#21 Python Tutorial for Beginners | For Loop in Python
Summary
TLDRThe video explains the difference between while and for loops in Python. While loops iterate based on a condition, for loops iterate through sequences like lists and strings. The script shows how to print list values with a for loop and use the range() function to print sequences of numbers, including in reverse order. It demonstrates how to use an if statement inside a for loop to selectively print values, like printing numbers not divisible by 5. The next video will cover printing patterns using nested for loops.
Takeaways
- π While loops are used for uncertain iterations, for loops work with sequences like lists, tuples, and strings
- ππ» For loops automatically iterate over the elements of a sequence, no need to manage iteration variable and conditions
- π Use 'for i in sequence:' syntax and indent the code block to use for loop
- π’ Can iterate over numeric ranges using the range() function - specify start, stop and step size
- β Range excludes the stop value, so make the stop value 1 more if you want to include it
- π Set step size as -1 in range() to iterate in reverse order
- π€ Use if condition inside for loop to filter values while iterating
- π― For loops can be nested inside other for loops similar to while loops
- π String, lists, tuples can directly be used in place of a variable in for loop syntax
- π For loops clean way to iterate as alliteration managed automatically
Q & A
What are the two types of loops discussed in the video?
-The two types of loops discussed are the while loop and the for loop.
How does a for loop differ from a while loop?
-A for loop works with sequences like lists, tuples, and strings and automatically iterates through the values, while a while loop depends on a condition and we have to manually increment/decrement the iterating variable.
How can we print values from a list using a for loop?
-We can print values from a list using a for loop by iterating through the list element by element using the iterating variable of the loop.
What is the syntax for using a for loop?
-The syntax is: for var in sequence: #loop body
How can we print numbers from 1 to 10 using a for loop?
-We can print numbers from 1 to 10 using the range() function along with a for loop. For example: for i in range(1, 11): print(i)
Can we use if statements inside a for loop?
-Yes, we can use if statements inside a for loop to add conditional logic and filtering.
How can we print numbers in reverse order using for loop?
-We can print numbers in reverse order by using range() with negative step size. For example: for i in range(20, 10, -1)
What collection types can be used with a for loop?
-For loops can be used with lists, tuples, strings, and any other iterable objects in Python.
Can we nest for loops in Python?
-Yes, we can nest one for loop inside another for loop in Python to create nested iterations.
What patterns will be discussed related to loops in the next video?
-In the next video, some patterns related to printing shapes and structures using loops will be discussed.
Outlines
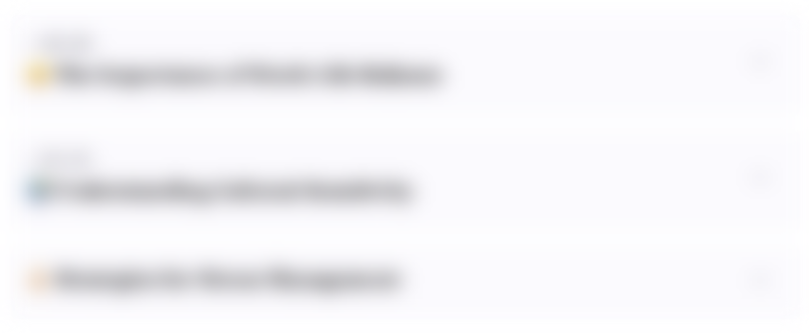
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowMindmap
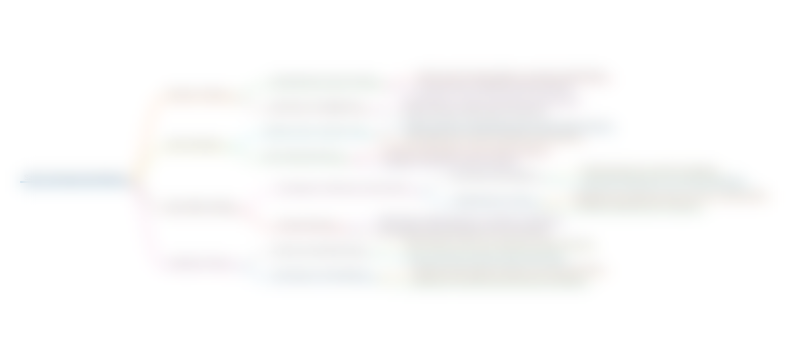
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowKeywords
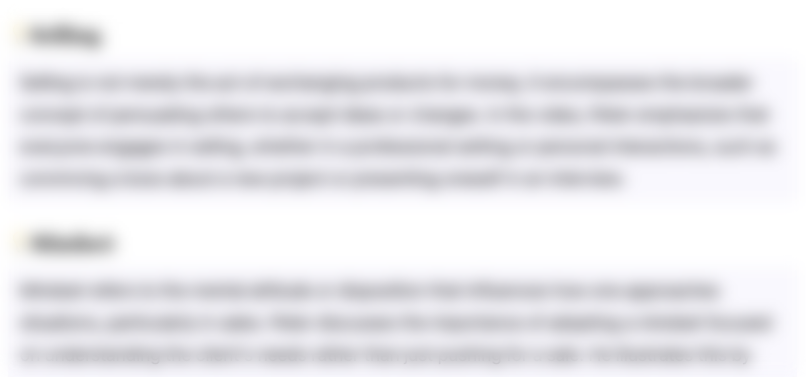
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowHighlights
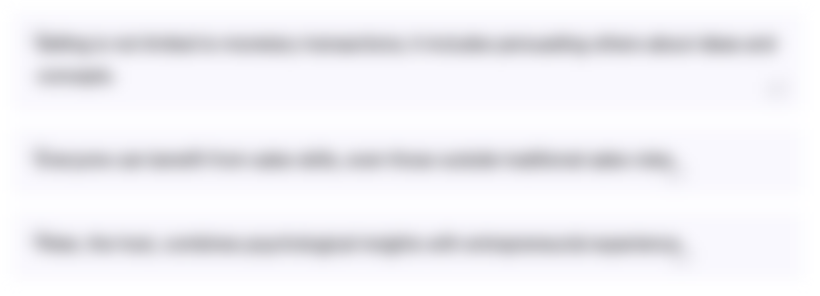
This section is available to paid users only. Please upgrade to access this part.
Upgrade NowTranscripts
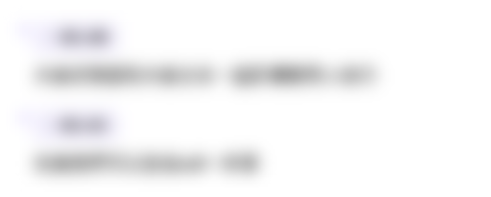
This section is available to paid users only. Please upgrade to access this part.
Upgrade Now5.0 / 5 (0 votes)