React Context API Tutorial | Quick and Easy
Summary
TLDRIn this tutorial, the host explains how to use React Context to manage and share state across components without the need for prop drilling. The video walks through the setup of a simple e-commerce project with a shopping cart, showing how state can be lifted and shared via Context. By using Context, developers can access and update state, such as the shopping cart contents, across different components (like the Nav and Checkout) more efficiently. The video also emphasizes simplifying code organization and reusability within React applications.
Takeaways
- 📚 React Context simplifies state management by allowing shared state across components without the need for prop drilling.
- 🛠️ The video introduces the concept of React Context and how it solves the problem of prop drilling in a multi-component application.
- 🛍️ In the demo project, the example revolves around a shopping cart system, where the app needs to update the cart count and display selected products in multiple components like the nav and checkout pages.
- 🚧 Without React Context, the state would have to be 'lifted up' and passed down through props, which can become cumbersome and lead to bloated components.
- 🔄 React Context allows for centralizing state in one place (context), making it easily accessible from any component without needing to pass props manually.
- 📦 The CartContext provider is introduced, which wraps the entire app and shares the state (items in the cart) across all relevant components like nav, product cards, and checkout.
- 🔗 The `useContext` hook is used in various components to access and update the shared state (e.g., adding items to the cart).
- 🔢 The cart updates dynamically as users add items, reflecting changes both in the nav (cart count) and the checkout page (list of items).
- 🛠️ Context modularizes the application state, making it easier to manage complex features like shopping carts, user authentication, and more.
- 🎉 The final demo shows how multiple components (Nav, Products, Checkout) can efficiently share and modify state using React Context, improving the maintainability and scalability of the code.
Q & A
What is React Context, and why is it useful?
-React Context is a way to manage state globally in an application, allowing components to access shared data without passing props manually through each level of the component tree. It helps avoid 'prop drilling,' where data needs to be passed down multiple levels of nested components.
What problem does 'lifting up the state' solve, and how does it work?
-Lifting up the state solves the issue of sharing state between sibling components. By moving the state up to a common parent component, the state can be passed down via props to the necessary child components. However, this can lead to prop drilling, which Context aims to solve.
How is 'prop drilling' avoided using React Context?
-Prop drilling is avoided by using React Context because Context allows state and functions to be shared directly between components without passing them down through every level of the component hierarchy. Any component that needs the data can access it via the Context API, bypassing intermediate components.
What is the purpose of the `CardProvider` in this example?
-The `CardProvider` is a component that wraps around parts of the application and provides state and functions (like `items` and `addToCart`) to its children. This allows any component inside the `CardProvider` to access these values via Context, without needing to pass them as props.
How does the `useContext` hook function in this implementation?
-The `useContext` hook allows components to consume the values provided by a Context. In this implementation, it is used to access the shared `items` and `addToCart` function from `CardContext` in components like the nav, product cards, and checkout pages.
Why is it necessary to wrap the application with the `CardProvider`?
-Wrapping the application with the `CardProvider` ensures that all child components within the wrapped area have access to the shared state and functions (like `items` and `addToCart`) provided by the Context. Without this, the components wouldn't be able to access the Context values.
How is the `items` array managed and updated in this example?
-The `items` array, which holds the products added to the cart, is managed using the `useState` hook inside the `CardProvider`. The `addToCart` function is used to update the `items` array by pushing new products (name and price) into it whenever an item is added to the cart.
How are the items displayed in both the nav and checkout page?
-In the nav, the `items.length` is used to display the total number of items in the cart. On the checkout page, the `items` array is mapped over to display each product's name and price. Both components access the `items` array through the `useContext` hook.
What would happen if the state was not managed with Context?
-If state wasn't managed with Context, you would have to lift the state up to the parent components and pass it down through props, resulting in extensive prop drilling. This approach makes the code harder to maintain and bloats the parent components with a lot of state management logic.
How does Context make the codebase more modular and scalable?
-Context allows you to organize different types of data into separate, modular files (e.g., `CardContext`, `UserContext`). This structure separates concerns, reduces prop drilling, and makes it easier to manage different parts of the application as it grows, improving scalability and maintainability.
Outlines
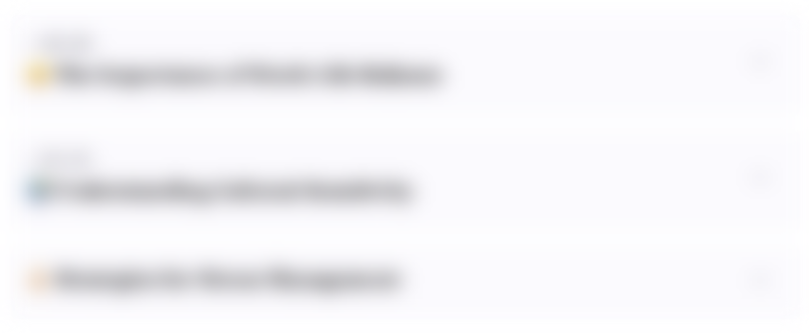
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
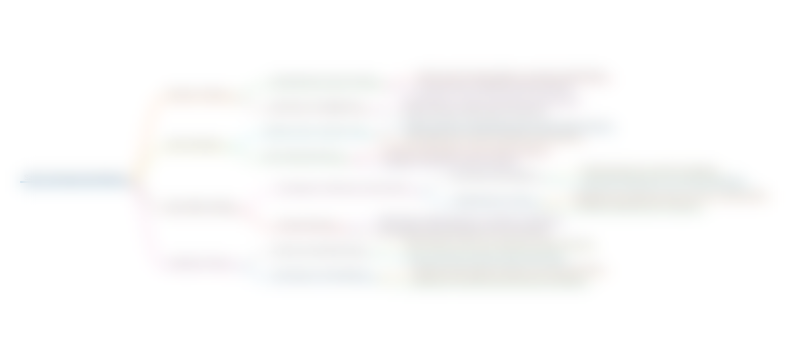
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
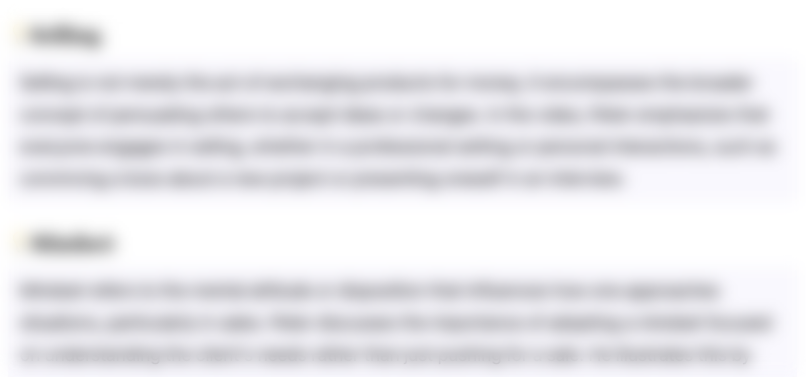
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
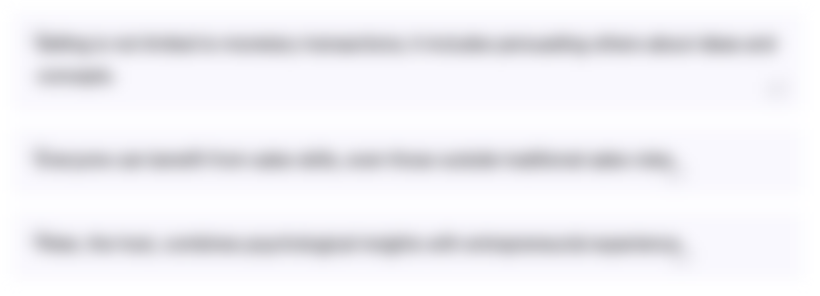
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
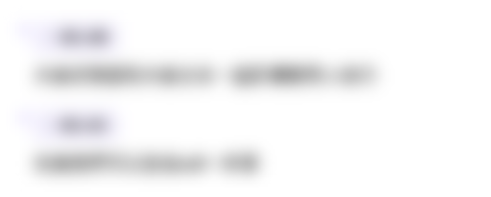
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

useContext Hook | Mastering React: An In-Depth Zero to Hero Video Series

React useContext() hook introduction 🧗♂️
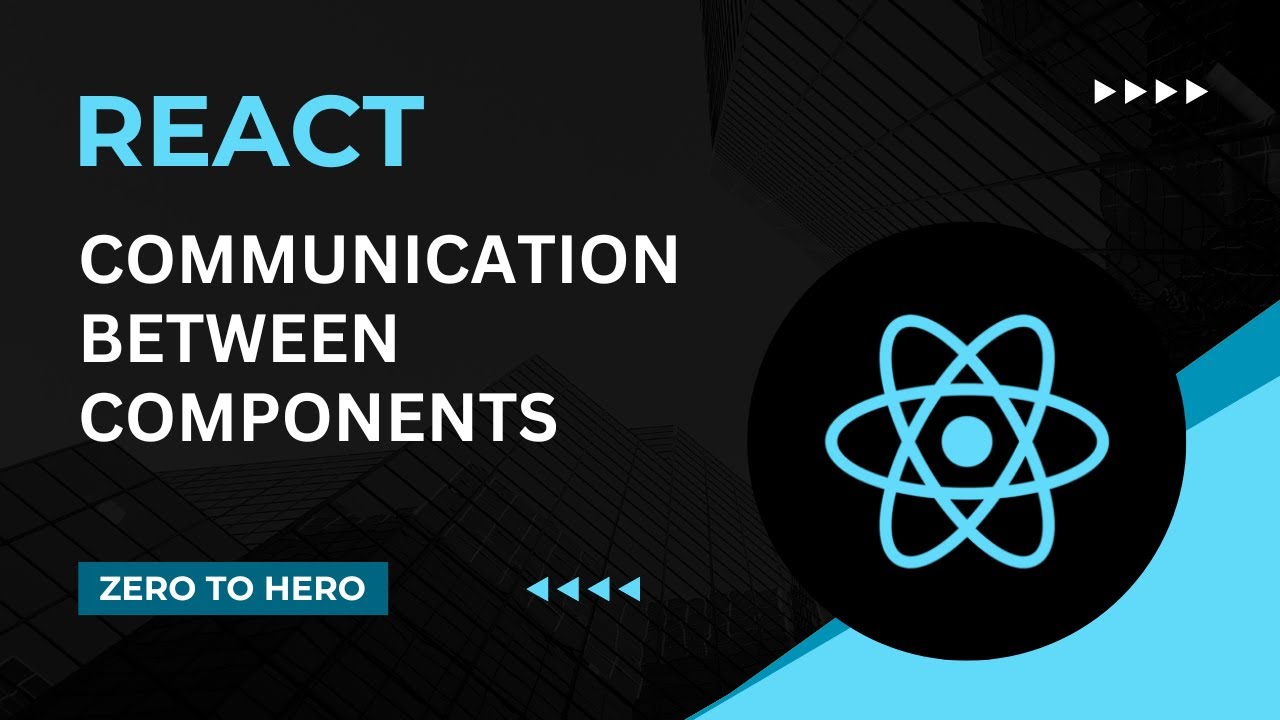
Communication between components | Mastering React: An In-Depth Zero to Hero Video Series
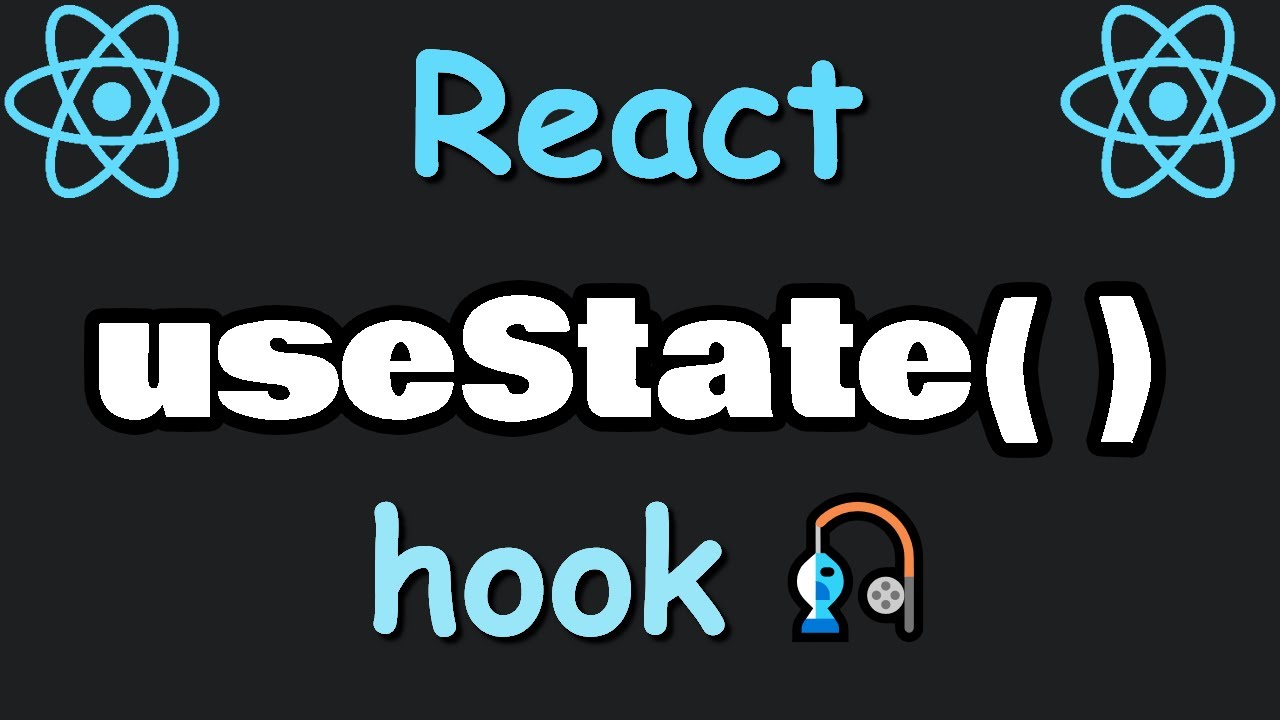
React useState() hook introduction 🎣

Component Composition | Lecture 108 | React.JS 🔥

The Key Prop | Lecture 128 | React.JS 🔥
5.0 / 5 (0 votes)