Lesson 5-2: Alternative and Chained Conditionals
Summary
TLDRThis lecture delves into the fundamentals of conditionals in programming, focusing on if statements and their structures. It explains alternative execution through if-else statements, emphasizing the necessity of pairing else with an if condition. Additionally, it introduces chained conditionals, where multiple if-else if clauses can be evaluated, with only the first true condition executing. Through practical examples, such as determining if an integer is negative or checking for evenness, the session illustrates these concepts clearly, reinforcing the importance of understanding how to handle various conditional scenarios effectively.
Takeaways
- ๐ An if statement executes a block of code based on whether a specified condition is true or false.
- ๐ Alternative execution involves using 'if' and 'else' to provide different outcomes depending on the condition's truth value.
- ๐ The 'else' clause must be associated with an 'if' block; it cannot stand alone.
- ๐ A binary choice is made in an if-else structure, where only one branch of code will execute at a time.
- ๐ The syntax for an if-else statement includes an 'if' condition followed by the 'else' block without an additional condition.
- ๐ When using an if-else statement, at least one conditional statement is required within the block; if none are present, 'pass' can be used to avoid errors.
- ๐ Chained conditionals can be structured using 'if', 'elif', and 'else' to evaluate multiple conditions sequentially.
- ๐ Only the first true condition in a chain will execute; subsequent conditions are ignored.
- ๐ The modulus operator (%) is useful for checking evenness and oddness of numbers within conditional statements.
- ๐ Practicing writing and testing conditional statements helps solidify understanding of flow control in programming.
Q & A
What is the primary focus of the lecture?
-The lecture focuses on conditionals, specifically the use of 'if' statements, alternative execution, and chain conditionals in programming.
How does alternative execution work in an if-else statement?
-In alternative execution, if a specified condition is met, the code within the 'if' block executes. If the condition is not met, the code within the 'else' block executes.
What is the importance of the Boolean expression in if statements?
-The Boolean expression determines whether the condition is true or false, guiding the execution flow of the code based on its evaluation.
Can 'else' exist independently without an 'if' statement?
-No, 'else' cannot stand alone; it must be associated with an 'if' statement to make logical sense.
What does the term 'chain conditionals' refer to?
-Chain conditionals refer to a series of 'if' and 'elif' statements where multiple conditions are checked sequentially. The first condition that evaluates to true will execute its corresponding block of code.
How do you determine if a number is even or odd using conditionals?
-You can determine if a number is even by using the modulus operator. If the remainder of the number divided by 2 is zero, the number is even; otherwise, it is odd.
What will happen if multiple conditions in a chain conditional are true?
-If multiple conditions are true, only the first condition that evaluates to true will execute, and subsequent conditions will be skipped.
What is the output of the code when an integer less than zero is input?
-If an integer less than zero is input, the program will print that the number is negative and then compute and print its square, confirming that the square is positive.
What is the significance of the 'pass' statement in conditional blocks?
-The 'pass' statement can be used in conditional blocks when no action is required, allowing the code to skip over the block without raising an error.
In what scenario would none of the branches in an if-elif statement execute?
-None of the branches would execute if all the conditions in the if-elif statement evaluate to false.
Outlines
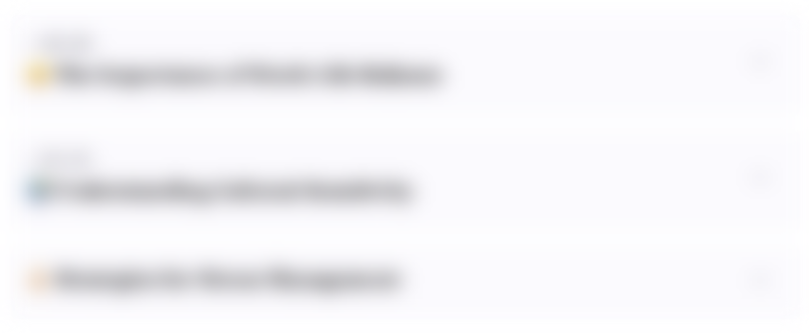
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
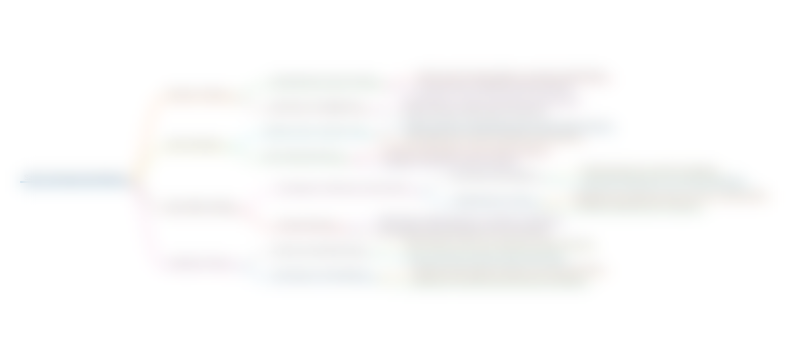
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
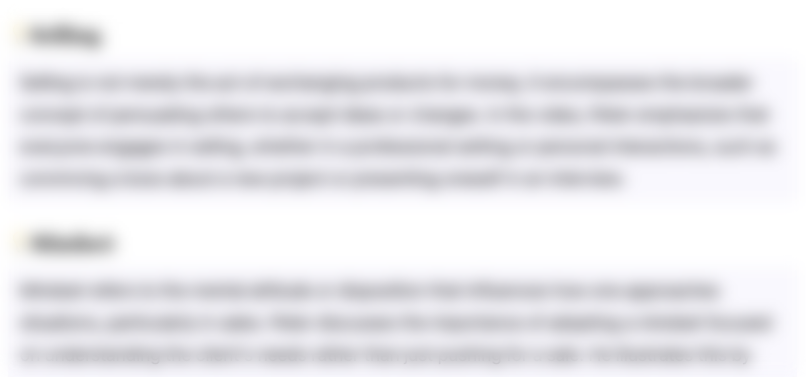
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
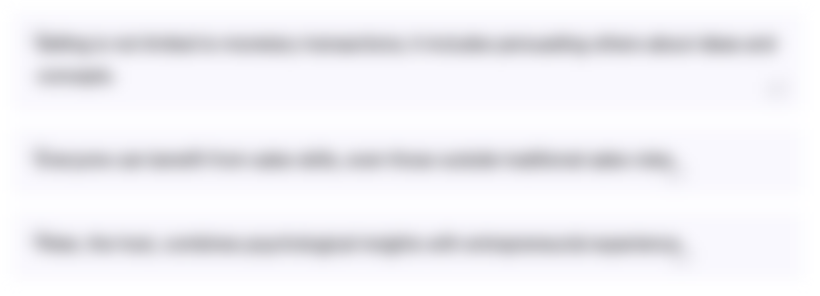
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
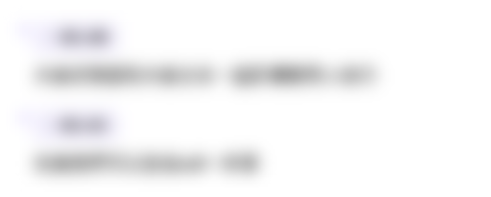
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

CSP: Conditionals pt 2B - if/else statements

Karel Python - if/else

Percabangan If dan Switch Case
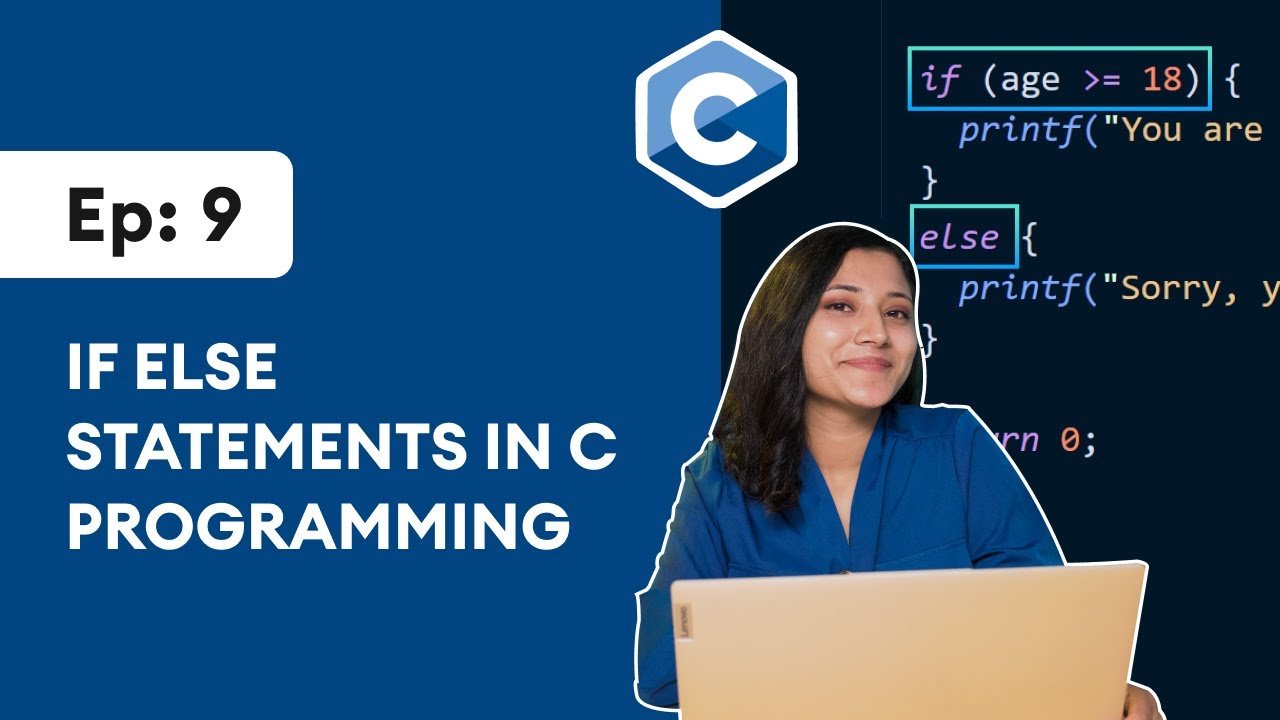
#9: If Else Statements in C | C Programming for Beginners
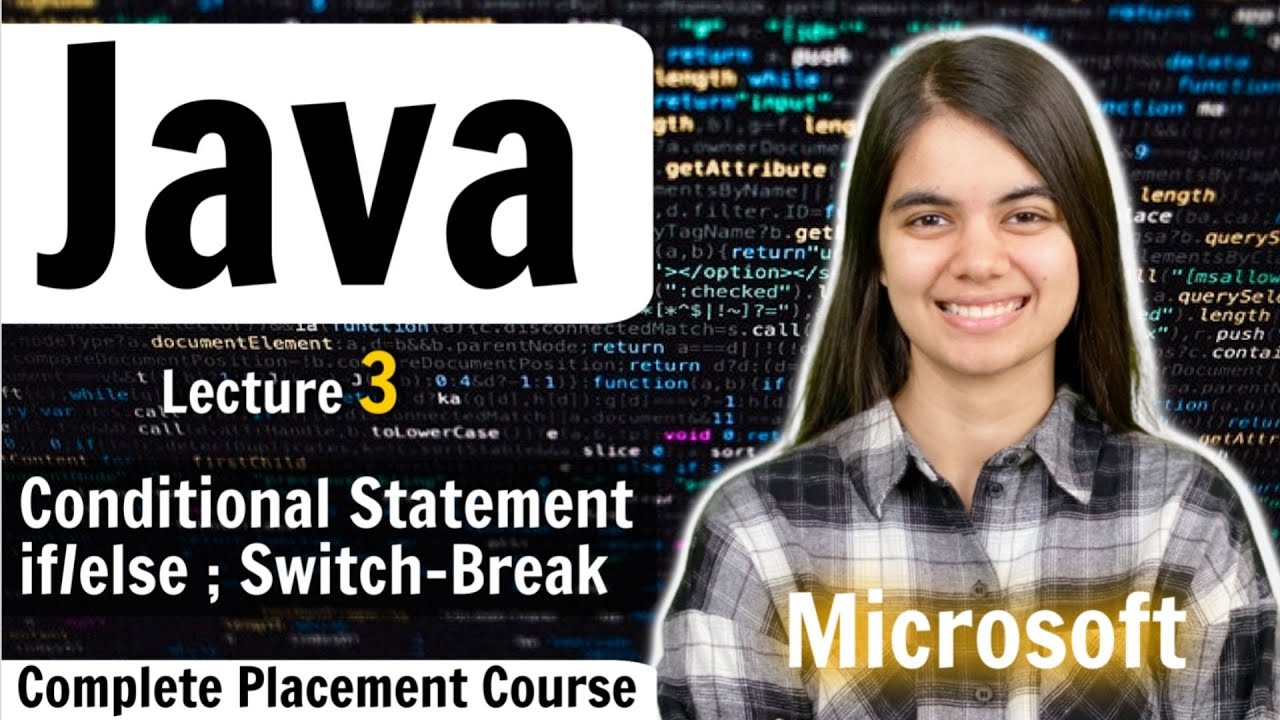
Conditional Statements | If-else, Switch Break | Complete Java Placement Course | Lecture 3
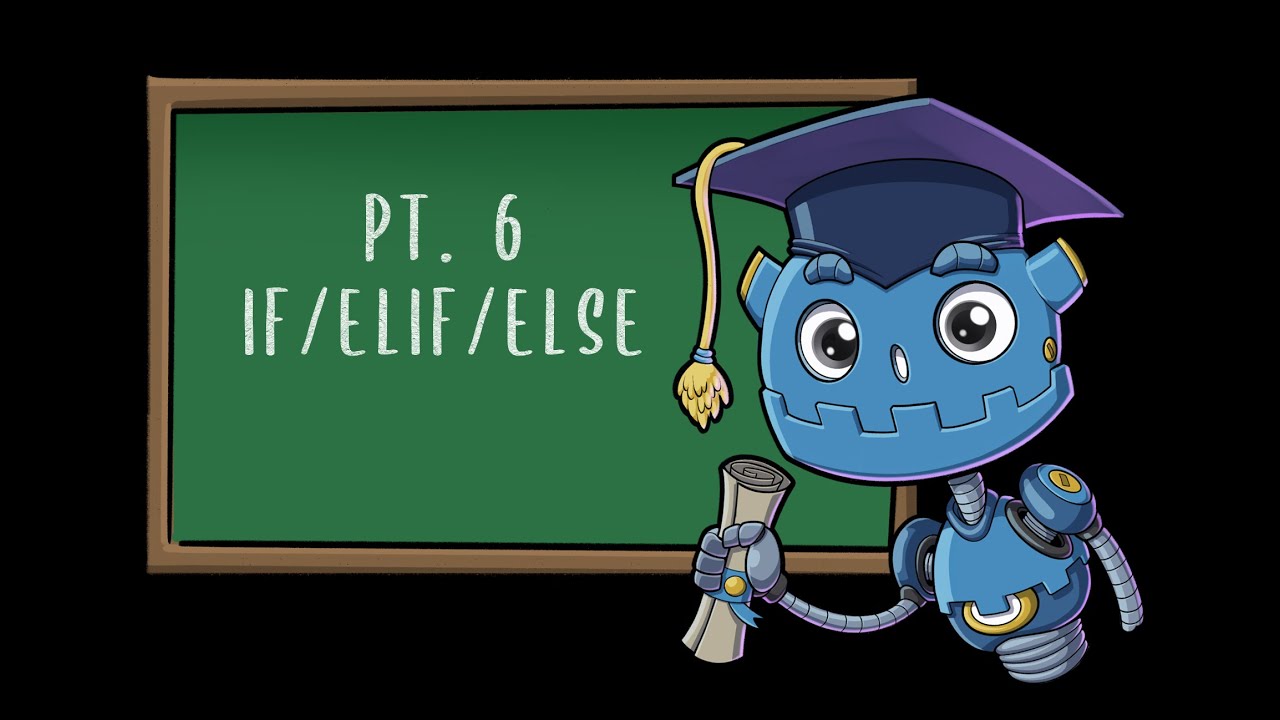
If/Elif/Else Statement Chain | Godot GDScript Tutorial | Ep 06
5.0 / 5 (0 votes)