Create Nested list using function | Python Essentials
Summary
TLDRIn this Python essentials video, Nikhil demonstrates how to create a nested list in Python. He starts by defining a function that generates a list of numbers from 0 to 9. Then, using a while loop, he initializes an empty list called 'nest_list' and uses the 'append' method to create a nested list structure with 10 lists, each containing numbers from 0 to 9. The video clarifies the difference between using 'extend' and 'append' for creating nested lists.
Takeaways
- 📝 The video explains how to create a nested list using Python lists, loops, and functions.
- 🧑🏫 The speaker mentions that using arrays would make this easier, but the example focuses on using Python lists.
- 🛠️ A user-defined function is used to generate a single list with values from 0 to 9.
- ⚙️ The function has no arguments and initializes an empty list, then appends values in a range of 0 to 9 using a for loop.
- 🔄 The video suggests an alternative approach using a while loop to create a similar list.
- 📑 The `extend()` function adds individual elements from the list to the main list, leading to a non-nested result.
- 📌 To create a nested list, the speaker switches to the `append()` method, which adds each generated list as an element within the main list.
- 🔢 The final nested list has 10 sub-lists, each containing numbers 0 to 9, as intended.
- 💡 The video contrasts the usage of `extend()` and `append()` to show how they affect the list structure differently.
- ✅ By using `append()`, the desired result of 10 lists nested within a larger list is achieved.
Q & A
What is the purpose of the video?
-The video aims to teach viewers how to create a nested list in Python using lists and functions.
What data structure does the video focus on, and why?
-The video focuses on lists because Python does not have arrays in the traditional sense, so nested lists are used to create multi-dimensional structures.
What is the first step in creating a nested list as explained in the video?
-The first step is to define a function that generates a single list containing the values 0 to 9.
How does the function 'listnum' generate the list?
-The function 'listnum' initializes an empty list, then uses a 'for' loop to iterate from 0 to 9, appending each value to the list, and finally returns the list.
Why does the function not take any arguments?
-The function does not require any arguments because it generates a predefined list of values (0 to 9), making input parameters unnecessary.
What mistake did the video highlight when trying to create a nested list using 'extend'?
-Using 'extend' caused the elements of each list to be added to a single list continuously, instead of creating a true nested list. 'Extend' merges elements, which prevents the formation of separate lists within the parent list.
What change is needed to correctly create a nested list?
-To correctly create a nested list, 'append' should be used instead of 'extend', which ensures that each list is added as an individual element to the main list.
What is the result when 'append' is used instead of 'extend'?
-When 'append' is used, the output is a nested list where each inner list (containing values 0 to 9) is a separate element, repeated 10 times.
How is the while loop used in creating the nested list?
-The while loop is used to repeatedly append the list generated by 'listnum' to the main nested list, iterating 10 times to create 10 inner lists.
What is the significance of adding an incrementer in the while loop?
-The incrementer ensures that the while loop progresses and eventually terminates, preventing an infinite loop from occurring.
Outlines
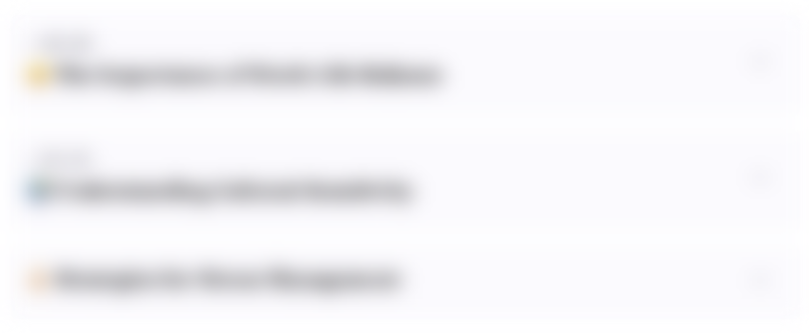
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
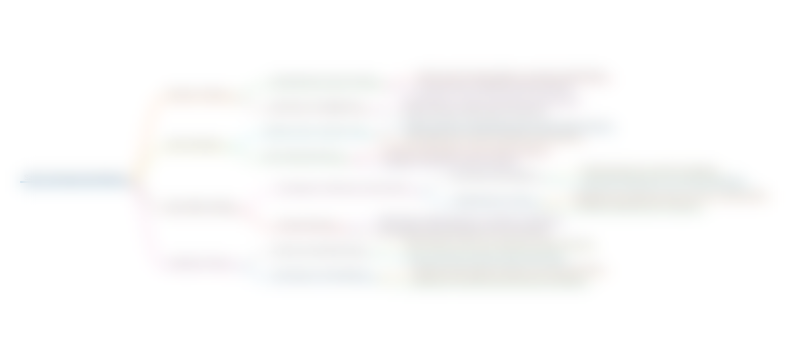
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
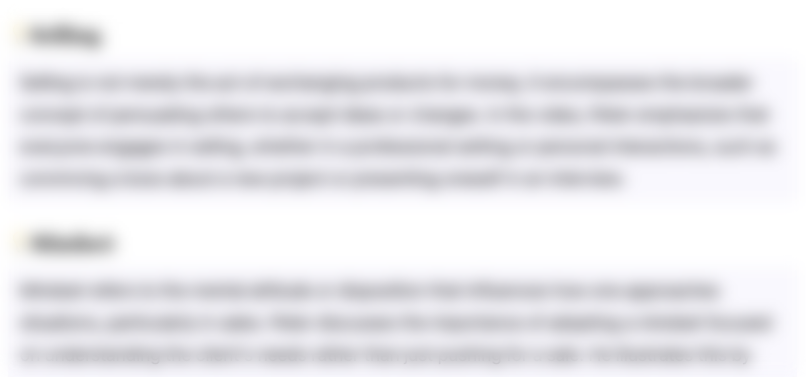
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
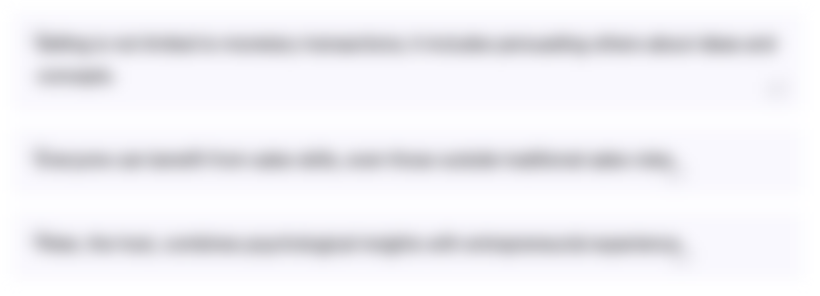
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
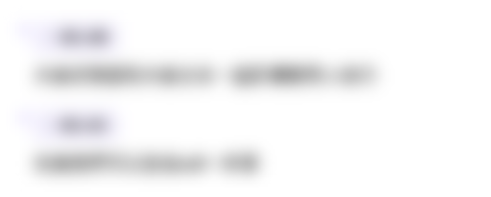
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео

Introduction To Lists In Python (Python Tutorial #4)
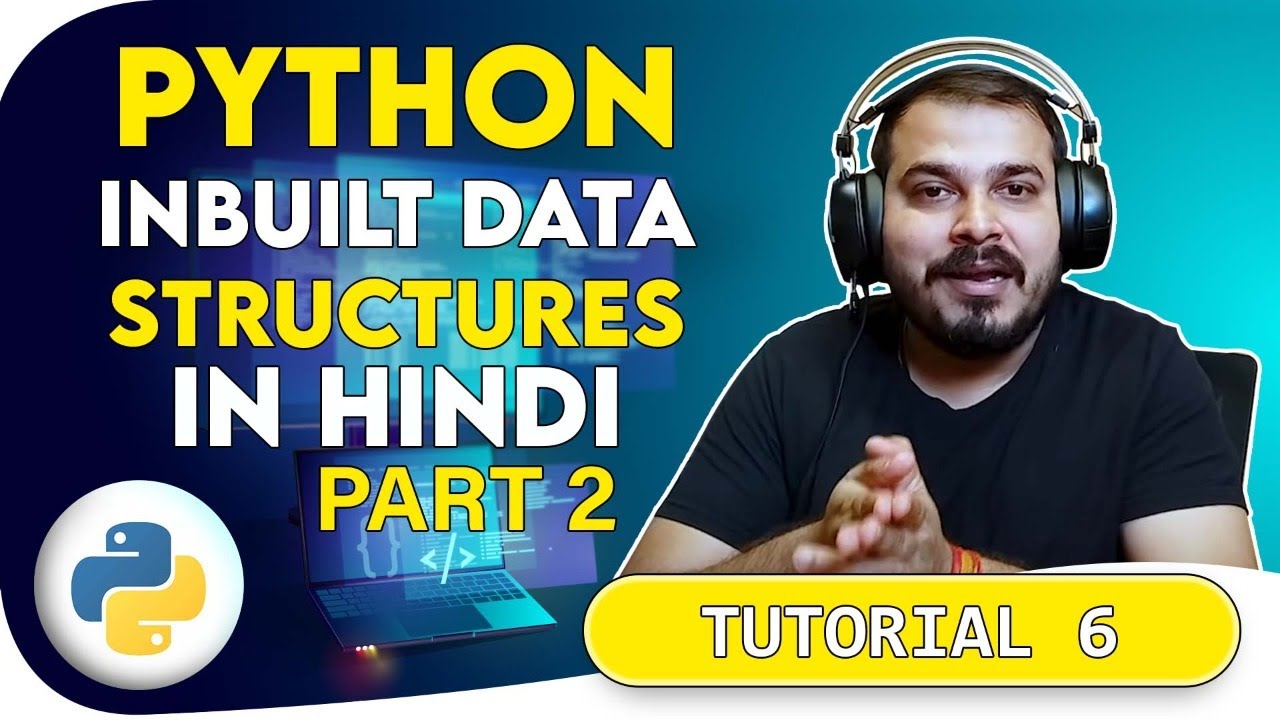
Tutorial 6- Python List And Its Inbuilt Function In Hindi
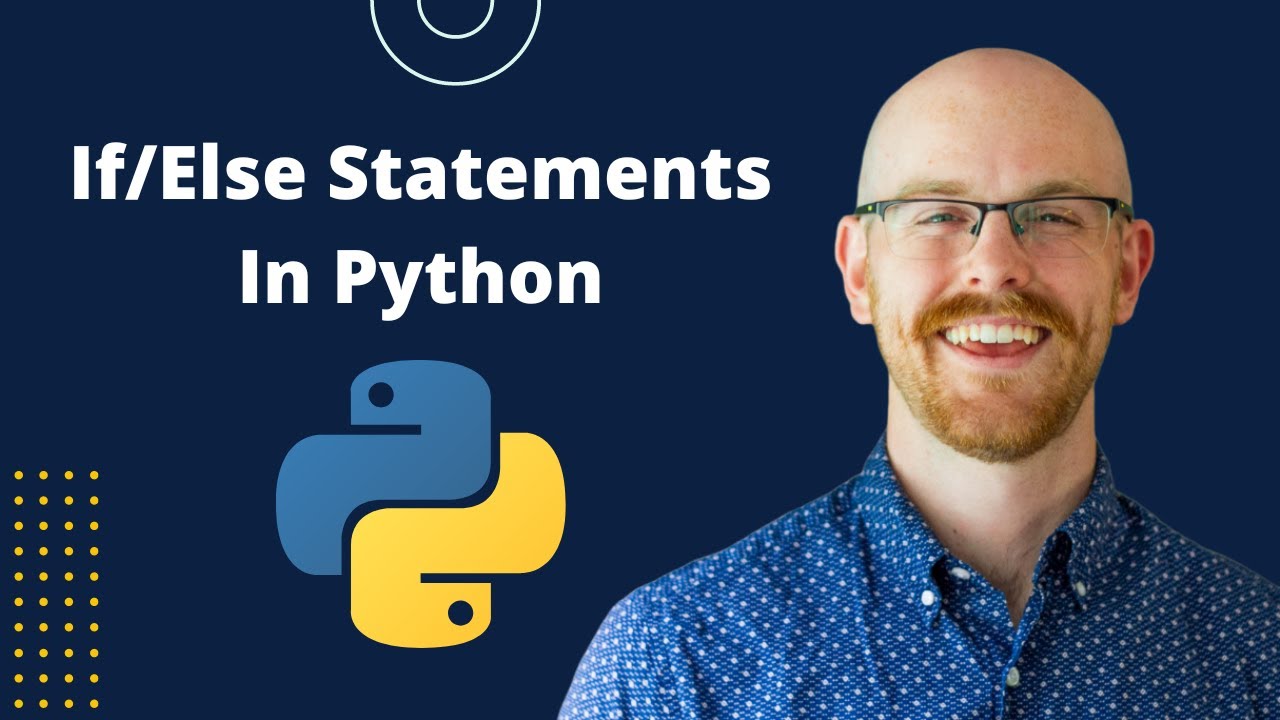
If Else Statements in Python | Python for Beginners
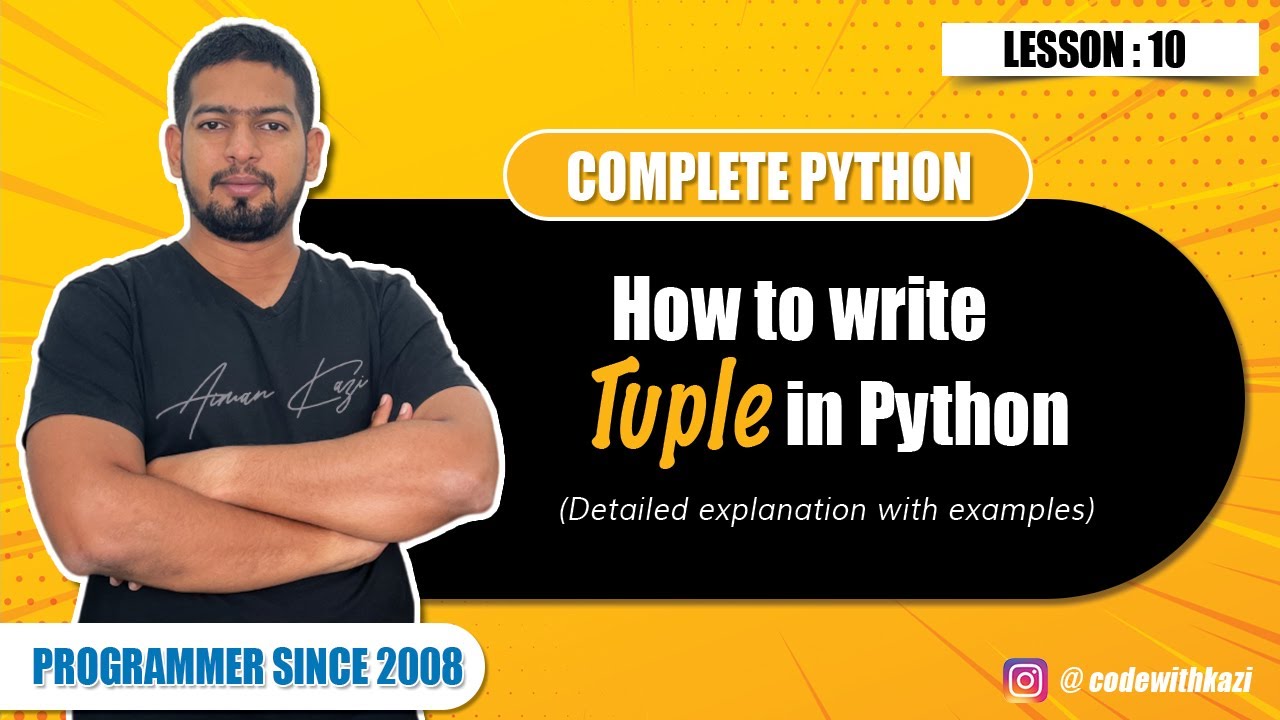
How to write tuple in python | Python Tutorials | Lesson 10
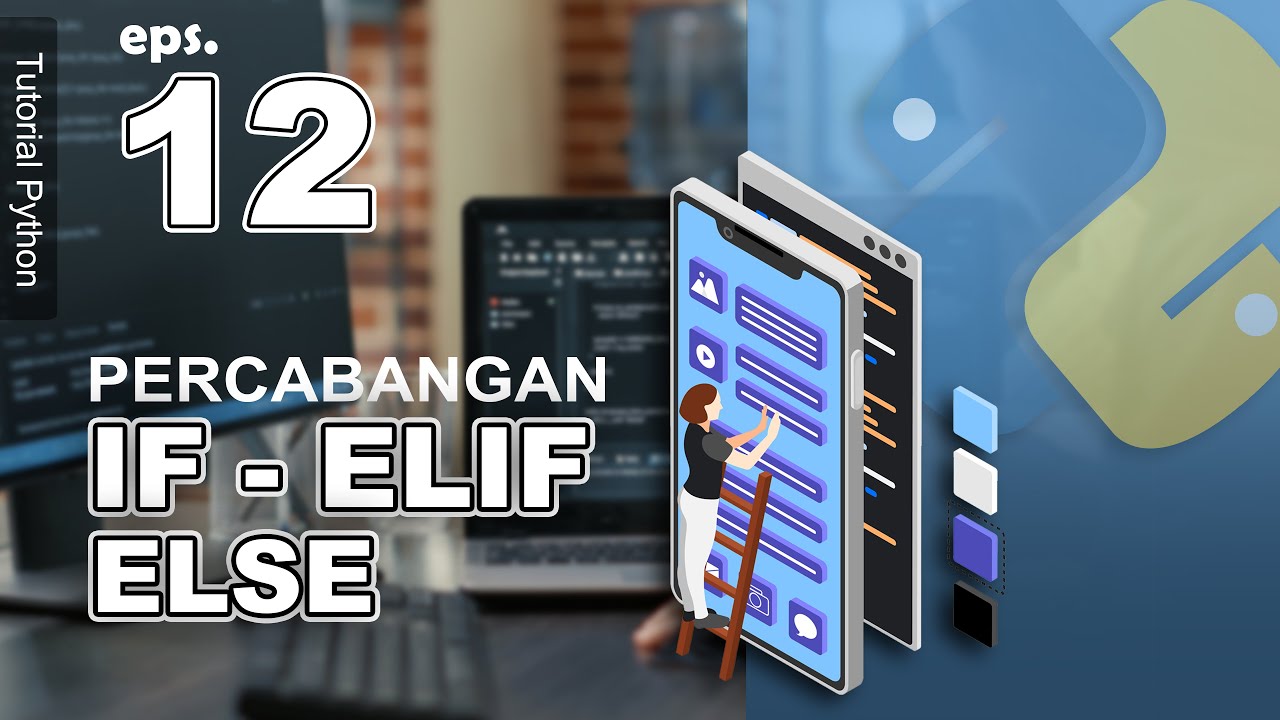
12 - IF - ELIF- ELSE (Conditional Statement) Branching - Indonesian Python Tutorial
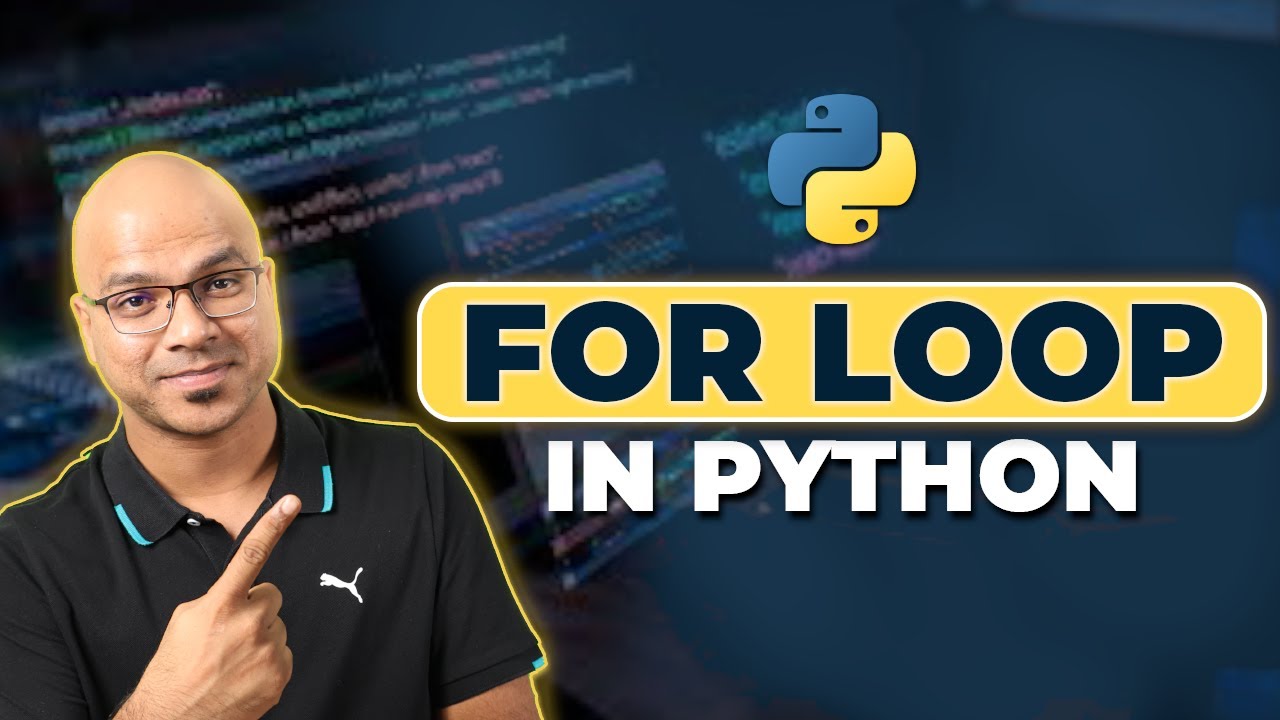
#21 Python Tutorial for Beginners | For Loop in Python
5.0 / 5 (0 votes)