RxJS Top Ten - Code This, Not That
Summary
TLDRThis video script offers an insightful introduction to RxJS, a JavaScript library for managing asynchronous streams of data. It explains the importance of RxJS in handling data over time, such as DOM events and WebSockets, and how it overcomes 'callback hell'. The tutorial covers the installation, basic concepts like observables, subjects, and operators, and demonstrates practical examples. It also addresses common issues like memory leaks and strategies to prevent them, making it an informative guide for developers looking to harness the power of reactive programming.
Takeaways
- 🎵 RxJS is a challenging but rewarding JavaScript library for managing data flow over time.
- 📈 RxJS is popular because it handles asynchronous data streams more efficiently than JavaScript alone.
- 🔄 The core concept in RxJS is the 'Observable,' which acts as a data wrapper that can be subscribed to.
- 🚰 Think of using RxJS like plumbing, with Observables as pipes for data.
- 🌳 RxJS is tree-shakable, meaning you should only import the classes and operators you need to minimize bundle size.
- 🔔 An Observable notifies subscribers whenever data changes, making it a key part of reactive programming.
- 🔄 Subjects in RxJS are hot Observables that allow new values to be pushed to them, like a pump.
- 🌟 Behavior Subjects store the last emitted value, ensuring all subscribers receive the latest data.
- ⚙️ Operators in RxJS help manipulate data flow; examples include 'map,' 'filter,' 'scan,' 'tap,' 'debounce,' and 'throttle.'
- 🛠️ RxJS offers tools for error handling (e.g., 'catchError') and memory leak prevention (e.g., 'takeWhile,' 'takeUntil').
Q & A
What is RxJS and why is it considered difficult but rewarding to learn?
-RxJS is a JavaScript library for reactive programming using Observables to handle asynchronous streams of data. It's considered difficult due to its complex concepts and functional programming approach, but rewarding because it provides powerful tools for controlling data flow over time.
How does RxJS compare in popularity to Angular and other libraries?
-RxJS is quite popular, with more daily npm downloads than Angular. It is one of the largest functional libraries, second only to lodash, indicating its widespread use in handling asynchronous data streams.
Why do we need RxJS when we already have Promises in JavaScript?
-While Promises are useful for handling single asynchronous values, RxJS is needed for dealing with real-time streams of data. Promises do not support multiple values over time, which is where RxJS, with its Observables, comes into play.
What is the fundamental class in RxJS and how is it used?
-The fundamental class in RxJS is the Observable. It acts as a wrapper for data that can be subscribed to, notifying subscribers of new data changes over time.
How can one prevent bundling the entire RxJS library in their project?
-To prevent bundling the entire library, one should import only the specific classes and operators needed in their source code. This practice, known as tree shaking, significantly reduces the final bundle size.
What is the difference between the 'of' and 'from' functions in RxJS?
-The 'of' function creates an Observable from a set of arguments and emits each of them as individual items. The 'from' function takes an array, promise, or iterable and emits each individual item from the source.
How can RxJS be used to handle DOM events?
-RxJS can create an Observable from DOM events using the 'fromEvent' function. By passing a DOM element and the event type, an Observable can be set up to emit events each time the specified event occurs on the element.
What are the differences between hot and cold observables in RxJS?
-Hot observables can have multiple subscriptions and can emit items regardless of the number of subscribers. Cold observables, on the other hand, create a new execution for each subscription and do not emit items until a subscription is made.
What is a Subject in RxJS and how does it differ from an Observable?
-A Subject in RxJS is a special type of Observable that allows values to be multicasted to many Observers. Additionally, it has an added 'next' method to push new values into the stream after it has been created, unlike a regular Observable.
What are some common operators used in RxJS to control the flow of data?
-Some common operators in RxJS include 'map' for transforming emitted values, 'filter' to emit only certain values, 'take' to emit only a specific number of values, and 'catchError' to handle errors within the Observable stream.
How can one prevent memory leaks when using RxJS subscriptions?
-Memory leaks can be prevented by unsubscribing from subscriptions when they are no longer needed. Alternatively, using operators like 'takeWhile' can automatically stop the subscription when a certain condition is met, thus avoiding the need for manual unsubscription.
What is the purpose of the 'switchMap' operator in RxJS?
-The 'switchMap' operator is used to switch from an outer Observable to an inner Observable based on the emitted values of the outer Observable. It is particularly useful for handling relational data or when you need to switch context based on the data flow.
What are 'combineLatest' and 'merge' operators in RxJS and how do they differ?
-The 'combineLatest' operator takes an array of Observables and emits an array containing the latest values from each Observable whenever any of them emit a new value. The 'merge' operator, on the other hand, subscribes to each Observable in the array and simply emits all values from them as they come in, without waiting for the others.
Outlines
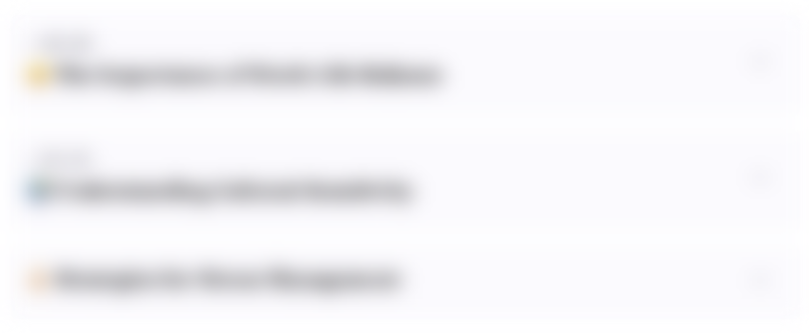
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
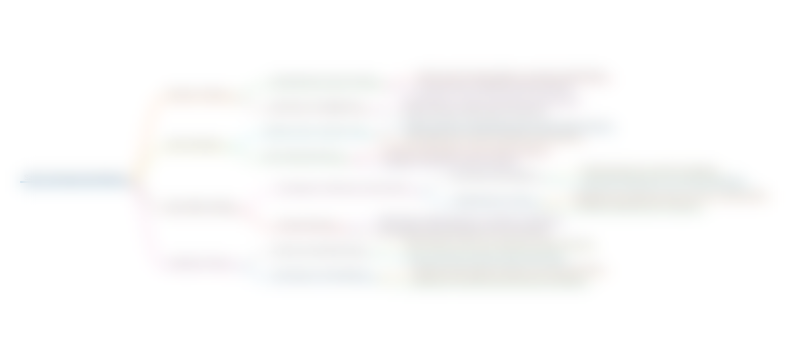
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
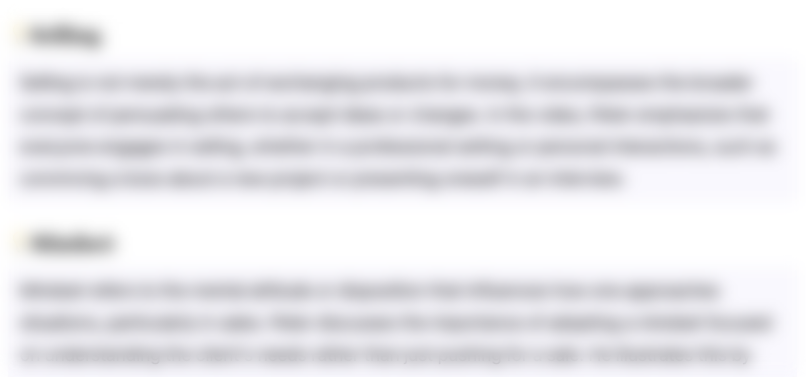
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
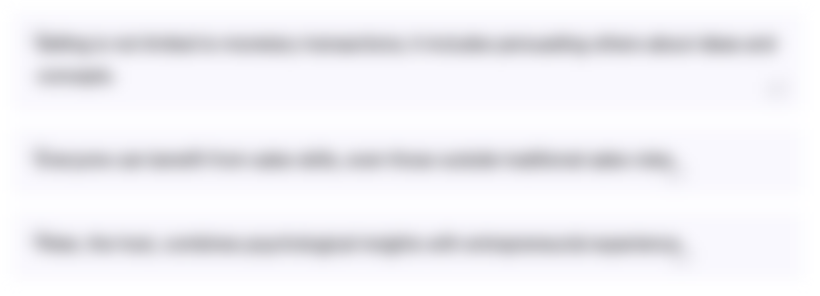
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
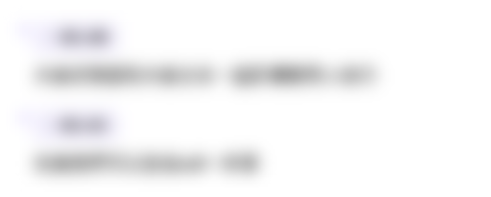
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
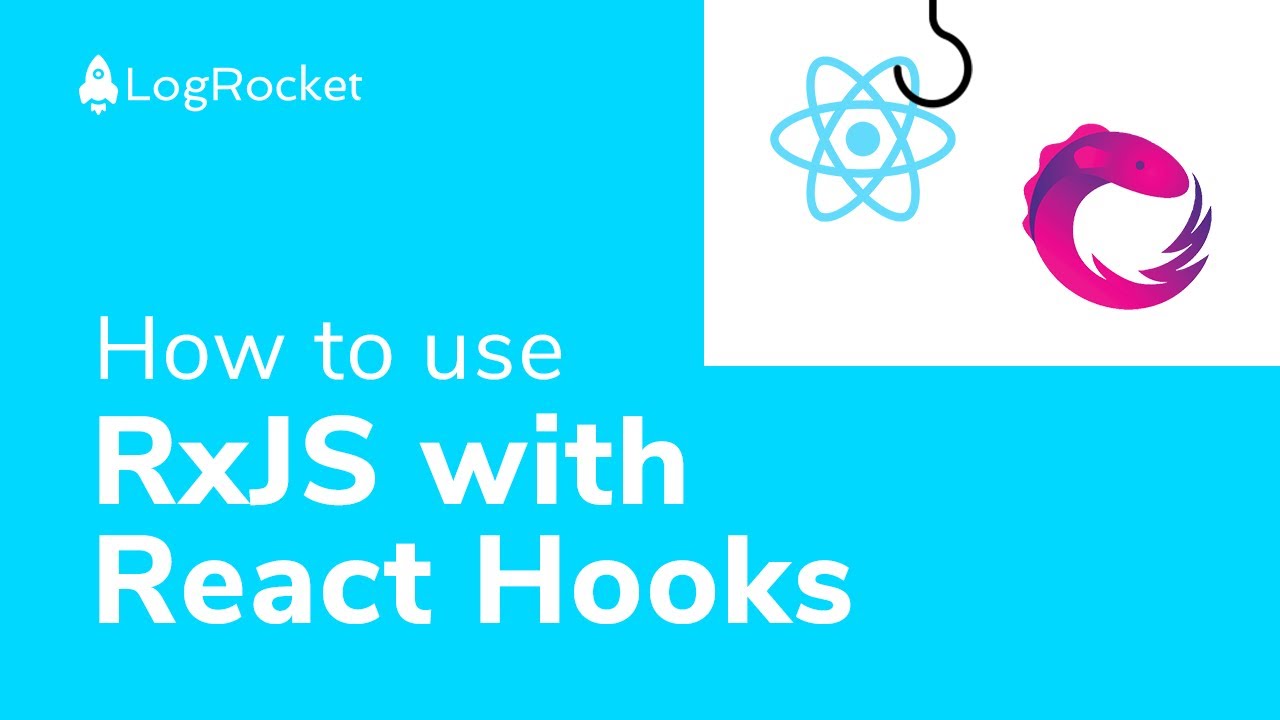
How to use RxJS with React Hooks
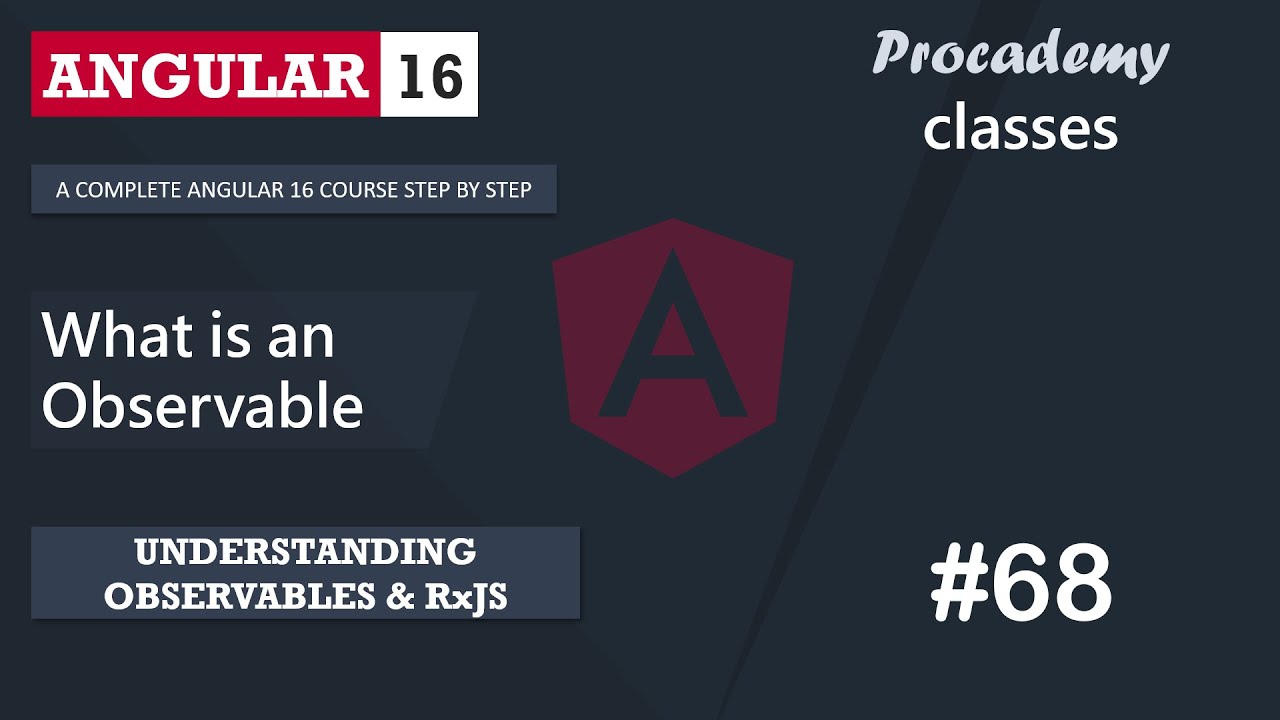
#68 What is an Observable | Understanding Observables & RxJS | A Complete Angular Course
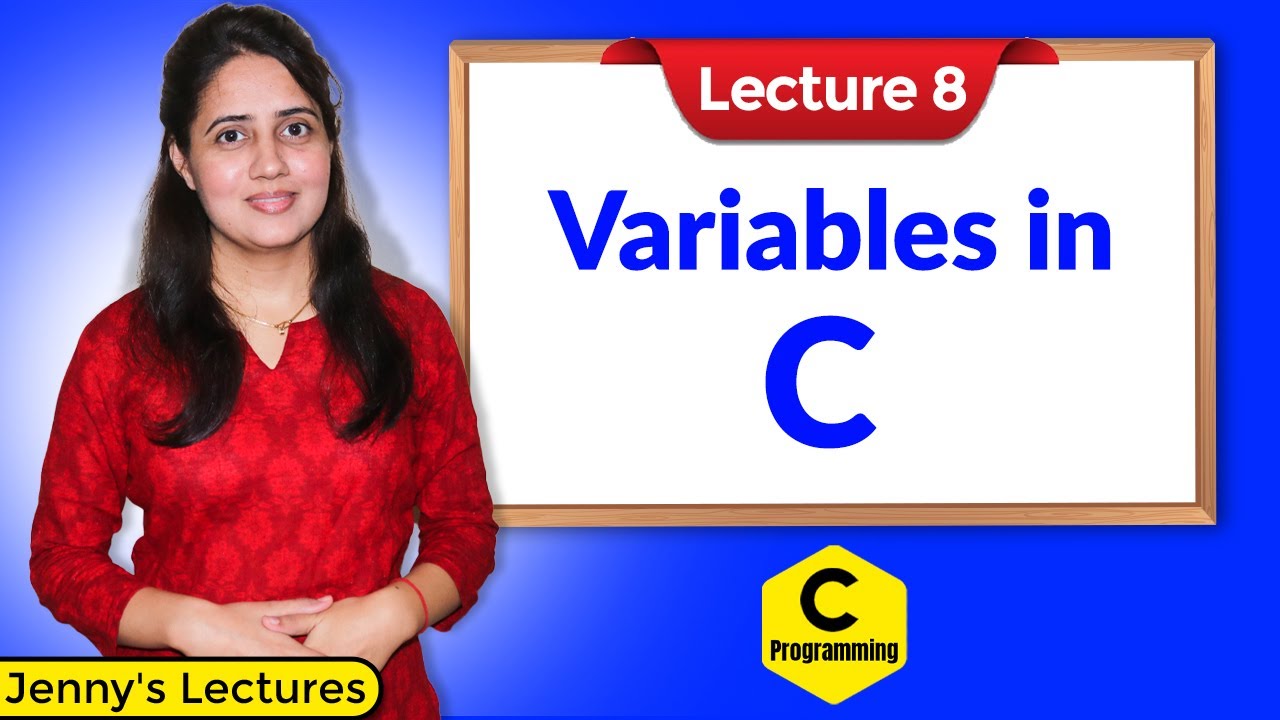
C_08 Variables in C Programming | C Programming Tutorials
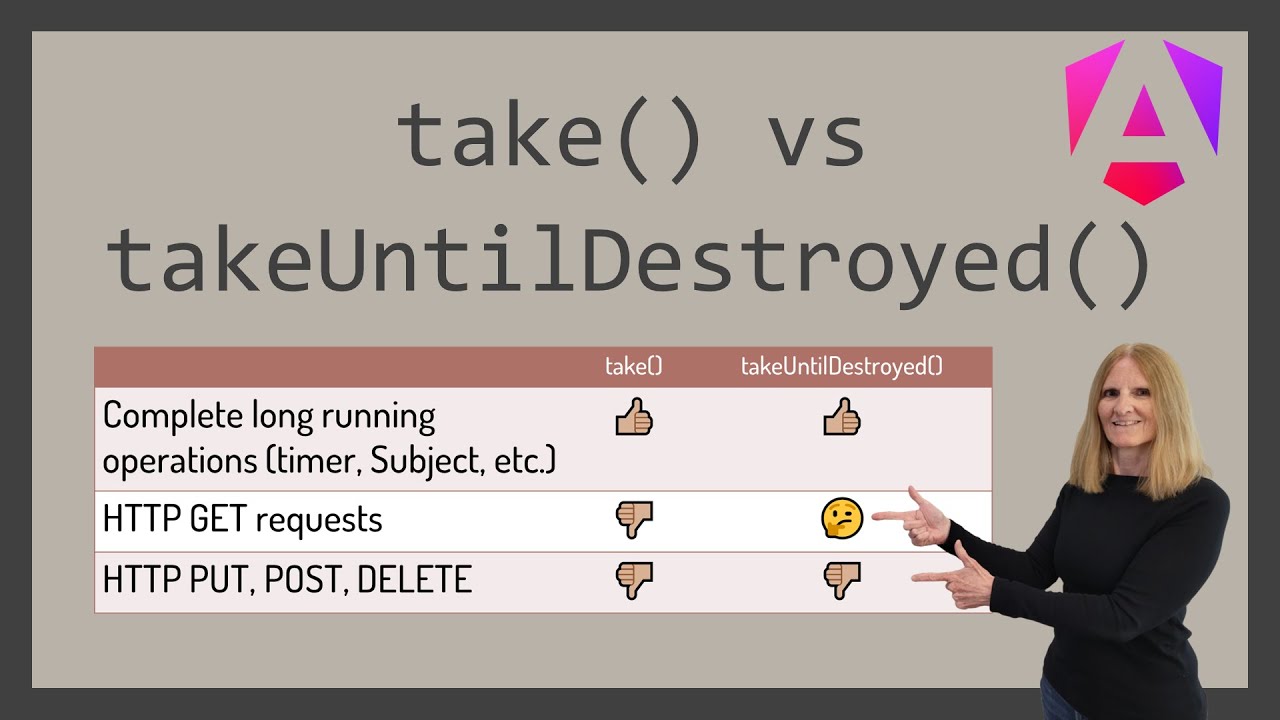
When to use take() vs takeUntilDestroyed()?
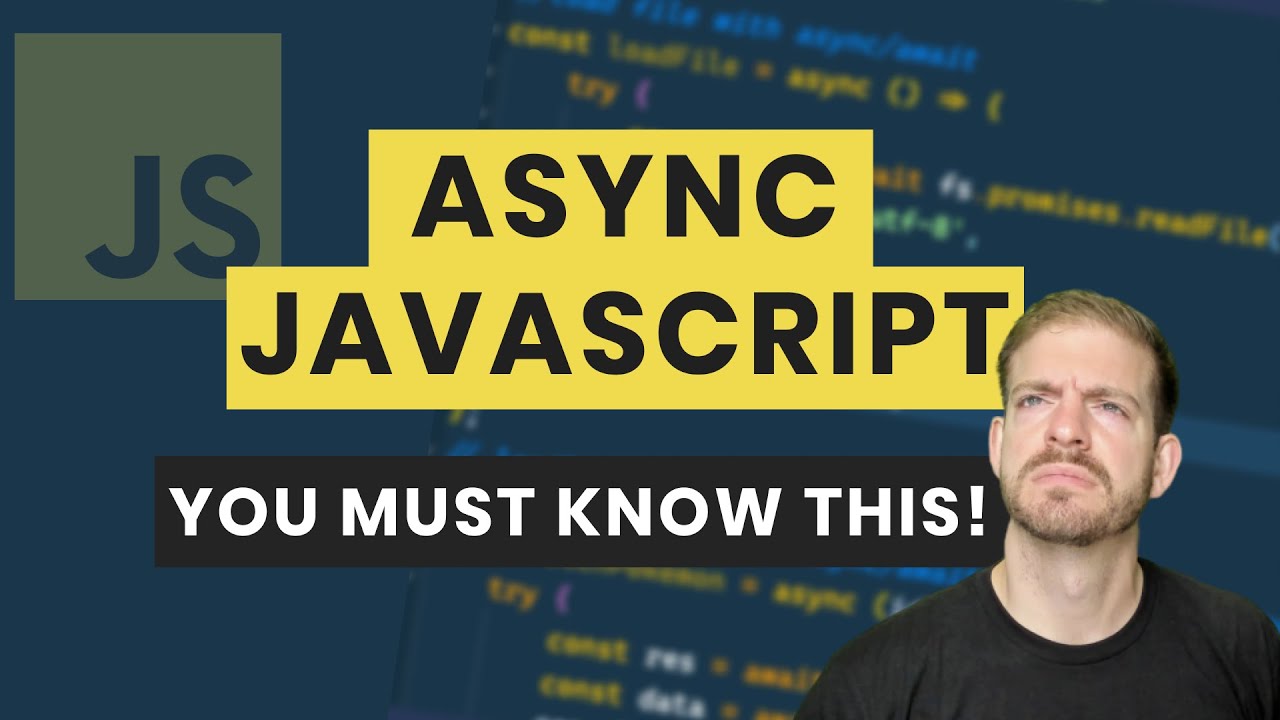
Asynchronous JavaScript in ~10 Minutes - Callbacks, Promises, and Async/Await

Variable Basics (Updated) | Godot GDScript Tutorial | Ep 1.1
5.0 / 5 (0 votes)