JAVA TECHNICAL QUESTION & ANSWERS FOR INTERVIEW PART - V ( MULTITHREADING - I )
Summary
TLDRThis video covers part one of a Java interview topic focused on multithreading. It explains key concepts such as processes and threads, highlighting how multithreading enables processes to run multiple threads concurrently. The video details process-based and thread-based multitasking, thread creation methods, and the lifecycle of a thread in Java. Important methods like `currentThread()`, `getName()`, and `join()` are discussed, alongside thread states such as new, runnable, running, blocked, waiting, and terminated. It concludes with the advantages of multithreading, including improved program performance by utilizing multiple CPUs.
Takeaways
- 📝 A process is a program under execution, transitioning from source code to its final output.
- 💻 Multithreading in Java allows a process to create and execute multiple threads concurrently, sharing resources.
- 🧵 A thread is a lightweight sub-process within a program, using fewer resources than a process, which helps to execute tasks concurrently.
- ⚡ The main advantage of multithreading in Java is improved performance by utilizing multiple CPUs to speed up execution.
- 🛠️ Threads in Java can be created either by extending the Thread class or by implementing the Runnable interface.
- 🏃 The main thread is the default thread that runs when a Java program starts, and it often spawns other child threads.
- 🔍 The `currentThread()` method retrieves the reference of the currently running thread, while `getName()` fetches the thread's name.
- ⏳ The `sleep()` method is used to suspend a thread for a specific period, and the `join()` method waits for other threads to finish before terminating.
- 🛑 The `run()` method serves as the entry point for thread execution, and the `start()` method triggers this execution.
- 🔄 Java threads go through various states, including New, Runnable, Running, Blocked, Waiting, Timed Waiting, and Terminated, based on their execution lifecycle.
Q & A
What is a process in Java?
-A process in Java is a program that is currently under execution. It starts as a source code, which is interpreted and executed, leading to a process that runs through the CPU.
What is multithreading in Java?
-Multithreading in Java is a programming concept that allows a process to create and execute multiple threads concurrently, sharing the process's resources to increase efficiency and performance.
What is multitasking in Java?
-Multitasking in Java is the ability of a program to perform multiple tasks simultaneously, allowing the execution of various tasks in parallel to improve program efficiency.
What is process-based multitasking in Java?
-Process-based multitasking involves executing multiple independent processes simultaneously, with each process running in parallel and independently of others.
What is thread-based multitasking in Java?
-Thread-based multitasking involves creating and managing lightweight sub-processes, called threads, within a program. These threads run concurrently to complete tasks faster and more efficiently.
What is a thread in Java?
-A thread in Java is a lightweight sub-process that runs independently within a program. Since it uses fewer resources, it is more efficient than a full process.
What are the two ways to create a thread in Java?
-The two ways to create a thread in Java are by extending the `Thread` class or by implementing the `Runnable` interface.
What is the main thread in Java, and why is it important?
-The main thread is the default thread that begins executing when a Java program starts. It is important because other child threads are spawned from it, and it usually performs shutdown operations before the program terminates.
What method is used to get the current thread in Java?
-The `currentThread()` method is used to obtain a reference to the currently running thread in Java.
What are the different states of a thread in Java?
-The different states of a thread in Java are: New (created but not started), Runnable (ready to run), Running (currently executing), Blocked (waiting for resources), Waiting (waiting for other threads to complete), Timed Waiting (waiting for a specified time), and Terminated (finished execution).
Outlines
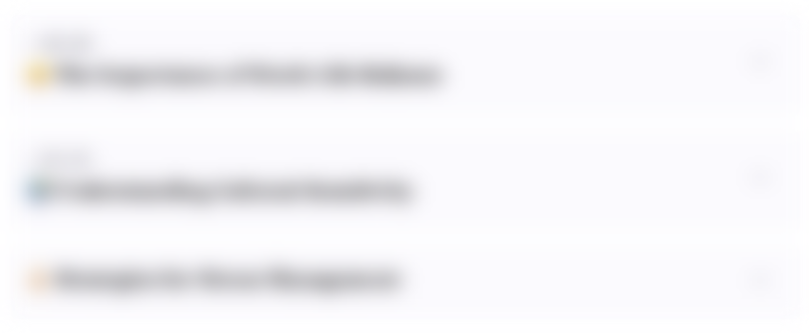
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
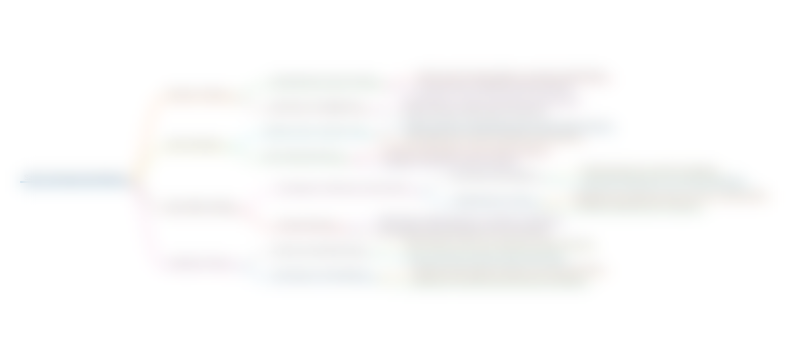
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
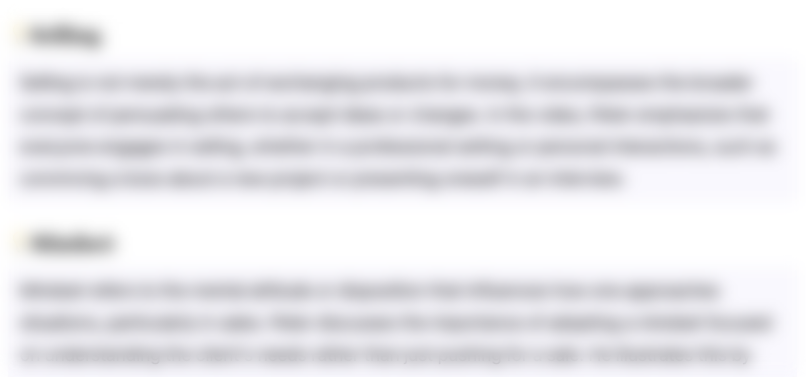
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
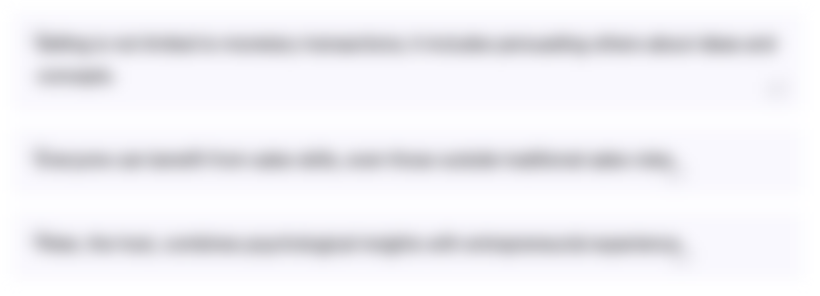
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
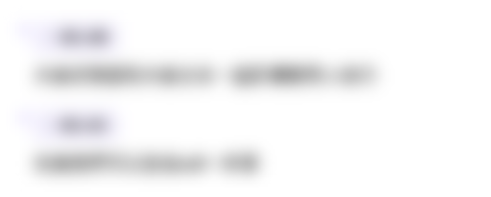
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video

JAVA TECHNICAL QUESTION & ANSWERS FOR INTERVIEW PART - V ( MULTITHREADING - II )
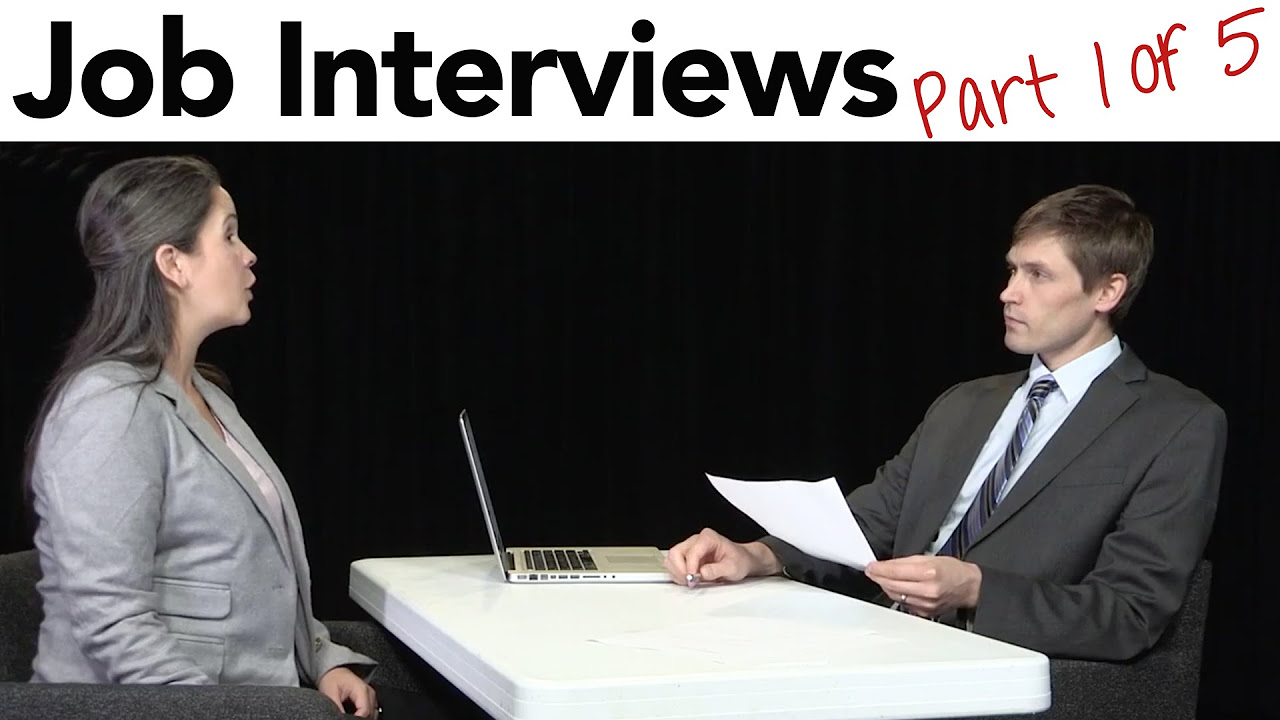
How to Interview for a Job in American English, part 1/5
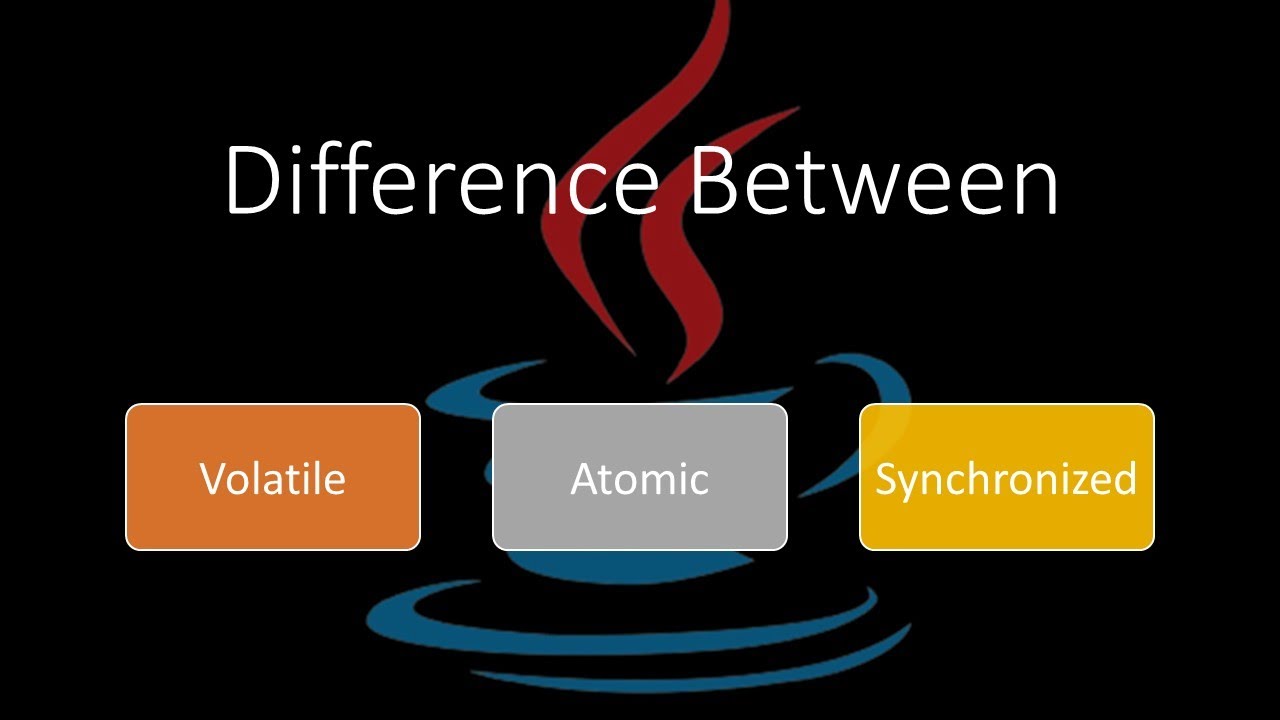
Difference Between Volatile, Atomic And Synchronized in Java | Race Condition In Multi-Threading

How to Write a Topic Sentence | Paragraph Writing: Part 1

#86 Multiple Threads in Java

Java threads 🧵
5.0 / 5 (0 votes)