C++ Tutorial For Beginners | C++ Programming | C++ | C++ Basics | C++ For Beginners | Simplilearn
Summary
TLDRThis tutorial introduces the fundamentals of C++ programming, starting with the 'Hello World' program and progressing to data types, variables, arrays, strings, and control structures like if-else and loops. It covers object-oriented features and demonstrates concepts through examples in VS Code. The instructor guides viewers on writing their first C++ program, utilizing arrays, strings, and functions, while emphasizing the language's versatility and intermediate nature between high and low-level programming.
Takeaways
- 😀 C++ is a popular programming language introduced by Bjarne Stroustrup in 1979, initially called 'C with classes'.
- 📘 C++ is a general-purpose, case-sensitive, pre-compiled language that combines features of both high-level and low-level languages.
- 🔧 C++ supports object-oriented features such as encapsulation, inheritance, and polymorphism, as well as procedural and functional programming paradigms.
- 👋 The 'Hello World' program is a traditional first program for beginners in C++, demonstrating basic syntax and output using `cout`.
- 📚 Header files like `#include <iostream>` are used to import features into a C++ program, and `using namespace std;` allows access to the standard library.
- 🔢 Data types in C++ include boolean, character, integer, and float, which define the kind of data a variable can store.
- 🔑 Variables are declared with a data type followed by a chosen name, such as `int data;` for an integer variable.
- 🔍 Arrays in C++ are collections of similar elements stored in contiguous memory locations, allowing multiple values of the same type to be stored in a single variable.
- 📝 Strings in C++ can be represented as C-style strings (arrays of characters) or string objects from the standard library, which facilitate text manipulation.
- 🔄 Conditional statements like `if` and `else` are used to execute code based on conditions, while loops such as `for` and `while` allow for repeated code execution.
- 🛠 Functions in C++ are blocks of code designed to perform specific tasks and can be called with parameters to execute repeated code segments efficiently.
Q & A
Who introduced the C++ programming language and in what year?
-C++ was introduced by Bjarne Stroustrup in the year 1979.
What was the initial name of C++ before it was renamed?
-Initially, C++ was called 'C with classes' as it was an extension to the C language.
Why is C++ considered an intermediate level language?
-C++ is considered an intermediate level language because it contains features of both high-level and low-level languages.
What are the three main features of object-oriented programming supported by C++?
-C++ supports the features of object-oriented programming such as encapsulation, inheritance, and polymorphism.
What is the purpose of the 'iostream' header file in C++?
-The 'iostream' header file in C++ is used to import features into the program, including functions for input and output operations.
What does 'using namespace std;' mean in C++?
-'using namespace std;' in C++ means that the program is utilizing all the components within the standard library.
What is the significance of the 'main' function in a C++ program?
-The 'main' function is significant in C++ as it is the entry point from where the execution of the program starts.
Can you explain the concept of data types in C++?
-In C++, data types act as keywords that define the kind of value a variable can hold, such as boolean, character, integer, and float.
What is an array in C++ and how is it declared?
-An array in C++ is a collection of similar kind of elements stored in contiguous memory locations. It is declared by mentioning the data type, followed by the array name and the number of elements within brackets.
How are strings represented in C++?
-In C++, strings can be represented as a collection of characters surrounded by double quotes. There are two ways to create a string: using C-style strings (arrays of characters) or using string objects from the standard library.
What is the difference between 'if' and 'if-else' statements in C++?
-The 'if' statement is used to execute a block of code if a condition is true. The 'if-else' statement includes an 'else' block that executes if the condition in the 'if' statement is false.
Can you describe the syntax and purpose of a 'for' loop in C++?
-A 'for' loop in C++ is a repetition control structure used to repeat a block of code for a fixed number of times. Its syntax includes an initialization, a condition to determine when to end the loop, and an updation part to update the loop variables.
What is a 'while' loop used for in C++ and what is its syntax?
-A 'while' loop in C++ is used when the exact number of repetitions is not known. It repeats the statements as long as the given condition is true. The syntax includes the 'while' keyword followed by the condition in brackets.
What is a function in C++ and how can it be defined?
-A function in C++ is a group of statements designed to perform a specific task. It can be defined by specifying the return type, function name, and parameters, followed by the code to be executed inside the function.
How can you find the number of even and odd elements in an array using C++?
-You can use a 'for' loop to iterate through the array elements and an 'if-else' statement to check if an element is even or odd. Increment the respective counters for even and odd elements based on the condition.
What is the purpose of the 'push_back' and 'pop_back' functions in C++ strings?
-The 'push_back' function is used to add an element at the end of a string, while the 'pop_back' function is used to remove the last element from the string.
Outlines
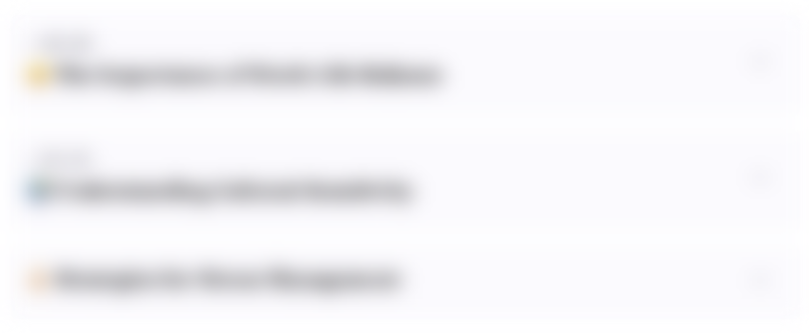
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
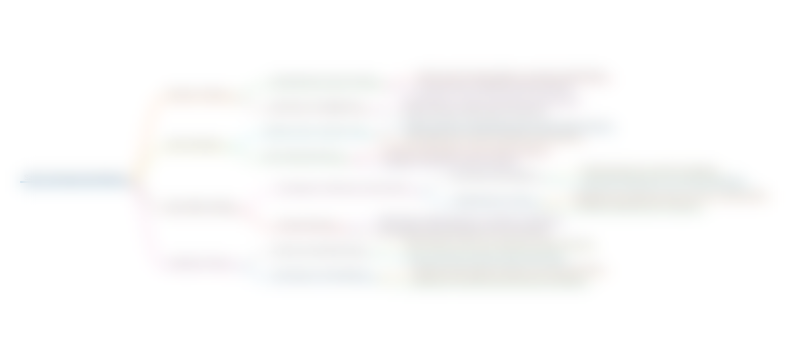
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
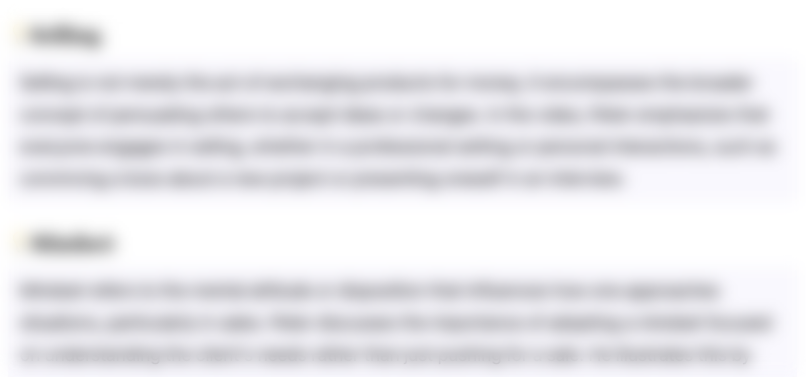
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
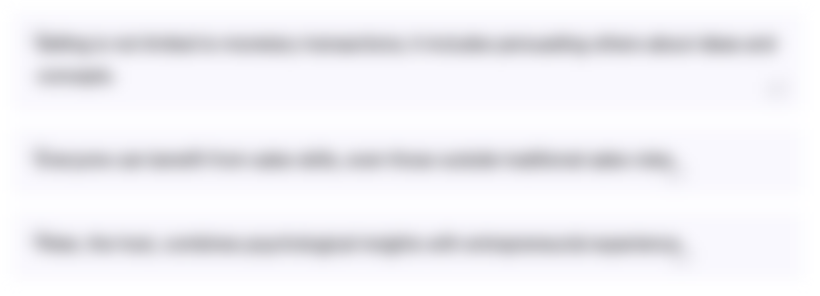
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
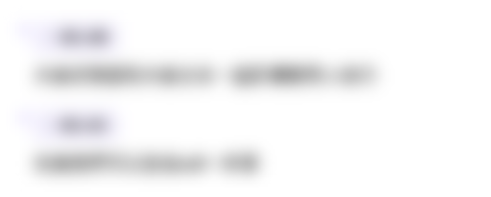
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
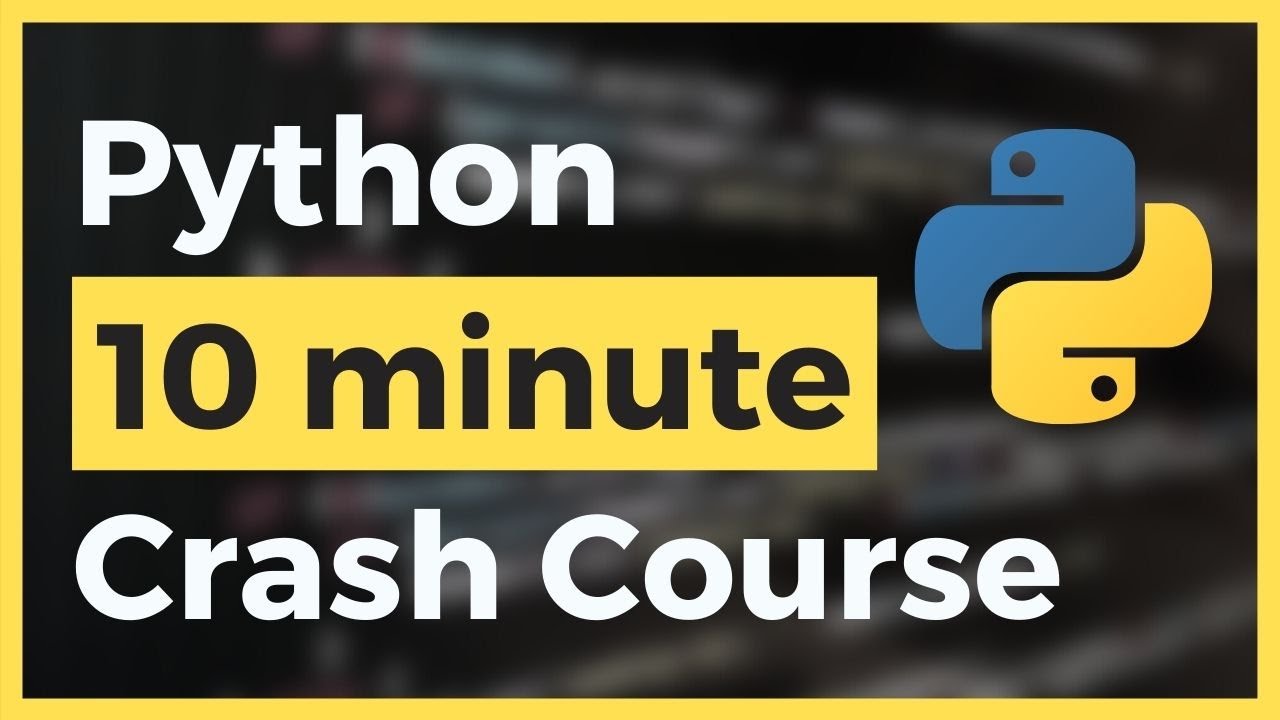
Learn Python in Less than 10 Minutes for Beginners (Fast & Easy)
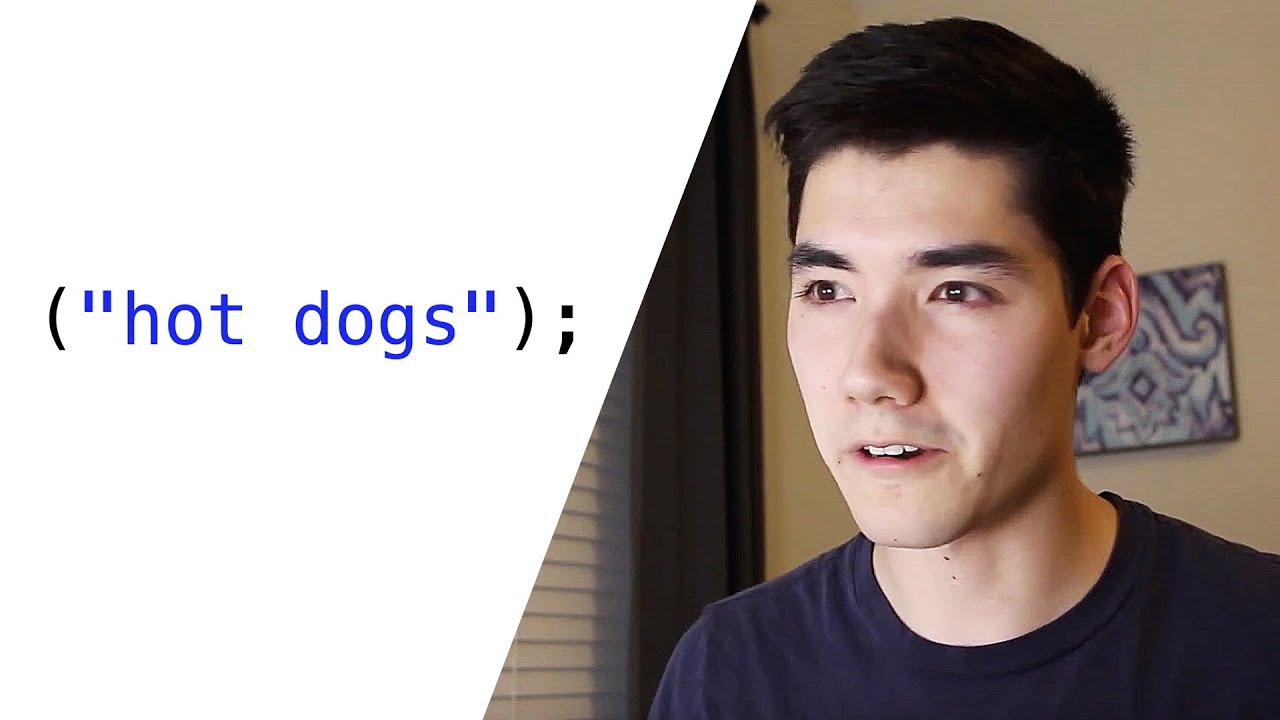
Learn Java in 14 Minutes (seriously)
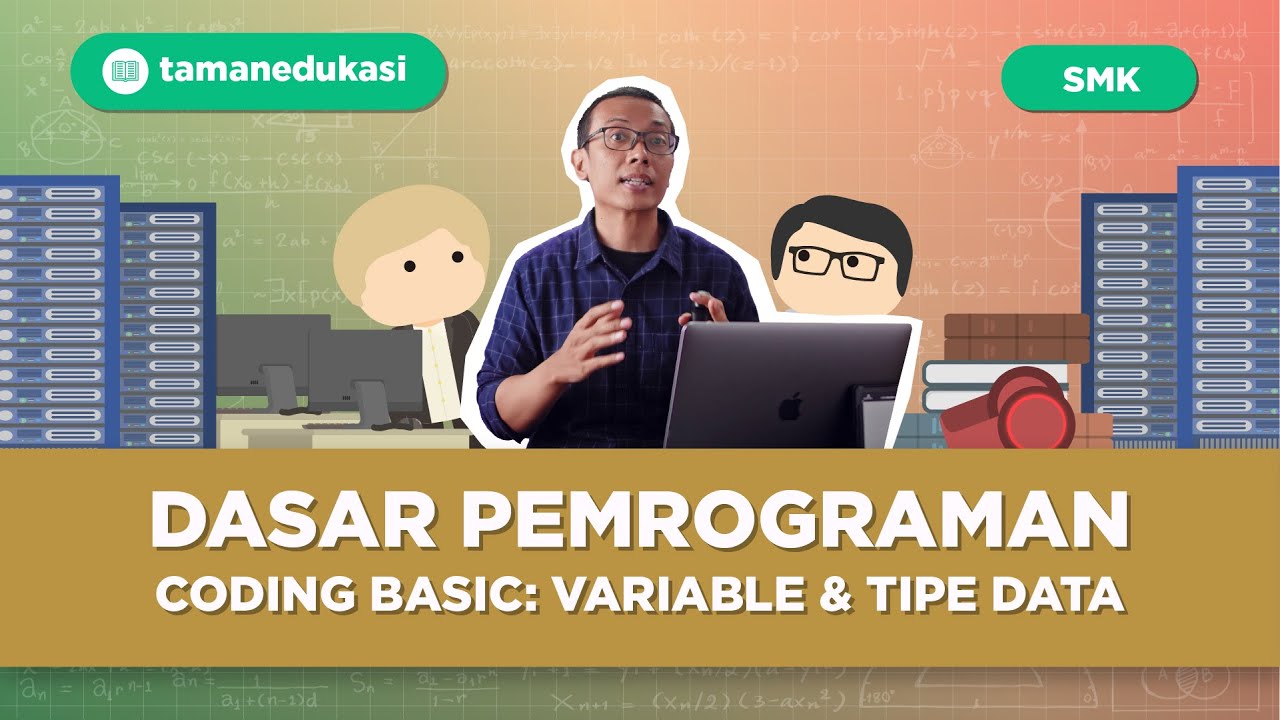
VARIABEL dan TIPE DATA dalam pemrograman yang penting untuk diketahui

Variables & Data Types In C: C Tutorial In Hindi #6
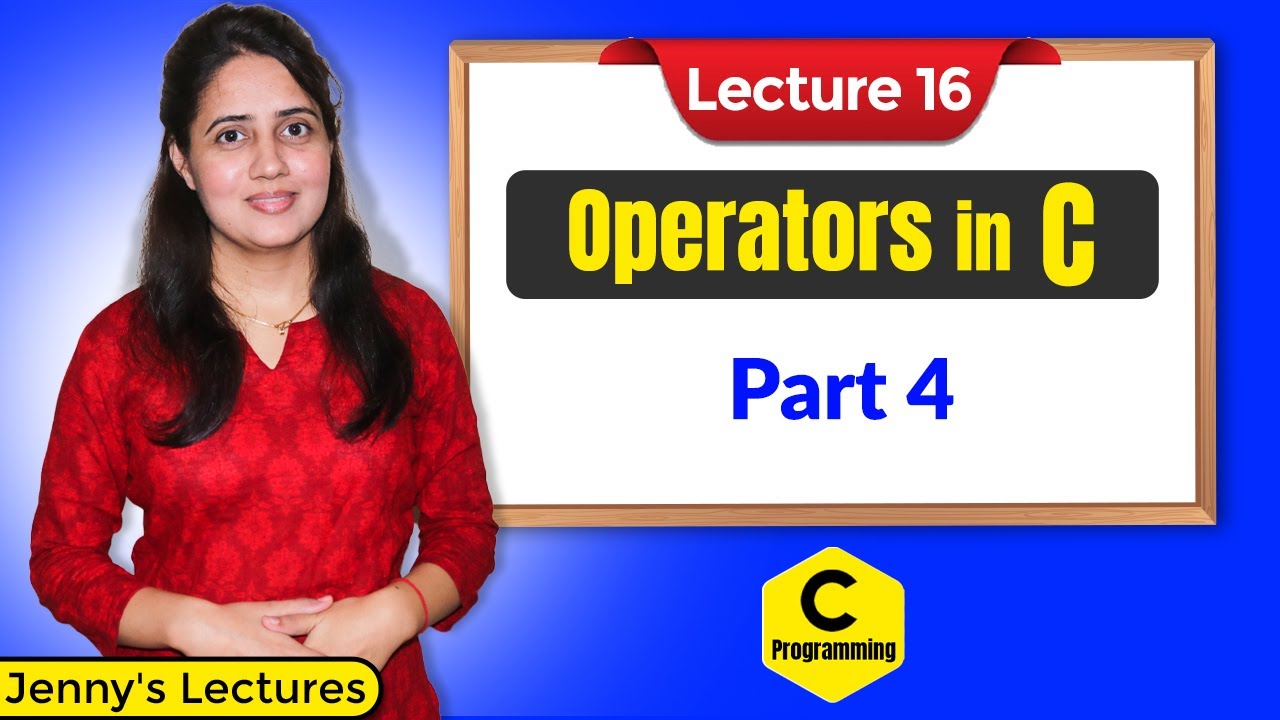
C_16 Operators in C - Part 4 | C Programming Tutorials
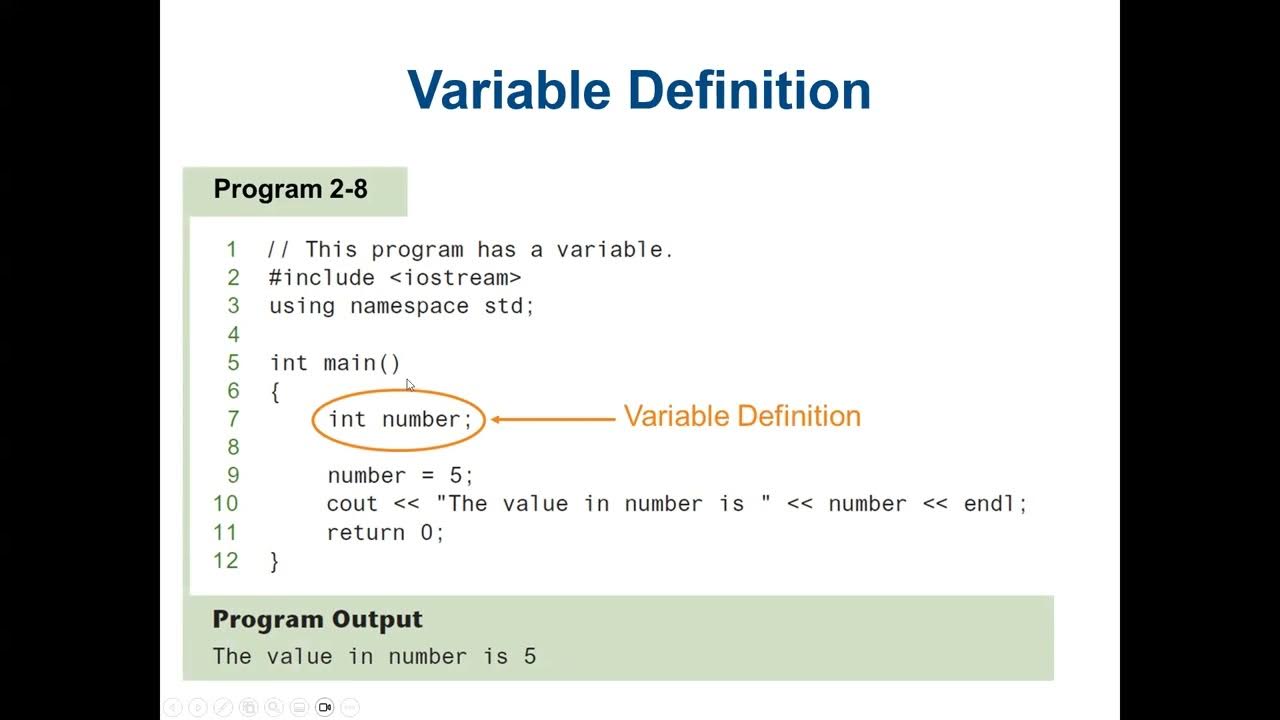
Introduction to C++, The Parts of a C++ Program, Identifier Naming Rules, output statements
5.0 / 5 (0 votes)