Asynchrony: Under the Hood - Shelley Vohr - JSConf EU
Summary
TLDRIn this talk, Shelley, a software engineer at GitHub, delves into asynchronous programming in JavaScript. She begins with basic principles and progresses to methodologies, highlighting the event loop, call stack, and run-to-completion semantics. Shelley contrasts callbacks, promises, generators, and async/await, explaining their roles and best use cases. She emphasizes the cognitive challenges of callbacks, the error handling in promises, and the intuitive, synchronous-like code structure of async/await. The presentation aims to equip developers with a deeper understanding of asynchronous tools in JavaScript.
Takeaways
- 🕒 Asynchronous programming in JavaScript deals with the 'now' and 'later' execution of code, managing tasks that will complete at an uncertain time.
- 🔁 The event loop is a central concept, running as an endless loop that processes chunks of code and manages the execution queue.
- 🆕 ES6 introduced microtasks, a queue that precedes the traditional task queue and helps in handling asynchronous operations more promptly.
- 💡 JavaScript's run-to-completion semantics ensure that a task finishes before the next begins, providing a safe execution context without concurrent modifications.
- 📈 Understanding the call stack is crucial for tracking function execution and returns, which is vital for managing asynchronous callbacks.
- 🔀 Callbacks, while simple, can lead to 'callback hell' due to their non-linear and complex nature, making code hard to follow and error-prone.
- 🔐 Promises introduced a new level of abstraction with placeholders for future values, offering better control and error handling for asynchronous operations.
- 🔁 Generators allow for a pause and resume pattern, making asynchronous code appear synchronous and simplifying error handling with traditional try/catch.
- 🔄 Async/await syntax, built on promises, makes asynchronous code more readable and manageable, using synchronous-style error handling with try/catch.
- 🛠️ Choosing the right asynchronous pattern depends on the specific requirements of the task, with each method having its strengths and appropriate use cases.
Q & A
What is the main focus of Shelley's talk?
-Shelley's talk focuses on the mechanics of asynchronous programming in JavaScript, including the basic principles, methodologies, and nuances that differentiate them.
What does the term 'asynch' refer to in the context of programming?
-In the context of programming, 'asynch' refers to asynchronous operations, which are operations that do not occur in a linear, sequential order but are managed over time with respect to 'now' and 'later'.
How is the event loop described in the talk?
-The event loop is described as an endlessly running, singly threaded loop that processes tasks in the order they are added to the queue, with each iteration running a small chunk of code.
What is the significance of JavaScript's run-to-completion semantics?
-JavaScript's run-to-completion semantics mean that the current task always finishes before the next task begins, ensuring that each task has complete control over all current states without interference from other tasks.
What is the role of the call stack in JavaScript's execution model?
-The call stack manages the execution of functions by keeping track of the location each function needs to return to once it's done executing, allowing for proper sequencing and memory management of function calls.
Why can callbacks lead to difficulties in understanding and managing asynchronous code?
-Callbacks can lead to difficulties because they require managing multiple asynchronous operations that may not execute in a linear, sequential order, which can result in complex and hard-to-follow code structures known as 'callback hell'.
What is the 'inversion of control' in the context of callbacks?
-Inversion of control in callbacks refers to the practice where the flow of the program is dictated by callback functions rather than the sequential order of the code, which can lead to trust issues and complexity.
How do promises change the way asynchronous operations are handled in JavaScript?
-Promises introduce a new way of handling asynchronous operations by providing a placeholder for future values, allowing developers to work with these values as if they were already available, and managing the eventual success or failure of these operations.
What is the microtask queue and how does it relate to promises?
-The microtask queue is a queue introduced in ES6 that allows certain asynchronous actions to be added to the end of the current microtask queue instead of waiting for the next event loop tick, which is particularly useful for promises to ensure their callbacks are executed immediately after the promise settles.
How do generators provide a different approach to asynchronous programming?
-Generators allow for a function to be paused and resumed multiple times, which enables a synchronous-looking code style while hiding the asynchronous operations as implementation details, making the code easier to reason about.
What is the main benefit of using async/await over traditional promises?
-Async/await simplifies the process of working with promises by making asynchronous code look and behave more like synchronous code, improving error handling and readability, and reducing the amount of boilerplate code needed.
Outlines
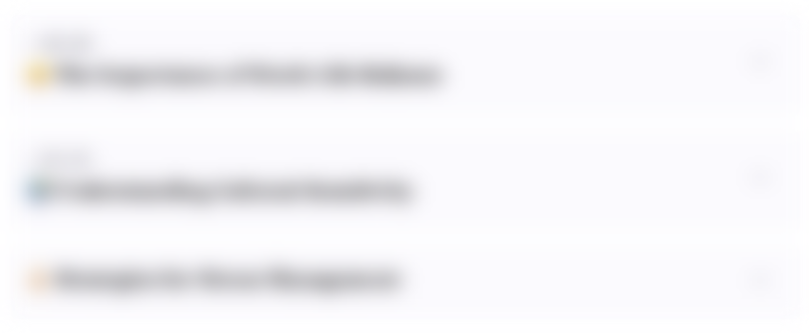
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифMindmap
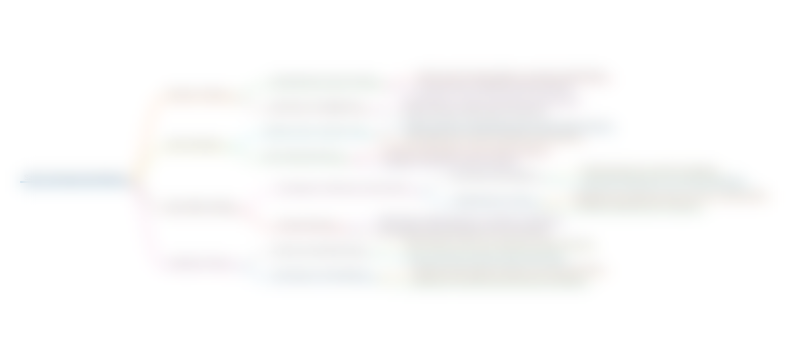
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифKeywords
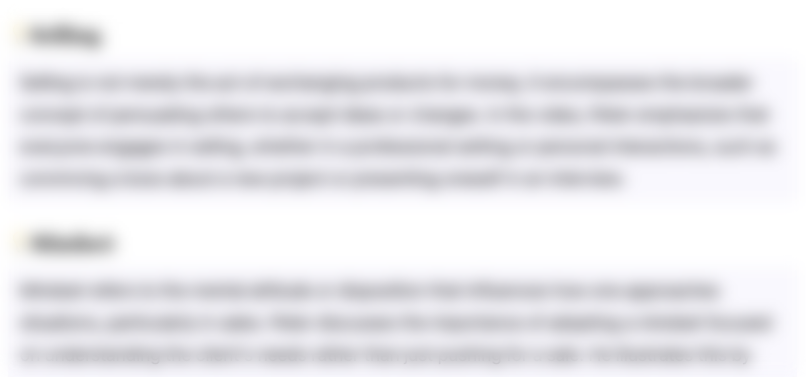
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифHighlights
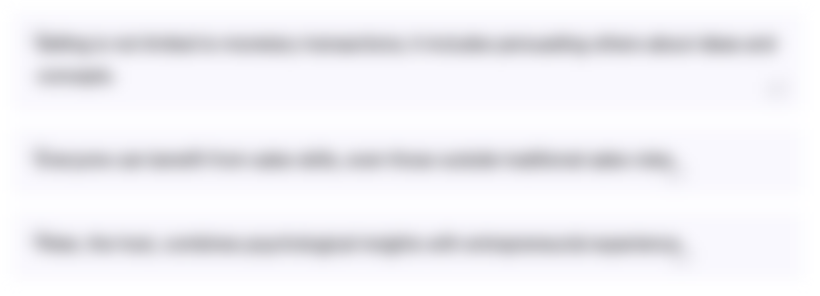
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифTranscripts
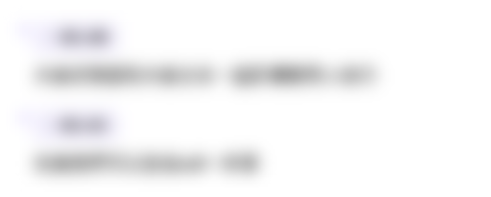
Этот раздел доступен только подписчикам платных тарифов. Пожалуйста, перейдите на платный тариф для доступа.
Перейти на платный тарифПосмотреть больше похожих видео
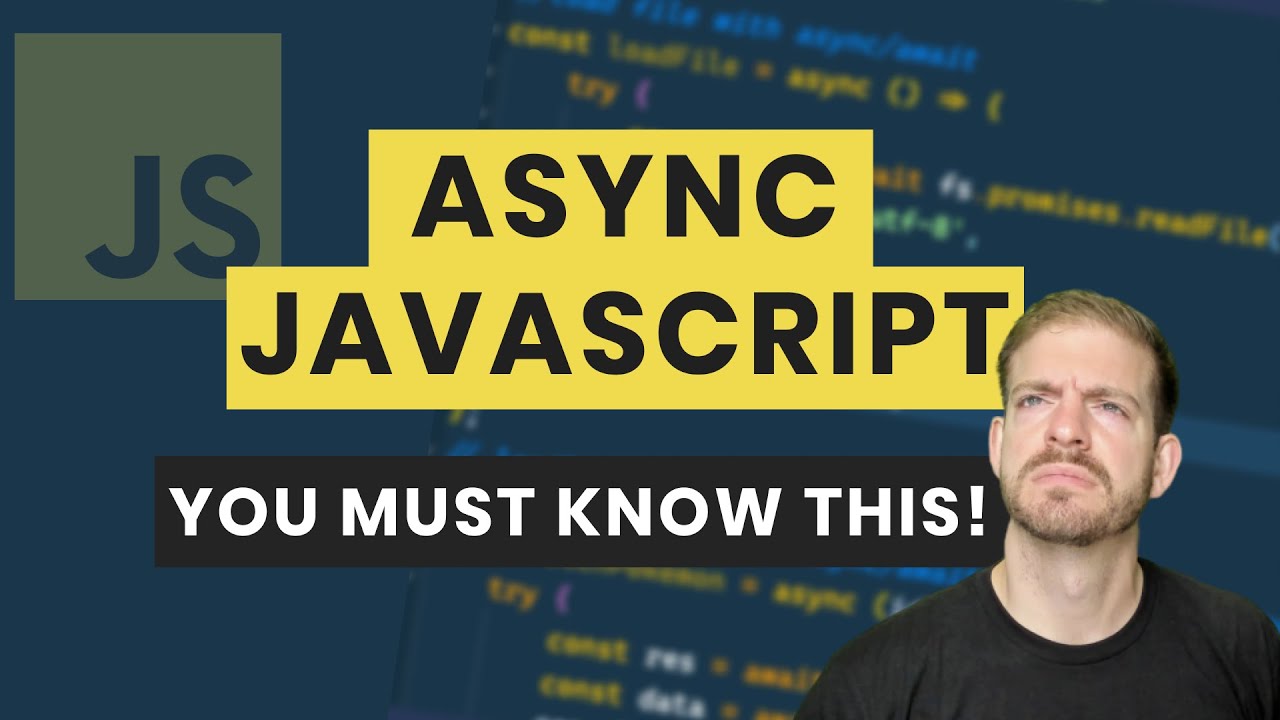
Asynchronous JavaScript in ~10 Minutes - Callbacks, Promises, and Async/Await
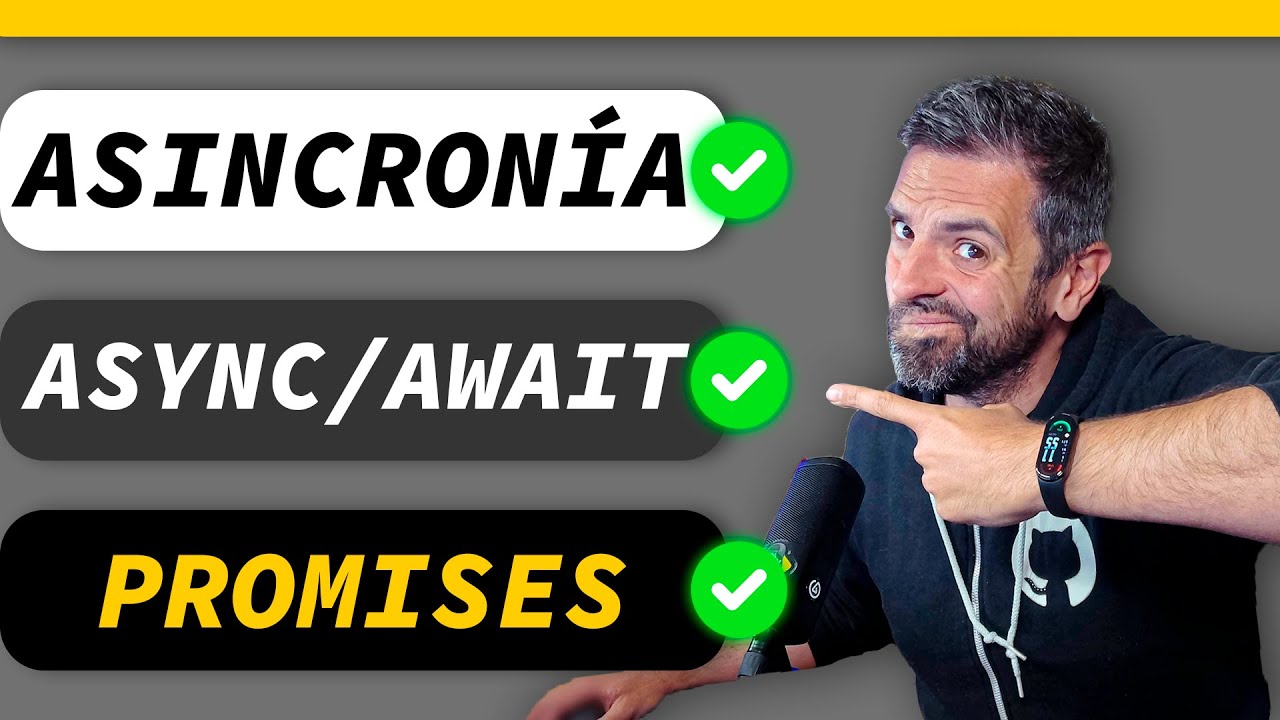
Asincronía en JavaScript: Todo lo que necesitas saber
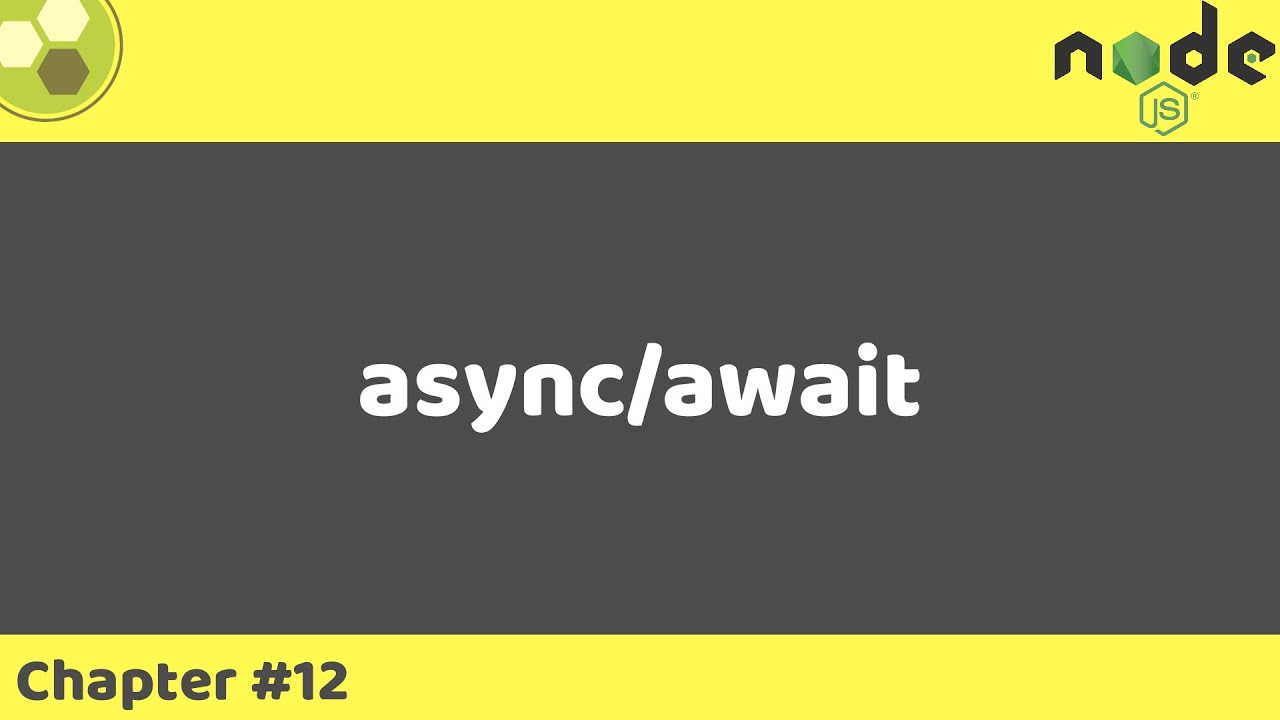
Node.js Tutorial #12 | async/await
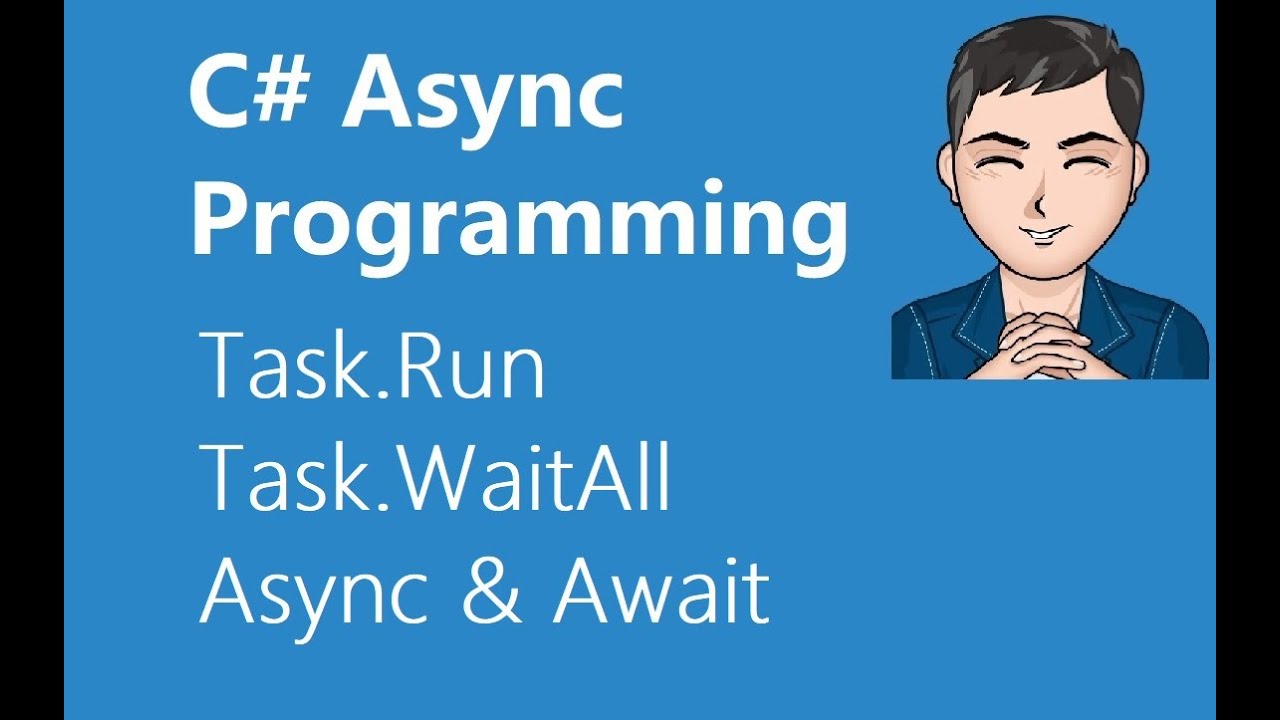
Asynchronous Programming in C# Explained (Task.Run, Task.WaitAll, Async and Await)
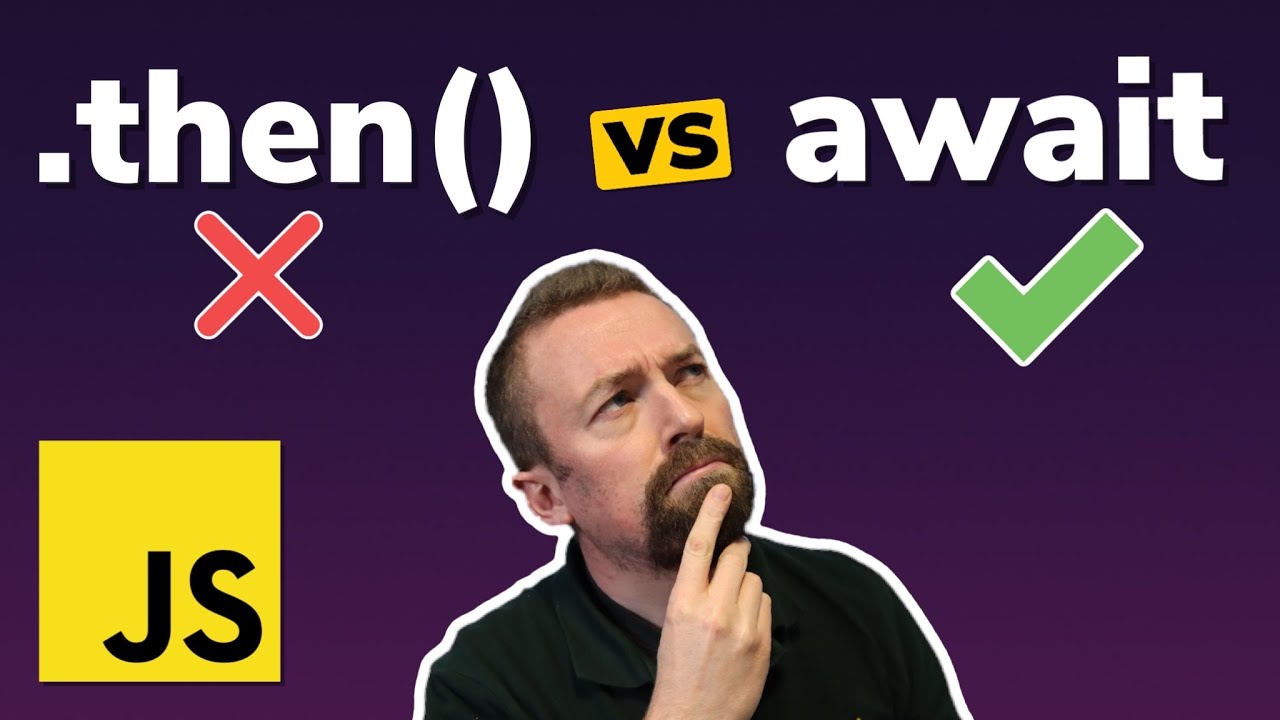
Javascript Promises vs Async Await EXPLAINED (in 5 minutes)
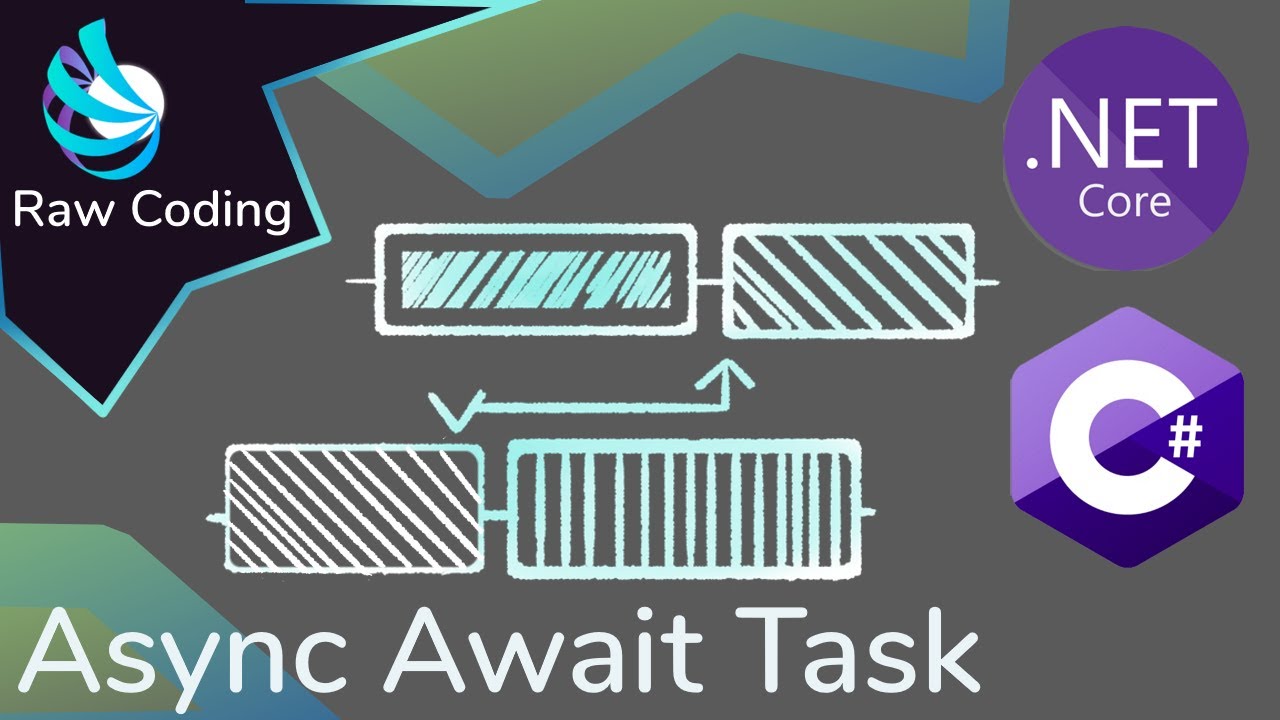
C# Async/Await/Task Explained (Deep Dive)
5.0 / 5 (0 votes)