Code Snake Game in Java
Summary
TLDRThis video tutorial guides viewers through the process of coding the classic game Snake using Java. The presenter starts by showing the final game, where the snake grows by eating red food and the player controls its movement with arrow keys. The game is developed using Java's built-in Graphics Library and runs in a 600x600 pixel window. The tutorial covers creating a custom JPanel for drawing, implementing game logic with a Timer for the game loop, handling key events for snake movement, detecting food collision to grow the snake, and updating the snake's body segments. It concludes with adding game over conditions for collision with walls or the snake's body and displaying the score. The presenter also mentions their ongoing series on game development in Java and invites viewers to subscribe for updates.
Takeaways
- 🎮 The tutorial demonstrates how to code the classic game Snake using Java with a built-in Graphics Library.
- 🔑 To start the game, players can use the arrow keys to control the snake's movement.
- 🍎 The snake grows by one segment each time it touches the red food item on the screen.
- 🏁 The objective is to grow the snake's body without colliding into the walls or its own body.
- 📐 The game area is a 600x600 pixel window divided into a grid of 25x25 pixel tiles.
- 📝 The game utilizes a custom JPanel class called `SnakeGame` to handle the drawing and logic of the game.
- 🔄 A game loop with a timer is implemented to continuously update and redraw the game at set intervals.
- ⏰ The game loop runs every 100 milliseconds, causing the snake to move and the game to refresh.
- ➡️ The snake's direction is controlled by setting velocity variables for the X and Y coordinates.
- 🚫 The snake cannot move in opposite directions to prevent it from moving backward into its own body.
- 🍂 The snake's body is represented as an ArrayList of `Tile` objects, with each segment drawn as a rectangle on the screen.
- 💥 The game ends if the snake collides with the walls or its own body, and a 'Game Over' message is displayed along with the score.
Q & A
What is the final result of the tutorial?
-The final result of the tutorial is a playable game of Snake in Java. The snake moves when arrow keys are pressed, grows by one segment when it touches food, and the game ends if the snake collides with the walls or its own body.
What programming language is used to create the Snake game?
-The programming language used to create the Snake game in the tutorial is Java.
How is the game window created in the tutorial?
-The game window is created using Java's Swing library by instantiating a JFrame object, setting its title to 'Snake', making it visible, and defining its size and location.
What is the purpose of the 'tile' class in the game?
-The 'tile' class is used to keep track of the X and Y positions for each segment of the snake's body and the food in the game.
How is the snake's movement controlled in the game?
-The snake's movement is controlled by detecting key presses using the KeyListener interface and updating the snake's X and Y coordinates based on the arrow keys pressed.
How does the snake grow in the game?
-The snake grows by adding a new segment to the snake's body ArrayList when the snake's head collides with the food.
What is the game loop responsible for in the game?
-The game loop is responsible for constantly redrawing the game panel with updated positions of the snake and food, creating the illusion of movement.
How is the food placed randomly on the screen?
-The food is placed randomly on the screen by using a Random object to generate random X and Y coordinates within the bounds of the game board.
What is the tile size used in the game?
-The tile size used in the game is 25x25 pixels, which determines the movement and grid size of the game board.
How is the game over condition checked?
-The game over condition is checked by detecting if the snake's head collides with its own body or with the walls of the game board.
What happens when the snake collides with the food?
-When the snake collides with the food, the snake eats the food, its body grows by one segment, and new food appears at a random location on the screen.
How is the score displayed and incremented in the game?
-The score is displayed at the top left of the game window and is incremented by one each time the snake eats the food, which corresponds to the length of the snake's body.
Outlines
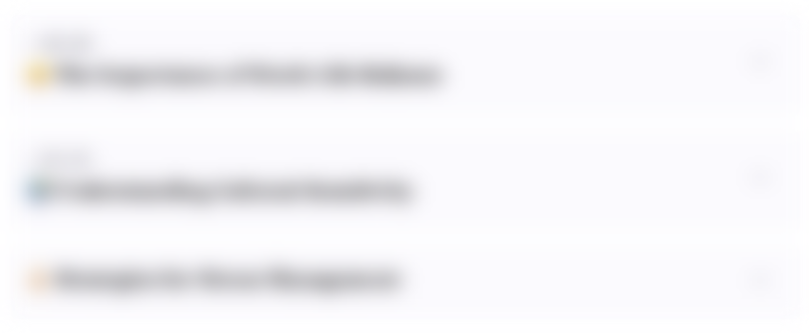
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
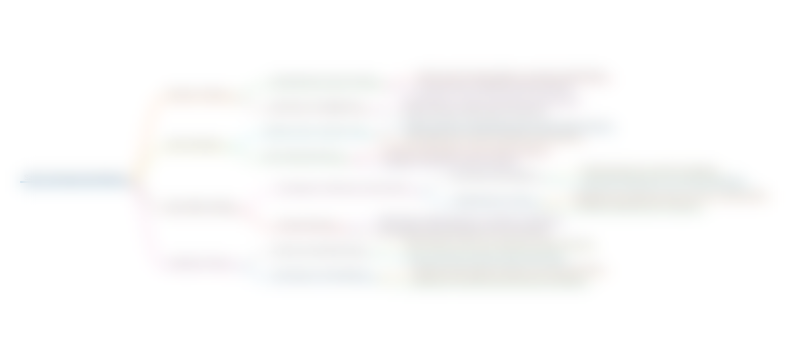
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
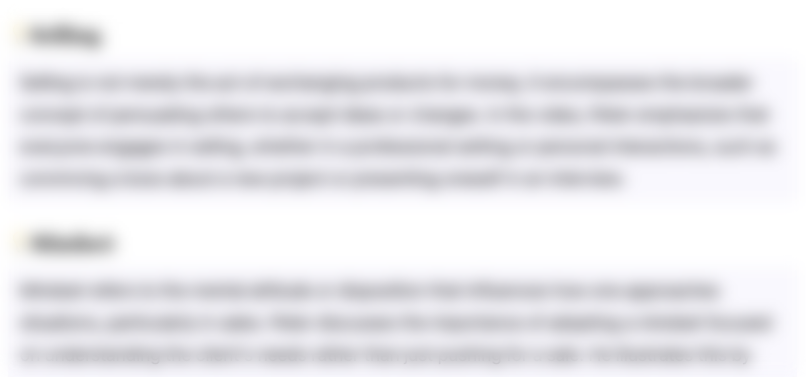
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
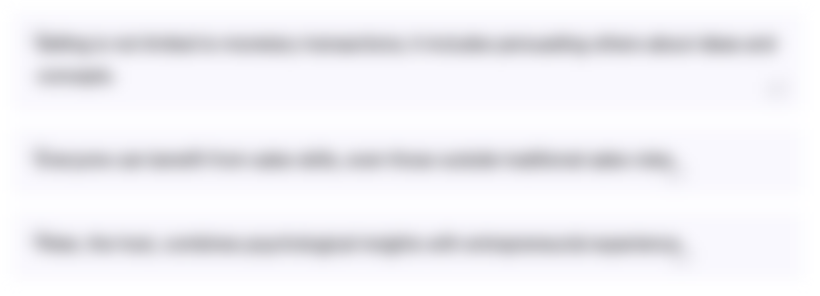
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
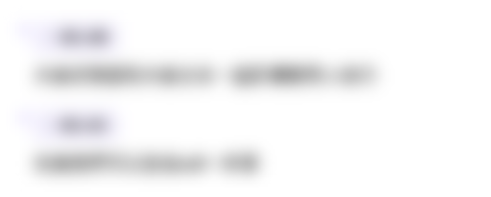
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
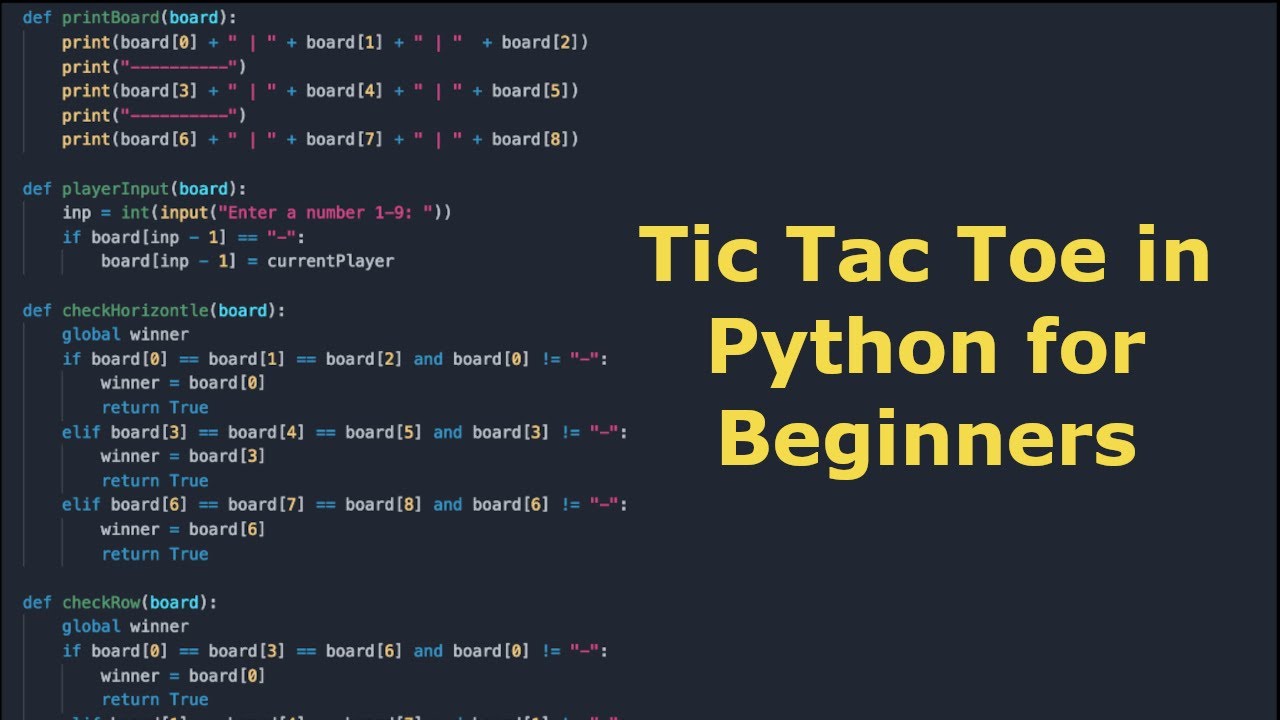
Python TIC TAC TOE Tutorial | Beginner Friendly Tutorial

How to Make a Simple and Very Easy Tic Tac Toe Game in Mit App Inventor
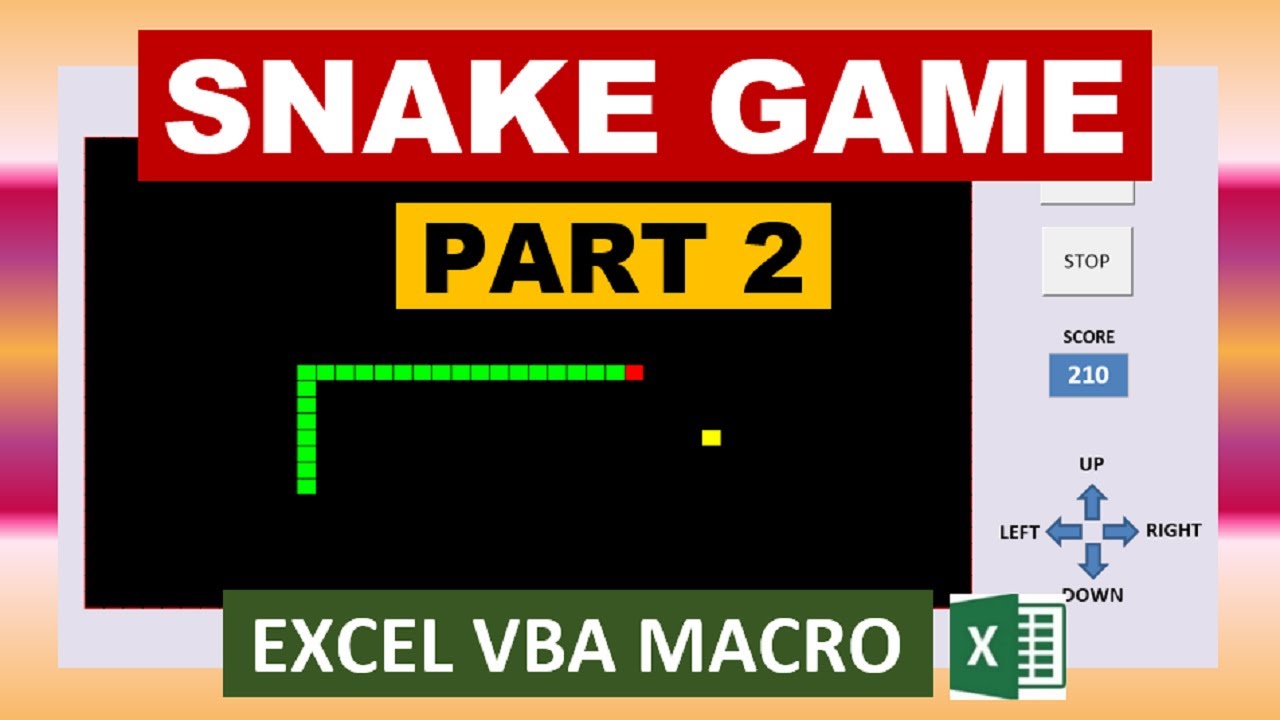
Excel Snake Game VBA Macro (Advanced) Part 2/2
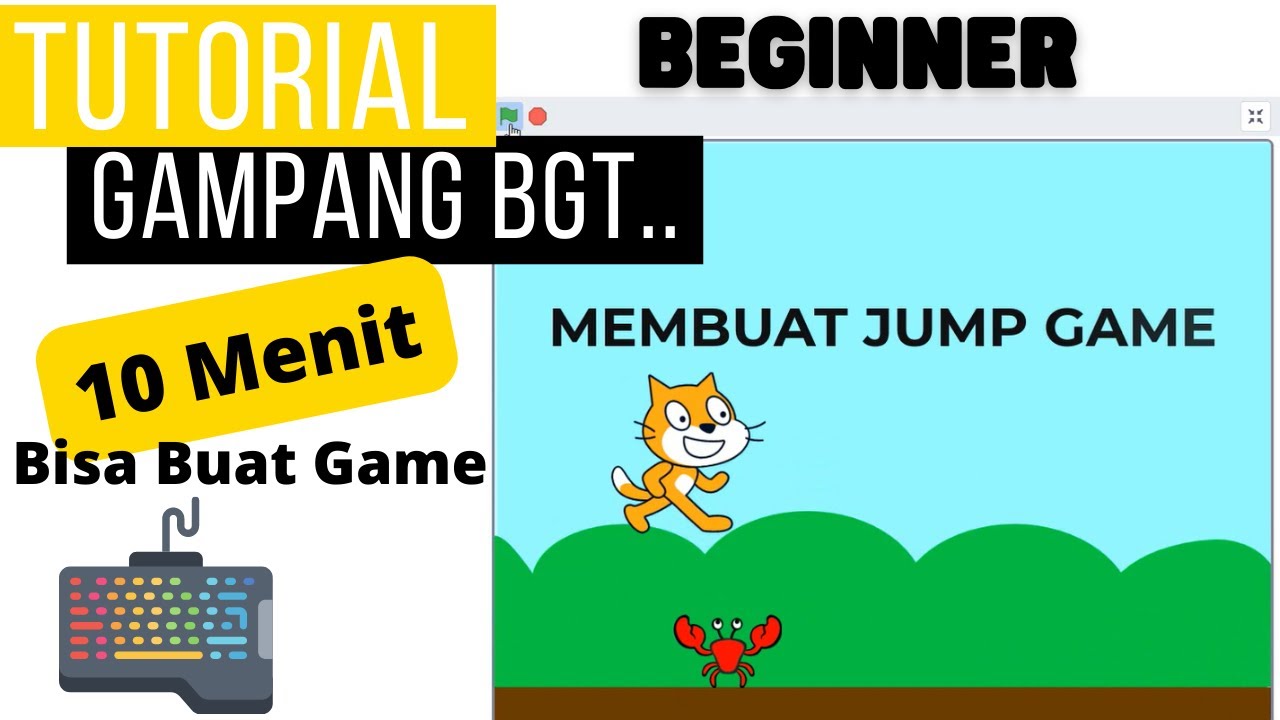
JUMP GAME DI SCRATCH | JUMPING GAME SCRATCH | MAPEL INFORMATIKA
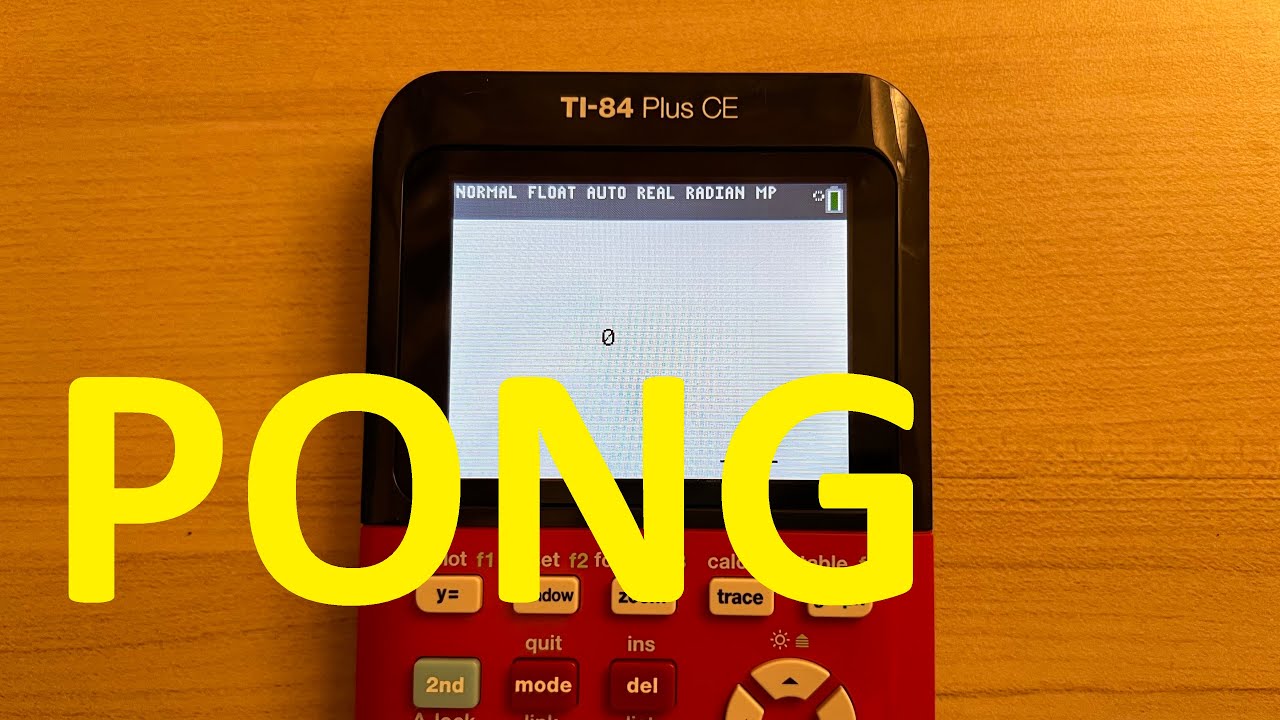
Program pong on the ti-84 plus CE
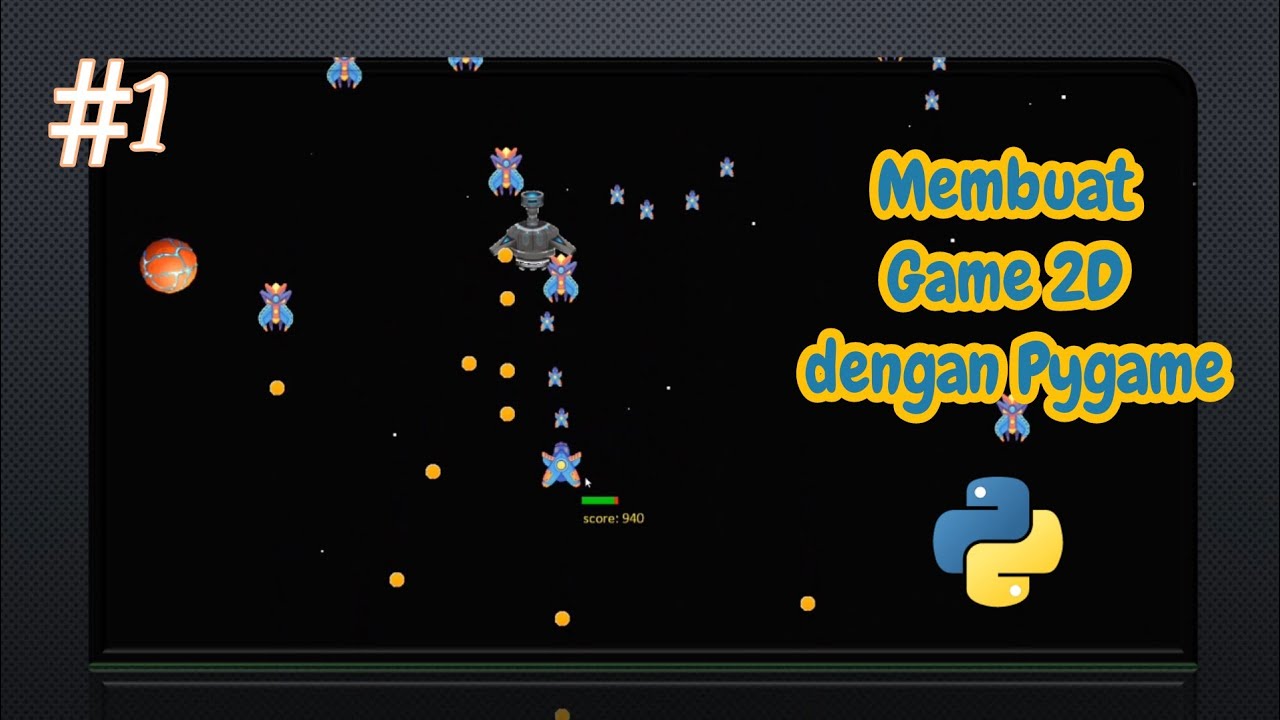
Membuat Game Arcade 2D dengan Pygame (Part 1) || Python
5.0 / 5 (0 votes)