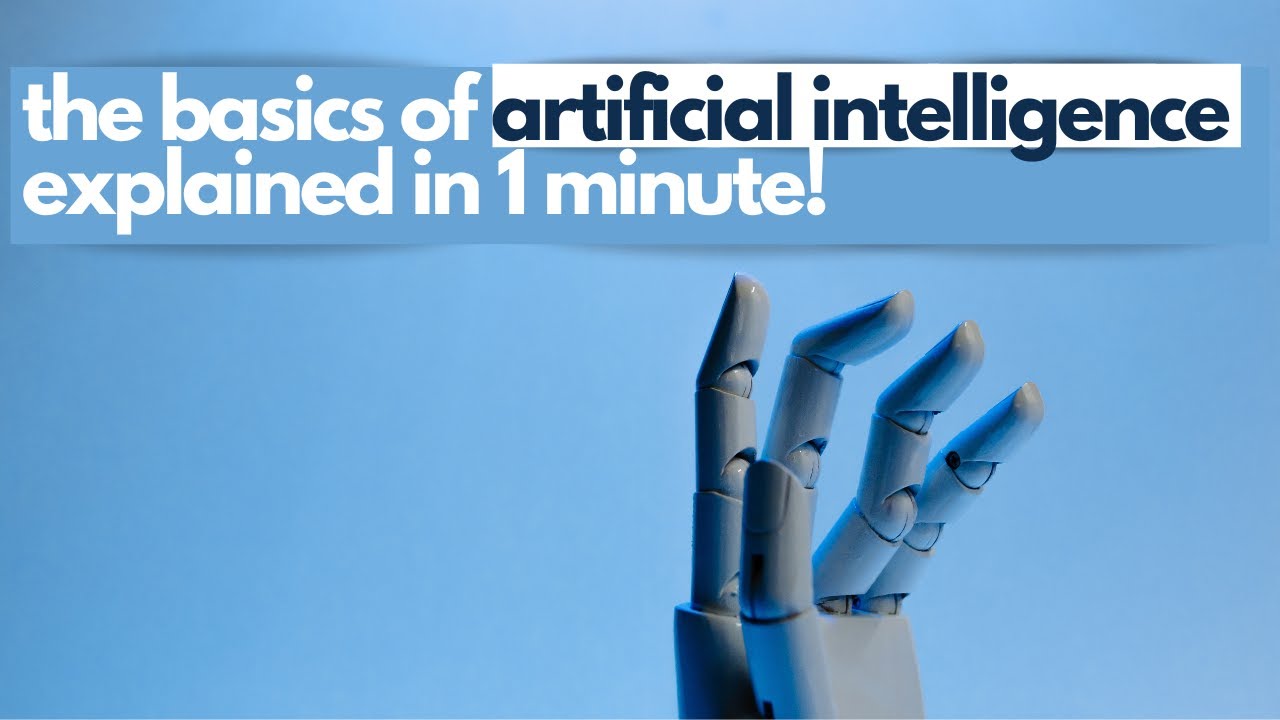
Artificial Intelligence Explained Simply in 1 Minute! ✨
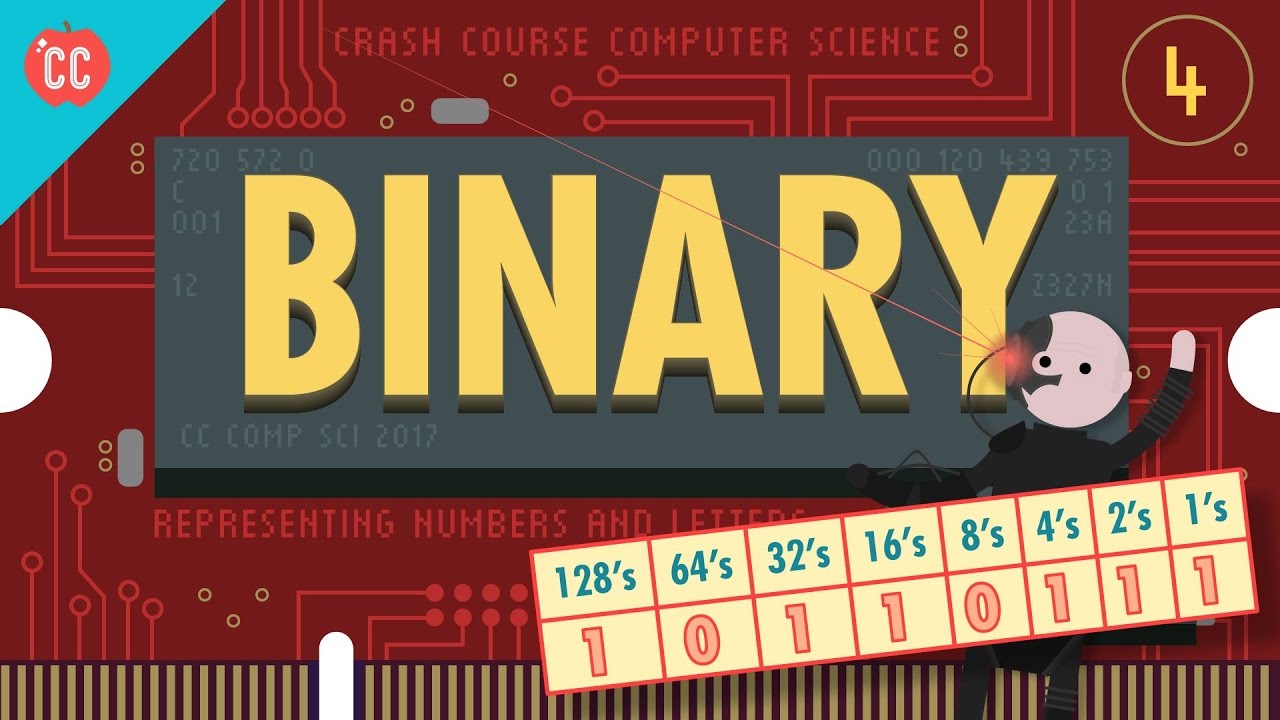
Representing Numbers and Letters with Binary: Crash Course Computer Science #4
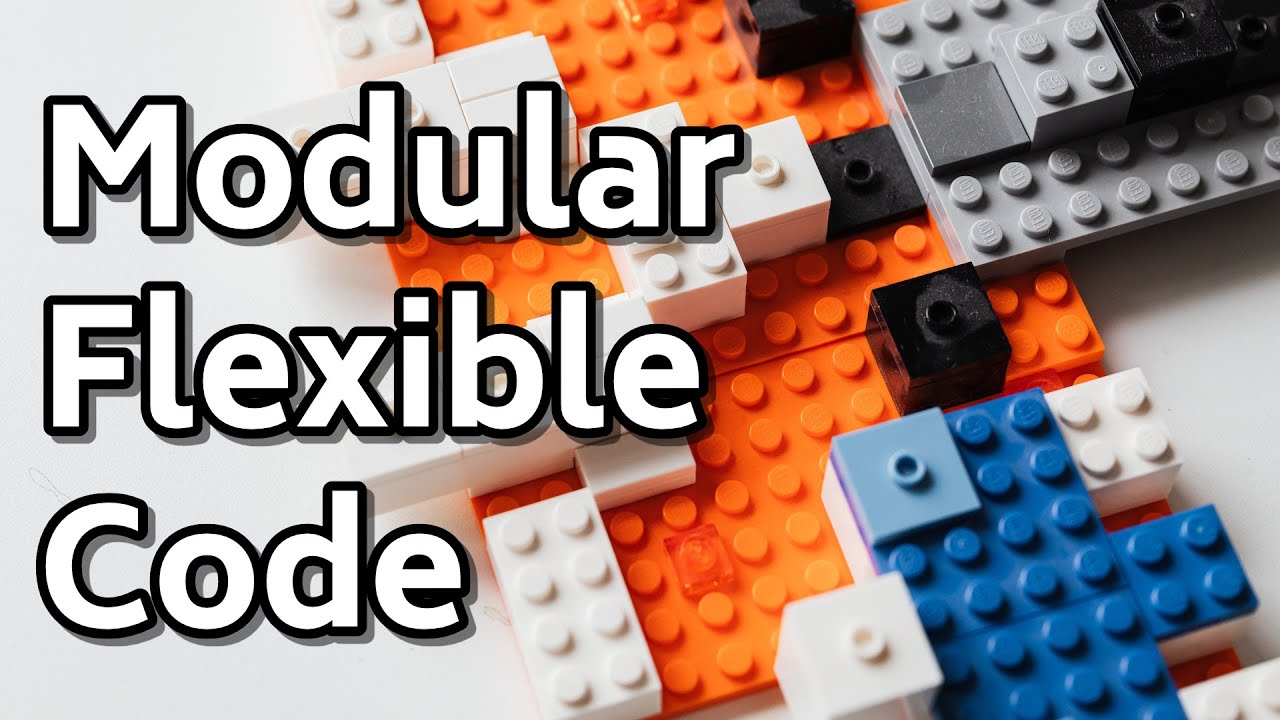
How to write more flexible game code
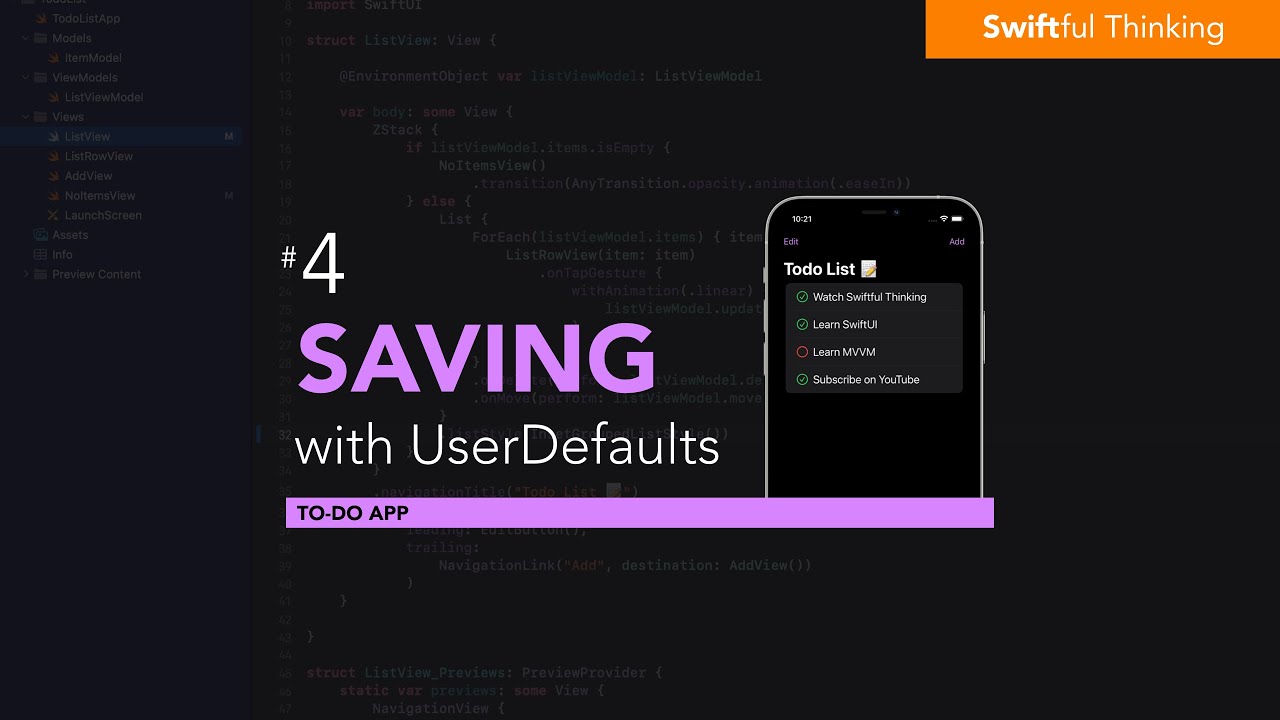
Save and persist data with UserDefaults | Todo List #4
5.0 / 5 (0 votes)