Node.js Tutorial #12 | async/await
Summary
TLDRThe video discusses asynchronous processes in JavaScript, focusing on the concept of promises. It explains how async/await simplifies handling asynchronous operations, making code more readable and easier to manage compared to traditional callbacks. The transcript includes practical examples, demonstrating how to return and handle promises effectively, and highlights the importance of managing errors and results in asynchronous functions. Additionally, it emphasizes the flexibility of async functions, allowing for clearer code organization and better control over asynchronous behavior.
Takeaways
- π Asynchronous processes simplify handling multiple tasks without blocking the main execution thread.
- π Promises are an easier way to manage asynchronous operations, making code more readable and maintainable.
- π Functions that return promises can be utilized to handle results of asynchronous calls effectively.
- π To work with promises, it's essential to understand how to use `.then()` and `.catch()` methods for handling resolved and rejected cases.
- π You do not need to write a return statement in functions that are asynchronous when they return a promise.
- π Proper error handling with promises allows developers to avoid complications in the execution flow.
- π Understanding the concept of 'await' allows for cleaner syntax in asynchronous code by pausing execution until a promise is settled.
- π Avoiding nested callbacks can be achieved through the use of promises, leading to more straightforward code structures.
- π It's crucial to understand how to test asynchronous functions to ensure they return the expected results.
- π Asynchronous functions can significantly enhance performance, especially when dealing with I/O operations or API requests.
Q & A
What is a promise in JavaScript?
-A promise is an object that represents the eventual completion or failure of an asynchronous operation and its resulting value.
How do you create a promise?
-You create a promise using the `Promise` constructor, which takes a function (executor) with two parameters: `resolve` and `reject`.
What are the two primary methods to handle a promise?
-You can use `.then()` to handle successful resolution and `.catch()` to handle errors.
What is the purpose of the `async` keyword in a function?
-The `async` keyword allows you to use `await` within a function, pausing the execution until the promise resolves.
How does the `await` keyword work?
-`await` pauses the function execution until the promise settles and returns the resolved value, or throws an error if rejected.
What does `Promise.all()` do?
-`Promise.all()` takes an array of promises and resolves when all the promises in the array have completed successfully.
Can promises be chained, and if so, how?
-Yes, promises can be chained. Each `.then()` call returns a new promise, allowing you to perform further actions based on the result of the previous promise.
What is the difference between synchronous and asynchronous functions?
-Synchronous functions execute sequentially, blocking further execution until they complete, while asynchronous functions allow other operations to continue while waiting for an operation to complete.
What happens if a promise is rejected?
-If a promise is rejected, the control goes to the nearest `.catch()` block in the promise chain, where you can handle the error.
How can you manage multiple asynchronous operations in JavaScript?
-You can manage multiple asynchronous operations using `Promise.all()`, `Promise.race()`, or by chaining promises to execute them in sequence.
Outlines
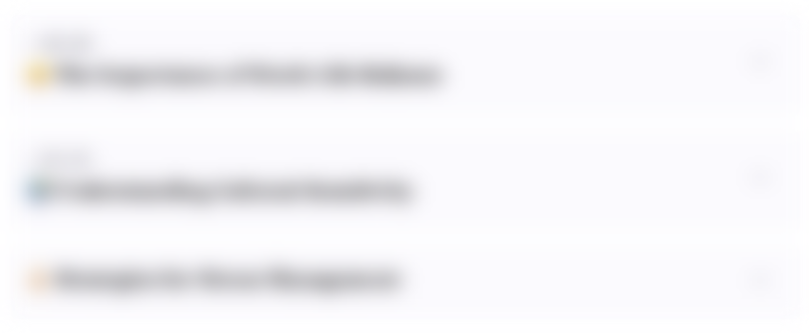
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
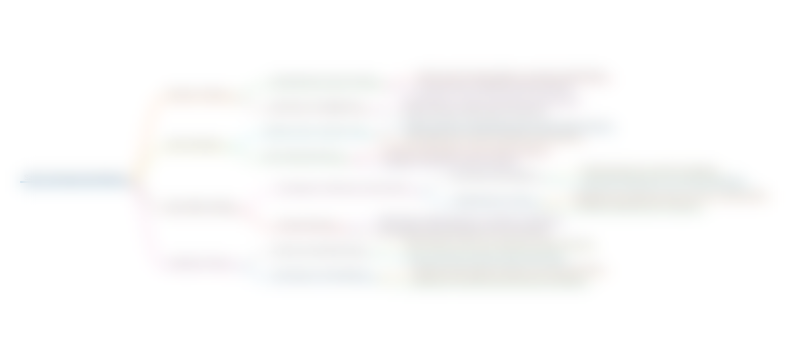
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
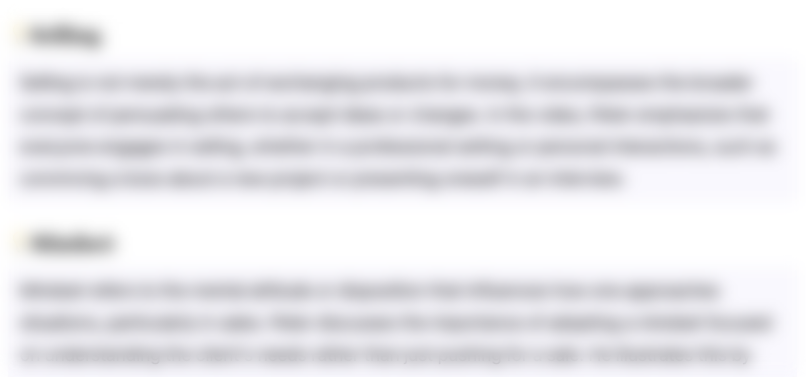
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
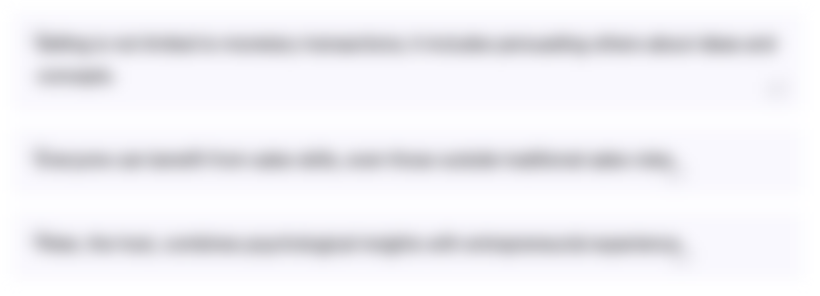
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
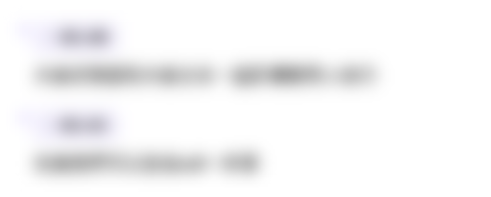
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
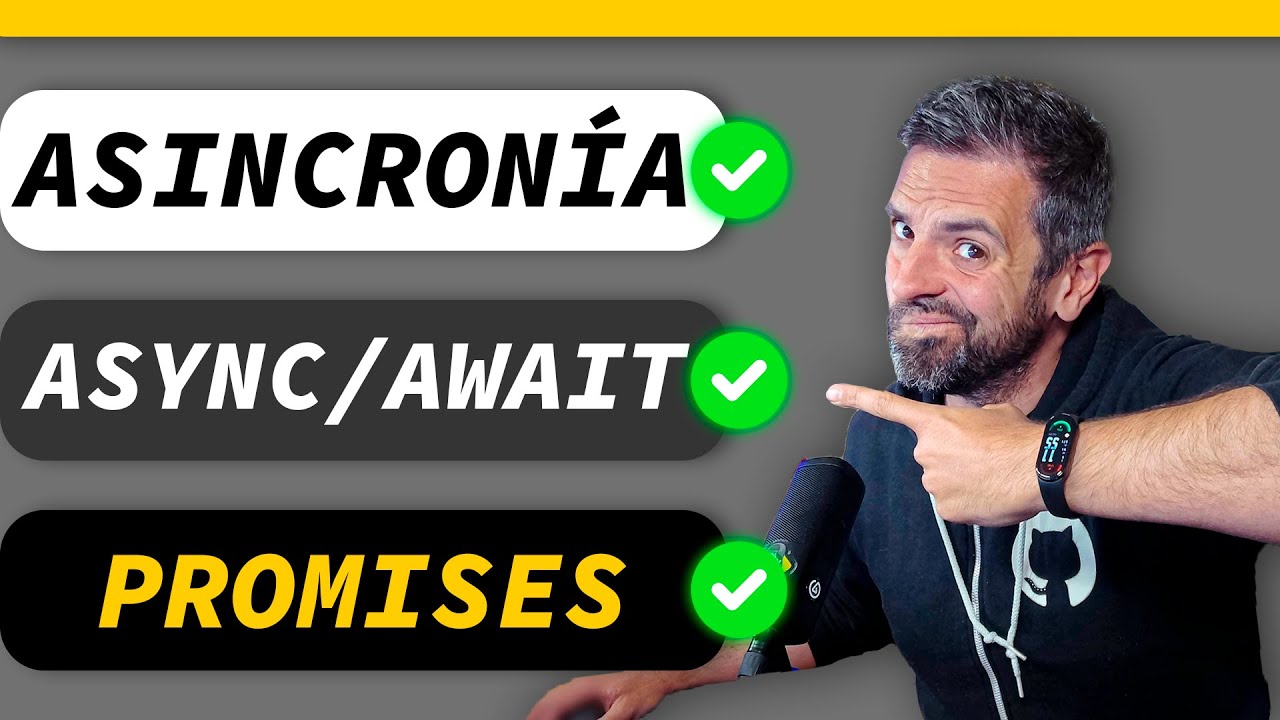
AsincronΓa en JavaScript: Todo lo que necesitas saber
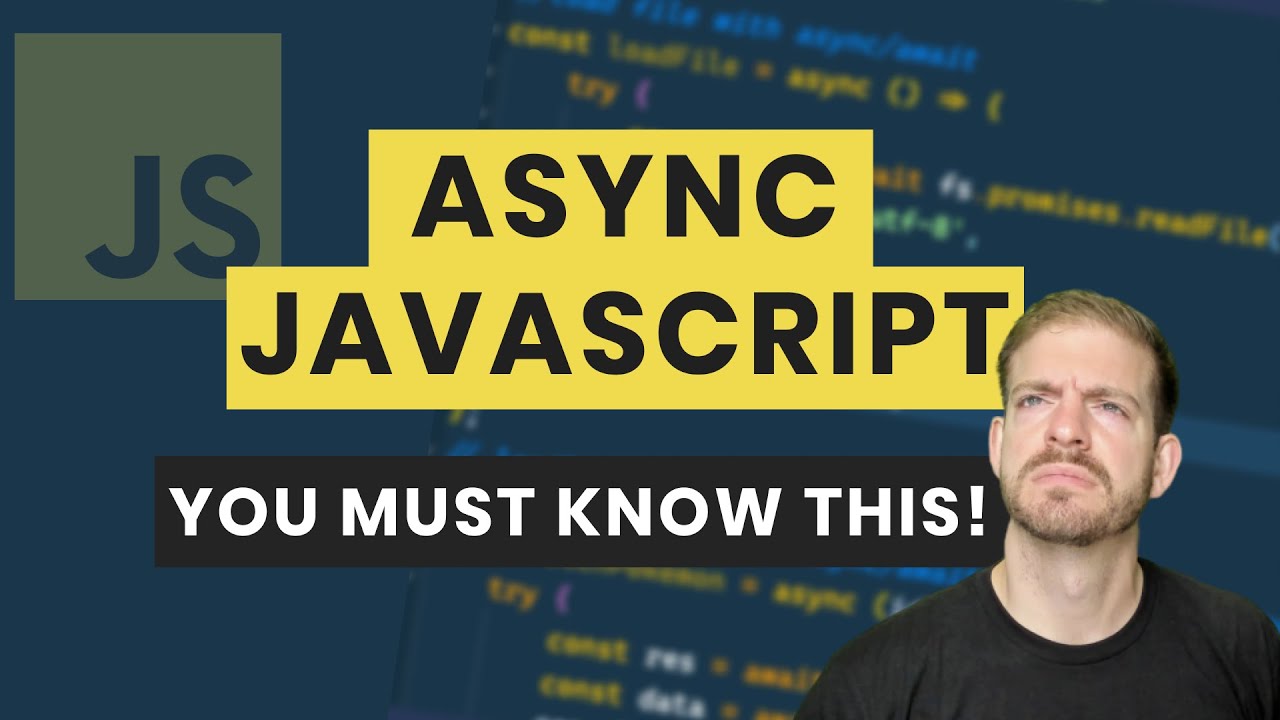
Asynchronous JavaScript in ~10 Minutes - Callbacks, Promises, and Async/Await

How JavaScript Works π₯& Execution Context | Namaste JavaScript Ep.1
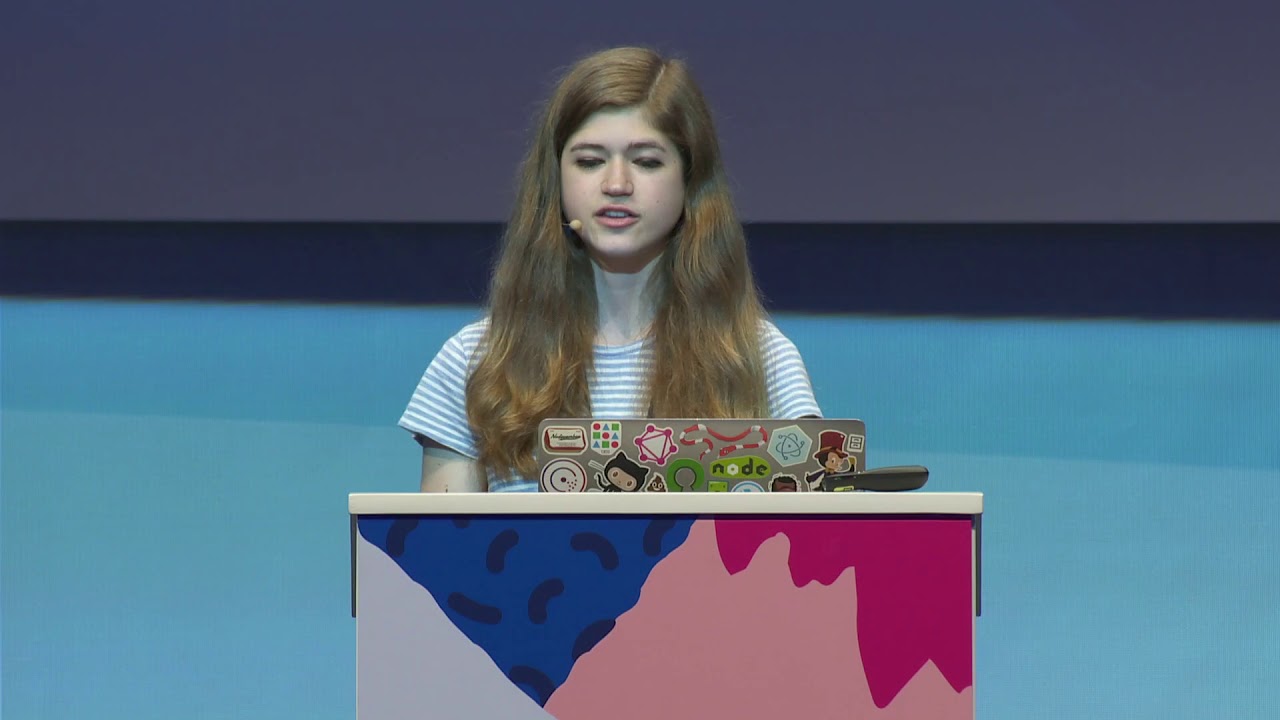
Asynchrony: Under the Hood - Shelley Vohr - JSConf EU
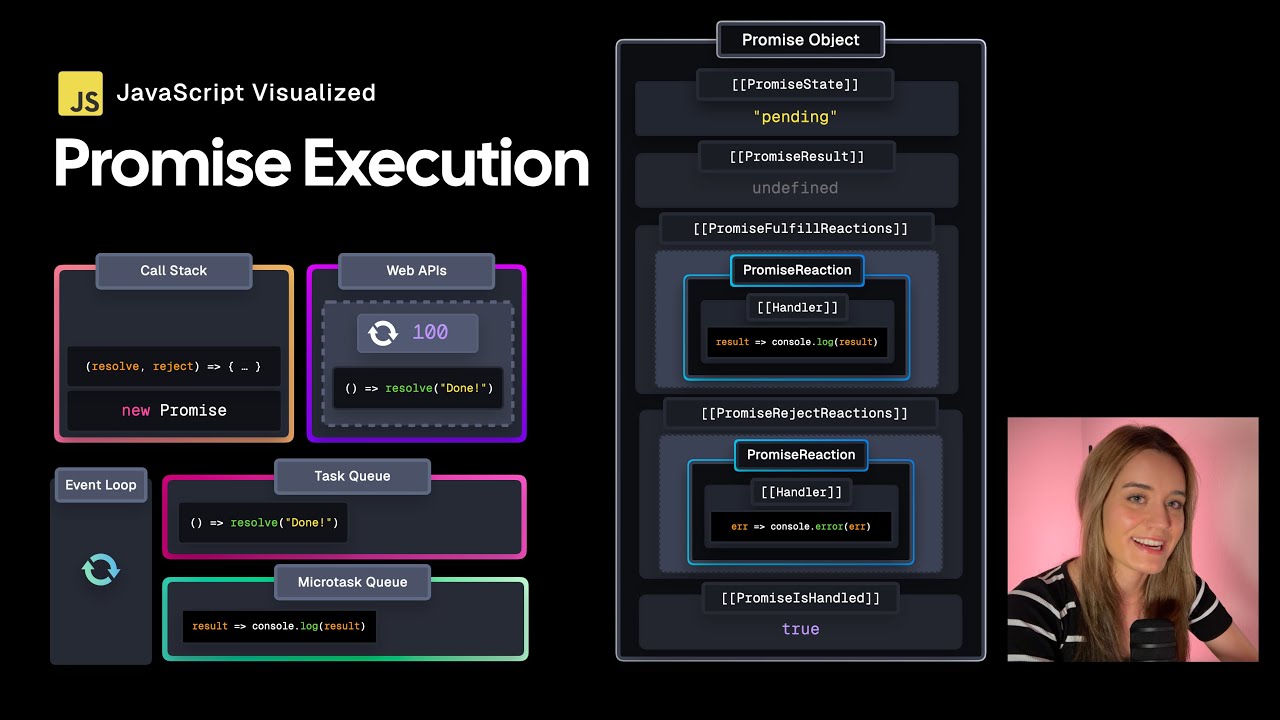
JavaScript Visualized - Promise Execution
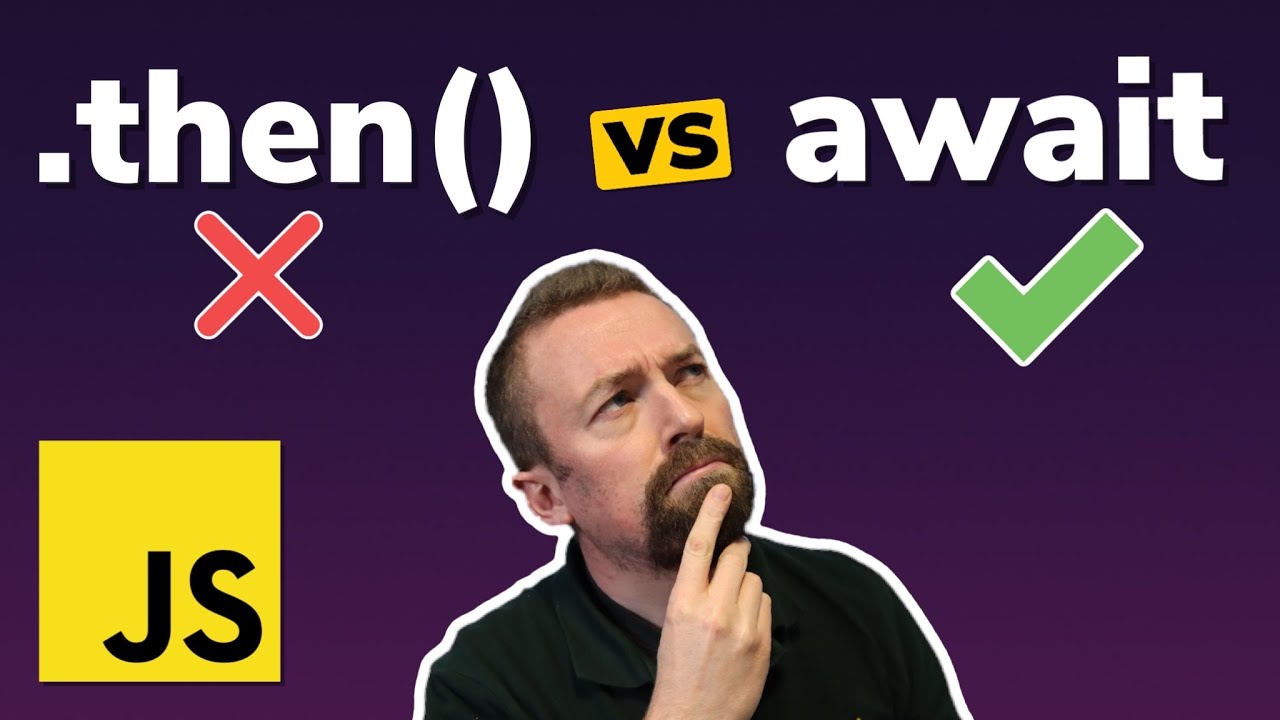
Javascript Promises vs Async Await EXPLAINED (in 5 minutes)
5.0 / 5 (0 votes)