Difference Between Volatile, Atomic And Synchronized in Java | Race Condition In Multi-Threading
Summary
TLDRThis video script delves into the intricacies of multithreading in Java, highlighting the challenges of race conditions when multiple threads access shared resources. It explains the use of 'synchronized', 'volatile', and 'atomic' to ensure thread safety, with examples to illustrate their application and the impact on data consistency. The script also clarifies the differences between these keywords and provides guidance on when to use each for optimal performance and reliability in concurrent programming scenarios.
Takeaways
- 🧵 Multithreading in Java allows for the simultaneous execution of two or more threads, which can lead to race conditions when shared resources are updated concurrently.
- 🔄 A race condition occurs when multiple threads access and modify a shared variable concurrently, leading to inconsistent and unpredictable results.
- 🔢 The script demonstrates a race condition with a simple example where two threads increment a shared variable, expecting a total of 30 but sometimes getting less due to concurrent access.
- 🔒 The 'synchronized' keyword in Java ensures that only one thread can access a method or block of code at a time for a given object, preventing race conditions by enforcing mutual exclusion.
- 🔄 Synchronization uses a locking mechanism where a thread must obtain a lock on an object before executing a synchronized method or block, causing other threads to wait.
- 🚫 Synchronized methods can lead to performance issues due to the overhead of lock management and have the potential for deadlocks and livelocks.
- 👀 The 'volatile' keyword in Java guarantees visibility of changes to a variable across threads, ensuring that a write to a volatile variable is immediately visible to other threads.
- 🔄 Volatile variables are useful for read/write operations but do not provide the atomicity of compound actions like incrementing a value.
- 🔢 'Atomic' variables from the java.util.concurrent.atomic package ensure that operations on them are performed atomically without the need for synchronization, providing a faster alternative for certain use cases.
- 🔄 Atomic variables use internal loops to retry actions if they are interrupted, ensuring that read-modify-write operations are completed without interference from other threads.
- ⚖️ Choosing between synchronized, volatile, and atomic depends on the specific needs of the multithreaded scenario, with each offering different trade-offs in terms of performance, simplicity, and safety.
Q & A
What is multithreading in Java?
-Multithreading is the process of executing two or more threads simultaneously, allowing for concurrent execution of tasks.
What is a race condition in multithreading?
-A race condition occurs when two or more threads try to update a mutually shared resource at the same time, potentially causing inconsistencies in data.
Can you explain the steps involved in the increment operation 'x = x + 1' in the context of a race condition?
-The increment operation 'x = x + 1' involves three steps: reading the value of x, adding one to this value, and storing the value back to x. A race condition can occur if two threads read the same value of x and then add one to it simultaneously, leading to incorrect data incrementation.
How does the 'synchronized' keyword solve race conditions?
-The 'synchronized' keyword ensures that only one thread can access a shared resource at a time by obtaining a lock on the object, making other threads wait until the lock is released.
What is cache incoherence and how does it relate to multithreading?
-Cache incoherence occurs when different threads or processes have different values of a variable in their respective caches, leading to inconsistent data. This can be resolved by using the 'volatile' keyword, which ensures that changes to a variable are immediately visible to all threads.
What is the 'volatile' keyword used for in Java?
-The 'volatile' keyword in Java is used to mark a variable as having its changes immediately visible to other threads, ensuring visibility and preventing cache incoherence.
How do atomic variables differ from synchronized methods or blocks?
-Atomic variables, such as AtomicInteger, guarantee that operations on the variable occur atomically, without interruption by other threads. They are part of the java.util.concurrent.atomic package and do not use locking mechanisms like synchronized methods or blocks.
Why might the performance of using atomic variables be faster compared to synchronized methods?
-Using atomic variables can be faster than synchronized methods because they do not involve the overhead of obtaining and releasing locks, and they are designed to perform operations atomically without locking.
What is the difference between 'volatile' and 'atomic' variables in terms of their use cases?
-Volatile variables are suitable for read and write operations where visibility is crucial, but no atomicity is required. Atomic variables are preferable for read-modify-write operations where both visibility and atomicity are necessary.
When should you use 'synchronized', 'volatile', or 'atomic' in multithreading?
-Use 'synchronized' for complete method or block synchronization when performance is not a critical concern. Use 'volatile' for visibility in read and write operations without the need for atomicity. Use 'atomic' variables for read-modify-write operations where atomicity is required.
Outlines
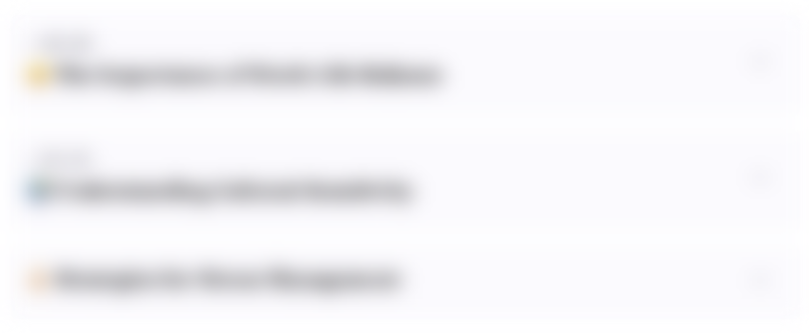
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
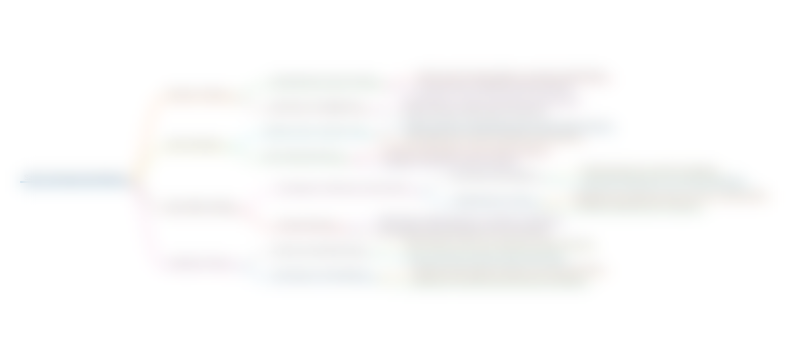
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
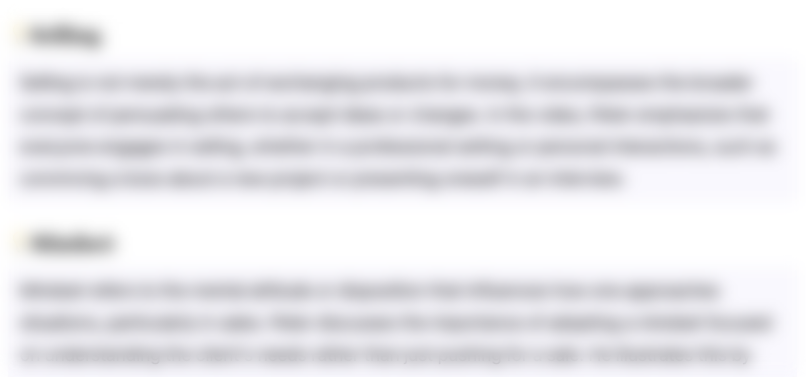
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
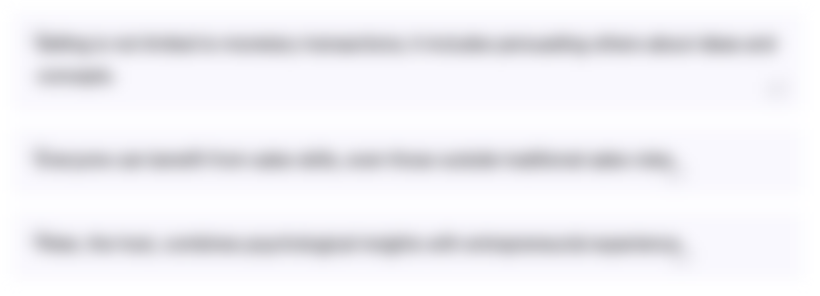
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
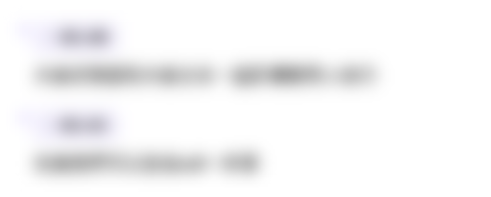
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowBrowse More Related Video
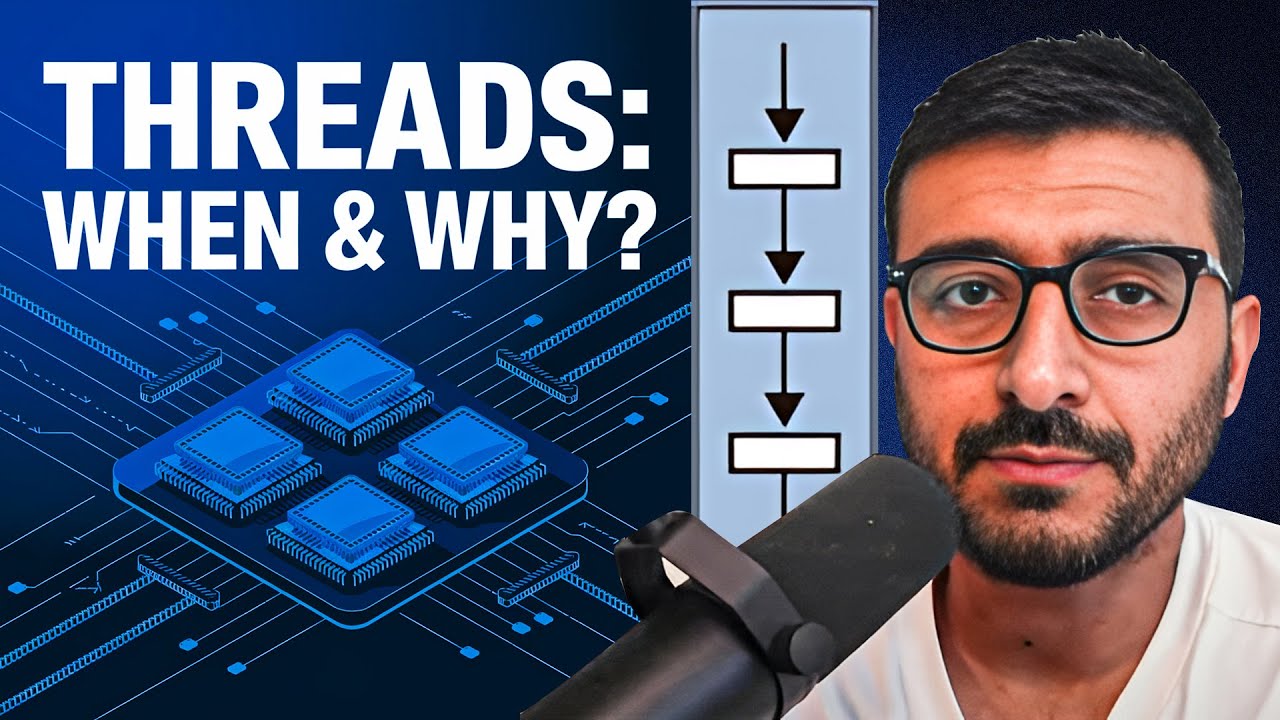
When do you use threads?
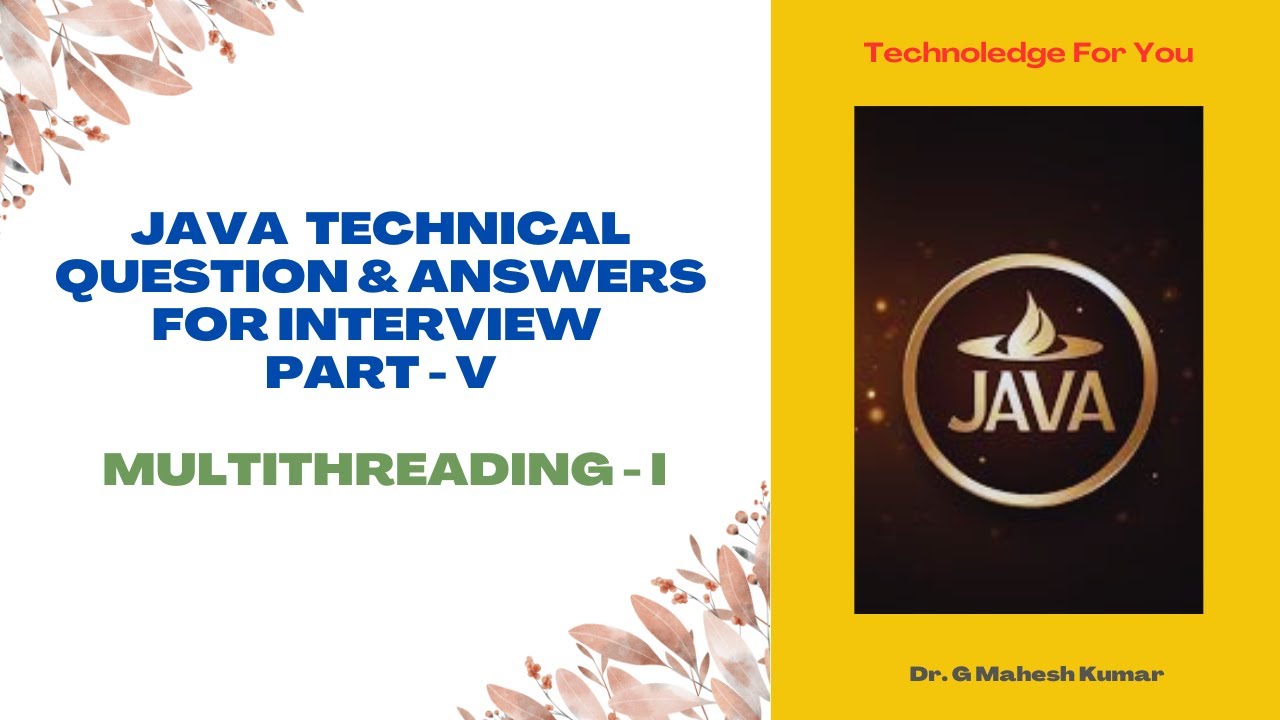
JAVA TECHNICAL QUESTION & ANSWERS FOR INTERVIEW PART - V ( MULTITHREADING - I )

#86 Multiple Threads in Java

L-3.4: Critical Section Problem | Mutual Exclusion, Progress and Bounded Waiting | Operating System

Virtual Threads in Java 21

JAVA TECHNICAL QUESTION & ANSWERS FOR INTERVIEW PART - V ( MULTITHREADING - II )
5.0 / 5 (0 votes)