React Hooks and Their Rules | Lecture 157 | React.JS ๐ฅ
Summary
TLDRReact hooks are special functions that allow developers to tap into React's internal mechanisms, such as managing state and side effects. They expose internal React functionalities and are essential for creating and accessing state in functional components. Hooks must be called in a consistent order at the top level of the component and can only be used within React functions or custom hooks. This structure ensures that React can correctly track and manage the hooks, avoiding confusion and maintaining the integrity of the application's state across renders.
Takeaways
- ๐ React hooks are special functions that allow interaction with React's internal mechanisms.
- ๐ Hooks expose internal React functionality like state management and side effect registration.
- ๐ณ The fiber tree is an internal data structure in React that hooks are linked to.
- ๐ Hooks start with 'use' to differentiate them from regular functions and for ease of identification.
- ๐ Custom hooks enable developers to reuse non-visual logic across different components.
- ๐ฏ There are nearly 20 built-in hooks in React, with useState and useEffect being the most commonly used.
- ๐ Hooks must be called at the top level of your code, avoiding conditionals, loops, or nested functions.
- ๐ Hooks rely on call order to maintain a linked list, which is crucial for React's tracking and management.
- ๐ซ Violating the call order can lead to broken references and render issues within the fiber tree.
- ๐ The first rule of hooks ensures that the linked list of hooks remains consistent across renders.
- ๐ง Understanding the 'why' behind React hooks and their rules helps developers write more effective and maintainable code.
Q & A
What are React hooks?
-React hooks are special functions built into React that allow developers to hook into some of React's internal mechanisms, such as creating and accessing state or registering side effects.
What is the fiber tree in React?
-The fiber tree is an internal data structure within React that represents the state of the UI. It is not directly accessible to developers but is used by hooks like `useState` and `useEffect` to manage state and side effects.
Why do all hooks start with the word 'use'?
-All hooks start with the word 'use' to make it easy for developers and React to distinguish hooks from other regular functions.
What are custom hooks in React?
-Custom hooks are developer-created functions that start with 'use' and allow for the reuse of non-visual logic, such as state management and side effects, across different components.
How many built-in hooks does React come with?
-React comes with almost 20 built-in hooks, including widely used ones like `useState`, `useEffect`, `useReducer`, and `useContext`.
What are the two simple rules that must be followed for hooks to work as intended?
-The two rules are: 1) Hooks can only be called at the top level in a function, not inside loops, conditions, or nested functions, and 2) Hooks can only be called from React functions, such as function components or custom hooks, not from regular functions or class components.
Why is it important to call hooks in the same order on every render?
-Calling hooks in the same order ensures that React can correctly associate each hook with its value and maintain the integrity of the linked list of hooks. This is crucial for React to function properly and track state and side effects across renders.
What happens if you break the first rule of hooks by using them conditionally?
-If you use hooks conditionally, the linked list of hooks can get broken between renders, causing React to become confused and unable to correctly manage state and side effects, which can lead to unexpected behavior in the application.
How are hooks enforced to follow the rules?
-React's ESLint rules automatically enforce the hook rules, preventing developers from breaking them and ensuring that hooks are used correctly within the code.
What is the advantage of using a linked list for managing hooks?
-A linked list allows React to associate each hook with its value based on the call order, which simplifies the process for developers as they don't have to manually assign names to each hook, reducing the potential for errors.
Why was the introduction of hooks a significant advancement for React?
-The introduction of hooks allowed function-based components to have their own state and run side effects, which was previously only possible with class-based components. This made React more flexible and popular by simplifying the way state and lifecycle logic are managed.
Outlines
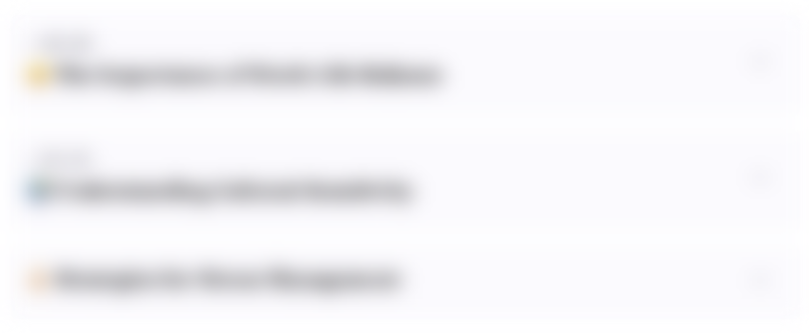
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
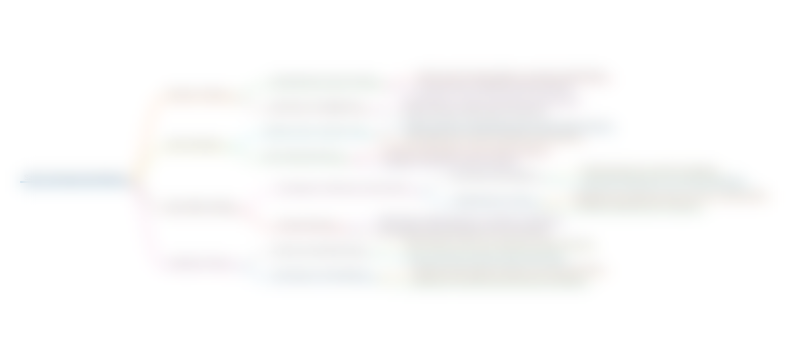
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
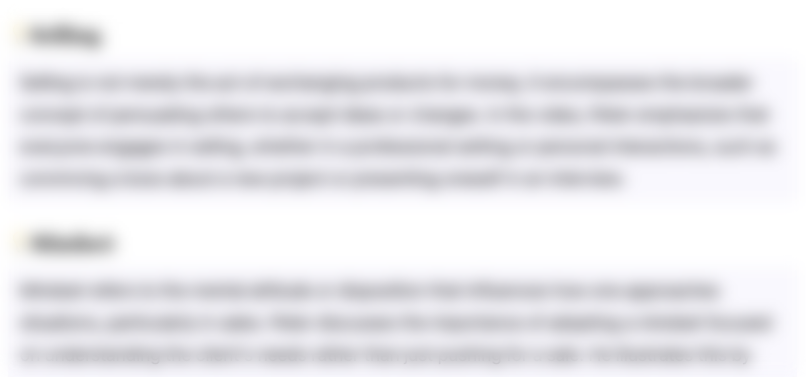
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
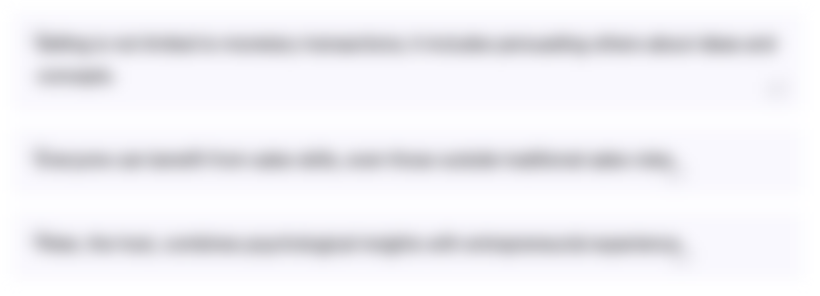
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
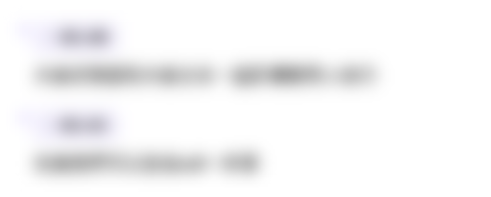
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
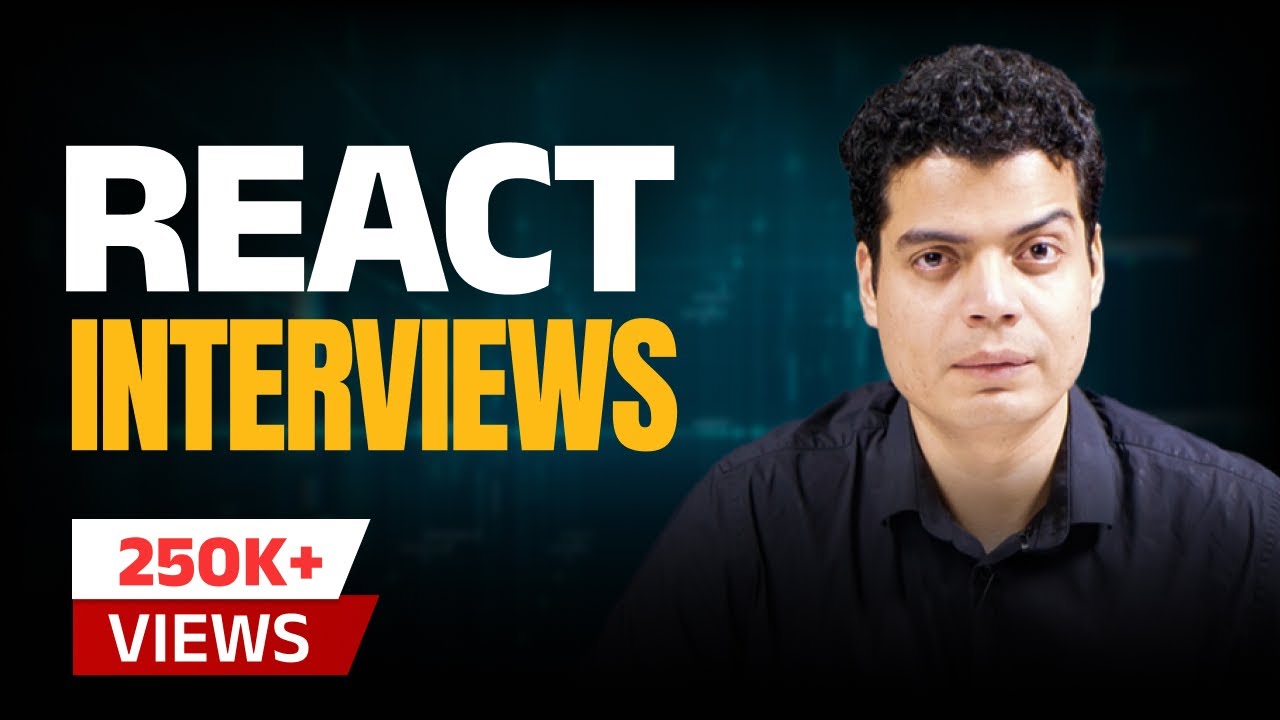
Mastering React: Decoding 09 Most Common Interview Questions | Tanay Pratap Hindi

What are Custom Hooks When to Create One | Lecture 166 | React.JS ๐ฅ
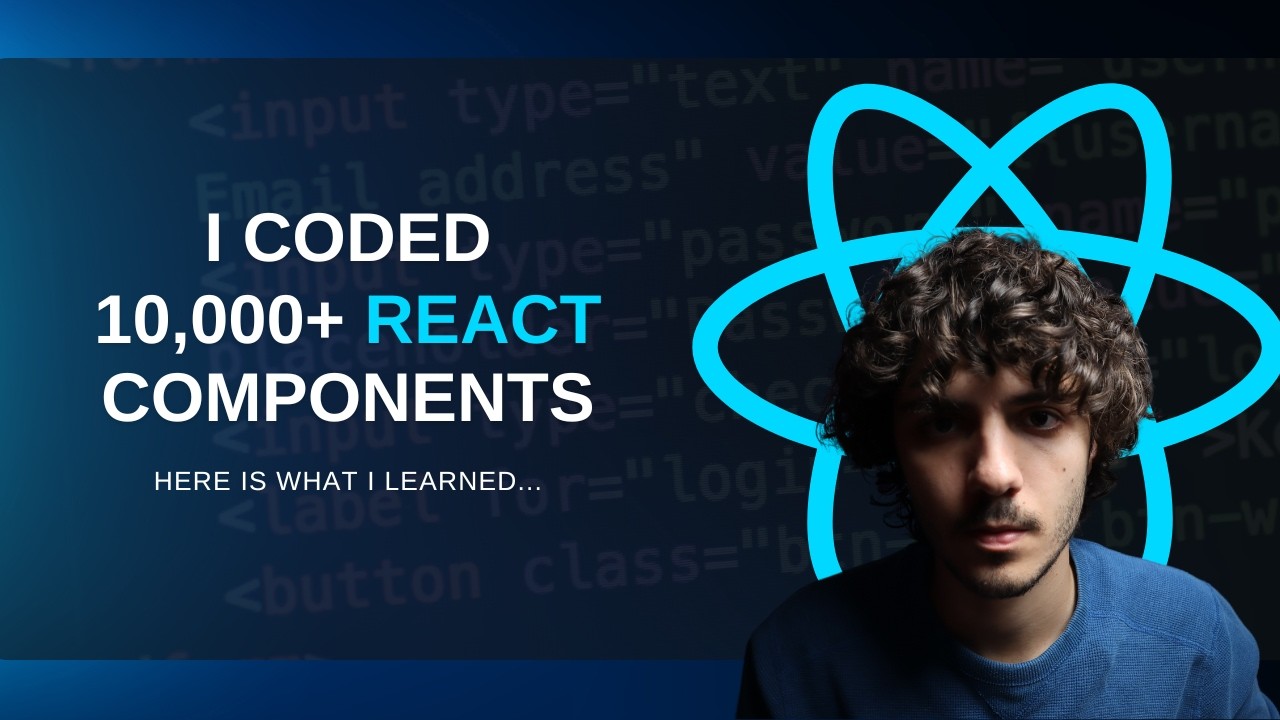
I Coded 10,000+ React Components, Here is What I Learned...
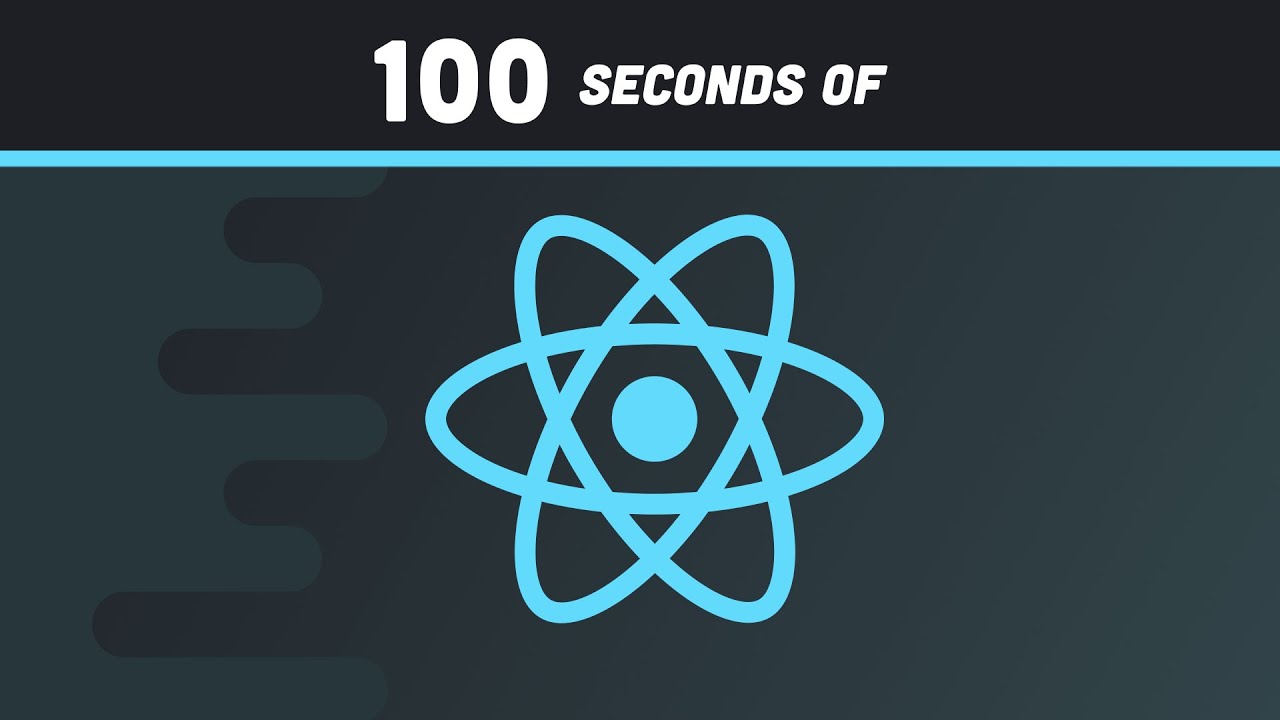
React in 100 Seconds

The useEffect Cleanup Function | Lecture 151 | React.JS ๐ฅ
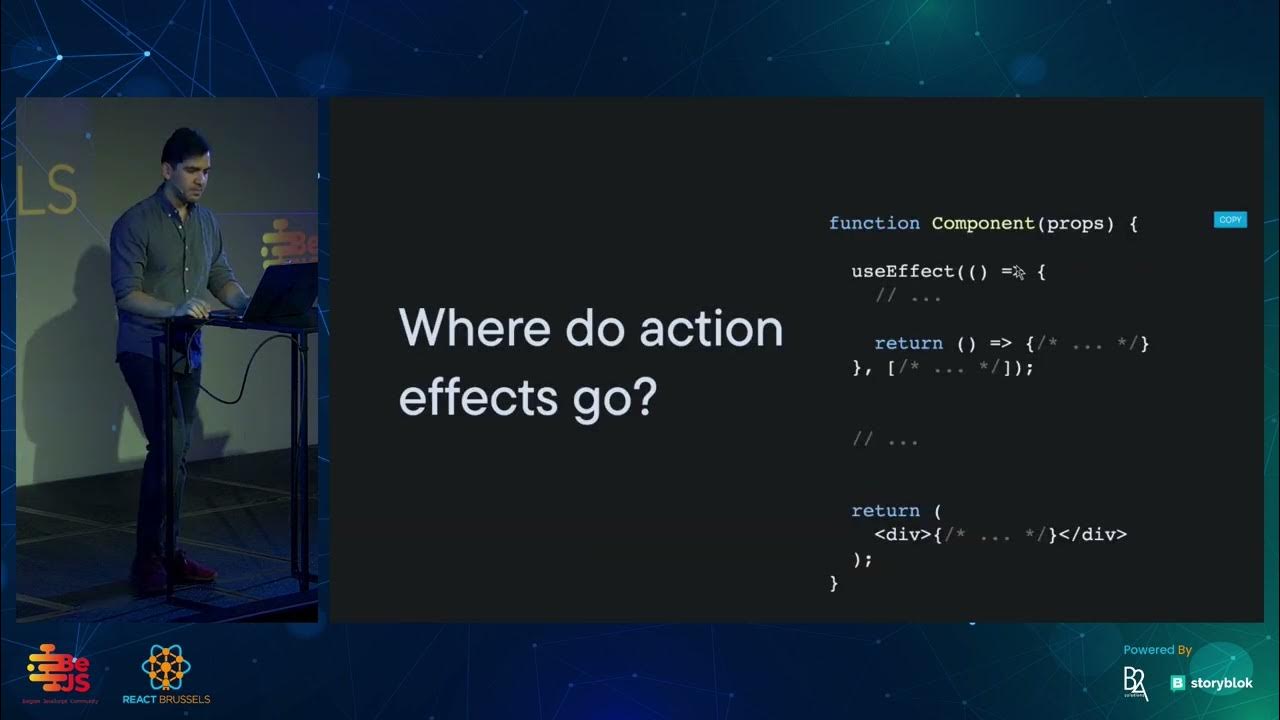
Goodbye, useEffect - David Khourshid
5.0 / 5 (0 votes)