Different Phases of Compiler
Summary
TLDRThis lecture covers the various phases of a compiler, including lexical analysis, syntax analysis, semantic analysis, intermediate code generation, code optimization, and target code generation. It illustrates how an arithmetic expression is processed through these phases, resulting in assembly language code. The lecture also introduces tools like Lex and Yacc for implementing compiler phases and mentions the LANCE C compiler platform for embedded processors.
Takeaways
- 📚 The lecture provides an overview of the different phases of a compiler.
- 🔍 The phases include the preprocessor, compiler, assembler, and linker/loader.
- 👨🏫 The lecture focuses on converting high-level language code into machine code.
- 🌐 The preprocessor removes preprocessor directives and embeds header files.
- 🔢 The compiler converts high-level language code into assembly language code.
- 📝 The lexical analysis phase involves tokenizing the input code.
- 🌳 The syntax analysis phase constructs a parse tree using context-free grammars.
- 🔍 The semantic analysis phase checks for type correctness and scope resolution.
- 💾 The intermediate code generator produces three-address code from the parse tree.
- 🔄 The code optimizer reduces the length of code and improves efficiency.
- 🛠️ The target code generator produces assembly code from the optimized intermediate code.
- 🛠️ Tools like Lex and Yacc are used to implement the lexical and syntax analysis phases.
- 📘 The LANCE C compiler platform is mentioned for implementing the front end of a C language compiler.
Q & A
What are the four main phases of a language translator in a compiler?
-The four main phases of a language translator in a compiler are the preprocessor, the compiler, the assembler, and the linker and loader.
What is the primary function of a preprocessor in a compiler?
-The primary function of a preprocessor is to convert high-level language code into pure high-level language code by embedding required header files and omitting preprocessor directives.
What does the lexical analysis phase of a compiler do?
-The lexical analysis phase takes lexems as input and generates tokens. It is responsible for identifying and categorizing sequences of characters into meaningful tokens that the compiler can understand.
What is a lexem and how does it differ from a word?
-A lexem is similar to a word but differs in that while words individually have their own meanings, a group of lexems in their entirety convey the meaning. For instance, 'x' individually doesn't convey any meaning until it is considered within the context of an entire arithmetic expression.
What are tokens and how are they generated?
-Tokens are the meaningful units of a programming language that represent identifiers, operators, literals, and other syntactic elements. They are generated by the lexical analyzer, which recognizes patterns using regular expressions.
Can you explain the role of regular expressions in lexical analysis?
-Regular expressions are used by the lexical analyzer to define patterns for recognizing different types of tokens. For example, a regular expression can define what constitutes a valid identifier in a language.
How does the syntax analyzer use context-free grammars?
-The syntax analyzer uses context-free grammars to analyze the stream of tokens and produce a parse tree. It follows a set of production rules to ensure that the tokens conform to the language's syntax.
What is the purpose of a parse tree in compiler design?
-A parse tree is a hierarchical structure that represents the syntactic structure of a program according to its grammatical rules. It is used to verify the syntactic correctness of the source code.
What does the semantic analyzer check for in a parse tree?
-The semantic analyzer checks for type correctness, array bounds, scope resolution, and logical consistency within the parse tree. It ensures that the program makes sense from a semantic point of view.
What is the intermediate code generator's role in the compiler?
-The intermediate code generator takes the semantically verified parse tree and produces intermediate code, such as three-address code, which is a lower-level representation of the program that can be further optimized or translated into target code.
How does the code optimizer reduce the length of the code?
-The code optimizer reduces the length of the code by eliminating unnecessary operations and variables, such as by directly assigning the result of an expression to a variable instead of using a temporary variable.
What tools can be used to implement the lexical analysis phase of a compiler?
-The tool Lex can be used to implement the lexical analysis phase. It generates a lexical analyzer from a user-provided specification.
What is the role of YACC in compiler implementation?
-YACC (Yet Another Compiler Compiler) is used to implement the syntax analysis phase. It generates a parser from a set of context-free grammar rules.
What is the significance of the Lance C compiler platform mentioned in the script?
-The Lance C compiler platform is a software platform that can be used to implement the entire front end of a C language compiler for embedded processors.
Outlines
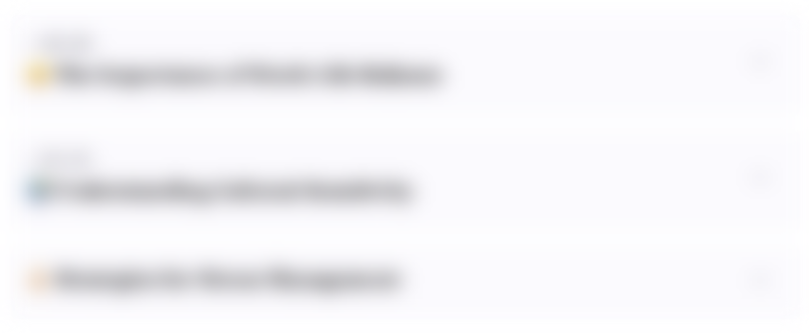
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
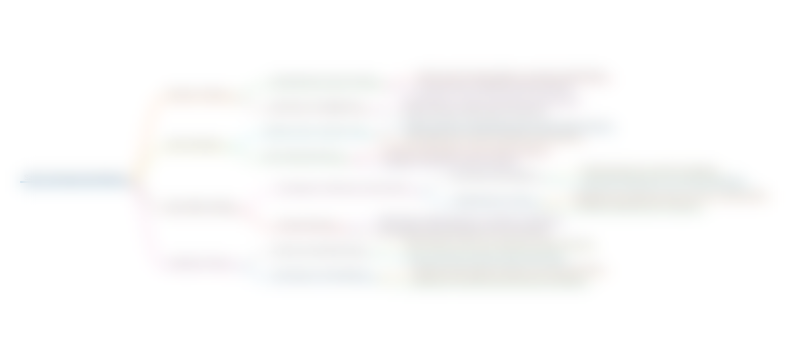
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
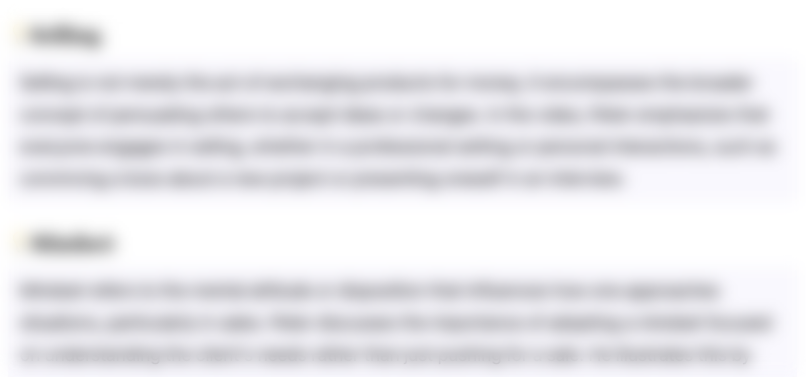
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
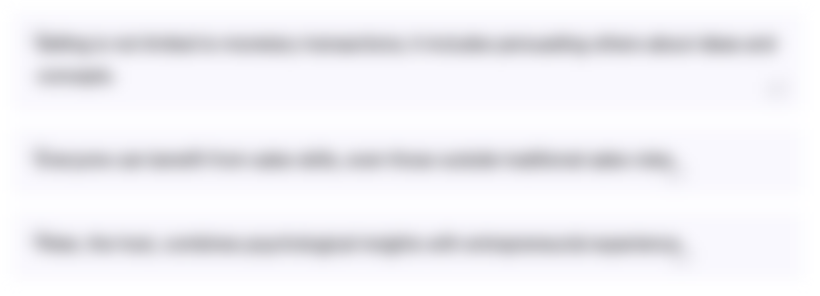
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
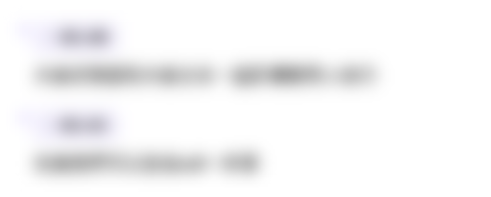
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)