Fetch Decode Execute Cycle in more detail
Summary
TLDRThe script delves into the CPU's fetch-decode-execute cycle, illustrating how a high-level program is translated into assembly and machine code. It explains the role of CPU components like the accumulator, ALU, and registers in executing a simple program involving arithmetic operations. The process is simplified for clarity, highlighting the CPU's function as an advanced adding machine, emphasizing the foundational role of memory addressing and data manipulation in computation.
Takeaways
- 💡 The central processing unit (CPU) operates through a fetch, decode, execute cycle when running a stored program.
- 🔍 High-level language code is translated into assembly language, which requires more commands and consideration of the accumulator.
- 📍 Assembly language can use direct or symbolic addressing to refer to memory locations.
- 🤖 Once assembly code is turned into machine code, it is stored in RAM, and the memory addresses are in binary.
- 🔢 The CPU contains several registers, including the accumulator, program counter, current instruction register, memory address register, and memory data register.
- 🔄 The program counter holds the address of the next instruction to be fetched and increments after each instruction is fetched.
- 📥 The memory address register is used to load the address of the instruction or data to be fetched from RAM.
- 📤 The memory data register is used to transfer data to and from the RAM.
- ⚙️ The control unit within the CPU decodes the instruction and directs the ALU to perform the necessary operations.
- 🧩 The arithmetic and logic unit (ALU) performs operations such as addition, using simple adder circuits.
- 🔚 The final step of the cycle is to store the result from the accumulator back into memory, completing the execution of the program.
Q & A
What is the fetch-decode-execute cycle in the context of a CPU?
-The fetch-decode-execute cycle is the fundamental process that a CPU follows to execute a program. It involves three main steps: fetching the instruction from memory, decoding the instruction to understand what it means, and then executing the instruction by performing the required operation.
Why is a high-level language easier to understand than assembly language?
-A high-level language is designed to be more human-readable and abstracts away the low-level details of the hardware. Assembly language, on the other hand, is closer to machine code and requires a deeper understanding of the CPU's architecture, making it less intuitive for humans.
What is direct addressing in assembly language?
-Direct addressing is a method in assembly language where memory addresses are referred to by their numerical values. It is a straightforward way to access data stored at specific memory locations.
What is symbolic addressing and how does it differ from direct addressing?
-Symbolic addressing is an alternative to direct addressing where memory addresses are referred to by symbolic names or labels instead of numerical values. This makes the code more readable and easier to maintain but requires the assembler to translate these symbols into actual memory addresses.
What is the purpose of the accumulator in a CPU?
-The accumulator is a register in the CPU that is used to hold intermediate results during arithmetic and logical operations. It plays a central role in many CPU operations, such as storing the result of an addition before it is stored back into memory.
Can you explain the role of the control unit in the CPU during program execution?
-The control unit manages the operation of the CPU, coordinating the flow of data and instructions between the various components of the CPU. It decodes the instructions and generates the necessary control signals to execute them, ensuring that the CPU operates in a coordinated and efficient manner.
What are the main components of the CPU that are involved in executing a program?
-The main components involved in executing a program are the control unit, the accumulator (part of the ALU), the program counter, the current instruction register, the memory address register, and the memory data register.
How does the program counter (PC) function during the execution of a program?
-The program counter holds the memory address of the next instruction to be executed. After an instruction is fetched, the PC is incremented to point to the next instruction. This ensures a sequential execution of instructions unless altered by control flow instructions like jumps or branches.
What is the role of the memory address register and memory data register during the fetch and execution of instructions?
-The memory address register holds the address of the memory location from which data or instructions are to be fetched. The memory data register is used to transfer data between the memory and the CPU's internal registers, such as the accumulator, during the fetch, decode, and execute stages.
How does the ALU contribute to the execution of arithmetic operations?
-The ALU (Arithmetic Logic Unit) performs arithmetic operations like addition, subtraction, multiplication, and division, as well as logical operations like AND, OR, and NOT. It is responsible for the computation part of the CPU's function, executing the arithmetic instructions decoded by the control unit.
What is the significance of the 'add' instruction in the context of the CPU's operation?
-The 'add' instruction is a fundamental operation in the CPU that tells the ALU to add the contents of a specified memory location to the value currently in the accumulator. It is an example of how the CPU performs basic arithmetic operations, which are the building blocks of more complex computations.
Outlines
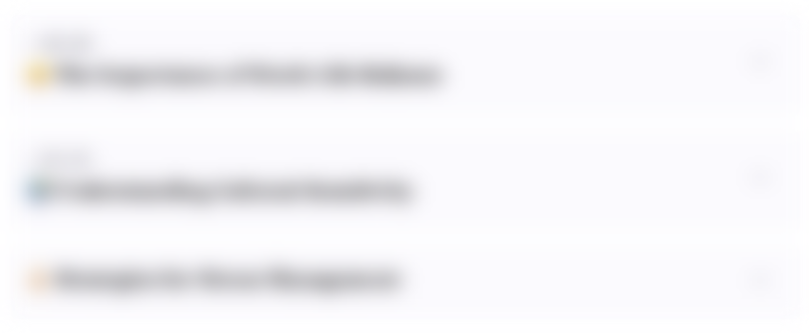
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
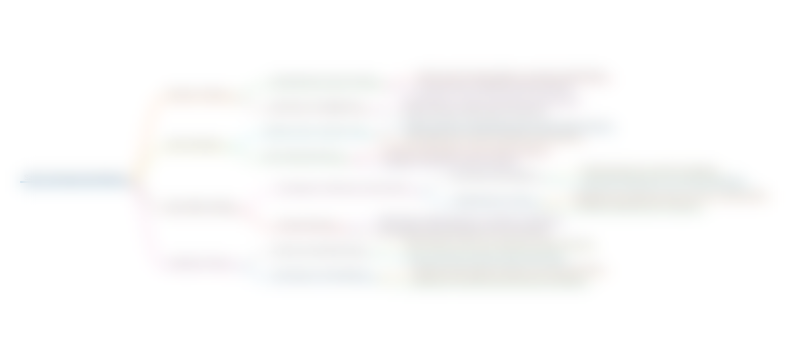
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
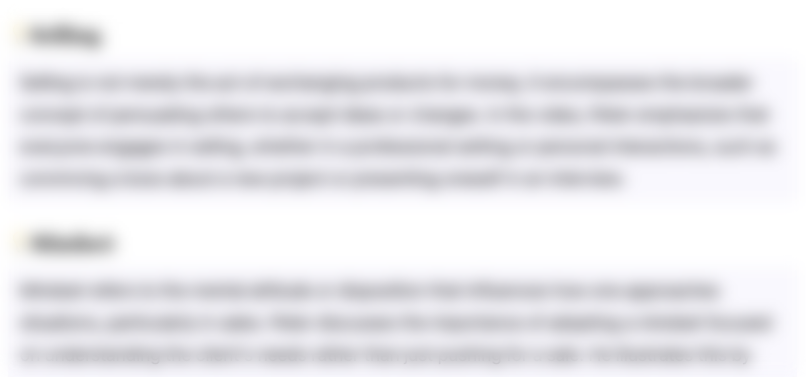
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
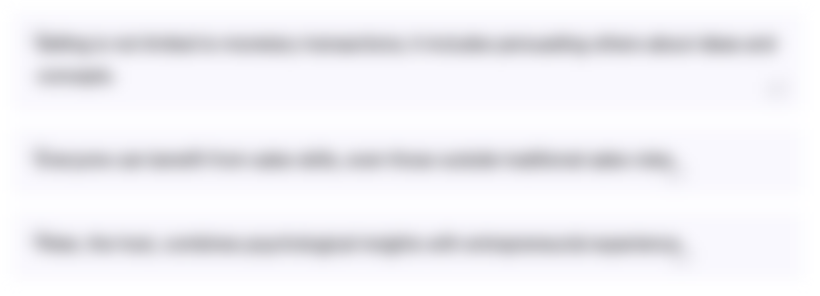
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
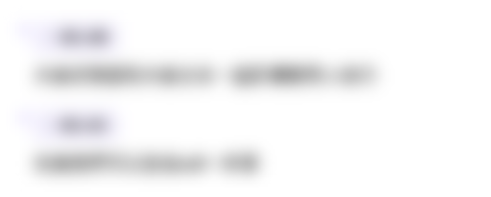
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)