Phases of Compiler | Compiler Design
Summary
TLDRThis video script offers an in-depth exploration of the compiler's six phases: Lexical Analysis, Syntax Analysis, Semantic Analysis, Intermediate Code Generation, Code Optimization, and Target Code Generation. It explains the transformation of high-level language into assembly language, emphasizing the role of the symbol table and error handling. The script uses a simple mathematical expression to illustrate each phase's function, highlighting the importance of tokenization, parse tree construction, semantic verification, and optimization, culminating in the generation of efficient assembly code.
Takeaways
- ๐ A compiler is a program that consists of six distinct phases, each responsible for a specific task in the process of translating high-level language code into assembly language.
- ๐ The Lexical Analyzer is the first phase, which breaks down the input high-level language into a stream of tokens, performing tokenization.
- ๐ The Syntax Analyzer, also known as the Parser, constructs a parse tree from the stream of tokens, ensuring the code adheres to the language's grammar rules.
- ๐ The Semantic Analyzer checks the parse tree for semantic correctness, including type checking, undeclared variables, and multiple declarations, using a symbol table to store variable information.
- ๐ ๏ธ Intermediate Code Generation takes the semantically verified parse tree and converts it into an intermediate representation, often in the form of three-address code.
- โ๏ธ Code Optimization is an optional phase that refines the three-address code to reduce program size and improve efficiency by minimizing the number of lines.
- ๐ฏ Target Code Generation is the final phase, translating the optimized intermediate code into assembly language, which is closer to machine code.
- ๐ The Symbol Table is a data structure used across all compiler phases to store and manage information about variables, such as their names and data types.
- ๐ฎโโ๏ธ The Error Handler is responsible for reporting errors detected during any phase of the compilation process back to the user.
- ๐ It is important to note that the compiler phases are not strictly sequential and can be executed in parallel, depending on the implementation.
- ๐ The script provides an example of compiling a simple line of code, demonstrating the role of each phase and the transformation from high-level language to assembly language.
Q & A
What are the six phases of a compiler?
-The six phases of a compiler are Lexical Analyzer, Syntax Analyzer, Semantic Analyzer, Intermediate Code Generation, Code Optimization, and Target Code Generation.
What is the primary input for the compiler?
-The primary input for the compiler is pure high-level language, which it then converts into assembly language.
What is the role of the Lexical Analyzer in the compilation process?
-The Lexical Analyzer takes the input stream of characters from the high-level language and converts it into a stream of tokens, a process known as tokenization.
What is a token in the context of a Lexical Analyzer?
-A token is a sequence of characters that represents the smallest unit of the language, such as identifiers, keywords, operators, and constants.
What does the Syntax Analyzer do with the stream of tokens?
-The Syntax Analyzer constructs a parse tree from the stream of tokens, following a set of rules known as grammar to ensure the input is syntactically correct.
What is the purpose of the Semantic Analyzer?
-The Semantic Analyzer verifies the parse tree semantically, checking for type correctness, undeclared variables, and multiple declarations, ensuring the program makes sense logically.
How does the Intermediate Code Generation phase represent the program?
-The Intermediate Code Generation phase represents the program in a form known as three-address code, which is a popular format for intermediate code.
What is the function of the Code Optimization phase?
-The Code Optimization phase aims to optimize the three-address code, improving the efficiency of the program by reducing the number of lines or the size of the code without changing its functionality.
What does the Target Code Generation phase produce?
-The Target Code Generation phase produces assembly code, which is a lower-level representation of the program that is closer to machine code.
What is the role of the Symbol Table in the compilation process?
-The Symbol Table is a data structure used to store information about the variables, their types, and other identifiers used in the program. It is utilized by various phases of the compiler, especially the Semantic Analyzer and the Lexical Analyzer, to keep track of declared elements.
What is the significance of the Error Handler in the compiler?
-The Error Handler is responsible for reporting errors detected during the compilation process to the user. It is used by all phases of the compiler to ensure that any issues are communicated effectively.
Outlines
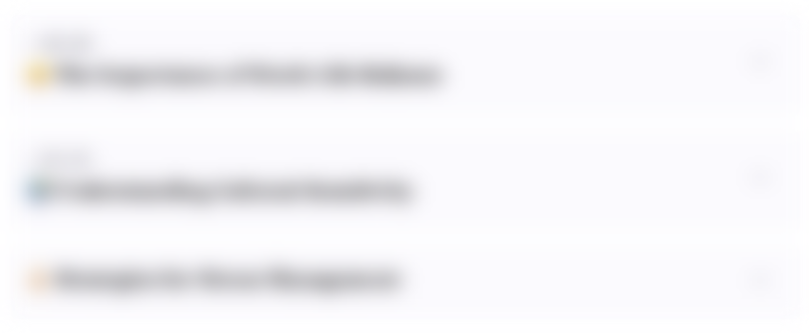
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
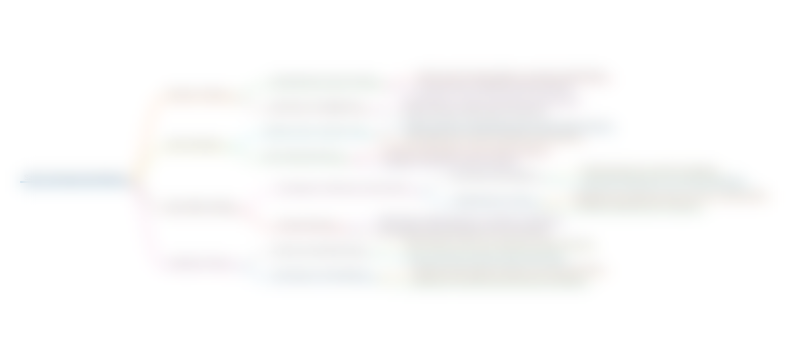
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
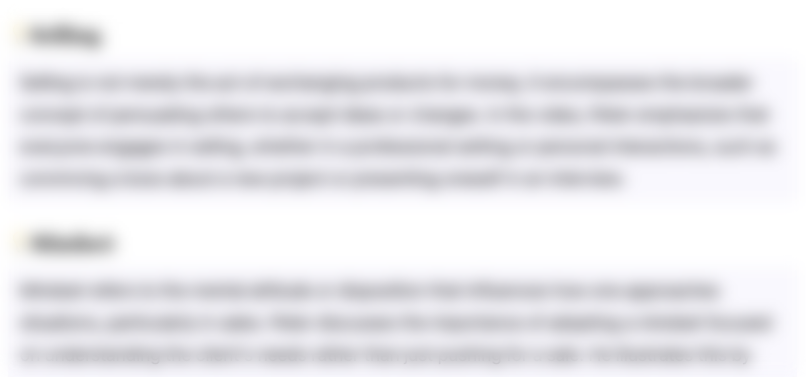
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
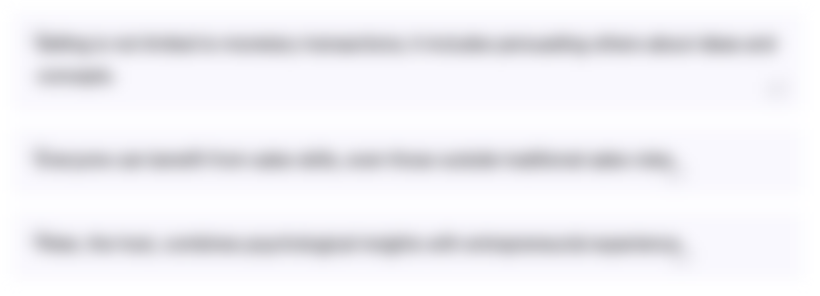
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
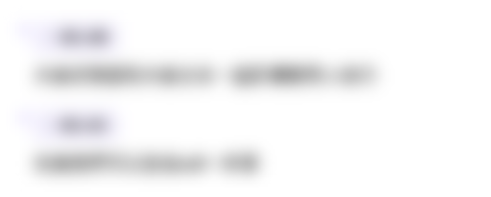
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)