Go (Golang) Tutorial #3 - Variables, Strings & Numbers
Summary
TLDRThis video tutorial introduces the basics of variable declaration in the Go programming language. It explains how to declare and initialize variables, focusing on strings and integers. The video covers multiple methods of variable declaration, including explicit typing, inferred typing, and shorthand syntax. It also discusses the use of integers, their bit sizes, and floats, highlighting the differences between types like int, uint, and float32/64. Additionally, the tutorial emphasizes best practices for using variables and resolving common errors in Go programming.
Takeaways
- 😀 Variables in Go can be declared using the `var` keyword, followed by the variable name, its type, and a value.
- 📚 Strings in Go are defined using double quotes, not single quotes.
- ⚠️ In Go, declared variables must be used, or you'll encounter an error.
- 💡 Go supports type inference, meaning the language can automatically determine the variable type based on its value.
- 📝 You can declare a variable without assigning it a value, in which case Go assigns it a default value.
- 🔄 Variables can be updated, but their types cannot be changed once declared.
- ⏩ Go offers a shorthand for variable declaration using `:=`, but this can only be used within functions.
- 🔢 Go has multiple integer types (e.g., `int8`, `int16`, `int32`), allowing for different ranges based on memory size.
- ➖ Unsigned integers (`uint`) in Go do not allow negative values and have a different range from signed integers.
- 🌊 Floats are used for decimal numbers in Go, and their precision can be specified using `float32` or `float64`.
Q & A
What is a variable in Go?
-A variable in Go is used to store information or data such as strings, numbers, arrays, etc. Variables must be declared before being used, and they have specific types such as string or int.
How do you declare a string variable in Go?
-A string variable in Go can be declared using the `var` keyword, followed by the variable name, the type `string`, and the value. For example: `var name1 string = "mario"`.
What happens if you declare a variable but don't use it in Go?
-In Go, if you declare a variable but don't use it, the compiler will throw an error. You need to use every declared variable to avoid this error.
What is type inference in Go?
-Type inference in Go allows the compiler to automatically determine the type of a variable based on the value it is assigned. For example: `var name2 = "luigi"` infers that `name2` is of type string.
How can you declare a variable without initializing it in Go?
-You can declare a variable without initializing it by specifying the type but not assigning a value, e.g., `var name3 string`. The variable will have a default value, which for strings is an empty string.
What is the shorthand for declaring a variable in Go?
-The shorthand for declaring a variable in Go is to use `:=` without the `var` keyword. For example: `name4 := "yoshi"` declares and initializes the variable `name4` as a string.
Why can't you use the shorthand variable declaration outside of functions in Go?
-The shorthand declaration using `:=` can only be used within functions in Go. If used outside of a function, the compiler will throw an error because it's not allowed in the global scope.
What is the difference between int and float in Go?
-In Go, an `int` is used for whole numbers, whereas a `float` is used for numbers with decimal points. For example, `var age int = 20` for an integer and `var score float64 = 21.5` for a floating-point number.
How does Go handle different bit sizes for integers?
-Go allows specifying the bit size for integers, such as `int8`, `int16`, `int32`, or `int64`. The larger the bit size, the larger the range of values that the integer can hold. For example, `int8` can hold values from -128 to 127, while `int16` has a larger range.
What is a uint in Go and how is it different from int?
-A `uint` is an unsigned integer in Go, meaning it can only hold non-negative values (no negatives). For example, `uint8` holds values from 0 to 255, unlike `int8`, which holds both negative and positive values.
Outlines
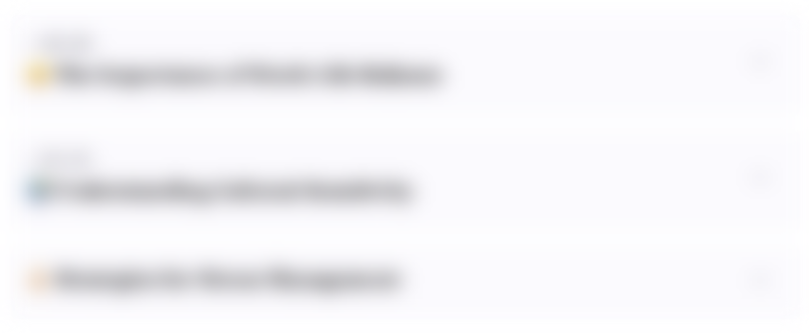
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードMindmap
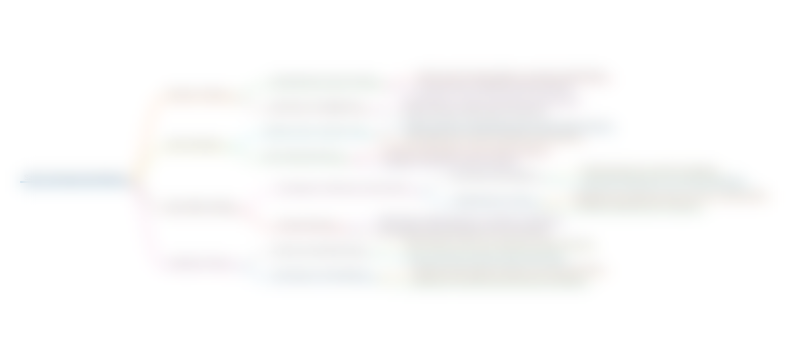
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードKeywords
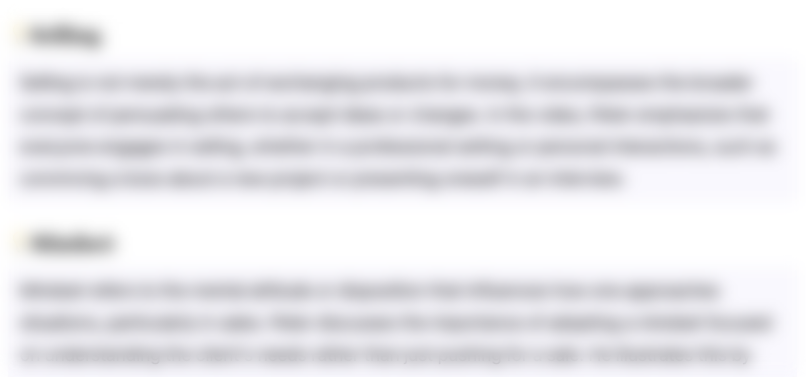
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードHighlights
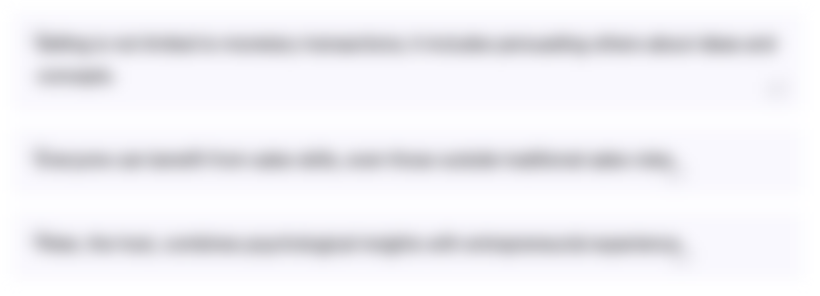
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレードTranscripts
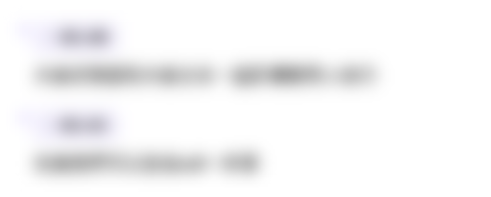
このセクションは有料ユーザー限定です。 アクセスするには、アップグレードをお願いします。
今すぐアップグレード5.0 / 5 (0 votes)