Object Oriented Programming Features Part 4 | C ++ Tutorial | Mr. Kishore
Summary
TLDRIn this Nourish 80 video, Kishore explores the concept of objects in C++, explaining how they are created and how memory allocation occurs. He clarifies that classes are logical representations, while objects are their physical counterparts, requiring memory. Kishore also discusses the two ways to define member functions: inside or outside the class, emphasizing the importance of not including complex expressions within inline functions. The video serves as an educational guide on object-oriented programming, highlighting encapsulation as a key feature that binds data members and functions into a cohesive unit.
Takeaways
- 📘 The concept of a class in C++ is an extension of the C structure, allowing for the storage of various types of variables and member functions.
- 🔑 To access class members, an object of the class must be declared because the class itself is a logical representation and does not allocate memory until objects are defined.
- 🏷️ By default, all class members are private if no access specifier is mentioned, adhering to the principle of encapsulation.
- 🔄 Member functions can be defined inside or outside the class, with definitions inside the class being inline functions and not suitable for complex expressions or loops.
- 📝 When defining member functions outside the class, the scope operator (::) is used to indicate that the function belongs to the class.
- 🔑 To call member functions or access class members, at least one object of the class must be declared, as objects are the physical representation of the class.
- 📚 An object is defined as a class variable, an instance of a class, and the physical representation of a class, requiring memory allocation.
- 🔍 Each object created from a class can store data separately, allowing for the management of distinct data instances.
- 🔑 Encapsulation is a key feature of OOP, binding data members and member functions into a single unit called a class, promoting data integrity and abstraction.
- 📈 The script provides a foundational understanding of classes and objects in C++, emphasizing the importance of memory allocation and access methods for class members.
- 🎓 The explanation of class and object definitions, along with the concept of encapsulation, sets the stage for further exploration of OOP features in subsequent sessions.
Q & A
What is the primary function of a class in C++?
-A class in C++ serves as an extension of the C structure, allowing for the storage of different types of variables and member functions within it.
Why is it necessary to declare an object from a class?
-Declaring an object from a class is necessary to access the class members because memory is not allocated when the class is declared; it is only allocated when objects are defined.
What is the default access specifier for class members if none is specified?
-If no access specifier is mentioned, by default, all class members become private.
What are the two ways to define member functions in a class?
-Member functions can be defined either inside the class, which makes them inline functions, or outside the class, which does not make them inline.
Why should looping statements or complex expressions be avoided when defining member functions inside the class?
-Looping statements or complex expressions should be avoided in inline member functions because they can lead to code bloat and are not suitable for inlining.
What is the purpose of the scope operator (::) when defining a member function outside the class?
-The scope operator (::) is used to indicate that the function defined outside the class belongs to the class, clarifying the association between the function and the class.
How does the creation of an object relate to memory allocation in C++?
-When an object is created from a class, memory is allocated for that object, making it the physical representation of the class, whereas the class itself is a logical representation.
What is the difference between a class and an object in terms of memory allocation?
-A class, when declared, does not allocate memory. Memory is allocated only when objects (instances of the class) are created.
How many objects can be declared from a single class?
-You can declare any number of objects from a single class, each object having its own separate memory allocation for the class members.
What is encapsulation in the context of object-oriented programming?
-Encapsulation is the process of binding data members and member functions into a single unit called a class, thus encapsulating the data and the methods that operate on the data within the class.
Why is encapsulation an important feature in object-oriented programming?
-Encapsulation is important because it promotes the bundling of data and methods that operate on the data, providing data hiding and abstraction, which simplifies the management and maintenance of code.
Outlines
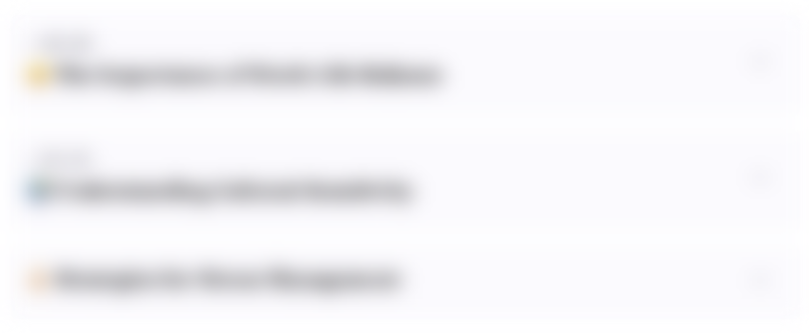
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
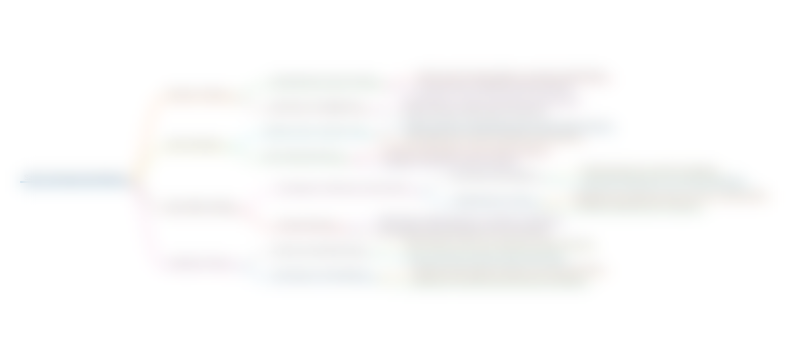
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
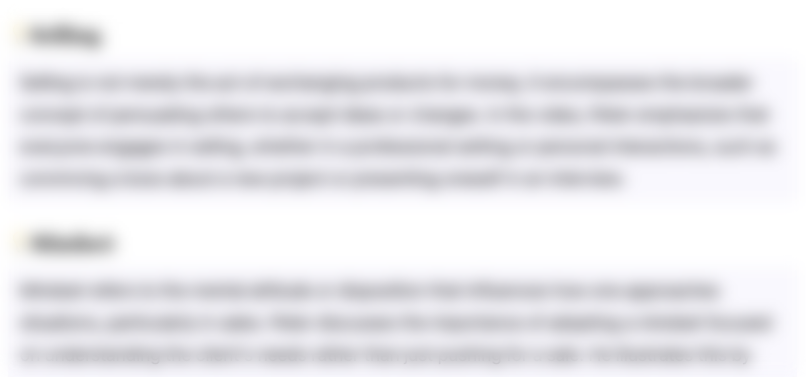
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
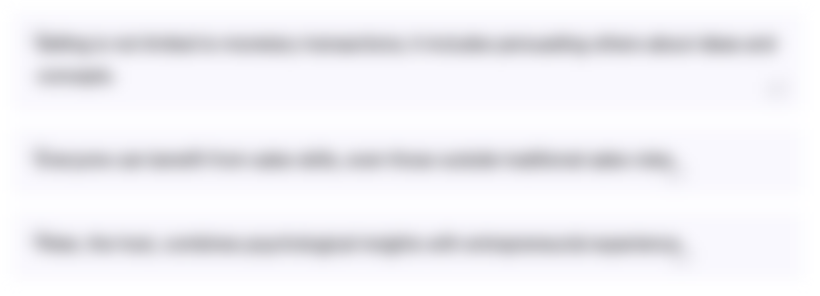
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
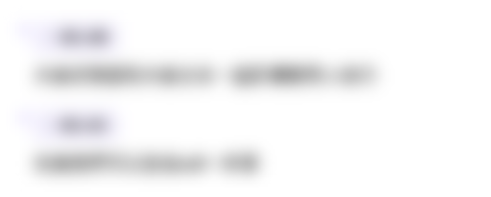
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
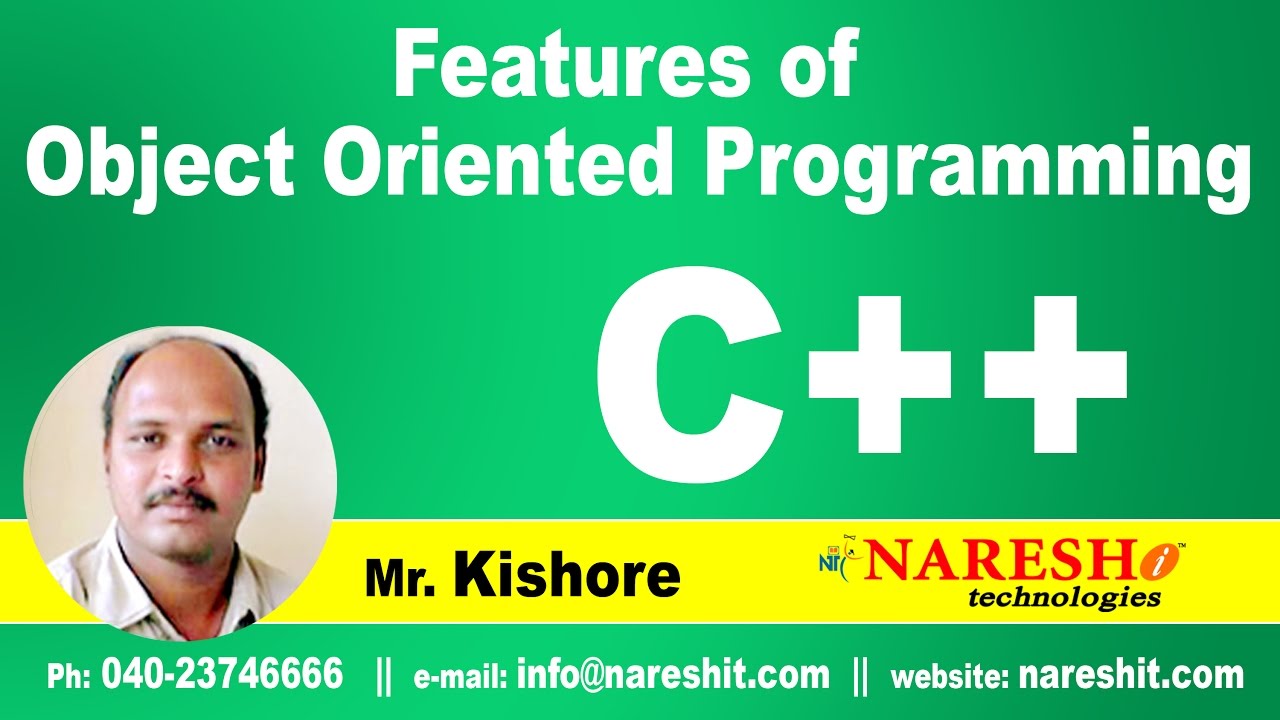
Object Oriented Programming Features Part 3 | C ++ Tutorial | Mr. Kishore
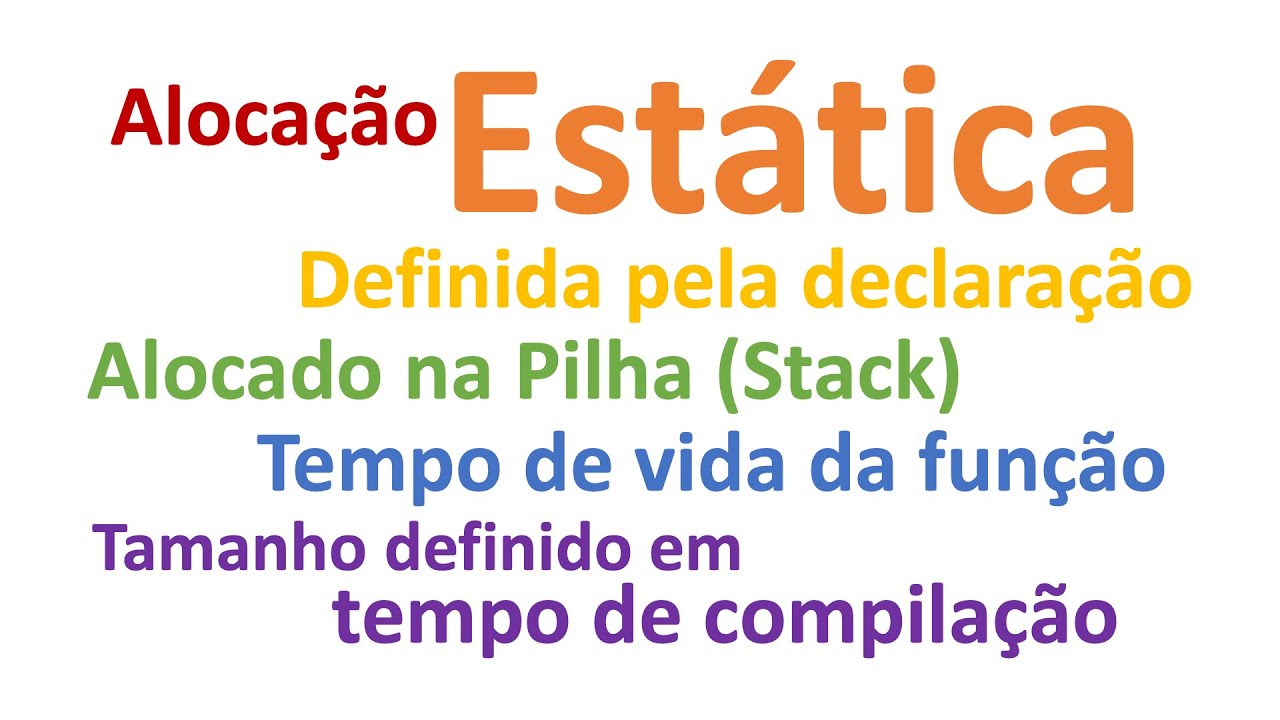
EDB1 IMD UFRN : Alocação Estática de Memória
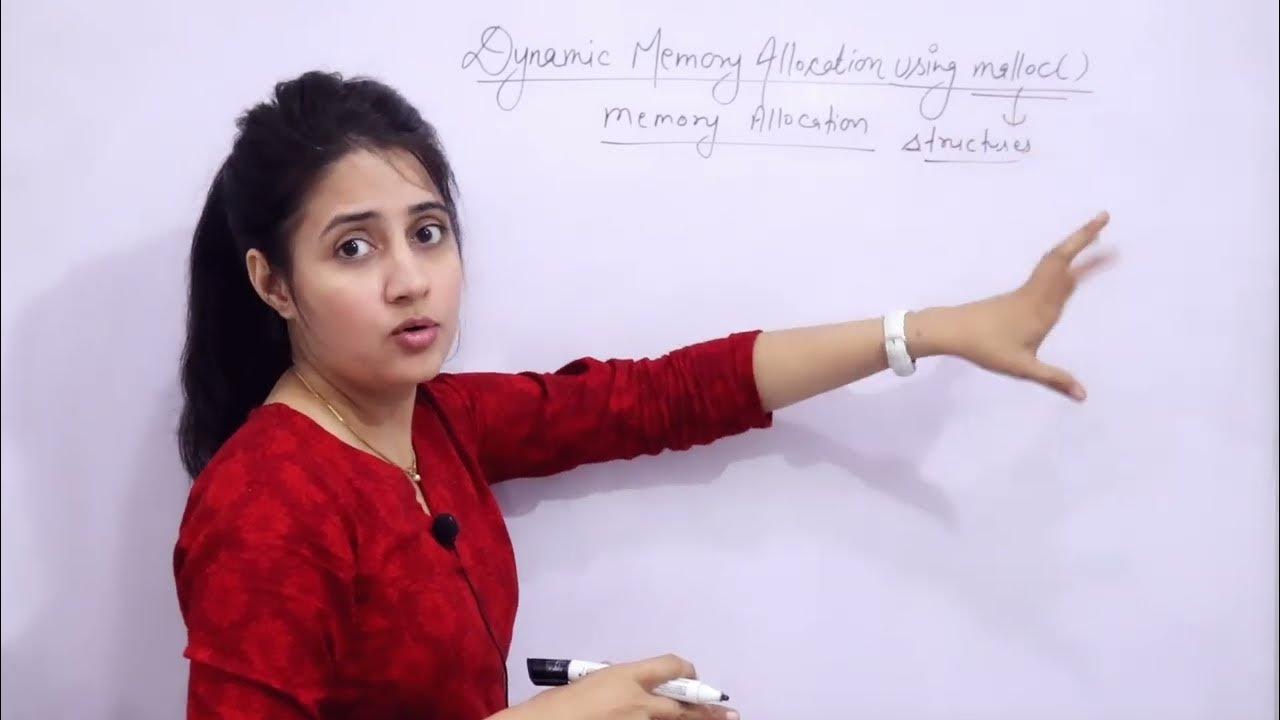
C_133 Dynamic Memory Allocation using malloc() | C Language Tutorials

C_132 Introduction to Dynamic Memory Allocation in C | SMA vs DMA

C_135 Dynamic Memory Allocation using realloc() | C Language Tutorials
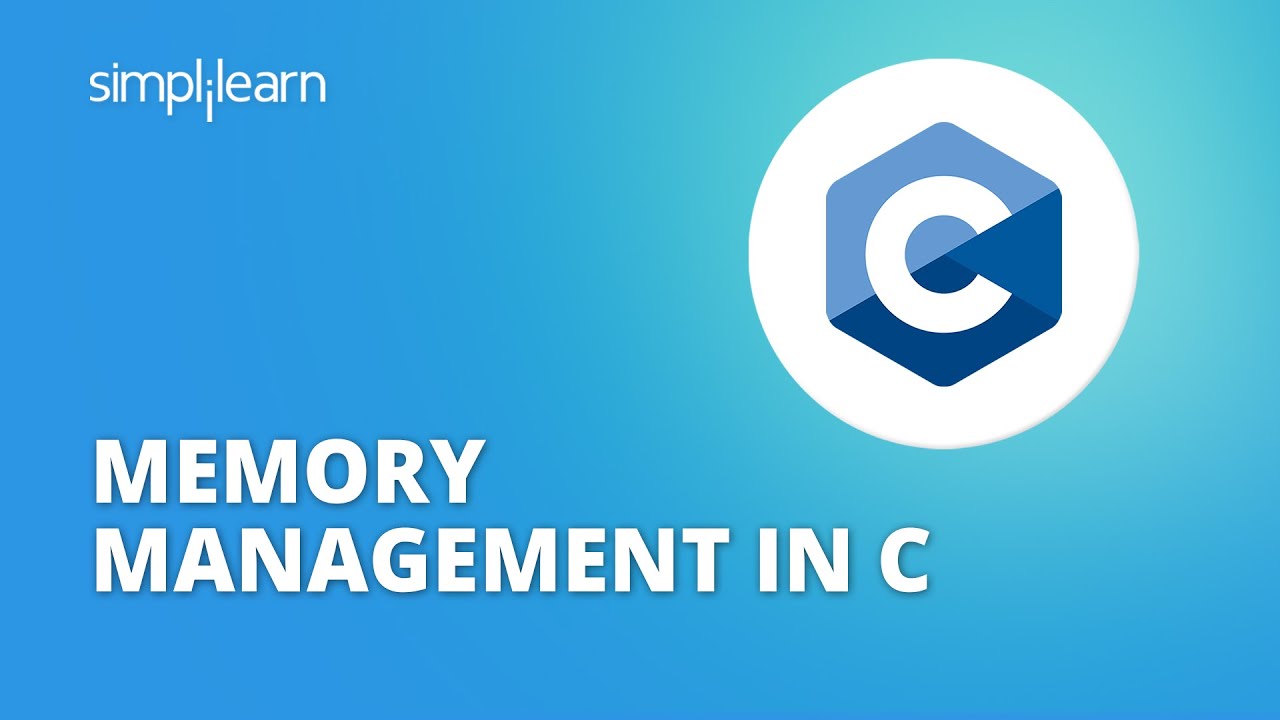
Dynamic Memory Allocation In C | C Memory Management Explained | C For Beginners | Simplilearn
5.0 / 5 (0 votes)