Object Oriented Programming Features Part 3 | C ++ Tutorial | Mr. Kishore
Summary
TLDRIn this 'Nourish 80' episode, Kishore dives into object-oriented programming, focusing on the distinctions between C structures and C++ classes. He elucidates that C++ classes support both variables and functions, unlike C structures, and highlights the default privacy of class members versus the public nature of C structure members. Kishore also explains concepts like encapsulation, data hiding, and inheritance, which are pivotal to object-oriented programming. The video is a comprehensive guide for beginners looking to understand the fundamentals of classes in C++.
Takeaways
- 📘 The class in C++ is an extension of the C structure, allowing both variables and member functions within its definition.
- 🔑 A major difference between C structures and C++ classes is that by default, C structure members are public, while C++ class members are private.
- 🏭 C++ classes support the concept of inheritance, unlike C structures, which cannot be used as a base for deriving other structures.
- 👥 C++ classes are used for constructing complex programs due to their support for inheritance, while structures are typically used for simpler programs.
- 📦 Classes in C++ act as a blueprint for creating objects, serving as a logical copy from which multiple physical instances (objects) are made.
- 🔒 Classes provide the concept of encapsulation, bundling data and member functions into a single unit and controlling access to the data through member functions.
- 🙈 Data hiding is achieved in classes through private access specifiers, ensuring that class data is not directly accessible from outside the class, promoting security.
- 📝 Member functions can be defined both inside and outside of the class definition, with different implications for inline functions and scope resolution.
- 👀 Access to private class members must be done through public member functions, illustrating the principle of data hiding and encapsulation.
- 🛠️ The class syntax includes the class keyword, class name, access specifiers, data members, member functions, and ends with a semicolon.
- 📚 Understanding the differences between C structures and C++ classes is crucial for utilizing object-oriented programming features effectively in C++.
Q & A
What is the main difference between C structures and C++ classes?
-The main difference is that C structures only allow variables inside them, while C++ classes allow both variables and member functions, which are functions defined within the class.
What are the three types of access specifiers provided by C++ classes?
-The three types of access specifiers are private, public, and protected. These specifiers determine the visibility and accessibility of the class members.
Why are member functions in a class necessary when the data members are private?
-Member functions are necessary to access and manipulate private data members, as direct access from outside the class is restricted. This is part of the encapsulation concept in object-oriented programming.
What is the purpose of encapsulation in object-oriented programming?
-Encapsulation is the mechanism of binding data (variables) and member functions together into a single unit, which is the class. It is used to hide the internal state of an object and require all interaction to be performed through an object's methods.
What is data hiding in the context of classes?
-Data hiding is the concept where the internal data (data members) of a class are kept private and are not directly accessible from outside the class, ensuring that the data can only be manipulated through member functions.
Why is it important to have at least one public member function in a class?
-At least one public member function is important because it allows for interaction with the class's private data members. Without a public interface, the class would be inaccessible and thus, not useful.
What is the default access level for class members in C++?
-By default, class members in C++ are private, meaning they are not accessible from outside the class unless specifically made public or protected through access specifiers.
Can structures in C++ be used for inheritance?
-No, structures in C++ do not participate in inheritance. Only classes can be used as base classes to derive other classes.
What is the difference between defining a member function inside and outside the class?
-Defining a member function inside the class makes it an inline function, which can be expanded at the point of use by the compiler. Defining it outside the class requires the use of the class name and scope operator, and it does not have the inline property.
How is an object of a class declared in C++?
-An object of a class is declared by specifying the class name followed by the object name. For example, if the class name is 'Student', an object can be declared as 'Student s;'.
What is the role of the scope operator (::) when defining a member function outside the class?
-The scope operator (::) is used to specify that the function being defined is a member of the class. It distinguishes the member function from global functions with the same name.
Outlines
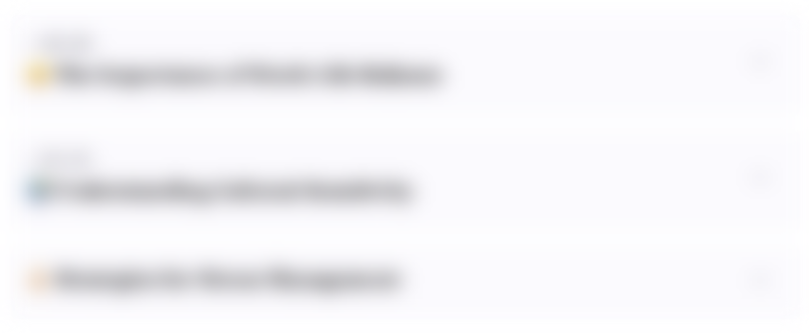
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
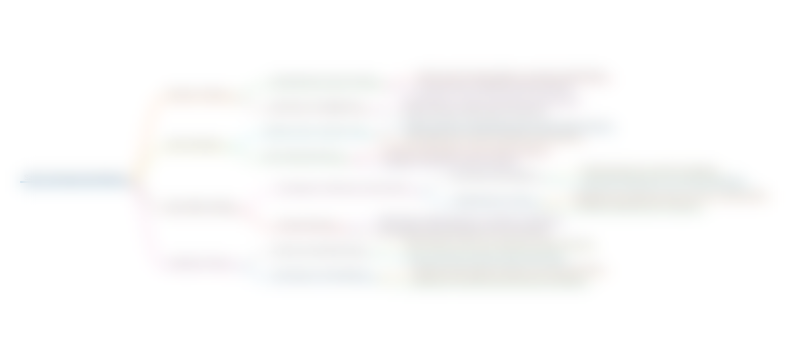
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
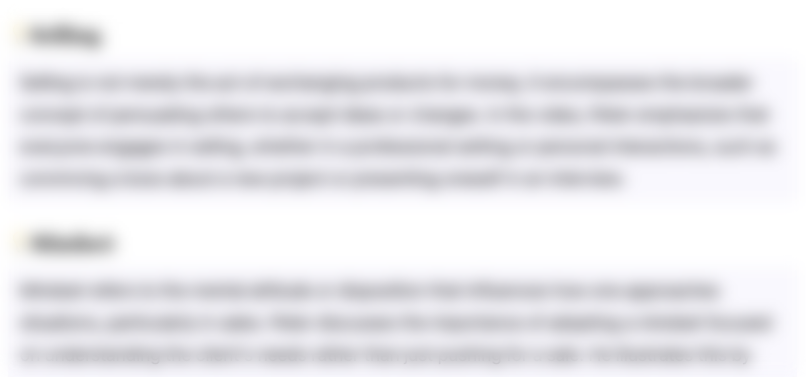
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
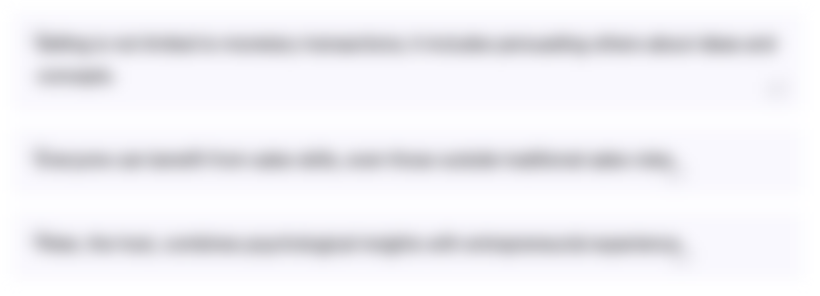
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
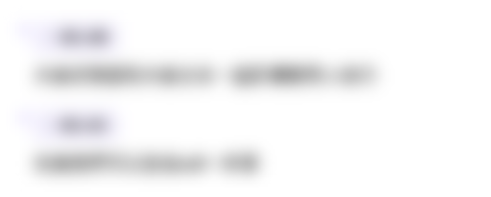
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
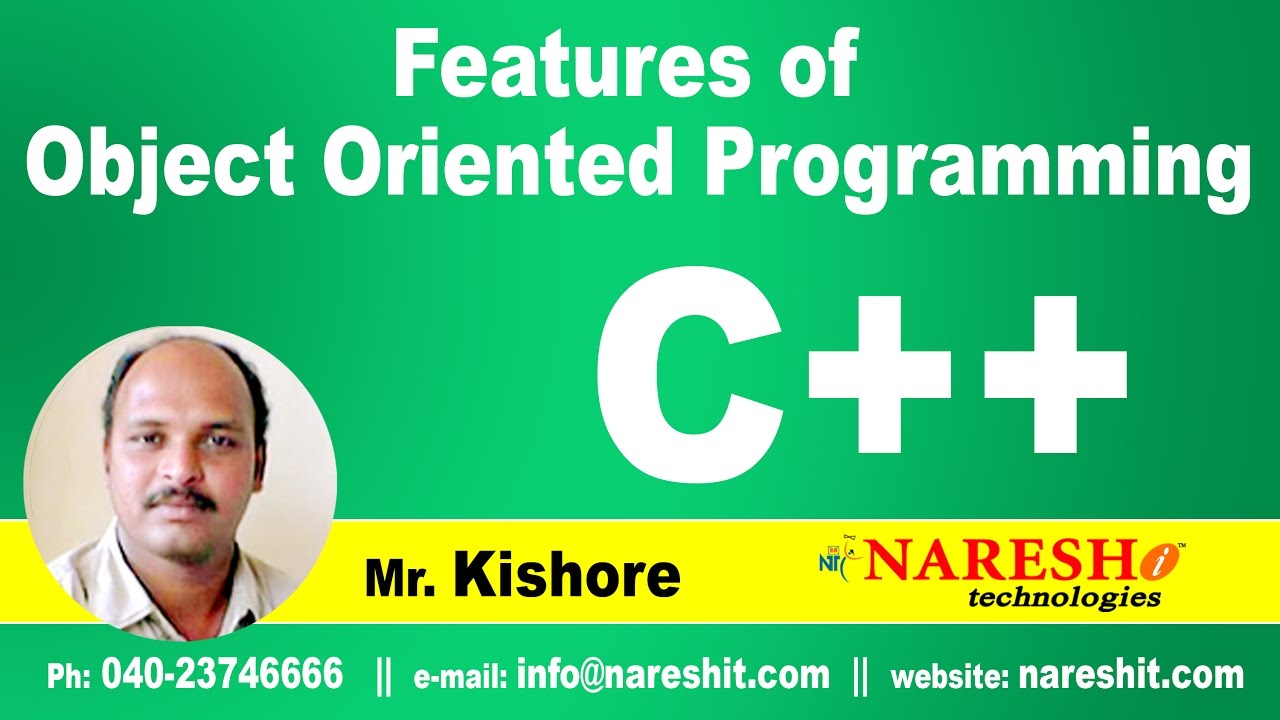
Object Oriented Programming Features Part 4 | C ++ Tutorial | Mr. Kishore
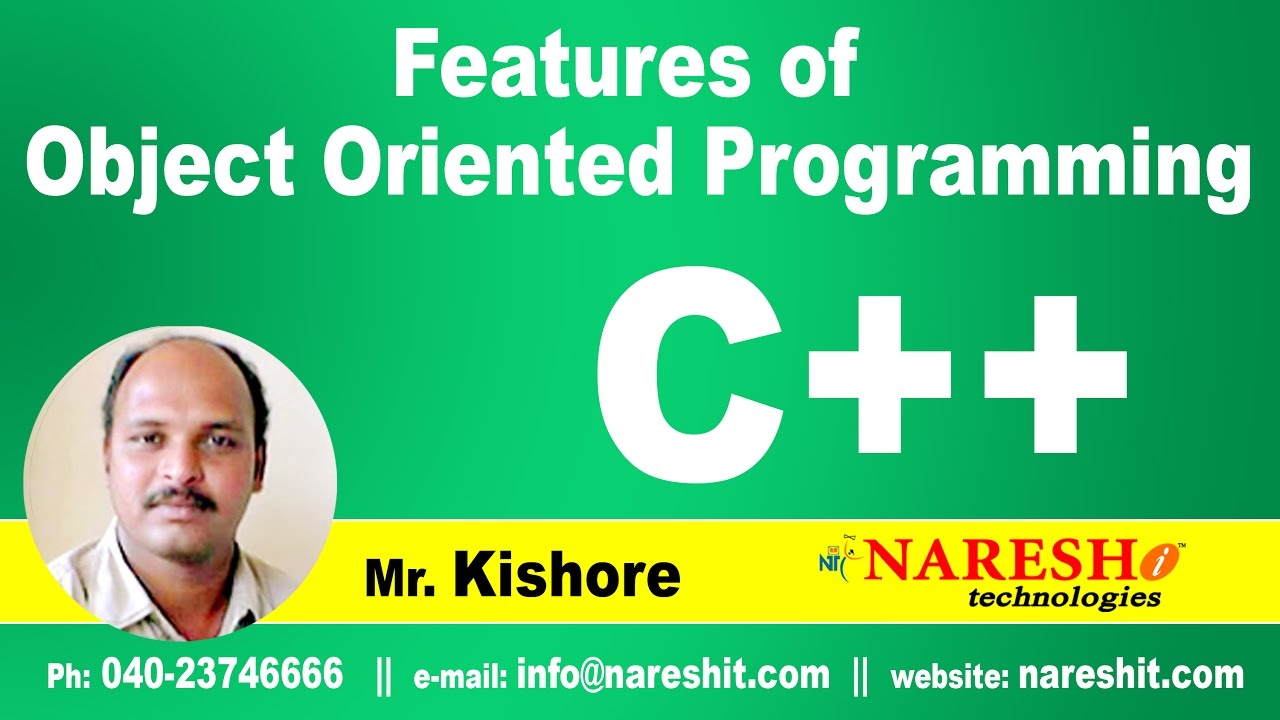
Features of Object Oriented Programming Part 2 | C ++ Tutorial | Mr. Kishore
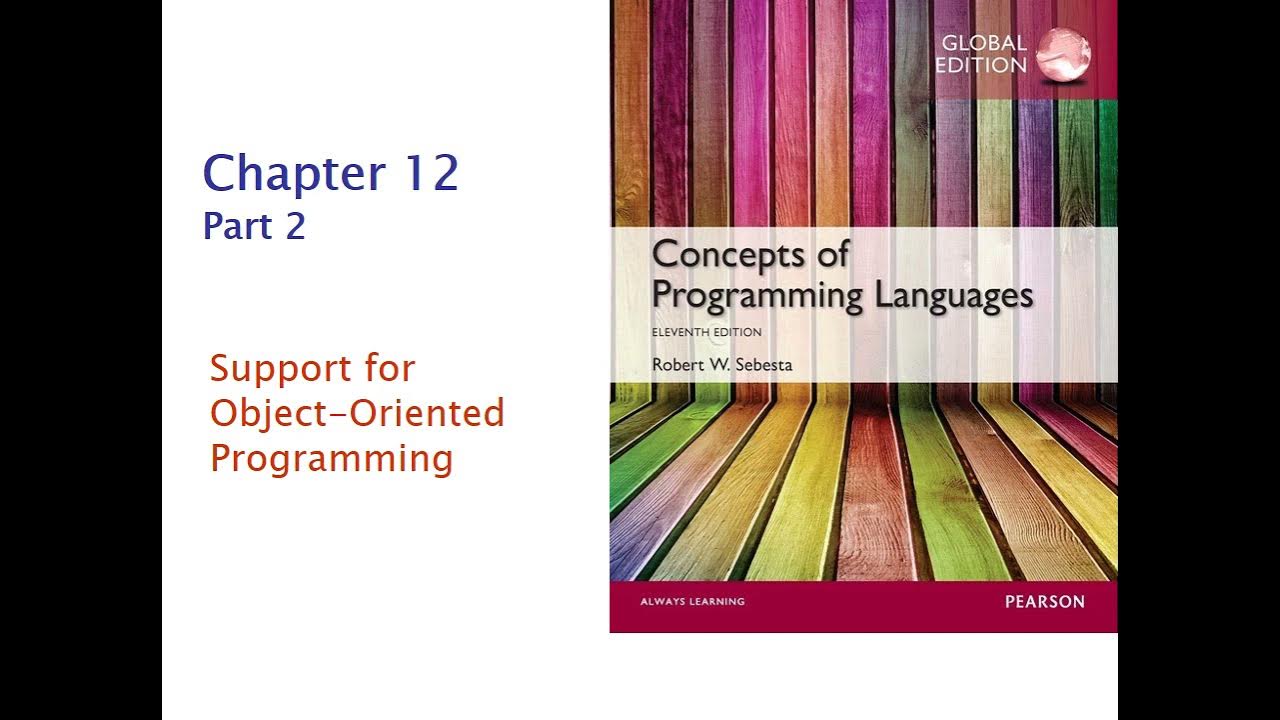
COS 333: Chapter 12, Part 2
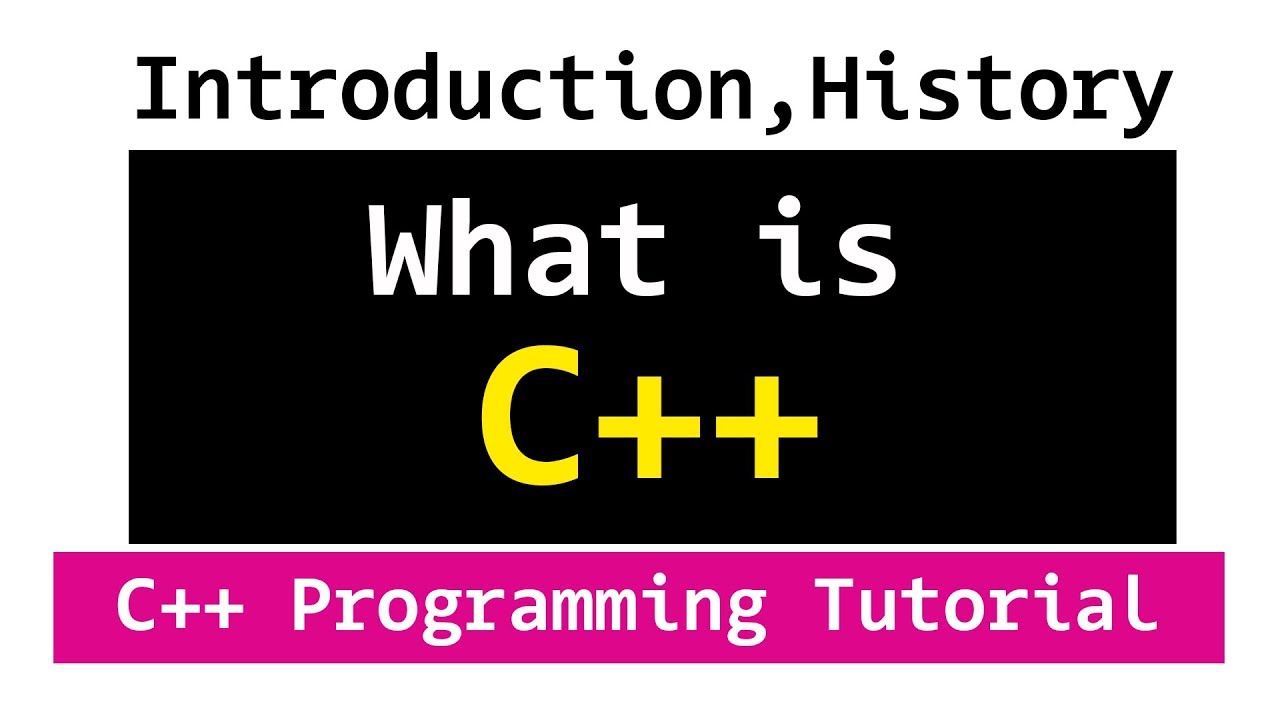
What is C++, Its Introduction and History | CPP Programming Video Tutorial
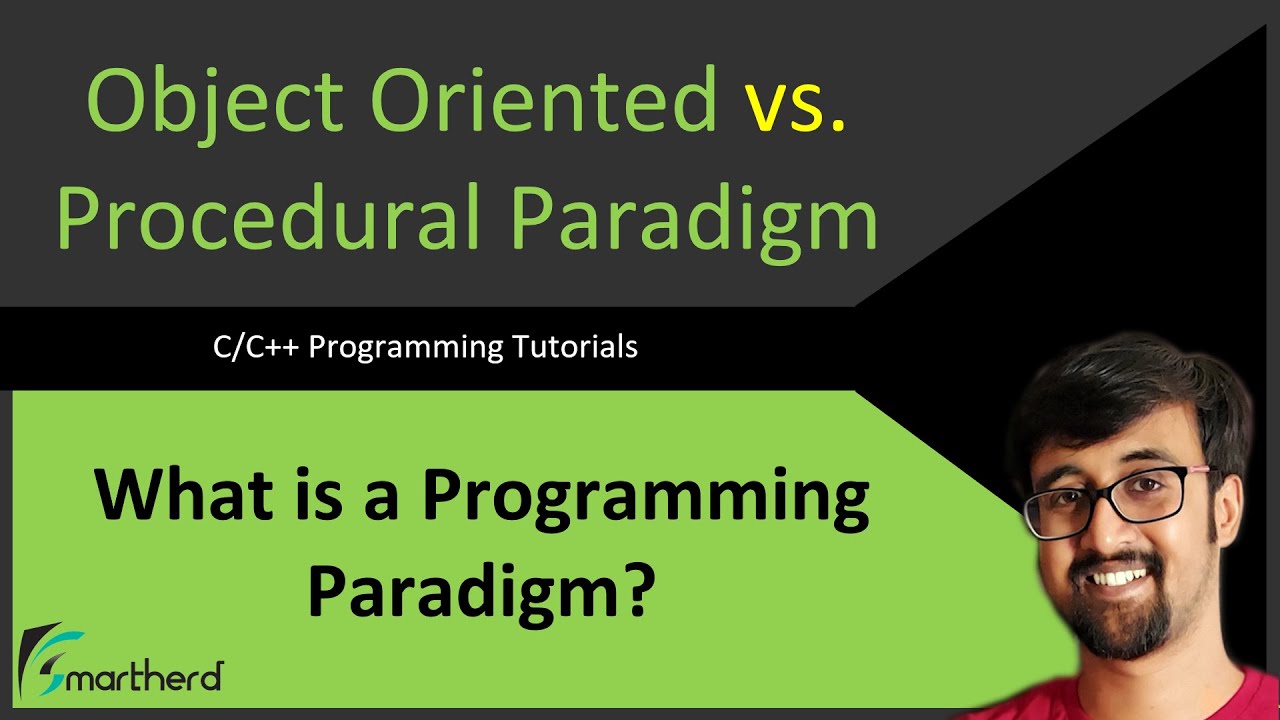
Object Oriented vs. Procedural Programming Paradigm
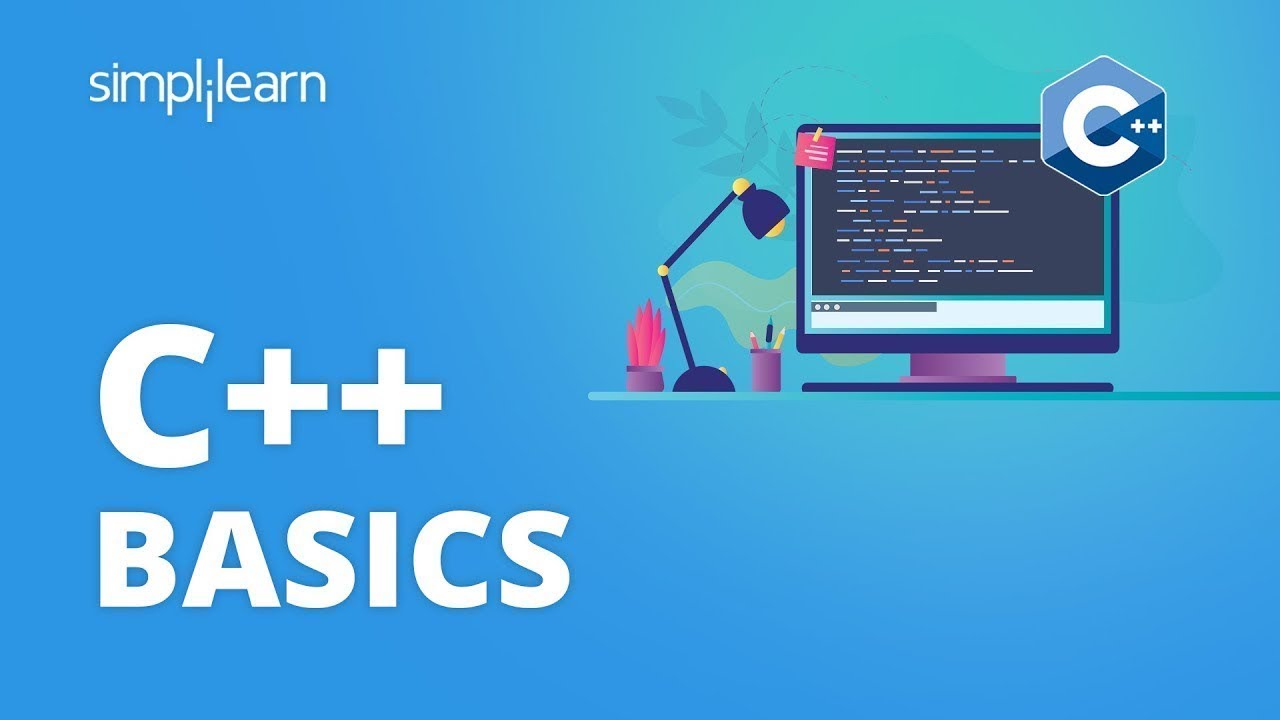
C++ Tutorial For Beginners | C++ Programming | C++ | C++ Basics | C++ For Beginners | Simplilearn
5.0 / 5 (0 votes)