Java threads 🧵
Summary
TLDRIn this tutorial, we dive into Java threads, starting with the basics of thread creation, properties, and execution. Learn how the JVM manages threads, with a focus on the main thread and how to interact with it using methods like `Thread.activeCount()`, `Thread.getName()`, and `Thread.setPriority()`. The video covers thread life cycle management, including checking if a thread is alive, using `Thread.sleep()`, and creating custom threads by extending the `Thread` class. We also explore daemon threads, which run in the background, and how to configure their behavior. This is part 1 of a 2-part series on threads and multi-threading in Java.
Takeaways
- 😀 A thread in Java is a virtual CPU that allows an application to execute multiple tasks concurrently, with each thread running in parallel with the main thread.
- 😀 The Java Virtual Machine (JVM) controls thread execution, and threads have priority levels. Higher priority threads are executed before lower priority ones.
- 😀 The main thread, which starts the Java program, can be checked and managed using methods like `Thread.currentThread().getName()` and `Thread.currentThread().setName()`.
- 😀 You can monitor the number of active threads in a program using `Thread.activeCount()`. This can be useful for debugging or tracking resource usage.
- 😀 Threads in Java also have a priority, ranging from 1 (lowest) to 10 (highest). The main thread by default has a priority of 5.
- 😀 A thread's state (alive or dead) can be checked with the `isAlive()` method, which returns `true` if the thread is currently executing.
- 😀 Java allows you to make a thread sleep for a specified amount of time with the `Thread.sleep()` method. This is useful for controlling the flow of execution, like implementing countdowns.
- 😀 You can create new threads in Java by extending the `Thread` class and overriding its `run()` method, which defines the task the thread will perform.
- 😀 To actually start a new thread, the `start()` method must be called; otherwise, the thread's `run()` method won't be executed.
- 😀 Threads can be either user threads or daemon threads. Daemon threads run in the background with low priority and are automatically terminated when all user threads finish.
- 😀 The JVM terminates when all user threads are finished, even if daemon threads are still running, making daemon threads suitable for background tasks like garbage collection.
Q & A
What is a thread in Java?
-A thread in Java is a unit of execution within a program. It acts like a virtual CPU that can run concurrently with other threads, allowing for parallel execution of code.
How does the Java Virtual Machine (JVM) manage multiple threads?
-The JVM allows multiple threads to run concurrently by managing their execution. Each thread has its own priority, and threads with higher priorities are executed before those with lower priorities.
What is the role of the 'main' thread in a Java program?
-The 'main' thread is the initial thread that starts when the JVM is launched. It calls the main method of the program and begins execution. Once it completes, the program stops unless other user threads are running.
How can you check the number of active threads in a Java program?
-You can use the `Thread.activeCount()` method to check how many threads are currently active in the program.
What does the `Thread.currentThread().getName()` method do?
-The `Thread.currentThread().getName()` method returns the name of the current executing thread. By default, the main thread is named 'main'.
How can you change the name of a thread in Java?
-You can change the name of the current thread using the `Thread.currentThread().setName()` method, where you pass a new name for the thread.
What does the `Thread.sleep()` method do in Java?
-The `Thread.sleep()` method pauses the execution of a thread for a specified amount of time, given in milliseconds. This can be useful for tasks like counting down or delaying execution.
What is the difference between the `start()` and `run()` methods when working with threads?
-The `start()` method is used to begin the execution of a new thread, while the `run()` method contains the code that the thread will execute. Calling `run()` directly does not start a new thread; it executes the code in the current thread.
What are user threads and daemon threads in Java?
-User threads are regular threads that perform tasks and keep the JVM alive until they complete. Daemon threads, on the other hand, are background threads with low priority, typically used for tasks like garbage collection. The JVM terminates when all user threads finish, regardless of daemon threads.
How can you check if a thread is alive in Java?
-You can check if a thread is alive by using the `Thread.isAlive()` method, which returns `true` if the thread is currently running and `false` if it has finished execution.
Outlines
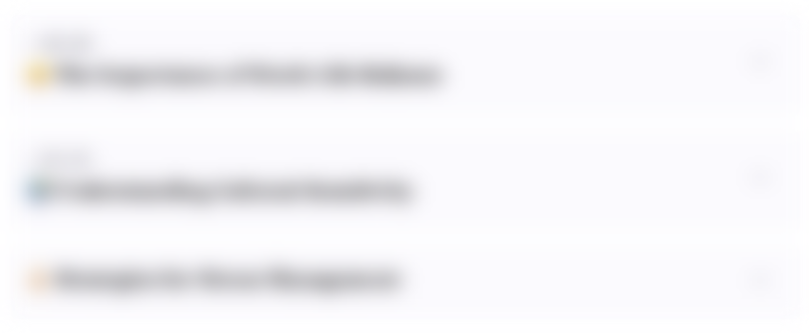
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
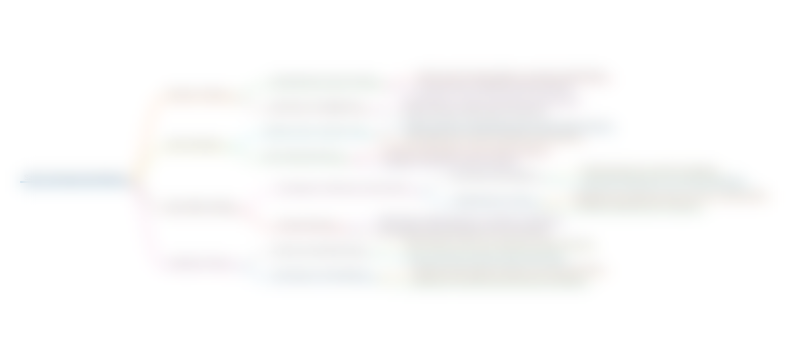
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
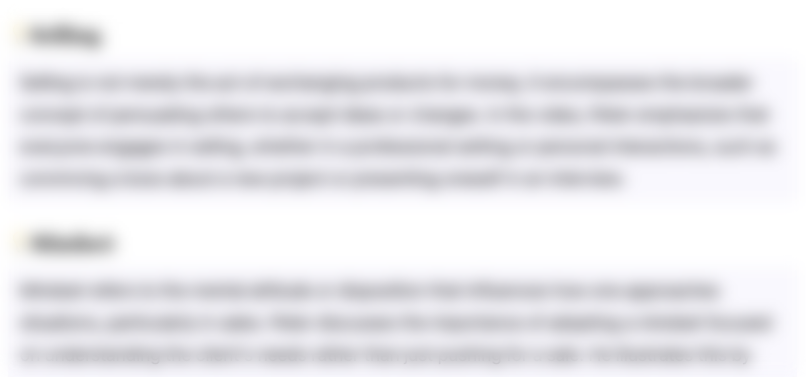
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
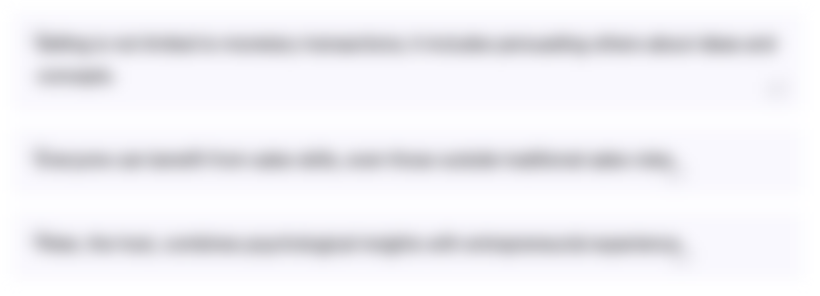
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
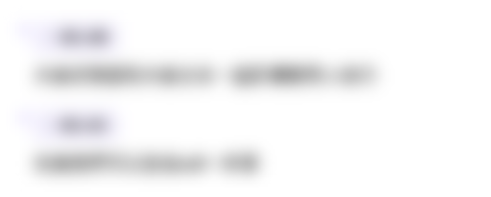
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
5.0 / 5 (0 votes)