Flutter Tutorial for Beginners #25 - Asynchronous Code
Summary
TLDRThis video provides a comprehensive introduction to asynchronous programming in Flutter, paralleling it with JavaScript's promises and async/await. It explains the concept of non-blocking code, demonstrating how to simulate network requests with delays using `Future.delayed`. Key functions such as `getData` illustrate how to handle multiple asynchronous requests, emphasizing the importance of using the `await` keyword to ensure that dependent requests are completed in the correct order. The video concludes with a preview of upcoming lessons focused on utilizing Flutter packages for making network requests, preparing viewers for practical applications in future projects.
Takeaways
- 😀 Asynchronous code allows actions to start now and finish in the future, making it essential for operations like API interactions.
- 😀 In Flutter, handling asynchronous code involves using async functions, the await keyword, and futures, similar to promises in JavaScript.
- 😀 The Future.delayed method simulates network requests by creating a delay, allowing the code to run non-blockingly while waiting for data.
- 😀 Non-blocking code means that other parts of the application can continue executing while waiting for an asynchronous task to complete.
- 😀 If a second asynchronous request depends on the first, you can use the await keyword to pause execution until the first request is complete.
- 😀 By declaring a function as async, you can use await to wait for future values, ensuring sequential execution of dependent requests.
- 😀 Values returned from asynchronous requests can be stored in variables, allowing them to be used later in the code.
- 😀 It's possible to have asynchronous calls that don't block the execution of subsequent code, which can run independently.
- 😀 Understanding asynchronous programming is crucial for working with third-party APIs and making effective network requests.
- 😀 Future lessons will delve into using Flutter packages to facilitate network requests and improve code functionality.
Q & A
What is asynchronous code?
-Asynchronous code allows an action to start and finish later, enabling other code to run in the meantime. It is particularly useful for tasks like interacting with APIs or databases that may take time to complete.
How does asynchronous code behave in Flutter?
-In Flutter, asynchronous code is non-blocking. While a request is being processed, the rest of the code continues to execute without waiting for the request to finish.
What are Futures in Dart?
-Futures are a type of data in Dart that represent a value that will be available at some point in the future, similar to promises in JavaScript.
How do async and await keywords work in Dart?
-The 'async' keyword indicates that a function contains asynchronous code, while 'await' is used to pause the execution until the awaited Future completes, allowing the function to return a value.
What is the purpose of the Future.delayed function?
-The Future.delayed function is used to simulate a delay in asynchronous operations, specifying a duration to wait before executing a callback function.
How can you handle dependent asynchronous requests in Flutter?
-To handle dependent requests, you can declare your function as 'async' and use 'await' before calling the first request. This ensures that the second request waits for the first one to complete.
What happens if you don’t use await for a dependent request?
-If you don't use 'await' for a dependent request, the second request may start before the first request completes, leading to incorrect or unavailable data.
How can you return values from asynchronous functions in Dart?
-You can return values from asynchronous functions by returning a value instead of printing it directly. Use 'await' to retrieve the value when calling the function.
What is the benefit of non-blocking code in applications?
-Non-blocking code improves application responsiveness by allowing other code to execute while waiting for asynchronous operations to complete, enhancing user experience.
What will the upcoming lessons cover regarding asynchronous operations in Flutter?
-The upcoming lessons will focus on using Flutter packages that facilitate making network requests and working with third-party APIs.
Outlines
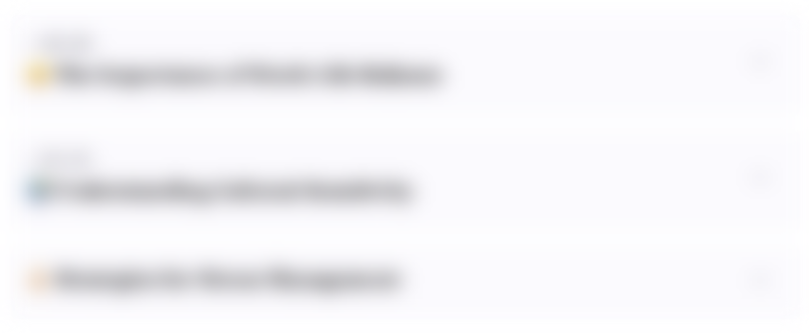
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
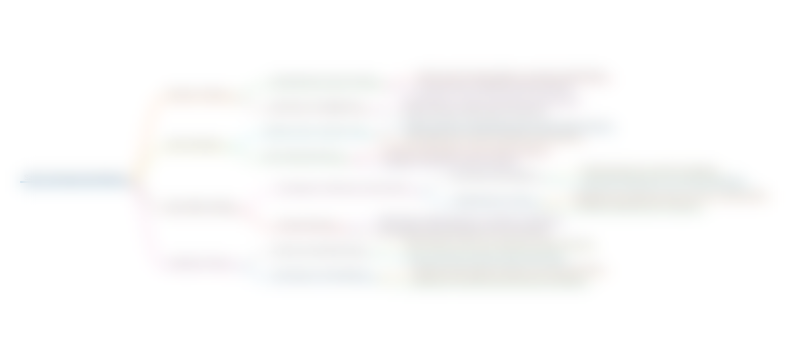
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
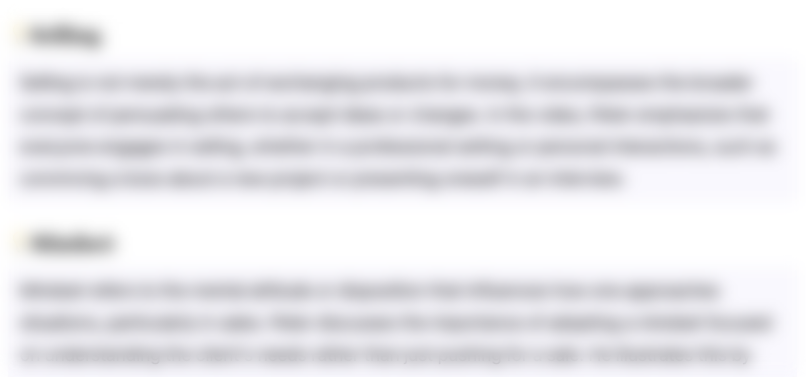
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
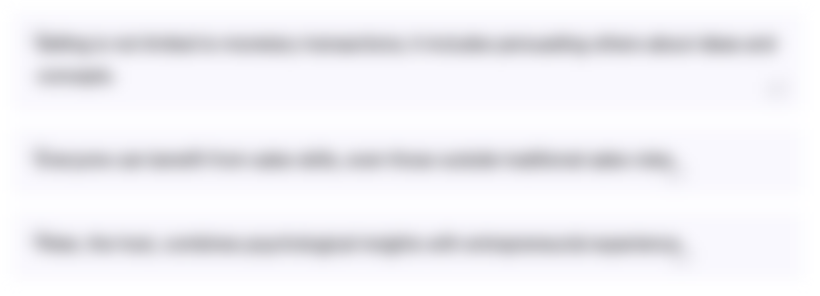
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
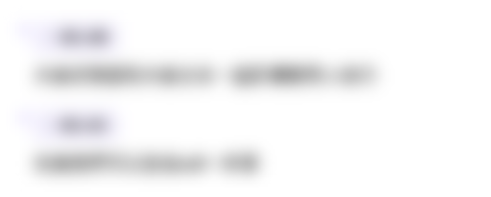
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
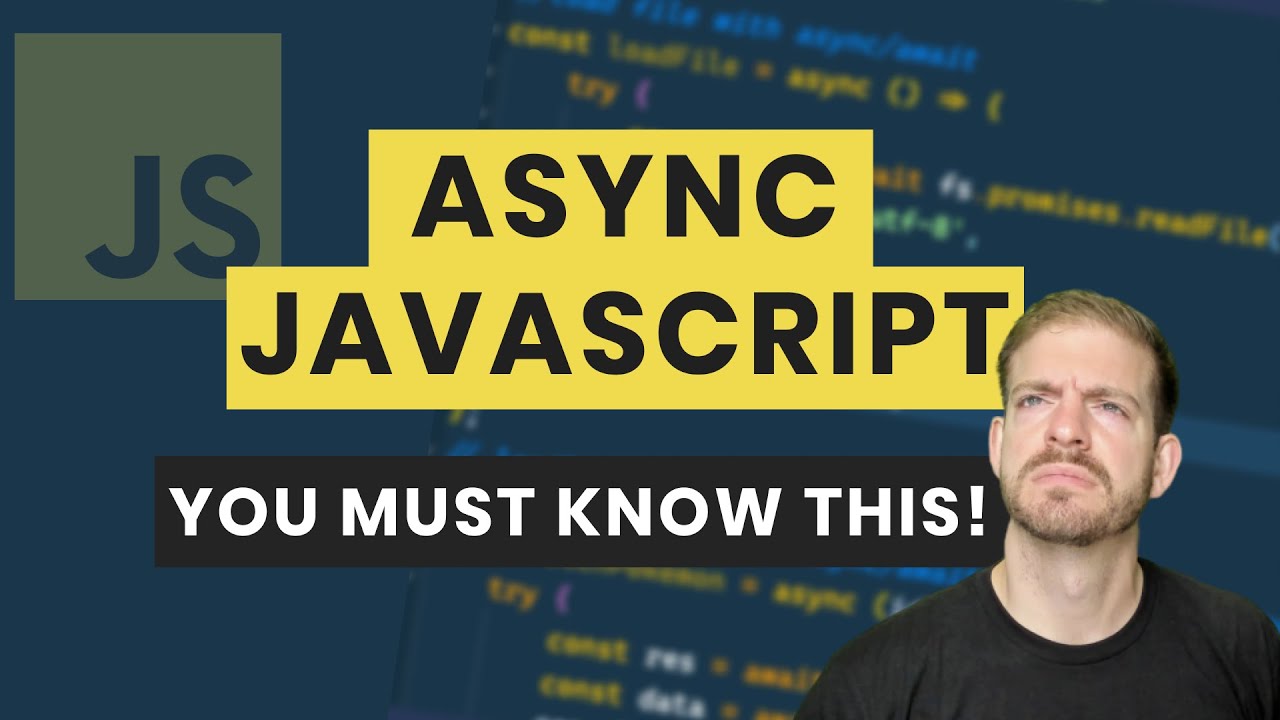
Asynchronous JavaScript in ~10 Minutes - Callbacks, Promises, and Async/Await
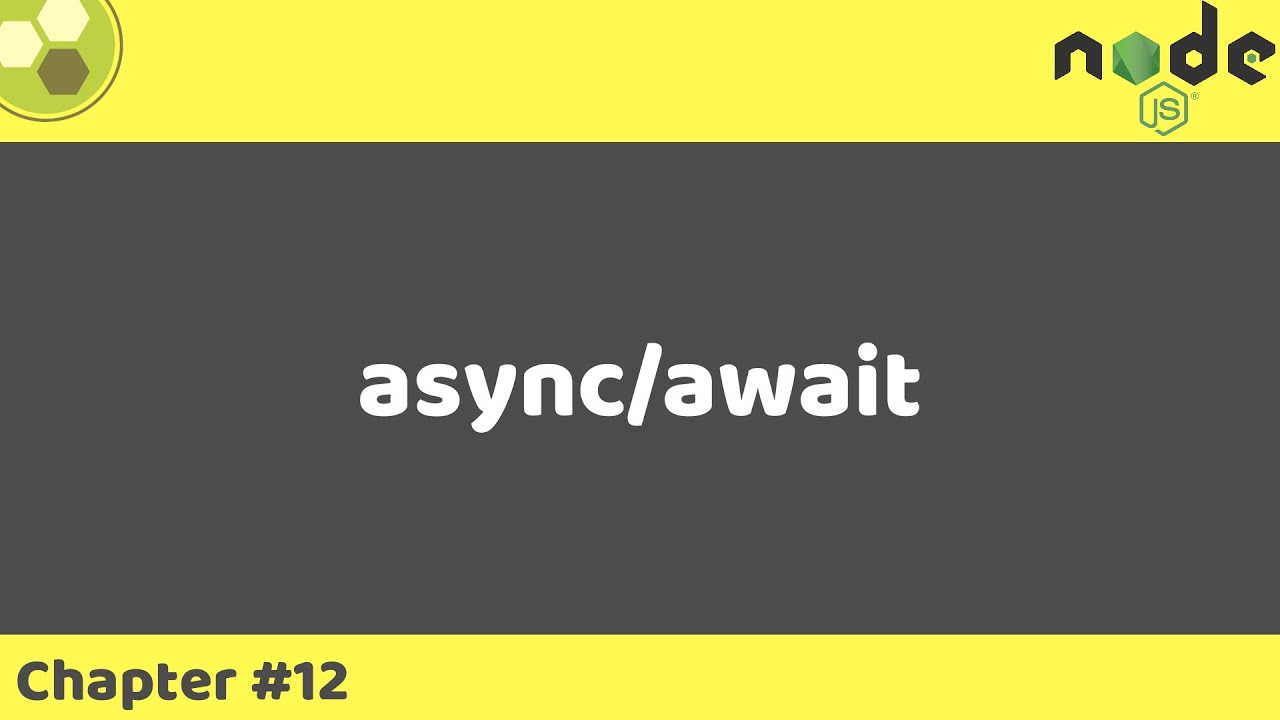
Node.js Tutorial #12 | async/await
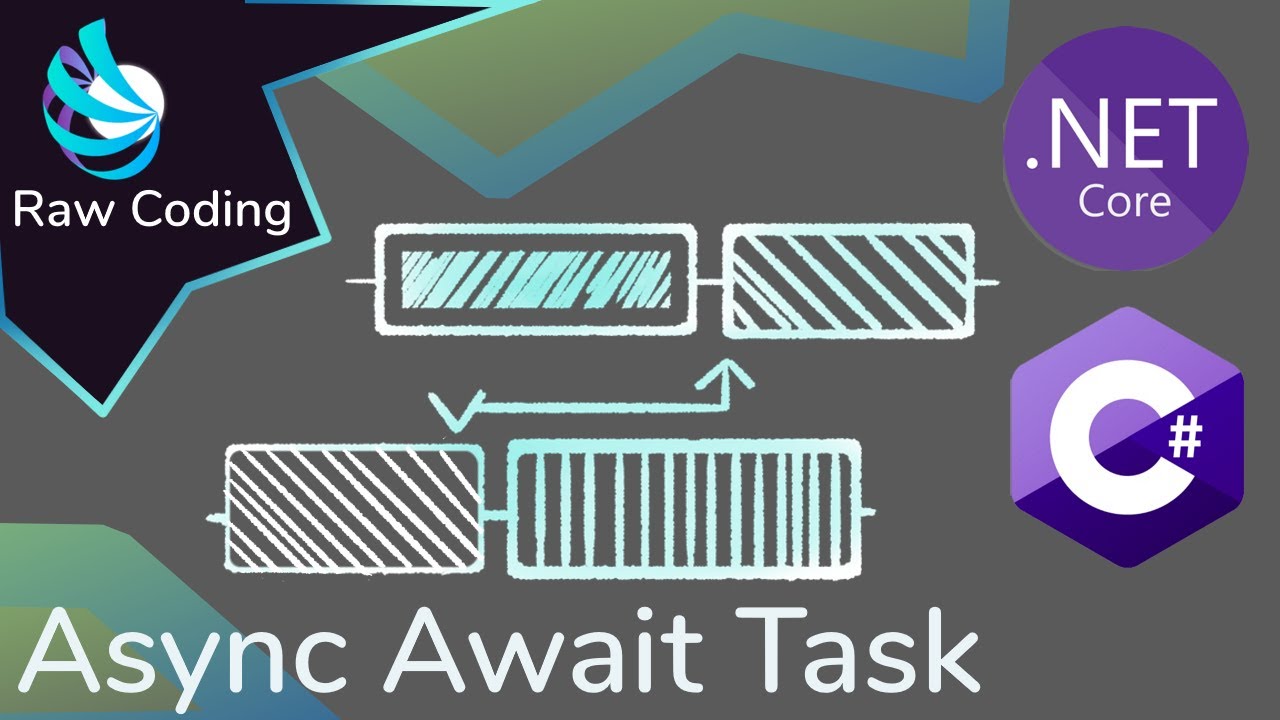
C# Async/Await/Task Explained (Deep Dive)
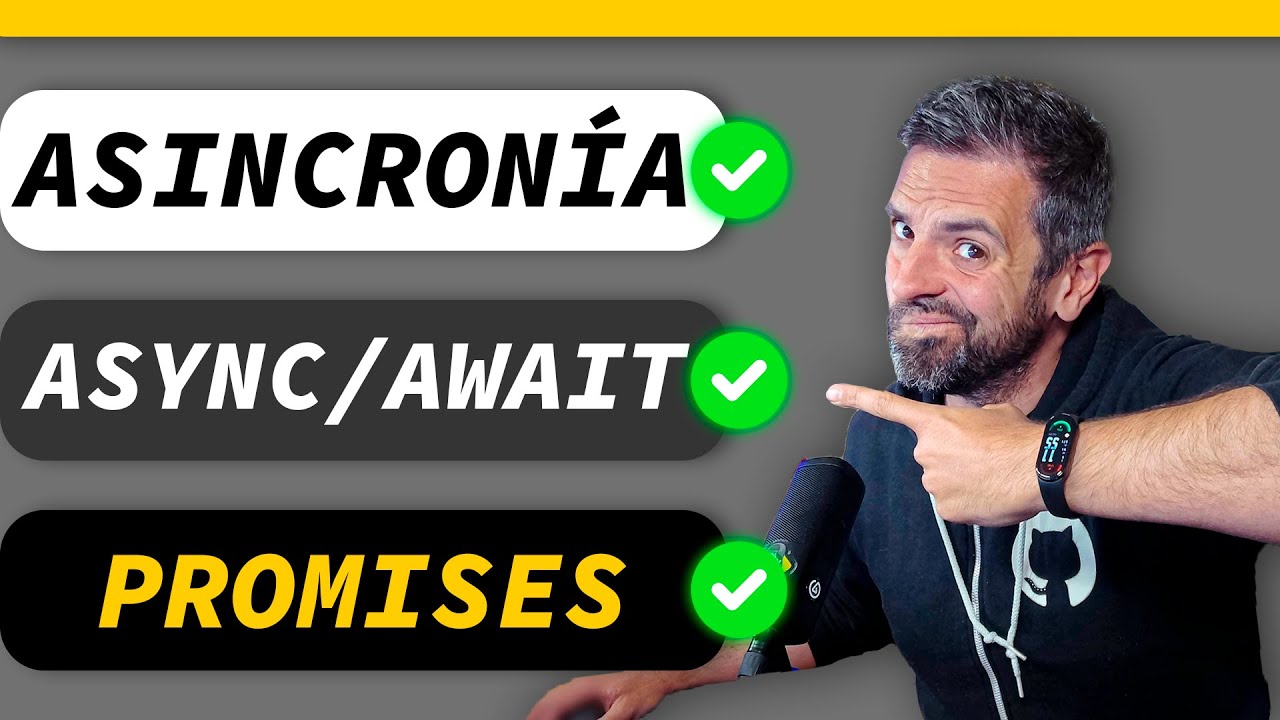
Asincronía en JavaScript: Todo lo que necesitas saber
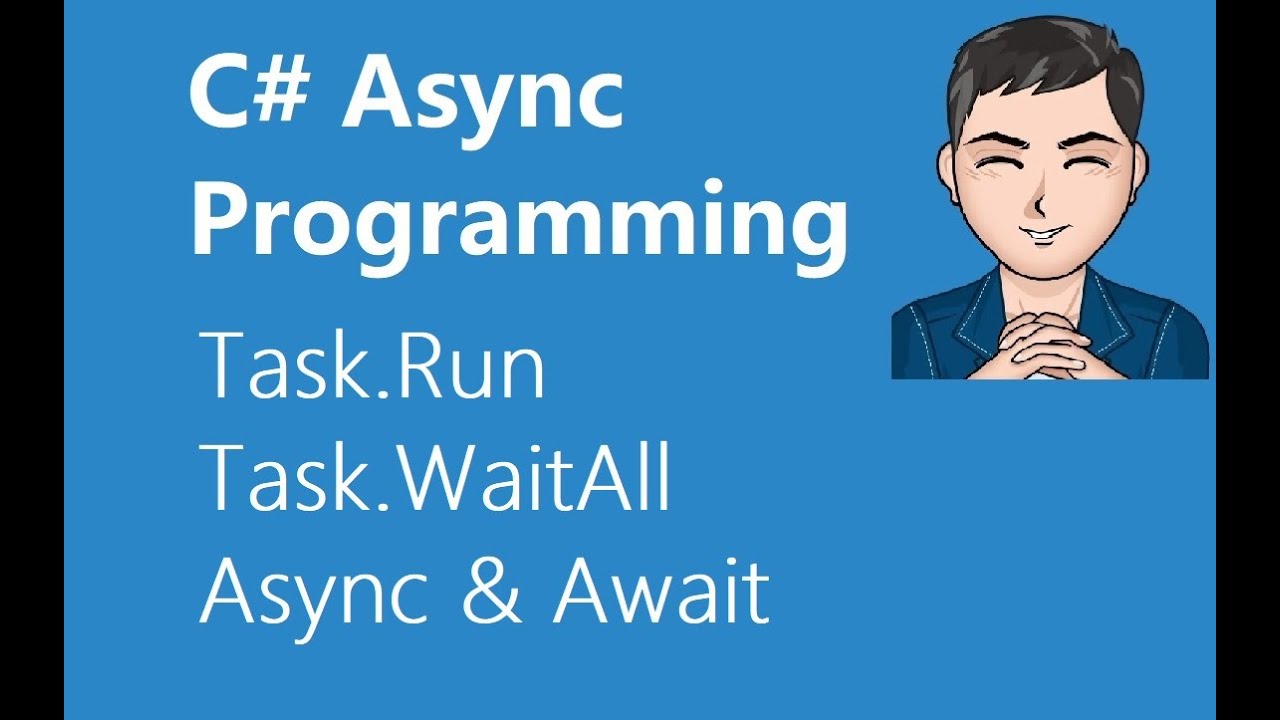
Asynchronous Programming in C# Explained (Task.Run, Task.WaitAll, Async and Await)
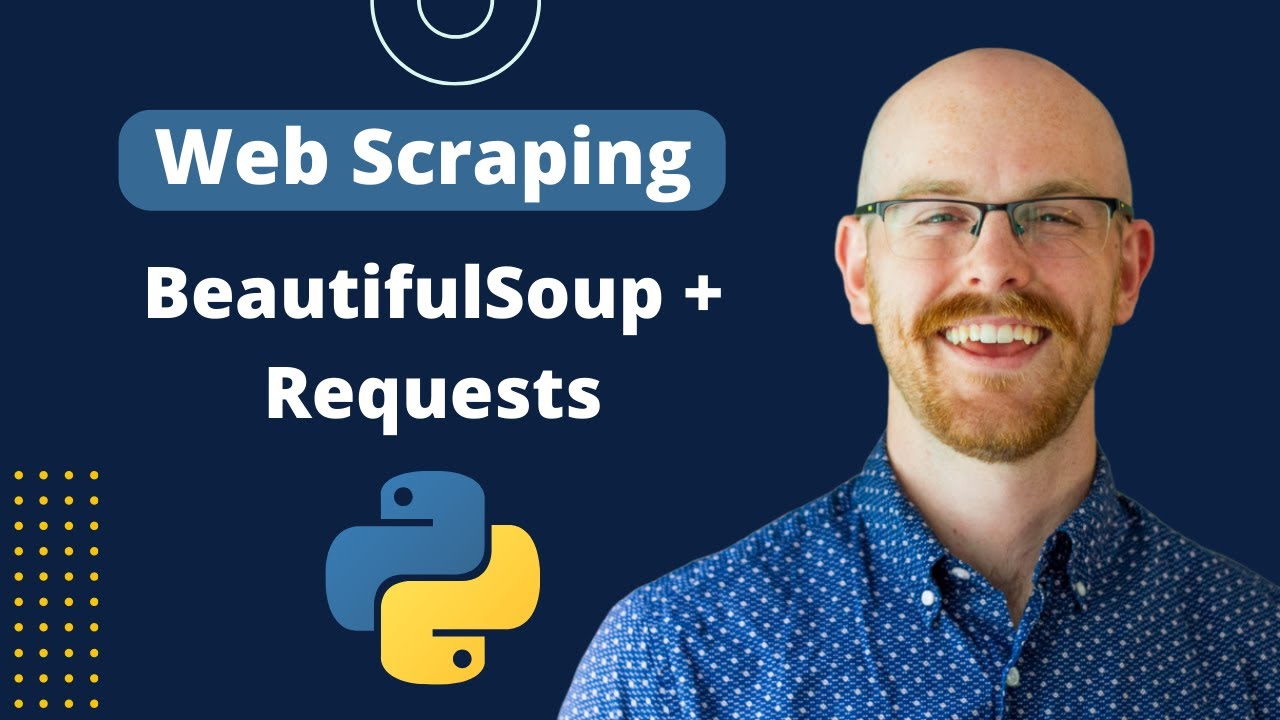
BeautifulSoup + Requests | Web Scraping in Python
5.0 / 5 (0 votes)