The Volatile Keyword in Java Explained with Example | Interview Question | Multithreading |
Summary
TLDRThis video script delves into the Java 'volatile' keyword, crucial for multi-threading scenarios and often misunderstood. It explains how 'volatile' ensures visibility of changes across threads by bypassing the cache and directly interacting with main memory. The concept is exemplified using the Singleton pattern, where 'volatile' prevents multiple instances due to thread caching issues. The script aims to clarify the importance and application of 'volatile' for those new to the concept, promising a valuable insight for bragging rights among peers.
Takeaways
- π‘ The 'volatile' keyword in Java is crucial for ensuring visibility of changes across multiple threads.
- π Volatile is often misunderstood, but it's essential for understanding synchronization in multi-threaded environments.
- π¬ It's used to prevent issues that arise from caching, where a thread might not see updates made by another thread.
- π₯οΈ CPUs use cache for faster data access, which can lead to inconsistencies if not managed properly with shared variables.
- π The 'volatile' keyword forces threads to read variables directly from main memory, not from their local cache.
- π This keyword is particularly important in the Singleton design pattern to prevent multiple instances from being created.
- πΊ The example of a 'TV set' class illustrates how 'volatile' can be used to ensure only one object is instantiated.
- π οΈ The Singleton pattern restricts instantiation of a class to a single object, often using a 'volatile' static variable to control this.
- π In multi-threading, 'volatile' helps in maintaining the integrity of the application state by ensuring all threads see the most recent value of a variable.
- π Understanding and correctly applying 'volatile' requires careful consideration and is a sign of a deeper grasp of Java's concurrency mechanisms.
Q & A
What is the 'volatile' keyword in Java?
-The 'volatile' keyword in Java is used to indicate that a variable's value may change at any time, and its changes should be immediately visible to other threads. It prevents the compiler and processor from applying certain optimizations that might cache the variable in registers or thread-local storage.
Why is the 'volatile' keyword important in multi-threading?
-In multi-threading, the 'volatile' keyword is crucial because it ensures that when one thread modifies a variable, the changes are immediately reflected in the main memory, and other threads reading the variable see the updated value, not a cached or stale value.
How does cache memory affect the visibility of variable changes in threads?
-Cache memory can cause visibility issues in threads because each thread may have its own local cache. If a thread updates a variable, the change might be stored in its local cache first and not immediately propagated to the main memory, causing other threads to see an outdated value.
What problem does the 'volatile' keyword solve in the context of the Singleton pattern?
-In the Singleton pattern, the 'volatile' keyword solves the issue of multiple threads creating multiple instances of a class when using double-checked locking. By declaring the instance variable as 'volatile', it ensures that when one thread creates an instance, other threads will see the updated instance and not create a new one.
Can you provide an example of a shared variable in Java that might require the 'volatile' keyword?
-An example of a shared variable that might require the 'volatile' keyword is a status flag that controls the flow of multiple threads. If the flag's state is crucial for thread synchronization, marking it as 'volatile' ensures that all threads see the most recent value of the flag.
How does the 'volatile' keyword interact with the main memory and CPU cache?
-When a variable is declared 'volatile', the CPU is instructed to read and write the variable directly from and to the main memory, bypassing the CPU cache. This ensures that every thread accessing the variable gets the most current value from memory.
What is the role of the 'volatile' keyword in ensuring the correct implementation of the Singleton pattern?
-In the Singleton pattern, the 'volatile' keyword ensures that when an instance of the class is created, it is immediately visible to all threads. This prevents multiple threads from creating separate instances due to cache inconsistencies, thus maintaining the Singleton property.
Why might declaring a variable as 'volatile' not be enough to ensure thread safety?
-Declaring a variable as 'volatile' alone is not enough for thread safety because it only ensures visibility of changes across threads. It does not provide atomicity for compound actions (like check-then-act operations) or protection against reordering of instructions by the compiler or CPU.
Can the 'volatile' keyword be applied to any variable type in Java?
-Yes, the 'volatile' keyword can be applied to any variable type in Java, including primitives, object references, and arrays. However, its effectiveness is most relevant for variables that are accessed by multiple threads.
What are some scenarios where using 'volatile' might not be the best solution for thread synchronization?
-Using 'volatile' might not be the best solution for thread synchronization when dealing with complex data structures that require atomic updates or when a more comprehensive locking mechanism is needed to maintain the order of operations. In such cases, other synchronization tools like 'synchronized' blocks or higher-level concurrency utilities from the `java.util.concurrent` package might be more appropriate.
Outlines
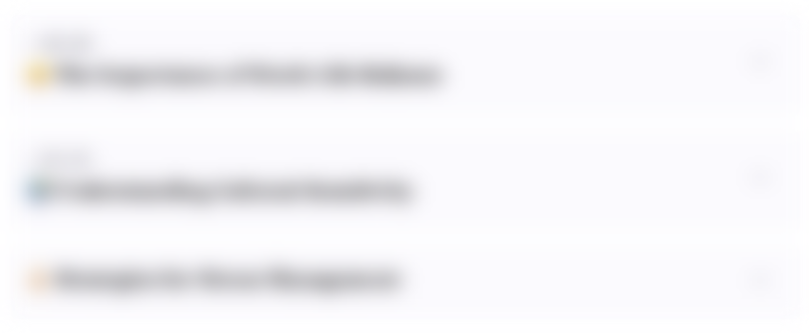
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
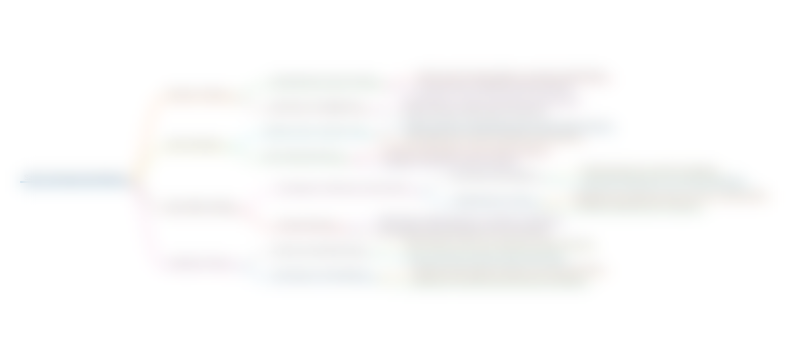
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
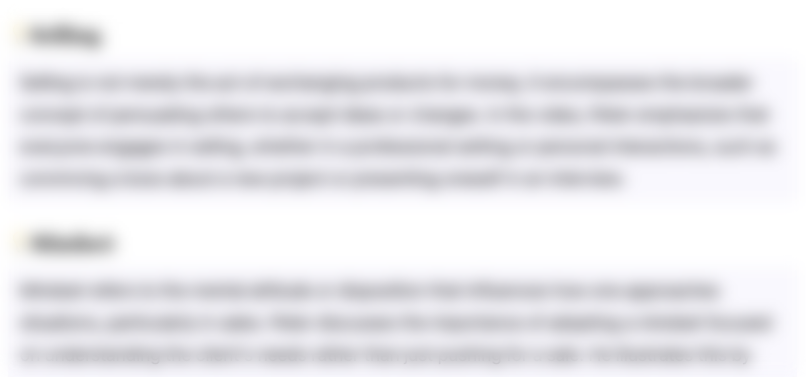
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
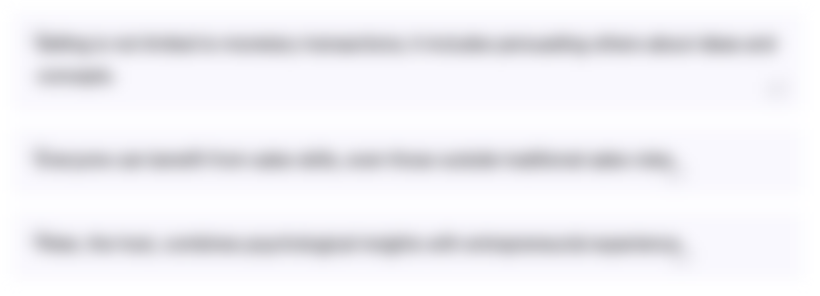
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
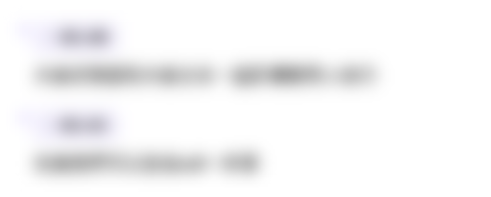
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

The Singleton Pattern Explained and Implemented in Java | Creational Design Patterns | Geekific
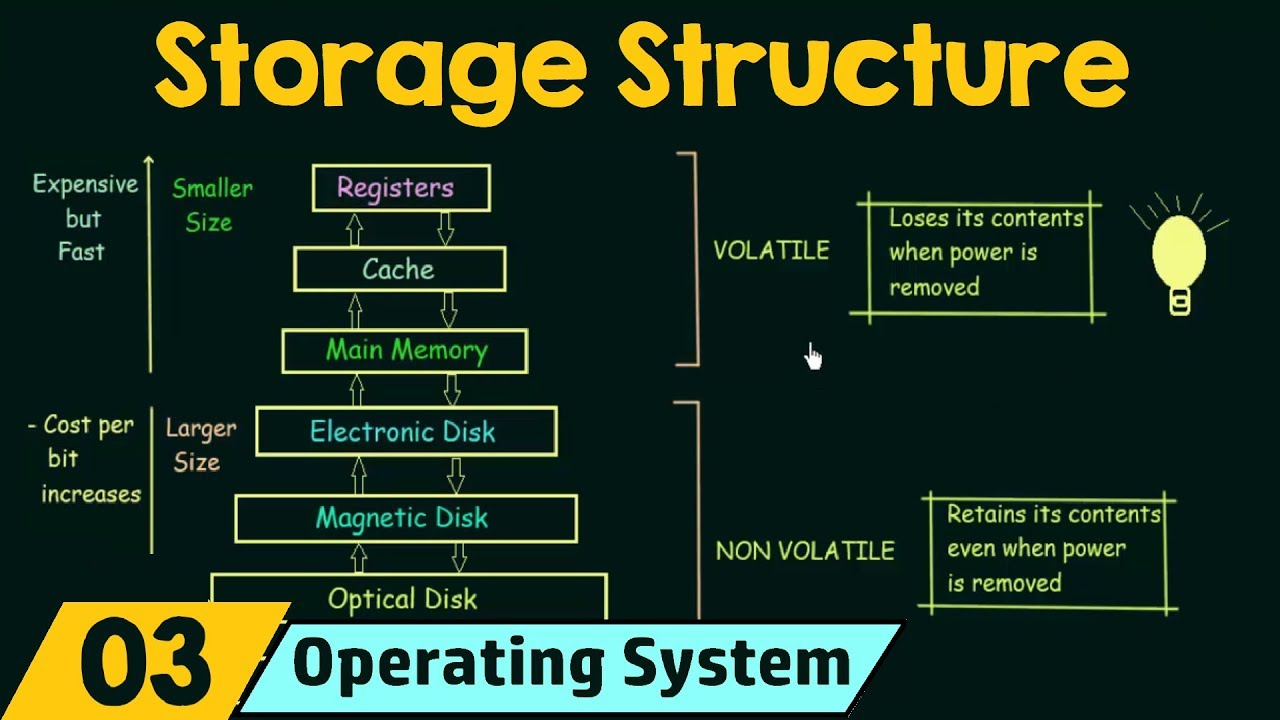
Basics of OS (Storage Structure)
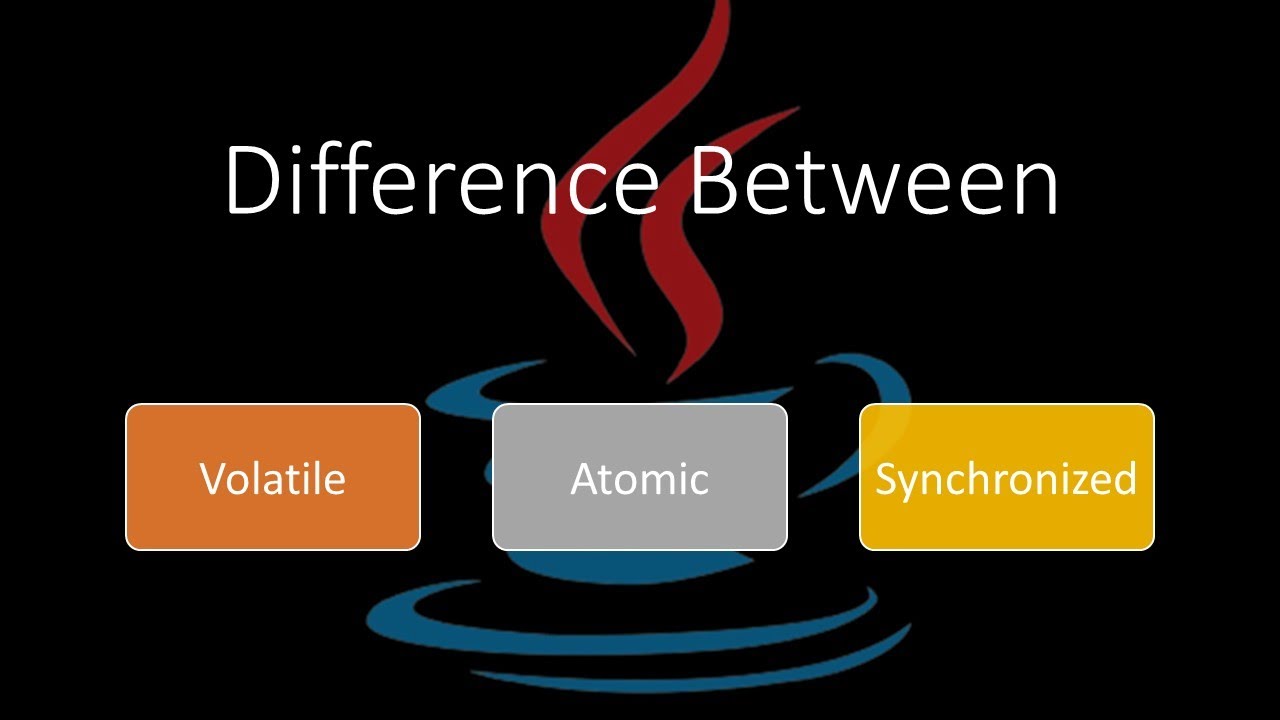
Difference Between Volatile, Atomic And Synchronized in Java | Race Condition In Multi-Threading
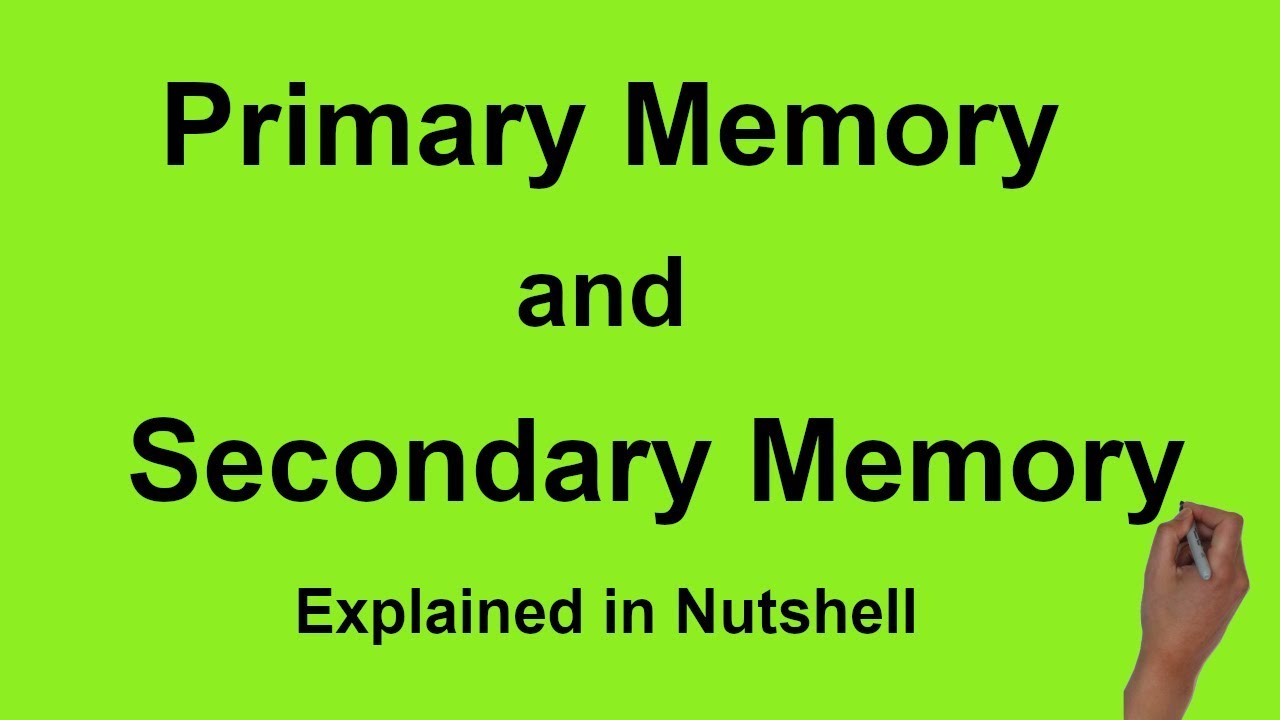
Primary Memory : Types and differences from Secondary Storage Memory

[Part 1] Unit 3.3 - Memory Units
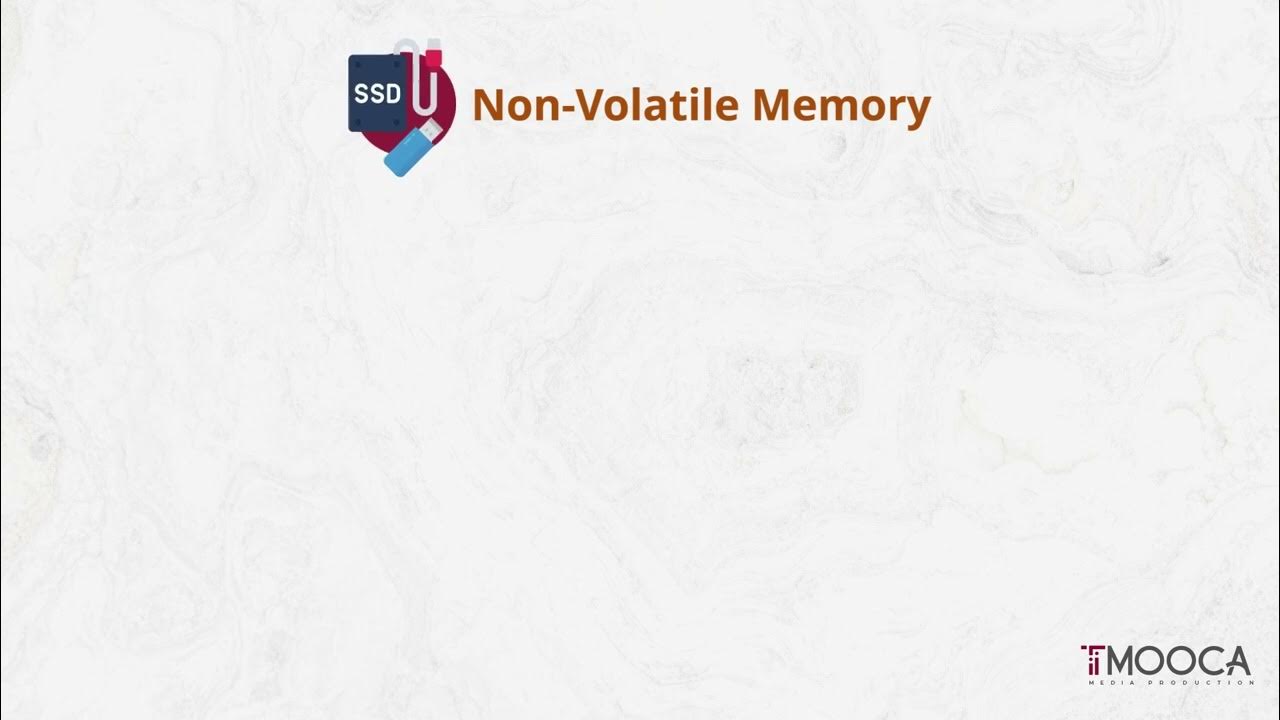
CH01_VID04_Memory Essentials
5.0 / 5 (0 votes)