SMART POINTERS in C++ (std::unique_ptr, std::shared_ptr, std::weak_ptr)
Summary
TLDRThis video script delves into the concept of smart pointers in C++, a feature designed to automate memory management. It explains that smart pointers, such as unique and shared pointers, act as wrappers around raw pointers to handle memory allocation and deallocation automatically. Unique pointers prevent copying to avoid multiple owners of the same memory, ensuring automatic deletion when out of scope. Shared pointers, on the other hand, use reference counting to manage multiple references safely. The script also touches on weak pointers for non-owning references. The presenter suggests that while smart pointers are beneficial for preventing memory leaks, they don't entirely replace the need for manual memory management with 'new' and 'delete', recommending the use of unique pointers by default due to their lower overhead.
Takeaways
- 📌 Smart pointers in C++ are designed to automate memory management, eliminating the need for manual calls to `new` and `delete`.
- 🔑 A smart pointer acts as a wrapper around a raw pointer, managing the allocation and deallocation of memory on the heap.
- 👤 Unique pointers (`std::unique_ptr`) are non-copyable and are used for exclusive ownership of a resource, automatically deleting the object when the pointer goes out of scope.
- 🔄 The copy constructor and assignment operator for unique pointers are deleted to prevent multiple owners of the same resource, which could lead to double deletion and undefined behavior.
- 🚫 `std::make_unique` is the preferred way to create unique pointers due to its exception safety; it avoids potential memory leaks if an exception occurs during object construction.
- 🤝 Shared pointers (`std::shared_ptr`) allow for shared ownership of a resource through reference counting, where the resource is only deleted when the last shared pointer goes out of scope.
- 📈 Reference counting in shared pointers involves allocating an additional control block to keep track of the number of references, which adds some overhead compared to unique pointers.
- 🔄 `std::make_shared` is the recommended method for creating shared pointers as it is more efficient and exception-safe compared to using `new` followed by the shared pointer constructor.
- 🔗 Weak pointers (`std::weak_ptr`) provide a non-owning reference to an object managed by shared pointers, useful for breaking reference cycles and can be used to check if the object is still valid.
- ♻️ Smart pointers help prevent memory leaks and simplify memory management, but they are not a complete replacement for `new` and `delete`, especially in cases where explicit control over memory allocation is necessary.
- 🛠️ The choice between unique and shared pointers depends on the use case, with unique pointers being the first preference due to lower overhead, followed by shared pointers when sharing is necessary.
Q & A
What are smart pointers in C++?
-Smart pointers in C++ are a feature that automates memory management. They wrap around a raw pointer, managing the allocation and deallocation of memory on the heap, so you don't have to manually call 'new' and 'delete'.
Why should one consider using smart pointers?
-Smart pointers should be considered for their ability to automate memory management, preventing memory leaks by ensuring that memory is properly freed when it's no longer needed, without the programmer having to explicitly call 'delete'.
What is the difference between a unique pointer and a shared pointer in C++?
-A unique pointer is a smart pointer that owns the object it points to and ensures that there is only one owner of the object. It cannot be copied. A shared pointer, on the other hand, maintains a reference count to keep track of how many pointers share ownership of the same object, and it can be copied.
Why are unique pointers called 'unique'?
-Unique pointers are called 'unique' because they must be the sole owner of the object they point to. Copying a unique pointer is not allowed to prevent multiple owners from causing issues when the memory is freed.
What is the preferred way to create a unique pointer in C++?
-The preferred way to create a unique pointer is by using 'std::make_unique', which is safer in terms of exception handling because it prevents the creation of a dangling pointer in case of an exception during object construction.
How does the reference counting mechanism work with shared pointers?
-Reference counting with shared pointers works by keeping track of how many shared pointers are pointing to a particular object. When the reference count reaches zero, indicating no shared pointers are referencing the object, the object is deleted and its memory is freed.
What is a weak pointer and what is its purpose?
-A weak pointer is a smart pointer that does not increase the reference count when it holds a reference to an object. It is used to break reference cycles and to hold a non-owning reference to an object that is managed by shared pointers.
What is the overhead associated with using shared pointers?
-The overhead associated with shared pointers comes from the additional memory required for the reference count and the control block, as well as the potential performance cost of incrementing and decrementing the reference count.
Why should one avoid using 'new' with shared pointers?
-Using 'new' with shared pointers is not recommended because it requires an additional allocation for the control block, which can be avoided by using 'std::make_shared', making the process more efficient and exception-safe.
What is the general recommendation for when to use unique pointers versus shared pointers?
-Unique pointers should be used as the first preference when you need a single owner for the object. Shared pointers should be used when you need to share ownership of an object between multiple pointers, but be aware of the additional overhead they introduce.
Outlines
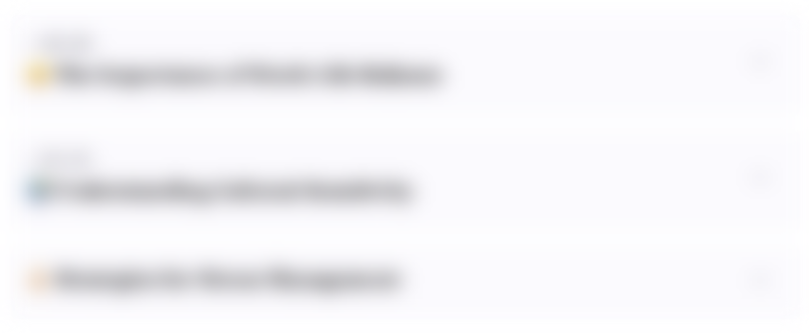
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
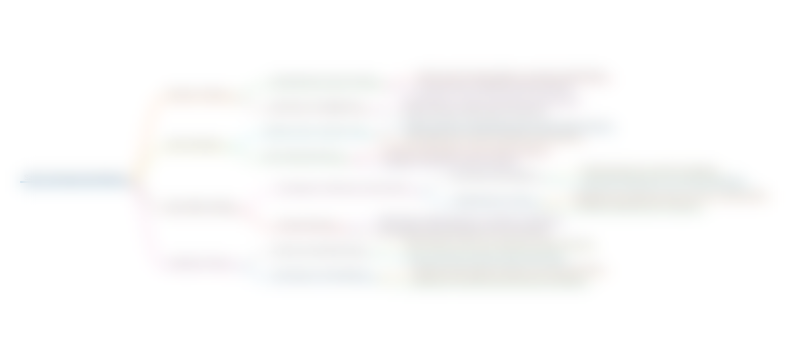
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
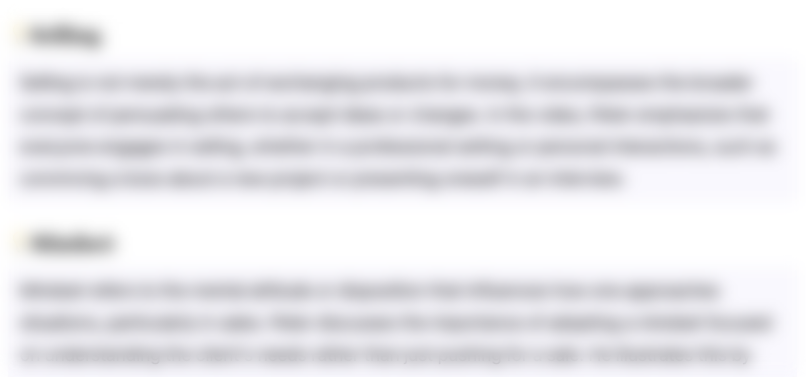
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
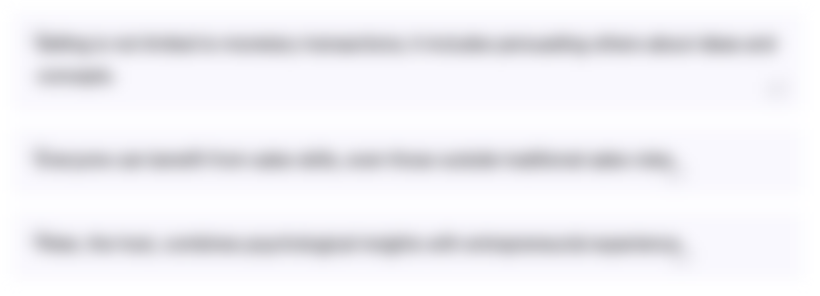
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
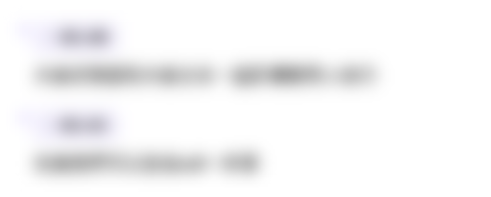
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)