JAVA TECHNICAL QUESTION & ANSWERS FOR INTERVIEW PART - V ( MULTITHREADING - II )
Summary
TLDRThis video focuses on Java multithreading concepts, particularly thread priority, synchronization, and inter-thread communication. It explains how thread priorities influence the order in which threads are executed, with methods like `setPriority` and `getPriority`. The discussion also covers synchronization techniques, the use of monitor objects to ensure mutual exclusivity, and how methods like `wait`, `notify`, and `notifyAll` coordinate thread actions. Additional topics include deadlock, daemon threads, and critical sections in Java, offering a comprehensive guide to managing multithreaded operations in Java applications.
Takeaways
- 🔧 Thread priority helps the scheduler decide the order of thread execution based on importance.
- 📊 Thread priority ranges from 1 (minimum) to 10 (maximum), with default values like 5 for normal priority and 7 for higher priorities.
- ⚙️ Use the `setPriority` method to assign thread priority and `getPriority` to retrieve the current priority of a thread.
- 🔒 Synchronization ensures that only one thread accesses a shared resource at a time, preventing conflicts.
- 🚦 A monitor is an object used for exclusive locking, allowing only one thread to access a resource while others wait.
- ⏳ The `yield` method allows threads with the same priority to share execution time, promoting fairness in thread scheduling.
- 🚨 The `interrupt` method halts a thread's execution, throwing an InterruptedException if an issue occurs.
- 👻 A daemon thread runs in the background, supporting the program without blocking its execution.
- 🔐 Synchronized blocks or methods prevent multiple threads from entering a critical section simultaneously, protecting shared resources.
- 🤝 Inter-thread communication helps threads coordinate actions when sharing resources, improving collaboration and efficiency.
Q & A
What is thread priority in Java?
-Thread priority is used by the thread scheduler to decide when each thread should be allowed to run. It is assigned based on the task's importance, allowing more urgent threads to be executed first.
Which method is used to set the priority for a thread in Java?
-The method used to set the priority for a thread in Java is `setPriority(int newPriority)`.
What is the valid range for thread priority values in Java?
-The valid range for thread priority values is from 1 to 10, where 1 is the minimum priority and 10 is the maximum priority.
What are the default priority values for threads in Java?
-By default, a thread has a priority value of 5, which is the normal priority. The constant values for minimum and maximum priority are 1 and 10 respectively.
What is synchronization in Java multithreading?
-Synchronization ensures that only one thread can access a shared resource at a time. It prevents multiple threads from accessing a resource simultaneously, avoiding data inconsistency.
What is a monitor in Java synchronization?
-A monitor is an object that acts as a mutually exclusive lock. It allows only one thread to access a shared resource at a time. Other threads wait until the current thread releases the lock.
What is the purpose of the `yield` method in Java?
-The `yield` method suggests that the current thread should pause its execution to allow other threads with the same priority a chance to execute.
What does the `interrupt` method do in a thread?
-The `interrupt` method interrupts the normal execution of a thread, causing it to throw an `InterruptedException`, which signals that the thread's flow has been disrupted.
What is a daemon thread in Java?
-A daemon thread is a background thread that does not prevent the program from exiting. It runs behind the scenes, supporting the main application.
How can you prevent multiple threads from accessing a critical section of code simultaneously in Java?
-You can prevent multiple threads from accessing a critical section of code by using synchronized blocks or synchronized methods.
What is a deadlock in Java multithreading?
-A deadlock occurs when two or more threads are waiting for each other to release resources they need, but none of them is willing to release their own resources, causing all of them to be stuck.
What is inter-thread communication in Java?
-Inter-thread communication is a mechanism that allows threads to coordinate their actions when working together, especially when sharing resources.
What are the `wait()`, `notify()`, and `notifyAll()` methods used for in Java threads?
-`wait()` causes a thread to wait until another thread invokes `notify()` or `notifyAll()` on the same object. `notify()` wakes up one waiting thread, while `notifyAll()` wakes up all the waiting threads on the same object.
Outlines
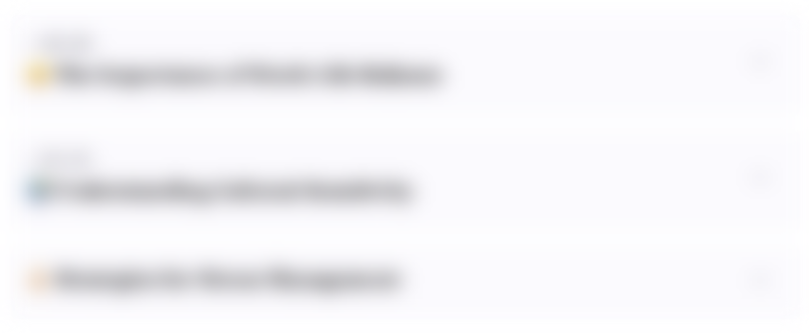
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
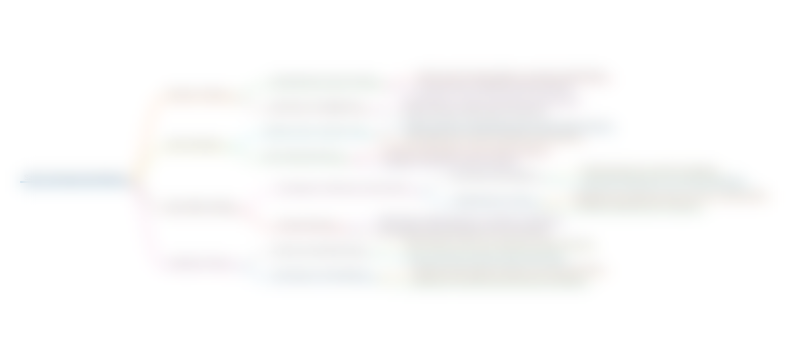
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
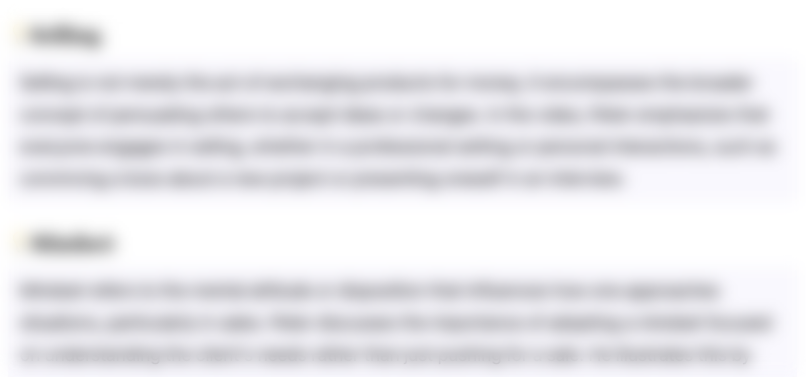
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
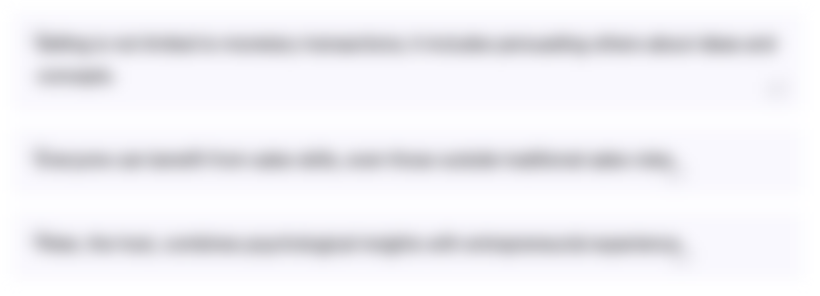
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
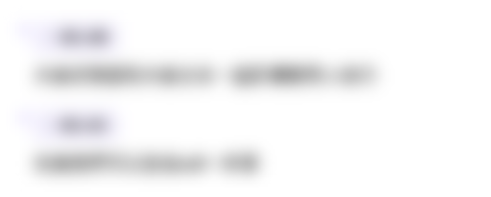
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
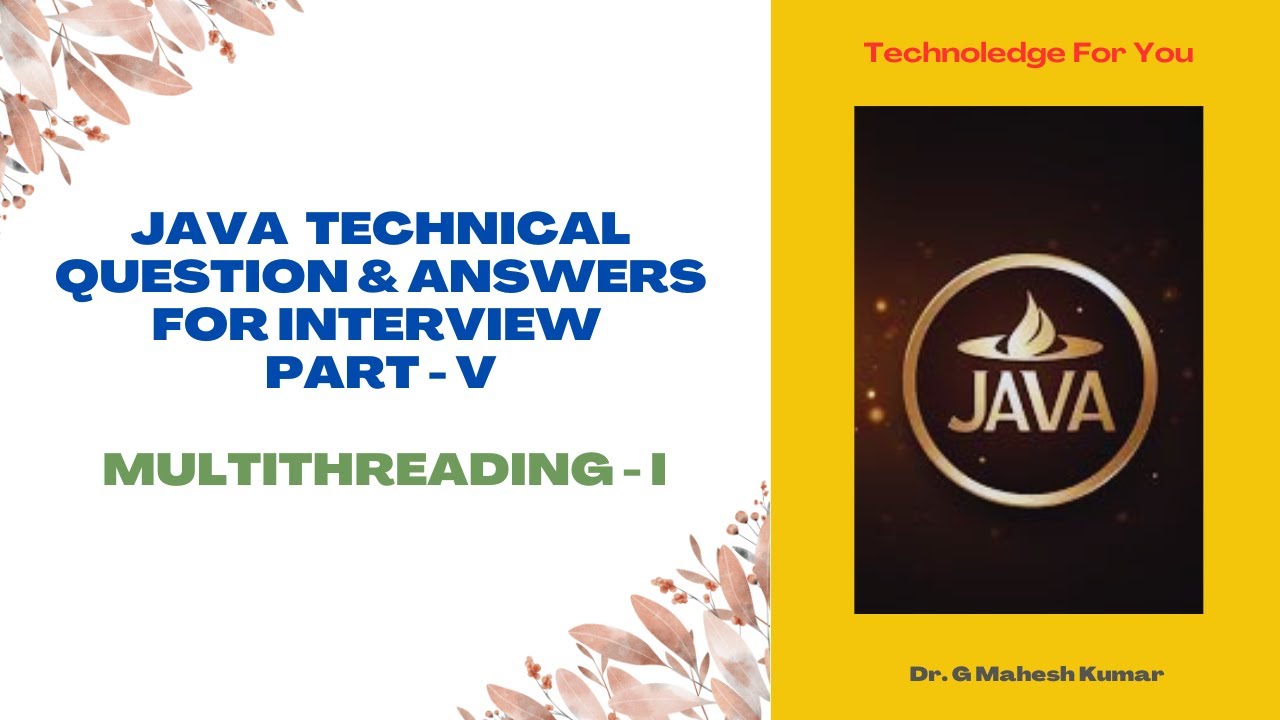
JAVA TECHNICAL QUESTION & ANSWERS FOR INTERVIEW PART - V ( MULTITHREADING - I )

#86 Multiple Threads in Java
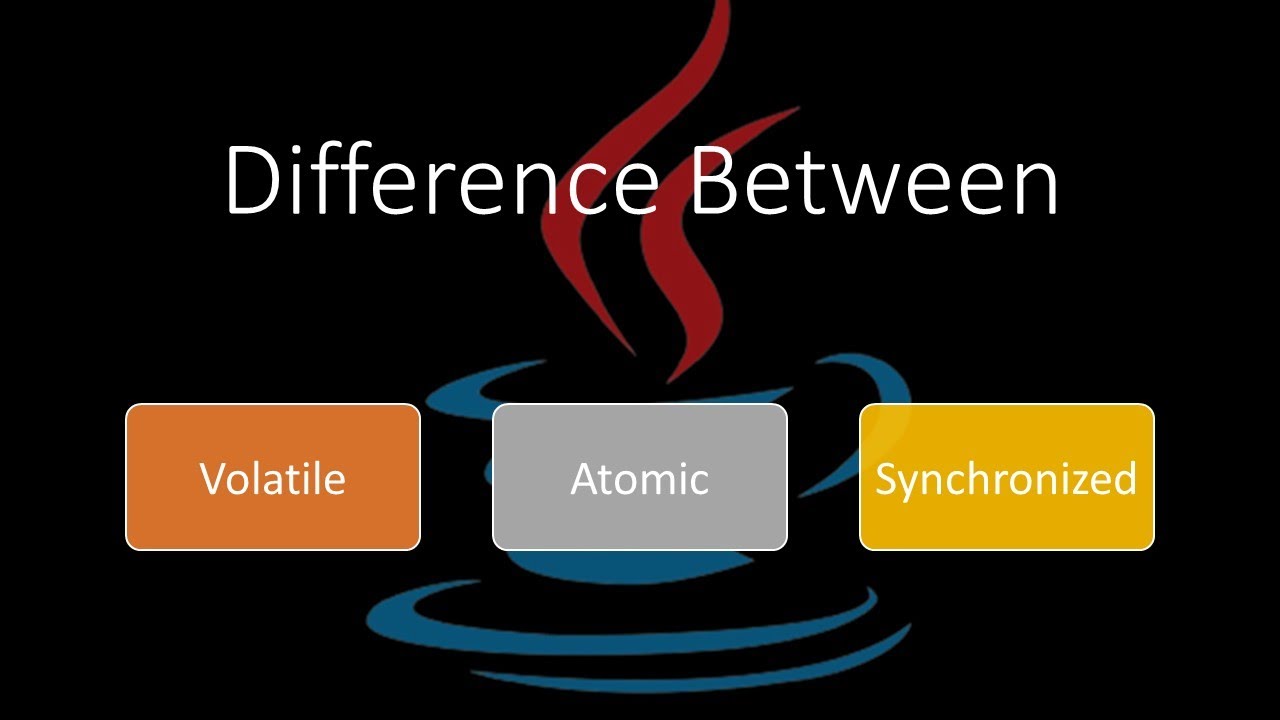
Difference Between Volatile, Atomic And Synchronized in Java | Race Condition In Multi-Threading

Virtual Threads in Java 21

Crack Your Java Interview With Most-Asked Questions | Java Fundamentals
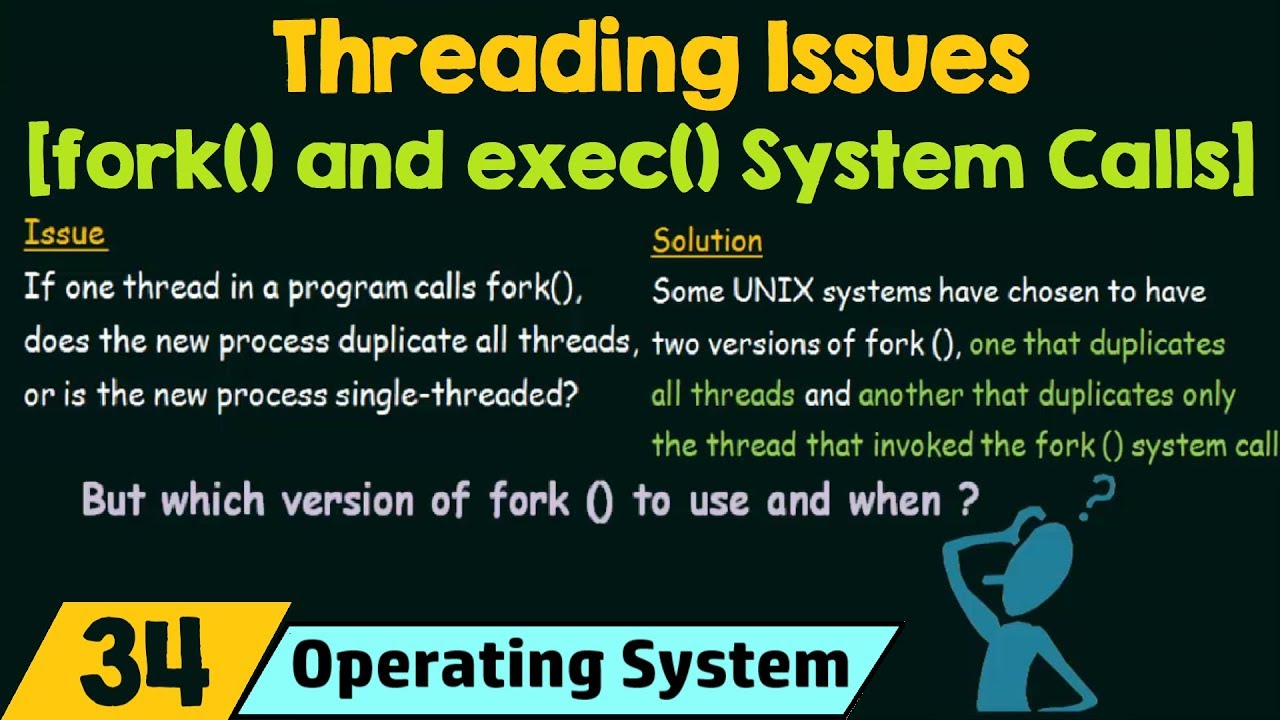
Threading Issues [fork() & exec() System Calls]
5.0 / 5 (0 votes)